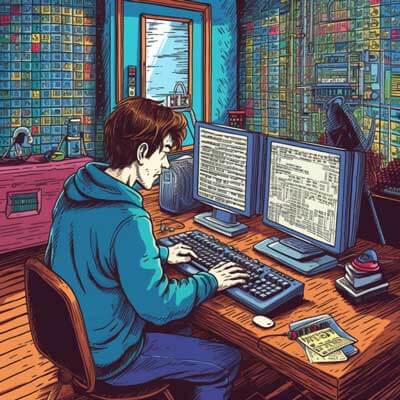
Table of Contents
What is the difference between a JavaScript class and a component?
In JavaScript, a class is a blueprint for creating objects with predefined properties and methods. It defines the structure and behavior of an object but does not directly render anything on the screen. On the other hand, a component is a reusable, self-contained piece of code that may include HTML, CSS, and JavaScript. A component represents a part of the user interface and can be rendered on the screen.
While both classes and components are used to organize and structure code, they serve different purposes. Classes focus on creating objects and defining their behavior, while components focus on creating reusable UI elements. In JavaScript, classes can be used to create components, but not all classes are components.
Related Article: Server-side rendering (SSR) Basics in Next.js
How to define a class in JavaScript
In JavaScript, a class can be defined using the class
keyword followed by the name of the class. Here's an example:
class Rectangle { constructor(width, height) { this.width = width; this.height = height; } getArea() { return this.width * this.height; } }
In the above example, we define a class called Rectangle
with a constructor
method and a getArea
method. The constructor
method is called when a new instance of the class is created and is used to set initial values for the object's properties. The getArea
method calculates and returns the area of the rectangle.
What is a constructor function in JavaScript?
A constructor function is a special method in a class that is called when a new instance of the class is created using the new
keyword. It is used to initialize the object's properties and perform any setup that is required. In JavaScript, the constructor function is defined using the constructor
keyword.
Here's an example of a constructor function:
class Person { constructor(name, age) { this.name = name; this.age = age; } }
In the above example, the Person
class has a constructor function that takes two parameters (name
and age
). When a new instance of the Person
class is created, the constructor function is called with the provided arguments, and the object's name
and age
properties are set accordingly.
How to create an instance of a class in JavaScript
To create an instance of a class in JavaScript, we use the new
keyword followed by the class name and any required arguments for the constructor function. Here's an example:
const rectangle = new Rectangle(10, 5);
In the above example, we create a new instance of the Rectangle
class with a width of 10 and a height of 5. The new
keyword calls the constructor function of the class and returns a new object that is an instance of the class. We can then assign this object to a variable (rectangle
in this case) to use it in our code.
Related Article: Advanced Node.js: Event Loop, Async, Buffer, Stream & More
Understanding the concept of inheritance in JavaScript classes
Inheritance is a fundamental concept in object-oriented programming that allows a class to inherit properties and methods from another class. In JavaScript, classes can inherit from other classes using the extends
keyword. This creates a parent-child relationship between the classes, where the child class inherits the properties and methods of the parent class.
Here's an example of inheritance in JavaScript classes:
class Animal { constructor(name) { this.name = name; } speak() { console.log(`${this.name} is speaking.`); } } class Dog extends Animal { breed; constructor(name, breed) { super(name); this.breed = breed; } bark() { console.log(`${this.name} is barking.`); } }
In the above example, we have a parent class called Animal
and a child class called Dog
that extends the Animal
class using the extends
keyword. The Dog
class has its own properties (breed
) and methods (bark
), and it also inherits the name
property and speak
method from the Animal
class using the super
keyword.
Exploring encapsulation in JavaScript classes
Encapsulation is the practice of bundling data and methods together within a class to hide the internal details and provide a clean and organized interface for interacting with the class. In JavaScript classes, encapsulation can be achieved by using the constructor
function and defining properties and methods within the class.
Here's an example of encapsulation in JavaScript classes:
class Counter { #count = 0; constructor() { this.#count = 0; } getCount() { return this.#count; } increment() { this.#count++; } decrement() { this.#count--; } }
In the above example, the Counter
class encapsulates a private count
property using the private field syntax (#count
). The getCount
method provides access to the value of the count
property, while the increment
and decrement
methods modify the value of the count
property. By encapsulating the count
property and providing methods to interact with it, we can control how the property is accessed and modified from outside the class.
Adding methods to a JavaScript class
Methods can be added to a JavaScript class by defining them within the class. A method is a function that is associated with an object and can be called on instances of the class. Methods can perform actions, manipulate data, and return values.
Here's an example of adding methods to a JavaScript class:
class Circle { radius; constructor(radius) { this.radius = radius; } getArea() { return Math.PI * this.radius ** 2; } getCircumference() { return 2 * Math.PI * this.radius; } }
In the above example, the Circle
class has two methods: getArea
and getCircumference
. The getArea
method calculates and returns the area of the circle based on its radius, while the getCircumference
method calculates and returns the circumference of the circle. These methods can be called on instances of the Circle
class to perform calculations specific to each instance.
The role of the prototype in JavaScript classes
In JavaScript, the prototype is an object that is associated with every function and class. It acts as a blueprint for creating new objects and provides a way to share properties and methods between multiple objects.
When a new object is created from a class, it inherits properties and methods from the prototype of that class. This allows for efficient memory usage since the properties and methods are shared among all instances of the class.
Here's an example of the prototype in JavaScript classes:
class Person { name; constructor(name) { this.name = name; } sayHello() { console.log(`Hello, my name is ${this.name}.`); } } const person1 = new Person('Alice'); const person2 = new Person('Bob'); person1.sayHello(); // Output: Hello, my name is Alice. person2.sayHello(); // Output: Hello, my name is Bob.
In the above example, the Person
class has a name
property and a sayHello
method. When we create new instances of the Person
class (person1
and person2
), they inherit the sayHello
method from the prototype of the Person
class. This allows us to call the sayHello
method on each instance and get the expected output.
Related Article: How to Use Map Function on Objects in Javascript
An overview of static methods in JavaScript classes
In JavaScript classes, static methods are methods that are associated with the class itself, rather than with instances of the class. They are defined using the static
keyword and can be called directly on the class without the need to create an instance of the class.
Here's an example of a static method in a JavaScript class:
class MathUtils { static add(a, b) { return a + b; } static subtract(a, b) { return a - b; } } console.log(MathUtils.add(5, 3)); // Output: 8 console.log(MathUtils.subtract(10, 4)); // Output: 6
In the above example, the MathUtils
class has two static methods: add
and subtract
. These methods can be called directly on the class itself (MathUtils.add
and MathUtils.subtract
) without the need to create an instance of the class. Static methods are commonly used for utility functions that are not specific to any particular instance of the class.
Understanding class composition in JavaScript
Class composition is a design pattern in object-oriented programming that allows for the creation of complex objects by combining smaller, more manageable objects. It promotes code reuse, modularity, and flexibility by breaking down complex behavior into smaller, reusable parts.
In JavaScript, class composition can be achieved by creating instances of other classes within a class and using them as components to build more complex objects.
Here's an example of class composition in JavaScript:
class Engine { start() { console.log('Engine started.'); } stop() { console.log('Engine stopped.'); } } class Car { engine; constructor() { this.engine = new Engine(); } start() { this.engine.start(); console.log('Car started.'); } stop() { this.engine.stop(); console.log('Car stopped.'); } } const car = new Car(); car.start(); // Output: Engine started. Car started. car.stop(); // Output: Engine stopped. Car stopped.
In the above example, the Engine
class represents the engine of a car and has start
and stop
methods. The Car
class has an engine
property which is an instance of the Engine
class. The Car
class also has its own start
and stop
methods which delegate the start and stop functionality to the Engine
instance and perform additional actions specific to the Car
class.
Additional Resources
- Inheritance and JavaScript Classes Within Components
- Purpose of Constructor in JavaScript Class Within a Component