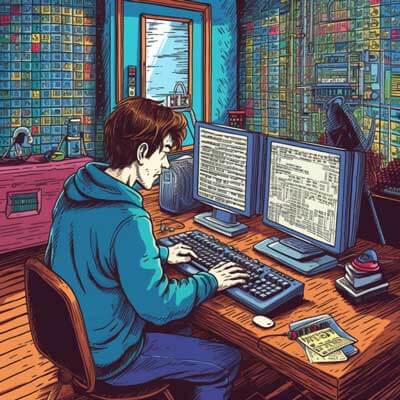
Table of Contents
Handling onClick Events in ReactJS Child Components
In ReactJS, handling events is a crucial aspect of building interactive user interfaces. The onClick event is one of the most commonly used events in React, as it allows you to respond to user clicks on various elements in your application. When it comes to handling onClick events in child components, there are a few different approaches you can take.
One approach is to define an onClick event handler directly in the child component itself. This allows the child component to handle the event independently, without relying on the parent component. Here's an example of how you can implement this:
import React from 'react';const ChildComponent = () => { const handleClick = () => { console.log('Button clicked!'); }; return ( <button onClick={handleClick}>Click Me</button> );};export default ChildComponent;
In this example, we define a handleClick function within the ChildComponent. This function will be called whenever the button is clicked. We then pass this function as the onClick prop of the button element. When the button is clicked, the handleClick function will be executed, and the message "Button clicked!" will be logged to the console.
Another approach is to define the onClick event handler in the parent component and pass it down to the child component as a prop. This allows the parent component to control the event handling logic, while still allowing the child component to trigger the event. Here's an example:
import React from 'react';import ChildComponent from './ChildComponent';const ParentComponent = () => { const handleClick = () => { console.log('Button clicked!'); }; return ( <div> <h1>Parent Component</h1> <ChildComponent onClick={handleClick} /> </div> );};export default ParentComponent;
In this example, we define the handleClick function in the ParentComponent and pass it down to the ChildComponent as the onClick prop. Inside the ChildComponent, we can access this prop and attach it to the button element as the onClick event handler. When the button is clicked, the handleClick function defined in the parent component will be executed.
Related Article: Sharing Variables Between Components in ReactJS
Passing onClick Events from Parent to Child Components
Passing onClick events from parent to child components in ReactJS is a common pattern that allows for better separation of concerns and reusability of components. By passing down event handlers as props, you can control the event handling logic from a higher-level component while still allowing child components to trigger the events.
To pass an onClick event from a parent component to a child component, you simply define the event handler in the parent component and pass it down to the child component as a prop. Here's an example:
import React from 'react';import ChildComponent from './ChildComponent';const ParentComponent = () => { const handleClick = () => { console.log('Button clicked!'); }; return ( <div> <h1>Parent Component</h1> <ChildComponent onClick={handleClick} /> </div> );};export default ParentComponent;
In this example, we define the handleClick function in the ParentComponent. We then pass it down to the ChildComponent as the onClick prop. Inside the ChildComponent, we can access this prop and attach it to the button element as the onClick event handler. When the button is clicked, the handleClick function defined in the parent component will be executed.
Now let's take a look at how the ChildComponent can receive and use the onClick prop:
import React from 'react';const ChildComponent = ({ onClick }) => { return ( <button onClick={onClick}>Click Me</button> );};export default ChildComponent;
In the ChildComponent, we destructure the onClick prop from the props object. We then attach it to the button element as the onClick event handler. When the button is clicked, the onClick prop passed down from the parent component will be executed.
This pattern of passing onClick events from parent to child components is not only useful for handling events but also for passing data or any other functionality between components. It promotes reusability and separation of concerns, making your code more maintainable and easier to understand.
The Purpose of onClick Event in ReactJS
In ReactJS, the onClick event is used to handle user clicks on elements within your application. It allows you to respond to user interactions and trigger specific actions or functions based on those interactions.
The purpose of the onClick event in ReactJS is to provide a way for you to define what happens when a user clicks on a certain element. This can include things like submitting a form, navigating to a different page, toggling the visibility of an element, or updating the state of a component.
The onClick event is not limited to a specific type of element. It can be used with various HTML elements such as buttons, links, checkboxes, or any other element that can receive user input.
Here's an example of how the onClick event is used in ReactJS:
import React from 'react';const Button = () => { const handleClick = () => { console.log('Button clicked!'); }; return ( <button onClick={handleClick}>Click Me</button> );};export default Button;
In this example, we define a Button component that renders a button element. We attach the handleClick function to the onClick event of the button element. When the button is clicked, the handleClick function will be executed, and the message "Button clicked!" will be logged to the console.
The purpose of the onClick event is to provide a way for you to define custom behavior when a user interacts with your application. It allows you to create dynamic and interactive user interfaces, making your ReactJS applications more engaging and user-friendly.
Event Handling in ReactJS: Best Practices
When it comes to event handling in ReactJS, there are a few best practices that can help you write cleaner and more maintainable code. These best practices include:
1. Use arrow functions or bind methods in event handlers:
- Use arrow functions to define event handlers inline. This allows you to access the component's state and props directly without the need for binding.
- Alternatively, you can bind your event handlers in the constructor or use the bind method when passing them as props to child components.
2. Avoid using anonymous functions as event handlers:
- Instead of using anonymous functions directly in the event handler, define the function separately and pass it as a prop. This helps with code readability and makes it easier to test and reuse your event handlers.
3. Use event.preventDefault() when necessary:
- If you need to prevent the default behavior of an event, such as preventing a form from submitting or a link from navigating, use the event.preventDefault() method within your event handler.
4. Use synthetic events:
- ReactJS provides synthetic events that wrap native browser events and provide a consistent interface across different browsers. Use these synthetic events instead of native browser events whenever possible.
5. Consider debouncing or throttling event handlers:
- If you have event handlers that are triggered frequently, such as scroll or resize events, consider debouncing or throttling them to improve performance and prevent unnecessary function calls.
6. Keep event handlers small and focused:
- Event handlers should ideally be small and focused on handling the specific event they are attached to. If you find that your event handler is becoming too complex or handling multiple events, consider refactoring it into smaller, more manageable functions.
Related Article: Exploring Key Features of ReactJS
Binding Event Handlers in ReactJS Components
In ReactJS, binding event handlers is a common practice when working with class components. Binding allows you to ensure that the correct context is maintained when the event handler is called, so that you can access the component's state and props correctly.
There are a few different ways to bind event handlers in ReactJS components. Let's take a look at some examples:
1. Binding in the constructor:
- One way to bind event handlers in a ReactJS component is to bind them in the constructor. This ensures that the binding only happens once, during the component's initialization. Here's an example:
import React, { Component } from 'react';class MyComponent extends Component { constructor(props) { super(props); this.handleClick = this.handleClick.bind(this); } handleClick() { console.log('Button clicked!'); } render() { return ( <button onClick={this.handleClick}>Click Me</button> ); }}export default MyComponent;
In this example, we bind the handleClick event handler in the constructor using the bind method. This ensures that when the handleClick function is called, it will have the correct context and be able to access the component's state and props.
2. Binding inline with arrow functions:
- Another way to bind event handlers in ReactJS is to use arrow functions inline. Arrow functions automatically bind the current context, so you don't need to explicitly bind them. Here's an example:
import React from 'react';const MyComponent = () => { const handleClick = () => { console.log('Button clicked!'); }; return ( <button onClick={handleClick}>Click Me</button> );};export default MyComponent;
In this example, we define the handleClick event handler using an arrow function. Since arrow functions automatically bind the current context, we don't need to explicitly bind it. The handleClick function can access the component's state and props directly.
3. Binding with arrow functions in class properties:
- If you are using class properties syntax in your ReactJS components, you can bind event handlers directly in the class property declaration. Here's an example:
import React, { Component } from 'react';class MyComponent extends Component { handleClick = () => { console.log('Button clicked!'); }; render() { return ( <button onClick={this.handleClick}>Click Me</button> ); }}export default MyComponent;
In this example, we define the handleClick event handler as a class property using an arrow function. This allows us to bind the event handler directly within the class property declaration, without the need for a constructor.
Arrow Functions vs Binding in ReactJS Event Handling
When it comes to event handling in ReactJS, there are two main approaches: using arrow functions and binding event handlers. Both approaches have their own advantages and disadvantages, and the choice between them depends on the specific requirements of your application.
Using arrow functions for event handlers is a popular approach in ReactJS. Arrow functions automatically bind the current context, so you don't need to explicitly bind the event handler. This can make your code more concise and easier to read. Here's an example:
import React from 'react';const MyComponent = () => { const handleClick = () => { console.log('Button clicked!'); }; return ( <button onClick={handleClick}>Click Me</button> );};export default MyComponent;
In this example, we define the handleClick event handler using an arrow function. Since arrow functions automatically bind the current context, we don't need to explicitly bind it. The handleClick function can access the component's state and props directly.
On the other hand, binding event handlers in ReactJS allows you to explicitly bind the event handler to the component's context. This ensures that the correct context is maintained when the event handler is called, so you can access the component's state and props correctly. Here's an example:
import React, { Component } from 'react';class MyComponent extends Component { constructor(props) { super(props); this.handleClick = this.handleClick.bind(this); } handleClick() { console.log('Button clicked!'); } render() { return ( <button onClick={this.handleClick}>Click Me</button> ); }}export default MyComponent;
In this example, we bind the handleClick event handler in the constructor using the bind method. This ensures that when the handleClick function is called, it will have the correct context and be able to access the component's state and props.
The choice between arrow functions and binding depends on the specific requirements of your application. If you don't need to access the component's state or props within the event handler, arrow functions can provide a more concise syntax. However, if you do need to access the component's state or props, binding the event handler can ensure that the correct context is maintained.
Ultimately, the choice between arrow functions and binding comes down to personal preference and the specific needs of your application. Both approaches are valid and widely used in ReactJS event handling.
Accessing Child Component State in ReactJS
In ReactJS, accessing the state of a child component from a parent component can be achieved through the use of props. By passing down the state of the child component as a prop, the parent component can access and use the child component's state.
Let's take a look at an example to understand how to access the child component state in ReactJS:
import React, { useState } from 'react';const ChildComponent = () => { const [count, setCount] = useState(0); const incrementCount = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button onClick={incrementCount}>Increment</button> </div> );};const ParentComponent = () => { return ( <div> <h1>Parent Component</h1> <ChildComponent /> </div> );};export default ParentComponent;
In this example, we have a ChildComponent that maintains its own state using the useState hook. It has a count state variable and an incrementCount function that updates the count state when the button is clicked.
The ParentComponent renders the ChildComponent and has access to its state. However, since the state is encapsulated within the ChildComponent, the ParentComponent cannot directly access or modify the state.
To access the child component state from the parent component, we can pass the state as a prop from the child to the parent. Here's an updated version of the example:
import React, { useState } from 'react';const ChildComponent = ({ count, incrementCount }) => { return ( <div> <p>Count: {count}</p> <button onClick={incrementCount}>Increment</button> </div> );};const ParentComponent = () => { const [count, setCount] = useState(0); const incrementCount = () => { setCount(count + 1); }; return ( <div> <h1>Parent Component</h1> <ChildComponent count={count} incrementCount={incrementCount} /> </div> );};export default ParentComponent;
In this updated example, we pass the count state and the incrementCount function from the ParentComponent to the ChildComponent as props. The ChildComponent can now access and use these props to display the count and trigger the incrementCount function.
Understanding the Component Lifecycle Method for Event Handling
In ReactJS, event handling is an important aspect of building interactive user interfaces. When it comes to managing event handling in a ReactJS component, understanding the component lifecycle methods can be beneficial.
The component lifecycle methods are special functions that are automatically called at different stages of a component's lifecycle. They provide hooks for you to perform certain actions or operations at specific points in the component's lifespan.
There are several lifecycle methods that can be used for event handling in ReactJS, but one important method is componentDidMount(). This method is called after the component has been rendered for the first time and is often used for setting up event listeners.
Let's take a look at an example to understand how componentDidMount() can be used for event handling:
import React, { Component } from 'react';class MyComponent extends Component { componentDidMount() { window.addEventListener('resize', this.handleResize); } componentWillUnmount() { window.removeEventListener('resize', this.handleResize); } handleResize() { console.log('Window resized!'); } render() { return ( <div> <h1>My Component</h1> </div> ); }}export default MyComponent;
In this example, we define a class component called MyComponent. Inside the componentDidMount() method, we use the addEventListener() method to attach an event listener for the window's resize event. We pass in the handleResize() method as the event handler.
When the component is initially rendered, the componentDidMount() method is called, and the event listener is set up. This allows the component to respond to the window's resize event.
To clean up the event listener when the component is unmounted from the DOM, we use the componentWillUnmount() method. Inside this method, we use the removeEventListener() method to remove the event listener for the window's resize event.
Understanding the component lifecycle methods and how they can be used for event handling in ReactJS is essential for building robust and well-structured applications.
Related Article: How to Render ReactJS Code with NPM
Implementing Event Delegation in ReactJS Child Components
Event delegation is a technique in ReactJS that allows you to handle events on multiple elements using a single event listener. Instead of attaching event listeners to individual elements, you attach a single event listener to a parent element and use event.target to determine which child element triggered the event.
Implementing event delegation in ReactJS child components can be done by attaching the event listener to a parent component and using the event.target property to identify the child component that triggered the event.
Let's take a look at an example to understand how event delegation can be implemented in ReactJS child components:
import React from 'react';const ParentComponent = () => { const handleClick = (event) => { if (event.target.tagName === 'BUTTON') { console.log('Button clicked!'); } }; return ( <div onClick={handleClick}> <button>Button 1</button> <button>Button 2</button> <button>Button 3</button> </div> );};export default ParentComponent;
In this example, we have a ParentComponent that renders three buttons within a div element. The onClick event listener is attached to the div element, and the handleClick function is called whenever a click event occurs within the div.
Inside the handleClick function, we use the event.target property to determine which element triggered the event. In this case, we check if the tag name of the event.target is 'BUTTON'. If it is, we log the message 'Button clicked!' to the console.
Event delegation is especially useful when you have a large number of similar elements that require event handling. Instead of attaching event listeners to each individual element, you can attach a single event listener to a parent component and use event.target to determine the specific child component that triggered the event.
Triggering Functions on Click in ReactJS Child Components
In ReactJS, triggering functions on click events in child components is a common task when building interactive user interfaces. There are several ways to achieve this, depending on the specific requirements of your application.
One approach is to define the function directly within the child component and attach it to the onClick event of the element that needs to trigger the function. Here's an example:
import React from 'react';const ChildComponent = () => { const handleClick = () => { console.log('Button clicked!'); }; return ( <button onClick={handleClick}>Click Me</button> );};export default ChildComponent;
In this example, we define the handleClick function within the ChildComponent. We then attach this function to the onClick event of the button element. When the button is clicked, the handleClick function will be executed, and the message "Button clicked!" will be logged to the console.
Another approach is to pass the function as a prop from the parent component to the child component. This allows the parent component to control the function that is triggered on click. Here's an example:
import React from 'react';const ChildComponent = ({ handleClick }) => { return ( <button onClick={handleClick}>Click Me</button> );};export default ChildComponent;
In this example, we destructure the handleClick prop from the props object in the ChildComponent. We then attach this prop to the onClick event of the button element. When the button is clicked, the handleClick function passed from the parent component will be executed.
Additional Resources
- ReactJS Tutorial - W3Schools