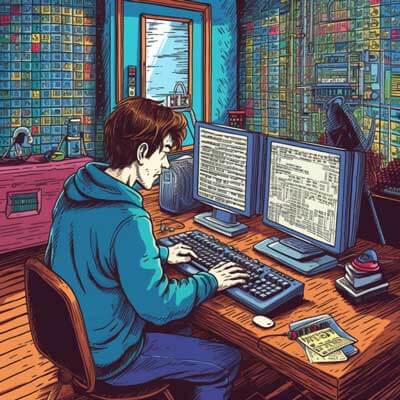
Table of Contents
Gin is a lightweight web framework for building web applications in Golang. It provides a robust set of features and performs exceptionally well in terms of speed and efficiency. In this article, we will explore how to implement real-time features and WebSockets in Gin, enabling bidirectional communication, building real-time chat systems, notifications, live dashboards, managing connection state, and scaling WebSockets.
Bidirectional Communication with WebSockets in Gin
WebSockets are a useful technology that allows bidirectional communication between a client and a server over a single, long-lived connection. This makes them ideal for real-time applications that require instant updates and responsiveness.
To implement WebSockets in Gin, we need to first establish a WebSocket connection between the client and the server. Gin provides a simple and intuitive way to handle WebSocket connections using the gin-gonic/gin
package.
Here's an example of how to handle a WebSocket connection in Gin:
package main import ( "github.com/gin-gonic/gin" "github.com/gorilla/websocket" "net/http" ) var upgrader = websocket.Upgrader{ CheckOrigin: func(r *http.Request) bool { // Allow all connections return true }, } func main() { r := gin.Default() r.GET("/ws", func(c *gin.Context) { conn, err := upgrader.Upgrade(c.Writer, c.Request, nil) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{ "error": err.Error(), }) return } // Handle WebSocket connection go handleWebSocketConnection(conn) }) r.Run(":8080") } func handleWebSocketConnection(conn *websocket.Conn) { for { // Read message from the client _, message, err := conn.ReadMessage() if err != nil { conn.Close() break } // Process the message // ... // Send response to the client err = conn.WriteMessage(websocket.TextMessage, []byte("Message received")) if err != nil { conn.Close() break } } }
In this example, we define a /ws
route that handles WebSocket connections. When a client requests this route, the server upgrades the HTTP connection to a WebSocket connection using the upgrader.Upgrade()
method. We then pass the WebSocket connection to the handleWebSocketConnection()
function, which reads messages from the client, processes them, and sends a response back.
This is a basic example, but you can extend it to implement more complex real-time features using WebSockets in Gin.
Related Article: Beego Integration with Bootstrap, Elasticsearch & Databases
Building Real-time Chat Systems in Gin
Real-time chat systems are a common use case for WebSockets. With Gin, you can easily build a real-time chat system that allows users to send and receive messages in real-time.
Here's an example of how to build a simple real-time chat system using WebSockets in Gin:
package main import ( "github.com/gin-gonic/gin" "github.com/gorilla/websocket" "net/http" ) var upgrader = websocket.Upgrader{ CheckOrigin: func(r *http.Request) bool { // Allow all connections return true }, } type Message struct { Username string `json:"username"` Text string `json:"text"` } var clients = make(map[*websocket.Conn]bool) var broadcast = make(chan Message) func main() { r := gin.Default() r.GET("/ws", func(c *gin.Context) { conn, err := upgrader.Upgrade(c.Writer, c.Request, nil) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{ "error": err.Error(), }) return } clients[conn] = true go handleWebSocketConnection(conn) }) go handleBroadcast() r.Run(":8080") } func handleWebSocketConnection(conn *websocket.Conn) { for { var message Message err := conn.ReadJSON(&message) if err != nil { conn.Close() delete(clients, conn) break } broadcast <- message } } func handleBroadcast() { for { message := <-broadcast for client := range clients { err := client.WriteJSON(message) if err != nil { client.Close() delete(clients, client) } } } }
In this example, we maintain a map of WebSocket connections (clients
) and a channel (broadcast
) to send messages to all connected clients. When a client sends a message, the server reads it, adds it to the broadcast
channel, and then broadcasts it to all connected clients. The clients then receive the message and update their chat interfaces accordingly.
This is a basic example, but you can enhance it by adding features like user authentication, message history, and private messaging.
Implementing Notifications using WebSockets in Gin
Notifications are another common use case for WebSockets. With Gin, you can easily implement real-time notifications that can be pushed from the server to the client instantly.
Here's an example of how to implement notifications using WebSockets in Gin:
package main import ( "github.com/gin-gonic/gin" "github.com/gorilla/websocket" "net/http" ) var upgrader = websocket.Upgrader{ CheckOrigin: func(r *http.Request) bool { // Allow all connections return true }, } type Notification struct { Title string `json:"title"` Message string `json:"message"` } var clients = make(map[*websocket.Conn]bool) func main() { r := gin.Default() r.GET("/ws", func(c *gin.Context) { conn, err := upgrader.Upgrade(c.Writer, c.Request, nil) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{ "error": err.Error(), }) return } clients[conn] = true go handleWebSocketConnection(conn) }) r.POST("/notification", func(c *gin.Context) { var notification Notification err := c.BindJSON(¬ification) if err != nil { c.JSON(http.StatusBadRequest, gin.H{ "error": err.Error(), }) return } sendNotification(notification) }) r.Run(":8080") } func handleWebSocketConnection(conn *websocket.Conn) { for { _, _, err := conn.ReadMessage() if err != nil { conn.Close() delete(clients, conn) break } } } func sendNotification(notification Notification) { for client := range clients { err := client.WriteJSON(notification) if err != nil { client.Close() delete(clients, client) } } }
In this example, we have a /notification
route that accepts POST requests and sends notifications to all connected clients. When a client sends a POST request to this route with a JSON payload containing the title and message of the notification, the server broadcasts it to all connected clients using the sendNotification()
function.
You can customize this example to include additional information in the notifications, such as the sender's name or a timestamp.
Creating Live Dashboards in Gin
Live dashboards are a valuable tool for monitoring and visualizing real-time data. With Gin, you can create live dashboards that update in real-time using WebSockets.
Here's an example of how to create a simple live dashboard in Gin:
package main import ( "github.com/gin-gonic/gin" "github.com/gorilla/websocket" "net/http" "time" ) var upgrader = websocket.Upgrader{ CheckOrigin: func(r *http.Request) bool { // Allow all connections return true }, } type DataPoint struct { Time time.Time `json:"time"` Value float64 `json:"value"` } var clients = make(map[*websocket.Conn]bool) func main() { r := gin.Default() r.GET("/ws", func(c *gin.Context) { conn, err := upgrader.Upgrade(c.Writer, c.Request, nil) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{ "error": err.Error(), }) return } clients[conn] = true go handleWebSocketConnection(conn) }) go generateDataPoints() r.Run(":8080") } func handleWebSocketConnection(conn *websocket.Conn) { for { _, _, err := conn.ReadMessage() if err != nil { conn.Close() delete(clients, conn) break } } } func generateDataPoints() { for { value := rand.Float64() * 100 dataPoint := DataPoint{ Time: time.Now(), Value: value, } for client := range clients { err := client.WriteJSON(dataPoint) if err != nil { client.Close() delete(clients, client) } } time.Sleep(1 * time.Second) } }
In this example, we generate random data points every second and send them to all connected clients. Clients receive the data points and update their dashboard interfaces accordingly.
You can customize this example by using real-time data from external sources or by implementing more sophisticated data visualization techniques.
Related Article: Applying Design Patterns with Gin and Golang
Managing Connection State with WebSockets in Gin
Managing connection state is crucial when working with WebSockets. In Gin, you can manage the connection state of WebSocket clients using a combination of maps and channels.
Here's an example of how to manage connection state with WebSockets in Gin:
package main import ( "github.com/gin-gonic/gin" "github.com/gorilla/websocket" "net/http" ) var upgrader = websocket.Upgrader{ CheckOrigin: func(r *http.Request) bool { // Allow all connections return true }, } type Client struct { Connection *websocket.Conn Send chan []byte } var clients = make(map[*Client]bool) var broadcast = make(chan []byte) func main() { r := gin.Default() r.GET("/ws", func(c *gin.Context) { conn, err := upgrader.Upgrade(c.Writer, c.Request, nil) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{ "error": err.Error(), }) return } client := &Client{ Connection: conn, Send: make(chan []byte), } clients[client] = true go handleWebSocketConnection(client) }) go handleBroadcast() r.Run(":8080") } func handleWebSocketConnection(client *Client) { defer func() { client.Connection.Close() delete(clients, client) }() for { _, message, err := client.Connection.ReadMessage() if err != nil { break } broadcast <- message } } func handleBroadcast() { for { message := <-broadcast for client := range clients { select { case client.Send <- message: default: close(client.Send) delete(clients, client) } } } }
In this example, we define a Client
struct that represents a WebSocket client and includes a channel (Send
) for sending messages to the client. We maintain a map of clients (clients
) and a channel (broadcast
) for broadcasting messages to all connected clients. When a client sends a message, the server adds it to the broadcast
channel, and the handleBroadcast()
function broadcasts it to all connected clients through their respective Send
channels.
This approach allows you to manage the connection state of WebSocket clients more efficiently and handle cases where clients are no longer reachable or when their Send
channels become full.
Scaling WebSockets in Gin
Scaling WebSockets in Gin can be achieved by leveraging load balancers and distributing WebSocket connections across multiple instances of your Gin application.
To scale WebSocket connections in Gin, you can use a load balancer such as Nginx or HAProxy to distribute incoming WebSocket connections across multiple instances of your Gin application. Each instance of the Gin application will handle a subset of the WebSocket connections, allowing you to handle a larger number of concurrent connections.
Here's an example of how to scale WebSockets in Gin using Nginx as a load balancer:
1. Set up multiple instances of your Gin application on different servers or virtual machines.
2. Install and configure Nginx on a separate server.
3. Configure Nginx to proxy WebSocket connections to the instances of your Gin application. Here's an example Nginx configuration:
http { ... upstream gin_app { server <gin_app_instance_1>; server <gin_app_instance_2>; server <gin_app_instance_3>; } server { ... location /ws { proxy_pass http://gin_app; proxy_http_version 1.1; proxy_set_header Upgrade $http_upgrade; proxy_set_header Connection "Upgrade"; } } }
In this example, we define an upstream
block with the addresses of the instances of the Gin application. We then configure the /ws
location to proxy WebSocket connections to the gin_app
upstream.
4. Start Nginx and verify that WebSocket connections are being distributed across the instances of your Gin application.
Best Practices for Using WebSockets in Golang
When using WebSockets in Golang, there are some best practices you should follow to ensure the security, performance, and reliability of your applications.
1. Use Goroutines: Goroutines are lightweight threads in Golang that allow you to handle multiple WebSocket connections concurrently. By using Goroutines, you can handle a large number of WebSocket connections efficiently.
2. Use Channels for Communication: Channels are a useful mechanism in Golang that enable safe communication between Goroutines. Use channels to send and receive messages between your WebSocket handlers and other parts of your application.
3. Use a Gorilla WebSocket Library: The Gorilla WebSocket library is a popular choice for working with WebSockets in Golang. It provides a high-level API for handling WebSocket connections and includes features like event-driven message handling, connection pooling, and more.
4. Secure Your WebSockets: When deploying WebSockets in production, make sure to secure your connections using SSL/TLS encryption. This ensures that your WebSocket traffic is encrypted and secure from eavesdropping and tampering.
5. Implement Connection State Management: Keep track of the connection state of your WebSocket clients and handle cases where connections are closed unexpectedly or become inactive. This helps you maintain a clean and reliable WebSocket infrastructure.
6. Implement Rate Limiting: To prevent abuse and ensure fair usage, consider implementing rate limiting for your WebSocket connections. This helps protect your server from excessive traffic and ensures a smooth experience for all users.
Advantages of Using Gin for Real-time Features
Gin is a lightweight web framework for building web applications in Golang. It offers several advantages when it comes to implementing real-time features and WebSockets:
1. Performance: Gin is known for its excellent performance and low memory footprint. It is designed to be fast and efficient, making it ideal for real-time applications that require high-performance and low-latency communication.
2. Ease of Use: Gin provides a simple and intuitive API for handling HTTP requests and WebSocket connections. It has a minimalistic syntax and a clear routing system, making it easy to understand and use.
3. Middleware Support: Gin has a useful middleware system that allows you to add functionality to your applications at various stages of the request/response cycle. You can use middleware to handle authentication, logging, error handling, and more, making it easy to implement common real-time features.
4. Scalability: Gin is designed to be scalable and can handle a large number of concurrent connections. It supports load balancing and can be easily deployed across multiple instances to handle high traffic loads.
5. Flexibility: Gin provides flexibility in terms of customization and extension. It allows you to easily integrate with other libraries and frameworks, making it suitable for a wide range of real-time applications.
Overall, using Gin for real-time features and WebSockets in Golang offers a combination of performance, ease of use, scalability, and flexibility, making it a great choice for building real-time applications.
Related Article: Golang & Gin Security: JWT Auth, Middleware, and Cryptography
Using WebSockets in Golang without Gin
While Gin provides a convenient and efficient way to implement WebSockets in Golang, you can also use the built-in net/http
package to work with WebSockets without using a web framework.
Here's an example of how to implement WebSockets in Golang without using Gin:
package main import ( "log" "net/http" "github.com/gorilla/websocket" ) var upgrader = websocket.Upgrader{ CheckOrigin: func(r *http.Request) bool { // Allow all connections return true }, } func main() { http.HandleFunc("/ws", handleWebSocketConnection) log.Fatal(http.ListenAndServe(":8080", nil)) } func handleWebSocketConnection(w http.ResponseWriter, r *http.Request) { conn, err := upgrader.Upgrade(w, r, nil) if err != nil { log.Println(err) return } for { _, message, err := conn.ReadMessage() if err != nil { conn.Close() break } // Process the message // ... // Send response to the client err = conn.WriteMessage(websocket.TextMessage, []byte("Message received")) if err != nil { conn.Close() break } } }
In this example, we define a /ws
route using the http.HandleFunc()
function. When a client requests this route, the server upgrades the HTTP connection to a WebSocket connection using the upgrader.Upgrade()
method. We then handle the WebSocket connection in the handleWebSocketConnection()
function, which reads messages from the client, processes them, and sends a response back.
This approach allows you to work directly with the net/http
package and provides more control and flexibility over your WebSocket implementation.
Alternatives to Gin for Real-time Applications in Golang
While Gin is a popular choice for building web applications with real-time features in Golang, there are also other frameworks and libraries available that can be used to achieve similar results. Some alternatives to Gin for real-time applications in Golang include:
1. Echo: Echo is a fast and minimalist web framework for Golang that provides a simple and flexible API for building web applications. It is known for its speed and performance, making it suitable for real-time applications.
2. Iris: Iris is a feature-rich web framework for Golang that offers a wide range of functionality for building web applications. It provides support for WebSockets and real-time features, making it a good choice for real-time applications with complex requirements.
3. Revel: Revel is a full-stack web framework for Golang that follows the Model-View-Controller (MVC) pattern. It provides a comprehensive set of features for building web applications, including support for WebSockets and real-time communication.
4. Gorilla WebSocket: Gorilla WebSocket is a library for building WebSocket applications in Golang. It provides a high-level API for handling WebSocket connections and includes features like event-driven message handling, connection pooling, and more.
These alternatives offer different features, performance characteristics, and levels of abstraction, allowing you to choose the one that best fits your specific requirements for real-time applications in Golang.
Additional Resources
- Introduction to Gin - Go Web Framework