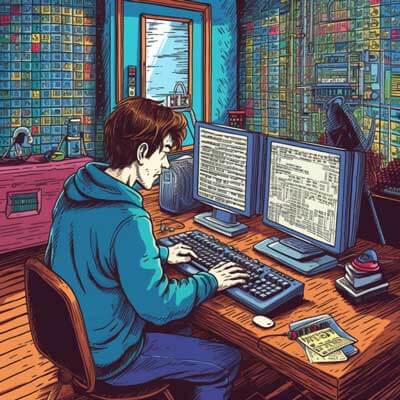
Table of Contents
The render method in ReactJS is responsible for rendering the component's user interface. It determines what gets displayed on the screen based on the component's current state and props. The render method is a crucial part of ReactJS as it allows developers to create dynamic and interactive UIs.
One of the key features of the render method is the ability to incorporate if statements. If statements allow developers to conditionally render different components or content based on certain conditions. This feature is essential for building responsive and customizable UIs in ReactJS.
Conditional Rendering in React
Conditional rendering refers to the process of displaying different content or components based on certain conditions. This allows developers to create dynamic UIs that can adapt to different scenarios or user interactions.
In React, conditional rendering can be achieved using if statements in the render method. By using if statements, developers can conditionally render different components or content depending on the values of variables or the state of the component.
Let's take a look at an example of conditional rendering in React:
import React from 'react'; class MyComponent extends React.Component { render() { const isLoggedIn = this.props.isLoggedIn; if (isLoggedIn) { return <h1>Welcome back!</h1>; } else { return <h1>Please log in.</h1>; } } }
In the above example, the render method checks the value of the isLoggedIn
prop. If it is true
, it renders the "Welcome back!" heading. Otherwise, it renders the "Please log in." heading. This allows the component to display different content based on whether the user is logged in or not.
Related Article: The Mechanisms Behind ReactJS’s High-Speed Performance
Rendering Based on Condition in React
To render different components based on a condition in React, you can use the conditional (ternary) operator. The conditional operator is a concise way to write if-else statements in a single line.
Here's an example of rendering based on a condition using the conditional operator:
import React from 'react'; class MyComponent extends React.Component { render() { const isLoggedIn = this.props.isLoggedIn; return ( <div> {isLoggedIn ? <h1>Welcome back!</h1> : <h1>Please log in.</h1>} </div> ); } }
In the above example, the render method uses the conditional operator to check the value of the isLoggedIn
prop. If it is true
, it renders the "Welcome back!" heading. Otherwise, it renders the "Please log in." heading. The conditional operator allows for concise and readable code when rendering based on a condition.
Using If Statements in React Render
In React, if statements can also be used directly in the render method to conditionally render different components or content. This provides more flexibility and allows for more complex conditions to be evaluated.
Here's an example of using if statements in the render method:
import React from 'react'; class MyComponent extends React.Component { render() { const isLoggedIn = this.props.isLoggedIn; if (isLoggedIn) { return <h1>Welcome back!</h1>; } else { return <h1>Please log in.</h1>; } } }
In the above example, the render method uses an if statement to check the value of the isLoggedIn
prop. If it is true
, it renders the "Welcome back!" heading. Otherwise, it renders the "Please log in." heading. Using if statements in the render method allows for more complex conditions to be evaluated and different components to be rendered based on those conditions.
Conditional Statements in ReactJS Render
In ReactJS, conditional statements in the render method allow developers to conditionally render different components or content based on certain conditions. This flexibility enables the creation of dynamic and interactive UIs.
Here's an example of using conditional statements in the render method:
import React from 'react'; class MyComponent extends React.Component { render() { const isLoggedIn = this.props.isLoggedIn; const isAdmin = this.props.isAdmin; if (isLoggedIn && isAdmin) { return <h1>Welcome back, Admin!</h1>; } else if (isLoggedIn) { return <h1>Welcome back!</h1>; } else { return <h1>Please log in.</h1>; } } }
In the above example, the render method uses conditional statements to check the values of the isLoggedIn
and isAdmin
props. If both conditions are true
, it renders the "Welcome back, Admin!" heading. If only the isLoggedIn
condition is true
, it renders the "Welcome back!" heading. If neither condition is true
, it renders the "Please log in." heading. Conditional statements in the render method allow for more complex conditions to be evaluated and different components to be rendered based on those conditions.
Related Article: How to Implement Hover State in ReactJS with Inline Styles
Rendering Components Conditionally in React
In React, components can also be conditionally rendered based on certain conditions. This allows for more granular control over the UI and enables the creation of dynamic and interactive components.
Here's an example of rendering components conditionally in React:
import React from 'react'; class MyComponent extends React.Component { render() { const isLoggedIn = this.props.isLoggedIn; return ( <div> {isLoggedIn ? <LoggedInComponent /> : <GuestComponent />} </div> ); } } class LoggedInComponent extends React.Component { render() { return <h1>Welcome back!</h1>; } } class GuestComponent extends React.Component { render() { return <h1>Please log in.</h1>; } }
In the above example, the render method conditionally renders the LoggedInComponent
or GuestComponent
based on the value of the isLoggedIn
prop. If it is true
, the LoggedInComponent
is rendered. Otherwise, the GuestComponent
is rendered. This allows for different components to be rendered based on certain conditions, providing more flexibility and control over the UI.
If-Else Statement in React Render
In React, if-else statements can be used in the render method to conditionally render different components or content based on certain conditions. This provides a straightforward way to handle multiple conditions and render different UI elements accordingly.
Here's an example of using if-else statements in the render method:
import React from 'react'; class MyComponent extends React.Component { render() { const isLoggedIn = this.props.isLoggedIn; const isAdmin = this.props.isAdmin; if (isLoggedIn && isAdmin) { return <h1>Welcome back, Admin!</h1>; } else if (isLoggedIn) { return <h1>Welcome back!</h1>; } else { return <h1>Please log in.</h1>; } } }
In the above example, the render method uses if-else statements to check the values of the isLoggedIn
and isAdmin
props. If both conditions are true
, it renders the "Welcome back, Admin!" heading. If only the isLoggedIn
condition is true
, it renders the "Welcome back!" heading. If neither condition is true
, it renders the "Please log in." heading. Using if-else statements in the render method allows for straightforward handling of multiple conditions and rendering different components or content based on those conditions.
Rendering Content Based on Condition in ReactJS
In ReactJS, content can be rendered based on a condition using if statements or other conditional rendering techniques. This allows developers to create dynamic and interactive UIs that can adapt to different scenarios or user interactions.
Here's an example of rendering content based on a condition in ReactJS:
import React from 'react'; class MyComponent extends React.Component { render() { const showContent = this.props.showContent; if (showContent) { return ( <div> <h1>Welcome!</h1> <p>This is some content that is conditionally rendered.</p> </div> ); } else { return null; } } }
In the above example, the render method checks the value of the showContent
prop. If it is true
, it renders a heading and a paragraph. If it is false
, it returns null
, indicating that no content should be rendered. This allows for the conditional rendering of content based on a specific condition.
Conditional Rendering with If Statements in React
Conditional rendering with if statements in React allows developers to conditionally render different components or content based on certain conditions. This provides a useful way to create dynamic UIs that can adapt to different scenarios or user interactions.
Here's an example of conditional rendering with if statements in React:
import React from 'react'; class MyComponent extends React.Component { render() { const isLoggedIn = this.props.isLoggedIn; if (isLoggedIn) { return ( <div> <h1>Welcome back!</h1> <p>This is some content that is conditionally rendered.</p> </div> ); } else { return null; } } }
In the above example, the render method uses an if statement to check the value of the isLoggedIn
prop. If it is true
, it renders a heading and a paragraph. If it is false
, it returns null
, indicating that no content should be rendered. Conditional rendering with if statements allows for the flexibility to render different components or content based on specific conditions.
Related Article: Handling Routing in React Apps with React Router
Rendering Different Components Based on Condition in React
In React, different components can be rendered based on a condition using if statements or other conditional rendering techniques. This allows for the creation of dynamic UIs that can adapt to different scenarios or user interactions.
Here's an example of rendering different components based on a condition in React:
import React from 'react'; class MyComponent extends React.Component { render() { const isAdmin = this.props.isAdmin; if (isAdmin) { return <AdminComponent />; } else { return <UserComponent />; } } } class AdminComponent extends React.Component { render() { return ( <div> <h1>Welcome, Admin!</h1> <p>This is the admin component.</p> </div> ); } } class UserComponent extends React.Component { render() { return ( <div> <h1>Welcome, User!</h1> <p>This is the user component.</p> </div> ); } }
In the above example, the render method uses an if statement to check the value of the isAdmin
prop. If it is true
, it renders the AdminComponent
. If it is false
, it renders the UserComponent
. This allows for different components to be rendered based on a specific condition, providing the ability to create dynamic and customizable UIs.
Implementing Conditional Rendering using If Statements in React
To implement conditional rendering using if statements in React, you can use the if statement directly in the render method. This allows for the evaluation of a condition and the rendering of different components or content based on that condition.
Here's an example of implementing conditional rendering using if statements in React:
import React from 'react'; class MyComponent extends React.Component { render() { const isLoggedIn = this.props.isLoggedIn; if (isLoggedIn) { return ( <div> <h1>Welcome back!</h1> <p>This is some content that is conditionally rendered.</p> </div> ); } else { return null; } } }
In the above example, the render method uses an if statement to check the value of the isLoggedIn
prop. If it is true
, it renders a heading and a paragraph. If it is false
, it returns null
, indicating that no content should be rendered. This implementation allows for the conditional rendering of components or content based on a specific condition.
Alternatives to Using If Statements for Conditional Rendering in React
While if statements are a common and straightforward way to implement conditional rendering in React, there are alternative techniques that can achieve the same result. These alternatives can provide more concise and readable code in certain scenarios.
One alternative to using if statements for conditional rendering is the conditional (ternary) operator. The conditional operator allows for the evaluation of a condition and the rendering of different components or content in a single line.
Here's an example of using the conditional operator for conditional rendering in React:
import React from 'react'; class MyComponent extends React.Component { render() { const isLoggedIn = this.props.isLoggedIn; return ( <div> {isLoggedIn ? ( <h1>Welcome back!</h1> ) : ( <h1>Please log in.</h1> )} </div> ); } }
In the above example, the conditional operator is used to check the value of the isLoggedIn
prop. If it is true
, it renders the "Welcome back!" heading. If it is false
, it renders the "Please log in." heading. The conditional operator provides a concise and readable way to achieve conditional rendering in React.
Another alternative to using if statements for conditional rendering is the logical && operator. The logical && operator allows for the rendering of a component or content only if a certain condition is met.
Here's an example of using the logical && operator for conditional rendering in React:
import React from 'react'; class MyComponent extends React.Component { render() { const isLoggedIn = this.props.isLoggedIn; return ( <div> {isLoggedIn && <h1>Welcome back!</h1>} </div> ); } }
In the above example, the logical && operator is used to check the value of the isLoggedIn
prop. If it is true
, it renders the "Welcome back!" heading. If it is false
, nothing is rendered. This technique provides a concise and readable way to conditionally render a component or content based on a specific condition.
Best Practices for Rendering Components Conditionally in React
When rendering components conditionally in React, there are some best practices to keep in mind to ensure clean and maintainable code.
1. Use descriptive variable names: When using if statements or other conditional rendering techniques, it's important to use descriptive variable names that clearly indicate the condition being evaluated. This helps improve code readability and makes it easier for other developers to understand the logic.
2. Extract complex conditions into separate functions or variables: If the condition for conditional rendering becomes complex, it's a good practice to extract it into a separate function or variable. This helps improve code readability and makes it easier to test and maintain.
import React from 'react'; class MyComponent extends React.Component { render() { const shouldRenderComponent = this.shouldRenderComponent(); if (shouldRenderComponent) { return <CustomComponent />; } else { return null; } } shouldRenderComponent() { const condition1 = this.props.condition1; const condition2 = this.props.condition2; return condition1 && condition2; } }
3. Use the conditional operator or logical && operator for concise rendering: In cases where the condition for conditional rendering is simple, it's recommended to use the conditional operator or logical && operator for more concise code. This helps improve code readability and reduces unnecessary lines of code.
4. Consider using a switch statement for multiple conditions: If there are multiple conditions for conditional rendering, using a switch statement can provide a more readable and maintainable code structure. This allows for more structured and organized handling of multiple conditions.
import React from 'react'; class MyComponent extends React.Component { render() { const condition = this.props.condition; switch (condition) { case 'condition1': return <Component1 />; case 'condition2': return <Component2 />; default: return null; } } }
5. Document the conditions for conditional rendering: When using conditional rendering, it's important to document the conditions that determine when components or content are rendered. This helps other developers understand the logic and makes it easier to maintain the code in the future.
Related Article: How to Implement onClick Functions in ReactJS
Rendering Different Components Based on a Condition in React
In React, it is possible to render different components based on a condition by using if statements or other conditional rendering techniques. This allows for the creation of dynamic and customizable UIs.
Here's an example of rendering different components based on a condition in React:
import React from 'react'; class MyComponent extends React.Component { render() { const condition = this.props.condition; if (condition === 'condition1') { return <Component1 />; } else if (condition === 'condition2') { return <Component2 />; } else { return null; } } }
In the above example, the render method uses if-else statements to check the value of the condition
prop. If it is 'condition1'
, it renders Component1
. If it is 'condition2'
, it renders Component2
. If neither condition is met, it returns null
, indicating that no component should be rendered. This allows for different components to be rendered based on a specific condition, providing the ability to create dynamic and customizable UIs.
Rendering Content Based on Multiple Conditions in ReactJS
In ReactJS, it is possible to render content based on multiple conditions by using logical operators and if statements. This allows for complex conditions to be evaluated and different content to be rendered based on those conditions.
Here's an example of rendering content based on multiple conditions in ReactJS:
import React from 'react'; class MyComponent extends React.Component { render() { const condition1 = this.props.condition1; const condition2 = this.props.condition2; if (condition1 && condition2) { return ( <div> <h1>Welcome back!</h1> <p>This is some content that is conditionally rendered.</p> </div> ); } else { return null; } } }
In the above example, the render method uses if statements to check the values of the condition1
and condition2
props. If both conditions are true
, it renders a heading and a paragraph. If either condition is false
, it returns null
, indicating that no content should be rendered. This allows for the conditional rendering of content based on multiple conditions.
Syntax for Using If Statements in the Render Method of a React Component
The syntax for using if statements in the render method of a React component is straightforward. Here's an example:
import React from 'react'; class MyComponent extends React.Component { render() { const condition = this.props.condition; if (condition) { return <h1>This is some content that is conditionally rendered.</h1>; } else { return null; } } }
In the above example, the render method uses an if statement to check the value of the condition
prop. If it is true
, it renders a heading. If it is false
, it returns null
, indicating that no content should be rendered. The syntax for using if statements in the render method allows for the evaluation of a condition and the rendering of different components or content based on that condition.