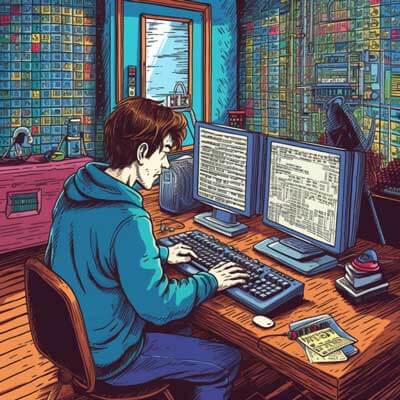
Table of Contents
When building web applications, one essential feature is the ability to accept payments from users. Payment gateways provide a secure and reliable way to handle online transactions. In this section, we will explore how to incorporate payment gateways into Gin apps using Golang.
To integrate payment gateways with Gin, we need to consider several factors. First, we need to choose a payment gateway provider that supports the desired payment methods and currencies. Some popular payment gateway providers include PayPal, Stripe, and Braintree.
Next, we need to set up the necessary configuration and APIs provided by the payment gateway provider. This typically involves creating an account, obtaining API keys, and configuring webhooks for handling payment notifications.
Once the payment gateway is set up, we can start integrating it into our Gin app. This involves creating routes and handlers for the payment process. For example, we may have a route for initiating a payment, a route for handling the payment callback from the payment gateway, and a route for displaying the payment status to the user.
Let's take a look at an example of integrating the Stripe payment gateway with a Gin app in Golang:
// Import the necessary packages import ( "github.com/gin-gonic/gin" "github.com/stripe/stripe-go" "github.com/stripe/stripe-go/charge" ) // Initialize the Stripe API client with your API key stripe.Key = "your_stripe_api_key" // Create a route for initiating a payment router.POST("/payment", func(c *gin.Context) { // Get the payment amount from the request amount := c.PostForm("amount") // Create a charge using the Stripe API params := &stripe.ChargeParams{ Amount: 1000, // Amount in cents Currency: "usd", Source: "tok_visa", // A test card token } ch, err := charge.New(params) if err != nil { // Handle the error c.JSON(500, gin.H{"error": err.Error()}) return } // Payment successful, display the payment status c.JSON(200, gin.H{"status": "success", "charge_id": ch.ID}) }) // Create a route for handling the payment callback router.POST("/payment/callback", func(c *gin.Context) { // Get the payment callback data from the request payload := c.PostForm("payload") // Verify the payment callback with the Stripe API event, err := webhook.ConstructEvent(payload, c.Request.Header.Get("Stripe-Signature"), "your_stripe_webhook_secret") if err != nil { // Handle the error c.JSON(500, gin.H{"error": err.Error()}) return } // Payment callback verified, process the payment // ... // Return a success response c.JSON(200, gin.H{"status": "success"}) })
In this example, we use the Stripe API client to create a charge with the specified amount and currency. We also handle the payment callback using the Stripe webhook library to ensure the payment is valid. Finally, we return the payment status to the user.
Integrating Popular Payment Gateways with Gin in Golang
When it comes to integrating popular payment gateways with Gin in Golang, there are several options available. Each payment gateway provider usually provides their own SDK or API client library for Golang, which makes it easier to interact with their services.
Let's take a look at how we can integrate PayPal, Stripe, and Braintree payment gateways with Gin in Golang.
Related Article: Integrating Beego & Golang Backends with React.js
Integrating PayPal Payment Gateway with Gin
To integrate the PayPal payment gateway with Gin, we need to use the PayPal SDK for Golang. The PayPal SDK provides a set of APIs for creating and managing payments, subscriptions, and transactions.
First, we need to import the PayPal SDK package:
import ( "github.com/paypal/payouts-sdk-go" )
Next, we need to set up the PayPal client with our client ID and secret:
paypalClient, err := payouts.NewClient(clientID, secret, payouts.APIBaseSandBox)
Once the client is set up, we can use it to create and manage payments. For example, to create a payment, we can use the following code:
paymentRequest := &payouts.CreatePaymentRequest{ Intent: "sale", Payer: &payouts.Payer{ PaymentMethod: "paypal", }, Transactions: []payouts.Transaction{ { Amount: &payouts.Amount{ Total: "10.00", Currency: "USD", }, }, }, RedirectURLs: &payouts.RedirectURLs{ ReturnURL: "https://example.com/return", CancelURL: "https://example.com/cancel", }, } response, err := paypalClient.Payments().CreatePayment(payouts.APIContext, paymentRequest)
This code creates a payment request with the specified amount and currency, and sets the return and cancel URLs. The response contains the payment ID and the payment approval URL, which can be used to redirect the user to the PayPal payment page.
Integrating Stripe Payment Gateway with Gin
To integrate the Stripe payment gateway with Gin, we need to use the Stripe SDK for Golang. The Stripe SDK provides a set of APIs for creating and managing payments, subscriptions, and transactions.
First, we need to import the Stripe SDK package:
import ( "github.com/stripe/stripe-go" "github.com/stripe/stripe-go/charge" )
Next, we need to set up the Stripe API client with our API key:
stripe.Key = "your_stripe_api_key"
Once the client is set up, we can use it to create charges. For example, to create a charge with the specified amount and currency, we can use the following code:
params := &stripe.ChargeParams{ Amount: 1000, // Amount in cents Currency: "usd", Source: "tok_visa", // A test card token } ch, err := charge.New(params)
This code creates a charge with the specified amount and currency, using a test card token for the payment source. The ch
variable contains the charge object, which can be used to retrieve the charge ID and other details.
Integrating Braintree Payment Gateway with Gin
To integrate the Braintree payment gateway with Gin, we need to use the Braintree SDK for Golang. The Braintree SDK provides a set of APIs for creating and managing payments, subscriptions, and transactions.
First, we need to import the Braintree SDK package:
import ( "github.com/braintree-go/braintree-go" )
Next, we need to set up the Braintree client with our merchant ID, public key, and private key:
var ( braintreeMerchID = "your_merchant_id" braintreePublicKey = "your_public_key" braintreePrivateKey = "your_private_key" ) gateway := braintree.New( braintree.Sandbox, braintreeMerchID, braintreePublicKey, braintreePrivateKey, )
Once the client is set up, we can use it to create transactions. For example, to create a transaction with the specified amount and currency, we can use the following code:
transactionRequest := &braintree.TransactionRequest{ Amount: decimal.NewFromFloat(10.00), PaymentMethodNonce: "nonce-from-the-client", } result, err := gateway.Transaction().Create(context.Background(), transactionRequest)
This code creates a transaction with the specified amount and currency, using a payment method nonce obtained from the client. The result
variable contains the transaction result, which can be used to retrieve the transaction ID and other details.
Related Article: Optimizing and Benchmarking Beego ORM in Golang
Go Libraries for Implementing SMS Functionalities in Gin Apps
Adding SMS functionalities to Gin apps can be achieved using various Go libraries. These libraries provide an easy-to-use interface for sending and receiving SMS messages, as well as handling other SMS-related tasks such as delivery status tracking and message formatting.
Let's explore some popular Go libraries for implementing SMS functionalities in Gin apps.
Twilio
Twilio is a cloud communications platform that provides APIs for SMS, voice, and video communication. The Twilio Go library allows developers to easily integrate Twilio's SMS functionalities into their Go applications.
To get started with Twilio, you need to sign up for a Twilio account and obtain your account SID and auth token. Once you have these credentials, you can use the Twilio Go library to send SMS messages.
First, import the Twilio Go library:
import ( "github.com/twilio/twilio-go" "github.com/twilio/twilio-go/rest/api/v2010" )
Next, create a new Twilio client with your account SID and auth token:
client := twilio.NewRestClientWithParams("your_account_sid", "your_auth_token", twilio.RestClientParams{})
Once the client is set up, you can use it to send SMS messages:
message, err := client.ApiV2010.CreateMessage(&api.CreateMessageParams{ MessagingServiceSid: "your_messaging_service_sid", Body: "Hello, world!", To: "+123456789", From: "your_twilio_phone_number", })
This code sends an SMS message with the specified body to the specified phone number using a Twilio messaging service.
MessageBird
MessageBird is a cloud communications platform that provides APIs for SMS, voice, and chat communication. The MessageBird Go library allows developers to easily integrate MessageBird's SMS functionalities into their Go applications.
To get started with MessageBird, you need to sign up for a MessageBird account and obtain your API key. Once you have the API key, you can use the MessageBird Go library to send SMS messages.
First, import the MessageBird Go library:
import ( "github.com/messagebird/go-rest-api" )
Next, create a new MessageBird client with your API key:
client := messagebird.New("your_api_key")
Once the client is set up, you can use it to send SMS messages:
message, err := client.NewMessage("your_originator", []string{"+123456789"}, "Hello, world!")
This code sends an SMS message with the specified body to the specified phone number using the specified originator.
Adding Voice Functionalities to Gin Applications using Go Libraries
In addition to SMS functionalities, it is also possible to add voice functionalities to Gin applications using various Go libraries. These libraries provide an easy-to-use interface for making and receiving voice calls, as well as handling other voice-related tasks such as call recording and call routing.
Let's explore some popular Go libraries for adding voice functionalities to Gin applications.
Related Article: Implementing Real-time Features with Gin & Golang
Twilio
As mentioned earlier, Twilio is a cloud communications platform that provides APIs for SMS, voice, and video communication. The Twilio Go library also allows developers to easily integrate Twilio's voice functionalities into their Go applications.
To get started with Twilio's voice functionalities, you need to sign up for a Twilio account and obtain your account SID and auth token. Once you have these credentials, you can use the Twilio Go library to make and receive voice calls.
To make a voice call, you can use the following code:
call, err := client.ApiV2010.CreateCall(&api.CreateCallParams{ Url: "http://example.com/voice.xml", To: "+123456789", From: "your_twilio_phone_number", })
This code initiates a voice call to the specified phone number using the specified Twilio phone number as the caller ID. The Url
parameter specifies the URL of an XML file that defines the behavior of the voice call.
To receive a voice call, you can use the following code:
handler := func(call *api.Call) { // Handle the voice call } webhook := client.NewCallWebhook() webhook.OnAnswered = handler http.Handle("/voice", webhook)
This code sets up a webhook endpoint for receiving voice calls. When a voice call is received, the handler
function is called to handle the call.
Plivo
Plivo is another cloud communications platform that provides APIs for SMS, voice, and video communication. The Plivo Go library allows developers to easily integrate Plivo's voice functionalities into their Go applications.
To get started with Plivo's voice functionalities, you need to sign up for a Plivo account and obtain your auth ID and auth token. Once you have these credentials, you can use the Plivo Go library to make and receive voice calls.
To make a voice call, you can use the following code:
response, err := client.Calls.Create(plivo.CreateCallParams{ From: "your_plivo_phone_number", To: "+123456789", Answer: "http://example.com/answer", })
This code initiates a voice call to the specified phone number using the specified Plivo phone number as the caller ID. The Answer
parameter specifies the URL of an XML file that defines the behavior of the voice call.
To receive a voice call, you can use the following code:
router.POST("/answer", func(c *gin.Context) { response := plivo.Response{} r := plivo.NewResponse() r.AddSpeak("Hello, world!") response.Add(r) c.XML(200, response) })
This code sets up a route for receiving voice calls. When a voice call is received, the specified XML response is returned to the caller.
Best Practices for Implementing Chatbots in Gin Applications
Chatbots have become increasingly popular in recent years, as they provide a convenient and efficient way for users to interact with applications. When implementing chatbots in Gin applications, it is important to follow best practices to ensure a smooth user experience and minimize development effort.
Here are some best practices for implementing chatbots in Gin applications:
1. Define Clear Use Cases
Before starting the implementation of a chatbot, it is important to define clear use cases and goals for the chatbot. This will help guide the design and development process and ensure that the chatbot meets the needs of the users.
For example, if the chatbot is intended to provide customer support, the use cases may include answering frequently asked questions, guiding users through troubleshooting processes, and escalating complex issues to human agents.
Related Article: Best Practices of Building Web Apps with Gin & Golang
2. Use a Natural Language Processing (NLP) Library
To enable the chatbot to understand and respond to user input in a natural and context-aware manner, it is recommended to use a Natural Language Processing (NLP) library. NLP libraries provide advanced algorithms and models for processing and understanding human language.
Some popular NLP libraries for Go include Go-NLP, spaGO, and Gobert. These libraries can help with tasks such as intent recognition, entity extraction, and sentiment analysis.
3. Implement Contextual Conversations
To provide a more conversational and interactive experience, it is important to implement contextual conversations in the chatbot. Contextual conversations allow the chatbot to remember previous interactions and use that information to guide future conversations.
For example, if a user asks the chatbot for restaurant recommendations and then asks for directions to a specific restaurant, the chatbot should be able to understand the context and provide relevant responses based on the previous conversation.
4. Provide Clear Help and Error Messages
To ensure a smooth user experience, it is important to provide clear help and error messages in the chatbot. Help messages can guide users on how to interact with the chatbot and provide examples of valid inputs.
Error messages should be informative and provide suggestions for correcting the input. For example, if the user provides an invalid date format, the chatbot can respond with a message like "Please provide the date in the format yyyy-mm-dd".
5. Test and Iterate
Like any other software development process, it is important to test and iterate on the chatbot implementation. This involves testing the chatbot with different user inputs and scenarios to ensure that it responds correctly and handles edge cases gracefully.
User feedback and analytics can also provide valuable insights for improving the chatbot. By continuously testing and iterating on the chatbot, you can make it more accurate, efficient, and user-friendly.
Implementing chatbots in Gin applications can greatly enhance the user experience and provide valuable functionality. By following these best practices, you can ensure that your chatbot is effective, user-friendly, and meets the needs of your users.
Related Article: Best Practices for Building Web Apps with Beego & Golang
Integrating IVRs in Gin Apps
Interactive Voice Response (IVR) systems allow users to interact with applications or services using their voice and touch-tone keypad inputs. IVRs are commonly used in call centers, customer support systems, and automated phone systems.
To integrate IVRs in Gin apps, we can use various technologies and libraries. Here, we will explore the process of integrating IVRs using the Twilio IVR system and the Twilio Go library.
To get started, you need to sign up for a Twilio account and obtain your account SID and auth token. Once you have these credentials, you can use the Twilio Go library to interact with the Twilio IVR system.
First, import the necessary packages:
import ( "github.com/gin-gonic/gin" "github.com/twilio/twilio-go" "github.com/twilio/twilio-go/rest/api/v2010" )
Next, create a Twilio client with your account SID and auth token:
client := twilio.NewRestClientWithParams("your_account_sid", "your_auth_token", twilio.RestClientParams{})
Once the client is set up, you can define routes and handlers for handling IVR interactions:
router.POST("/ivr", func(c *gin.Context) { // Get the user's input from the request digits := c.PostForm("Digits") // Handle the user's input based on the IVR menu options switch digits { case "1": // Handle option 1 c.XML(200, gin.H{"Response": "Option 1 selected"}) case "2": // Handle option 2 c.XML(200, gin.H{"Response": "Option 2 selected"}) default: // Invalid option c.XML(200, gin.H{"Response": "Invalid option"}) } })
In this example, we define a route /ivr
for handling IVR interactions. The user's input is retrieved from the request, and the appropriate action is taken based on the selected option.
To initiate an IVR call, you can use the following code:
call, err := client.ApiV2010.CreateCall(&api.CreateCallParams{ Url: "http://example.com/ivr.xml", To: "+123456789", From: "your_twilio_phone_number", })
This code initiates an IVR call to the specified phone number using the specified Twilio phone number as the caller ID. The Url
parameter specifies the URL of an XML file that defines the IVR menu options and behavior.
In the XML file (ivr.xml
), you can define the IVR menu options and behavior using the TwiML markup language. For example:
<Response> <Gather numDigits="1" action="/ivr"> <Say>Please press 1 for option 1, or 2 for option 2.</Say> </Gather> </Response>
This XML file defines an IVR menu with two options. The user is prompted to press 1 for option 1 or 2 for option 2. The numDigits
attribute specifies the number of digits to gather from the user, and the action
attribute specifies the URL to submit the user's input.
Different Communication Interfaces for Gin Applications
Gin applications can benefit from using various communication interfaces to provide a seamless and interactive user experience. These communication interfaces can include chatbots, IVRs, voice assistants, and more.
Let's explore some different communication interfaces that can be integrated into Gin applications.
Chatbots
Chatbots are computer programs that use natural language processing (NLP) to simulate human-like conversations. They can be integrated into Gin applications to provide real-time assistance, answer frequently asked questions, and handle customer support inquiries.
IVRs
Interactive Voice Response (IVR) systems allow users to interact with applications or services using their voice and touch-tone keypad inputs. IVRs are commonly used in call centers, customer support systems, and automated phone systems.
Related Article: Golang Tutorial for Backend Development
Voice Assistants
Voice assistants, such as Amazon's Alexa, Apple's Siri, or Google Assistant, can be integrated into Gin applications to provide voice-based interactions and perform tasks based on user commands. Voice assistants can be used for tasks such as searching for information, controlling smart home devices, or making online purchases.
To integrate voice assistants into Gin applications, developers can leverage the respective APIs provided by the voice assistant platforms. These APIs allow developers to handle voice commands, retrieve information from external services, and provide responses back to the user.
Push Notifications
Push notifications are messages that are sent to a user's device, even when the user is not actively using the application. Push notifications can be used in Gin applications to send updates, reminders, or alerts to users.
SMS and Email
SMS and email are classic communication interfaces that can be integrated into Gin applications to send notifications, confirmations, or alerts to users. SMS and email are widely supported by mobile devices and can reach users even when they are not actively using the application.
To integrate SMS and email functionalities into Gin applications, developers can use third-party providers like Twilio or SendGrid. These providers offer APIs for sending and receiving SMS messages and emails, providing a reliable and scalable solution for communication.
Additional Resources
- Implementing SMS and Voice Functionalities in Gin Apps using Go-Plivo
- Integrating SMS and Voice Functionalities in Gin Apps with Go-Twilio