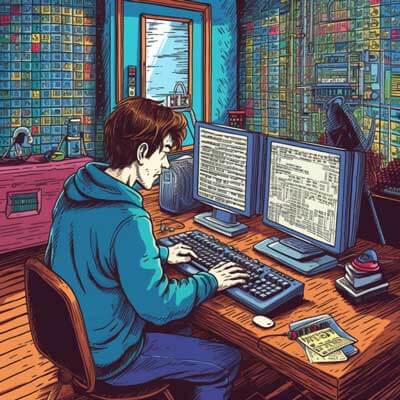
Table of Contents
What is the Go programming language?
The Go programming language, also known as Golang, is a statically typed, compiled language developed by Google. It was designed to provide a simple and efficient way to write reliable and scalable software. Go has gained popularity among software engineers for its simplicity, performance, and built-in support for concurrency.
Related Article: Applying Design Patterns with Gin and Golang
What is the Gin framework?
Gin is a lightweight web framework for Go that provides a fast and flexible way to build web applications. It is built on top of the net/http package in Go's standard library and offers a simple and intuitive API for handling HTTP requests and responses. Gin is known for its high performance and low memory footprint, making it a popular choice among developers for building APIs and web services.
How can Go libraries be used to add i18n support in Gin applications?
Go provides several libraries that can be used to add internationalization (i18n) support to Gin applications. One popular library is go-i18n, which provides a simple and efficient way to manage translations in multiple languages. Here's an example of how you can use go-i18n in a Gin application:
First, install the go-i18n library using the following command:
go get github.com/nicksnyder/go-i18n/v2/i18n
Next, create a translations directory in your project's root directory and add translation files for each supported language. For example, you can create a translations/en-us.all.json file for English (US) translations and a translations/es-es.all.json file for Spanish (Spain) translations.
In your Gin application, load the translation files and set the language based on the user's preferences. Here's an example:
package main import ( "github.com/gin-gonic/gin" "github.com/nicksnyder/go-i18n/v2/i18n" "golang.org/x/text/language" ) func main() { r := gin.Default() // Load translation files bundle := i18n.NewBundle(language.English) bundle.RegisterUnmarshalFunc("json", json.Unmarshal) err := bundle.LoadMessageFile("translations/en-us.all.json") if err != nil { panic(err) } // Set language based on user's preferences r.Use(func(c *gin.Context) { acceptLanguage := c.GetHeader("Accept-Language") lang, _ := language.MatchStrings(bundle.LanguageTags(), acceptLanguage) localizer := i18n.NewLocalizer(bundle, lang) c.Set("Localizer", localizer) c.Next() }) // Example route r.GET("/hello", func(c *gin.Context) { localizer := c.MustGet("Localizer").(*i18n.Localizer) message := localizer.MustLocalize(&i18n.LocalizeConfig{ MessageID: "hello", }) c.String(http.StatusOK, message) }) r.Run(":8080") }
In this example, we create a bundle and register the translation files using the go-i18n library. We then set the language based on the user's preferences using the Accept-Language header. Finally, we use the localizer to retrieve and display translated messages in our Gin routes.
What is character encoding and how is it handled in Gin?
Character encoding is the process of representing characters in a digital form. In web applications, character encoding is important for handling different languages, special characters, and character sets.
In Gin, character encoding is handled automatically by the net/http package in Go's standard library. By default, Gin uses UTF-8 encoding, which is a widely-used character encoding standard that supports a wide range of characters from different languages.
When handling HTTP requests, Gin automatically decodes the request body and URL parameters using the UTF-8 encoding. Similarly, when sending response bodies, Gin automatically encodes the data using the UTF-8 encoding.
Here's an example of how Gin handles character encoding:
package main import ( "github.com/gin-gonic/gin" ) func main() { r := gin.Default() // Example route with URL parameters r.GET("/hello/:name", func(c *gin.Context) { name := c.Param("name") c.String(http.StatusOK, "Hello, %s!", name) }) r.Run(":8080") }
In this example, we define a route with a URL parameter named "name". When a request is made to this route, Gin automatically decodes the URL parameter using the UTF-8 encoding and passes it to our route handler function.
Related Article: Enterprise Functionalities in Golang: SSO, RBAC and Audit Trails in Gin
What is UTF-8 and why is it important for multi-language support?
UTF-8 (Unicode Transformation Format 8-bit) is a variable-width character encoding standard that can represent any character in the Unicode standard. It is widely used and supported by modern systems and applications.
UTF-8 is important for multi-language support because it allows us to represent characters from different languages and character sets in a single encoding. This means that we can store and transmit text data in multiple languages without the need for separate encodings or character sets.
In the context of Gin applications, UTF-8 encoding is important for handling multi-language content, such as internationalized strings and user-generated text. By using UTF-8 encoding, Gin can handle and display text data from different languages and character sets correctly.
How can locale-specific URLs be generated in Gin?
Generating locale-specific URLs in Gin can be done by using the built-in support for route groups and URL parameters. Here's an example of how you can generate locale-specific URLs in Gin:
package main import ( "github.com/gin-gonic/gin" ) func main() { r := gin.Default() // Route group for English (US) enGroup := r.Group("/en-us") { enGroup.GET("/hello", func(c *gin.Context) { c.String(http.StatusOK, "Hello!") }) } // Route group for Spanish (Spain) esGroup := r.Group("/es-es") { esGroup.GET("/hola", func(c *gin.Context) { c.String(http.StatusOK, "¡Hola!") }) } r.Run(":8080") }
In this example, we define two route groups, one for English (US) and one for Spanish (Spain). Each route group has its own base URL ("/en-us" and "/es-es") and contains routes specific to that locale.
What is content negotiation and how is it implemented in Gin?
Content negotiation is the process of selecting the appropriate representation of a resource based on the client's preferences and capabilities. In the context of web applications, content negotiation is important for handling different content types, such as HTML, JSON, XML, and others.
In Gin, content negotiation can be implemented using the gin.Context.Negotiate
method. This method takes a list of preferred content types and returns the most appropriate representation based on the client's preferences. Here's an example of how content negotiation can be implemented in Gin:
package main import ( "github.com/gin-gonic/gin" ) func main() { r := gin.Default() r.GET("/data", func(c *gin.Context) { c.Negotiate(gin.Negotiate{ Offered: []string{"application/json", "application/xml"}, Data: gin.H{ "message": "Hello, World!", }, }) }) r.Run(":8080") }
In this example, we define a route that returns data in either JSON or XML format based on the client's preferences. We use the gin.Context.Negotiate
method to handle content negotiation and pass a list of preferred content types ("application/json" and "application/xml") and the data to be returned.
Gin automatically selects the most appropriate representation based on the client's preferences and returns the corresponding data. If the client does not specify a preferred content type, Gin uses the first content type in the list as the default.
Which Go libraries are commonly used for internationalization?
There are several Go libraries commonly used for internationalization (i18n) in Go applications. Some popular ones include:
- go-i18n: A useful and flexible i18n library that provides translation management and localization features. It supports multiple translation file formats and provides an easy-to-use API for handling i18n in Go applications.
- gotext: A lightweight i18n library that focuses on simplicity and ease of use. It provides a simple API for managing translations and supports multiple translation file formats.
- go-localize: A comprehensive i18n library that supports translation management, localization, and date/time formatting. It provides a wide range of features and supports multiple translation file formats.
These libraries provide different features and capabilities, so it's important to choose the one that best fits the requirements of your project.
Related Article: Optimizing and Benchmarking Beego ORM in Golang
How can multi-language support be implemented in a Gin application?
Multi-language support can be implemented in a Gin application by using Go libraries for internationalization (i18n) and following best practices for handling translations and localized content. Here's an example of how multi-language support can be implemented in a Gin application:
1. Choose an i18n library: Select a Go i18n library that best fits the requirements of your project, such as go-i18n, gotext, or go-localize.
2. Set up translation files: Create translation files for each supported language and store them in a translations directory. These files should contain key-value pairs that map translation IDs to translated strings.
3. Load translation files: In your Gin application, load the translation files using the chosen i18n library. This typically involves registering the translation files and setting the default language.
4. Set language based on user preferences: Use the Accept-Language header or other means to determine the user's preferred language. Set the language in the Gin context or request object for subsequent requests.
5. Retrieve and display translated content: Use the i18n library to retrieve translated strings based on their translation IDs. Display the translated content in your Gin routes and views.
What are the benefits of using the Gin framework for internationalization and encoding?
Using the Gin framework for internationalization and encoding in your Go applications offers several benefits:
- Simplicity and ease of use: Gin provides a simple and intuitive API for handling HTTP requests and responses, making it easy to implement internationalization and encoding features in your application.
- Performance: Gin is known for its high performance and low memory footprint, making it well-suited for handling internationalized content and character encoding.
- Flexibility: Gin allows you to easily organize and manage routes, route groups, and middleware, making it flexible for implementing internationalization and encoding features.
- Compatibility: Gin is built on top of the net/http package in Go's standard library, which provides excellent support for internationalization and encoding out of the box. This ensures compatibility with other Go libraries and tools.
- Community and ecosystem: Gin has a large and active community of developers, which means you can find plenty of resources, tutorials, and libraries to support your internationalization and encoding needs.
Overall, using the Gin framework for internationalization and encoding can help simplify the development process and improve the performance and flexibility of your Go applications.
Additional Resources
- Gin i18n Middleware