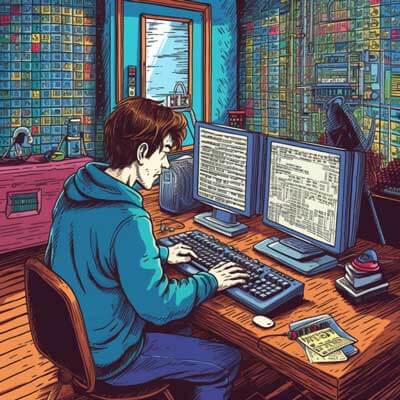
Table of Contents
Overview of Python's Increment Operator
The increment operator is a shorthand notation that allows you to increment the value of a variable by a certain amount. In Python, the increment operator is represented by the '+=' symbol.
To work with the increment operator in Python, you first need to declare a variable. A variable is a container that holds a value. You can assign a value to a variable using the assignment operator '='. Once you have a variable, you can then use the increment operator to increase its value.
Let's take a look at an example:
x = 5 x += 1 print(x) # Output: 6
In the example above, we declare a variable 'x' and assign it a value of 5. We then use the increment operator '+=' to increase the value of 'x' by 1. Finally, we print the value of 'x', which is now 6.
Related Article: How to Print a Python Dictionary Line by Line
Incrementing by 1 in Python
Incrementing by 1 is a common operation in programming. Python provides a simple and concise way to increment a variable by 1 using the increment operator '+='.
Here's an example:
x = 7 x += 1 print(x) # Output: 8
In the example above, we declare a variable 'x' and assign it a value of 7. We then use the increment operator '+=' to increase the value of 'x' by 1. The final value of 'x' is 8.
Increment in Loops
The increment operator is often used in loops to iterate over a sequence of values. Let's consider an example using a 'for' loop:
for i in range(5): print(i) i += 1
In the example above, we use the 'range' function to generate a sequence of numbers from 0 to 4. Inside the loop, we print the value of 'i' and then use the increment operator '+=' to increase its value by 1. However, it's important to note that the increment operator does not affect the iteration of the loop. The 'for' loop automatically increments the value of 'i' for each iteration.
Increment a Variable
Python provides various ways to increment a variable. Apart from the increment operator '+=', you can also use other arithmetic operators in combination with the assignment operator '=' to achieve the same result.
Let's explore some examples:
x = 5 x = x + 1 print(x) # Output: 6
In the example above, we declare a variable 'x' and assign it a value of 5. We then use the addition operator '+' and the assignment operator '=' to increment the value of 'x' by 1. The final value of 'x' is 6.
You can also use other arithmetic operators like subtraction, multiplication, and division in combination with the assignment operator to increment a variable by a specific amount.
Related Article: How to Use Matplotlib for Chinese Text in Python
Additional Resources
- Python Variables