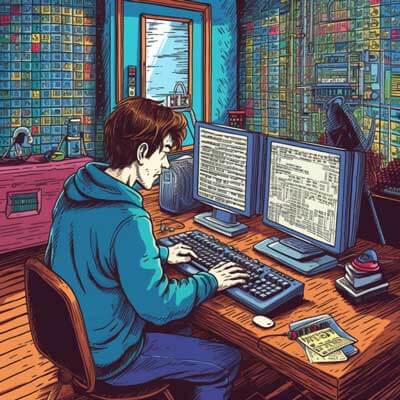
Table of Contents
Integrating Payment Gateways in Laravel
Integrating payment gateways is a crucial aspect of building web applications that require online transactions. Laravel, being a popular PHP framework, provides seamless integration with various payment gateways, making it easier for developers to implement secure and reliable payment processing functionality.
Related Article: How to Use the PHP Random String Generator
Stripe Integration in Laravel
Stripe is a widely used payment gateway that offers a simple and developer-friendly API for processing payments. Integrating Stripe with Laravel is straightforward and can be done using Laravel Cashier, a Laravel package that provides an expressive, fluent interface to Stripe's subscription billing services.
To get started with Stripe integration in Laravel, follow these steps:
Step 1: Install Laravel Cashier using Composer:
composer require laravel/cashier
Step 2: Set up your Stripe API keys in the .env
file:
STRIPE_KEY=your-stripe-public-key STRIPE_SECRET=your-stripe-secret-key
Step 3: Configure your Stripe plans and billing options in the config/services.php
file:
'stripe' => [ 'model' => App\Models\User::class, 'key' => env('STRIPE_KEY'), 'secret' => env('STRIPE_SECRET'), ],
Step 4: Add the Billable
trait to your User model:
use Laravel\Cashier\Billable; class User extends Authenticatable { use Billable; }
With these steps in place, you can now use Laravel Cashier's API to handle payment-related tasks, such as creating subscriptions, managing invoices, and handling webhooks.
PayPal Integration in Laravel
PayPal is another popular payment gateway that provides a secure and reliable way to process online payments. Laravel provides native support for integrating PayPal into your applications using the paypal/rest-api-sdk-php
package.
To integrate PayPal with Laravel, follow these steps:
Step 1: Install the PayPal SDK using Composer:
composer require paypal/rest-api-sdk-php
Step 2: Set up your PayPal API credentials in the .env
file:
PAYPAL_CLIENT_ID=your-paypal-client-id PAYPAL_SECRET=your-paypal-secret
Step 3: Configure the PayPal SDK with your credentials in the config/services.php
file:
'paypal' => [ 'client_id' => env('PAYPAL_CLIENT_ID'), 'secret' => env('PAYPAL_SECRET'), 'settings' => [ 'mode' => env('PAYPAL_MODE', 'sandbox'), 'http.ConnectionTimeOut' => 30, 'log.LogEnabled' => true, 'log.FileName' => storage_path('logs/paypal.log'), 'log.LogLevel' => 'DEBUG', ], ],
Step 4: Use the PayPal SDK to create and process payments in your Laravel application:
use PayPal\Api\Amount; use PayPal\Api\Payer; use PayPal\Api\Payment; use PayPal\Api\RedirectUrls; use PayPal\Api\Transaction; $apiContext = new \PayPal\Rest\ApiContext( new \PayPal\Auth\OAuthTokenCredential( config('services.paypal.client_id'), config('services.paypal.secret') ) ); $payment = new Payment(); $payment->setIntent('sale'); $payer = new Payer(); $payer->setPaymentMethod('paypal'); $payment->setPayer($payer); $amount = new Amount(); $amount->setTotal('10.00'); $amount->setCurrency('USD'); $payment->setTransactions([ (new Transaction())->setAmount($amount) ]); $redirectUrls = new RedirectUrls(); $redirectUrls->setReturnUrl('http://example.com/success') ->setCancelUrl('http://example.com/cancel'); $payment->setRedirectUrls($redirectUrls); $payment->create($apiContext);
These steps will enable you to integrate PayPal seamlessly into your Laravel application, allowing you to process payments and handle various PayPal-related tasks.
SMS Functionality in Laravel
Adding SMS functionality to your Laravel application can enhance user engagement and provide important notifications. Laravel has built-in support for integrating SMS functionalities using popular services like Twilio and Nexmo.
Related Article: How to Use Laravel Folio: Tutorial with Advanced Examples
Voice Functionality in Laravel
Voice functionality, including features like IVR (Interactive Voice Response) systems, can be implemented in Laravel applications using services like Nexmo. Laravel provides an interface to interact with Nexmo's API, making it easy to build voice-based applications.
Twilio Integration for SMS Functionality in Laravel
Twilio is a cloud communications platform that provides APIs for SMS, voice, and video communication. Integrating Twilio with Laravel allows you to send SMS messages, make phone calls, and perform other communication tasks.
To integrate Twilio for SMS functionality in your Laravel app, follow these steps:
Step 1: Install the Twilio package using Composer:
composer require twilio/sdk
Step 2: Set up your Twilio credentials in the .env
file:
TWILIO_SID=your-twilio-sid TWILIO_AUTH_TOKEN=your-twilio-auth-token TWILIO_PHONE_NUMBER=your-twilio-phone-number
Step 3: Use the Twilio API to send SMS messages in your Laravel application:
use Twilio\Rest\Client; $client = new Client(config('services.twilio.sid'), config('services.twilio.auth_token')); $client->messages->create( '+1234567890', // recipient's phone number [ 'from' => config('services.twilio.phone_number'), 'body' => 'Hello, this is a test message from Twilio!' ] );
With these steps, you can send SMS messages using Twilio in your Laravel application.
Nexmo Integration for Voice Functionality in Laravel
Nexmo is a cloud communications platform that provides APIs for SMS, voice, and other communication services. Laravel provides an interface to interact with Nexmo's API, allowing you to implement voice functionality in your Laravel applications.
To integrate Nexmo for voice functionality in your Laravel app, follow these steps:
Step 1: Install the Nexmo package using Composer:
composer require nexmo/client
Step 2: Set up your Nexmo credentials in the .env
file:
NEXMO_API_KEY=your-nexmo-api-key NEXMO_API_SECRET=your-nexmo-api-secret NEXMO_PHONE_NUMBER=your-nexmo-phone-number
Step 3: Use the Nexmo API to make voice calls in your Laravel application:
use Nexmo\Client; $client = new Client([ 'api_key' => config('services.nexmo.api_key'), 'api_secret' => config('services.nexmo.api_secret') ]); $response = $client->calls->create([ 'to' => [[ 'type' => 'phone', 'number' => '+1234567890' // recipient's phone number ]], 'from' => [ 'type' => 'phone', 'number' => config('services.nexmo.phone_number') ], 'answer_url' => ['http://example.com/answer'] ]); echo $response->getId();
These steps will enable you to integrate Nexmo for voice functionality in your Laravel application, allowing you to make voice calls and handle other voice-related tasks.
Chatbot Best Practices in Laravel
Building chatbots in Laravel can enhance user interactions and provide automated support. Here are some best practices to consider when building chatbots in Laravel:
1. Design conversational flows: Plan and design the conversational flow of your chatbot to ensure smooth interactions with users. Use tools like BotMan, a chatbot framework for Laravel, to define conversation patterns and handle user input.
2. Natural Language Processing (NLP): Integrate NLP services like Dialogflow or Wit.ai to understand and interpret user input. NLP allows your chatbot to handle complex queries and provide accurate responses.
3. State management: Implement a state management system to keep track of the user's conversation history. Laravel's session and database drivers can be used to store and retrieve conversation states.
4. Error handling: Handle errors gracefully by providing meaningful error messages and fallback responses when the chatbot encounters unknown or unsupported requests.
5. Integration with external APIs: Integrate your chatbot with external APIs to fetch data or perform actions on behalf of the user. Laravel provides convenient ways to interact with external APIs using HTTP clients like Guzzle.
Related Article: How To Convert A String To A Number In PHP
IVR Creation in Laravel Applications
IVR (Interactive Voice Response) systems allow users to interact with a phone system through voice or keypad inputs. Laravel provides the tools to create IVR systems using services like Nexmo.
To create an IVR system in Laravel, follow these steps:
Step 1: Set up a route in your Laravel application to handle incoming calls:
use Illuminate\Http\Request; Route::post('/ivr', function (Request $request) { // Handle incoming call and respond with IVR menu options });
Step 2: Use Laravel's session or database drivers to store the state of the IVR session and handle user input.
Step 3: Define IVR menu options and handle user input accordingly:
use Nexmo\Call\NCCO\Action\Talk; use Nexmo\Call\NCCO\Action\Input; $ncco = []; if ($request->input('dtmf')) { // Handle user input } else { // Play IVR menu options $ncco[] = new Talk('Welcome to the IVR system. Press 1 for sales, 2 for support, or 3 for billing.'); $ncco[] = new Input('dtmf'); } return response()->json($ncco);
With these steps, you can create interactive IVR systems in Laravel applications, allowing users to navigate through menus and perform actions using voice or keypad inputs.
Building Text Interfaces in Laravel
Building text interfaces in Laravel allows users to interact with your application through text-based channels like SMS, chat, or command-line interfaces. Laravel provides various libraries and packages that make it easy to build text interfaces.
Some popular libraries and packages for building text interfaces in Laravel include:
1. BotMan: BotMan is a PHP library that provides a framework-agnostic way to build chatbots and conversational agents. It supports multiple messaging platforms, including Facebook Messenger, Slack, and Telegram.
2. Inertia.js: Inertia.js is a server-side rendering (SSR) framework that allows you to build single-page applications (SPAs) with Laravel and Vue.js. It provides a seamless way to create text interfaces by rendering views on the server and updating the page content dynamically.
3. Laravel Zero: Laravel Zero is a micro-framework for building console applications with Laravel. It simplifies the process of building command-line interfaces (CLIs) by providing a clean and intuitive API.
These libraries and packages offer useful tools and abstractions to build text interfaces in Laravel applications, allowing you to create interactive and user-friendly experiences.
Setting Up Stripe and PayPal as Payment Gateways in Laravel Cashier
Laravel Cashier is a package that provides an expressive, fluent interface to Stripe's subscription billing services. With Laravel Cashier, you can easily set up Stripe and PayPal as payment gateways in your Laravel application.
To set up Stripe as a payment gateway using Laravel Cashier, follow these steps:
Step 1: Install Laravel Cashier using Composer:
composer require laravel/cashier
Step 2: Set up your Stripe API keys in the .env
file:
STRIPE_KEY=your-stripe-public-key STRIPE_SECRET=your-stripe-secret-key
Step 3: Configure your Stripe plans and billing options in the config/services.php
file:
'stripe' => [ 'model' => App\Models\User::class, 'key' => env('STRIPE_KEY'), 'secret' => env('STRIPE_SECRET'), ],
Step 4: Add the Billable
trait to your User model:
use Laravel\Cashier\Billable; class User extends Authenticatable { use Billable; }
To set up PayPal as a payment gateway using Laravel Cashier, follow these steps:
Step 1: Install the PayPal SDK using Composer:
composer require paypal/rest-api-sdk-php
Step 2: Set up your PayPal API credentials in the .env
file:
PAYPAL_CLIENT_ID=your-paypal-client-id PAYPAL_SECRET=your-paypal-secret
Step 3: Configure the PayPal SDK with your credentials in the config/services.php
file:
'paypal' => [ 'client_id' => env('PAYPAL_CLIENT_ID'), 'secret' => env('PAYPAL_SECRET'), 'settings' => [ 'mode' => env('PAYPAL_MODE', 'sandbox'), 'http.ConnectionTimeOut' => 30, 'log.LogEnabled' => true, 'log.FileName' => storage_path('logs/paypal.log'), 'log.LogLevel' => 'DEBUG', ], ],
Step 4: Use Laravel Cashier's API to handle PayPal payments and subscriptions:
$user = User::find(1); $user->newSubscription('default', 'monthly') ->create($paymentMethod, [ 'email' => $user->email, ]);
With these steps, you can set up Stripe and PayPal as payment gateways in your Laravel application using Laravel Cashier.
Integrating Twilio for SMS Functionality in Laravel Apps
Twilio is a cloud communications platform that provides APIs for SMS, voice, and video communication. Integrating Twilio with Laravel allows you to add SMS functionality to your applications.
To integrate Twilio for SMS functionality in your Laravel app, follow these steps:
Step 1: Install the Twilio package using Composer:
composer require twilio/sdk
Step 2: Set up your Twilio credentials in the .env
file:
TWILIO_SID=your-twilio-sid TWILIO_AUTH_TOKEN=your-twilio-auth-token TWILIO_PHONE_NUMBER=your-twilio-phone-number
Step 3: Use the Twilio API to send SMS messages in your Laravel application:
use Twilio\Rest\Client; $client = new Client(config('services.twilio.sid'), config('services.twilio.auth_token')); $client->messages->create( '+1234567890', // recipient's phone number [ 'from' => config('services.twilio.phone_number'), 'body' => 'Hello, this is a test message from Twilio!' ] );
With these steps, you can integrate Twilio for SMS functionality in your Laravel application, allowing you to send SMS messages and handle other communication tasks.
Related Article: How To Add Elements To An Empty Array In PHP
Implementing Voice Functionality using Nexmo in Laravel
Nexmo is a cloud communications platform that provides APIs for SMS, voice, and other communication services. Laravel provides an interface to interact with Nexmo's API, making it easy to implement voice functionality in your Laravel applications.
To implement voice functionality using Nexmo in your Laravel app, follow these steps:
Step 1: Install the Nexmo package using Composer:
composer require nexmo/client
Step 2: Set up your Nexmo credentials in the .env
file:
NEXMO_API_KEY=your-nexmo-api-key NEXMO_API_SECRET=your-nexmo-api-secret NEXMO_PHONE_NUMBER=your-nexmo-phone-number
Step 3: Use the Nexmo API to make voice calls in your Laravel application:
use Nexmo\Client; $client = new Client([ 'api_key' => config('services.nexmo.api_key'), 'api_secret' => config('services.nexmo.api_secret') ]); $response = $client->calls->create([ 'to' => [[ 'type' => 'phone', 'number' => '+1234567890' // recipient's phone number ]], 'from' => [ 'type' => 'phone', 'number' => config('services.nexmo.phone_number') ], 'answer_url' => ['http://example.com/answer'] ]); echo $response->getId();
These steps will enable you to implement voice functionality using Nexmo in your Laravel application, allowing you to make voice calls and handle other voice-related tasks.
Best Practices for Building Chatbots in Laravel
Building chatbots in Laravel can enhance user interactions and provide automated support. Here are some best practices to consider when building chatbots in Laravel:
1. Design conversational flows: Plan and design the conversational flow of your chatbot to ensure smooth interactions with users. Use tools like BotMan, a chatbot framework for Laravel, to define conversation patterns and handle user input.
2. Natural Language Processing (NLP): Integrate NLP services like Dialogflow or Wit.ai to understand and interpret user input. NLP allows your chatbot to handle complex queries and provide accurate responses.
3. State management: Implement a state management system to keep track of the user's conversation history. Laravel's session and database drivers can be used to store and retrieve conversation states.
4. Error handling: Handle errors gracefully by providing meaningful error messages and fallback responses when the chatbot encounters unknown or unsupported requests.
5. Integration with external APIs: Integrate your chatbot with external APIs to fetch data or perform actions on behalf of the user. Laravel provides convenient ways to interact with external APIs using HTTP clients like Guzzle.
Creating IVRs (Interactive Voice Response) in Laravel Applications
IVR (Interactive Voice Response) systems allow users to interact with a phone system through voice or keypad inputs. Laravel provides the tools to create IVR systems using services like Nexmo.
To create an IVR system in Laravel, follow these steps:
Step 1: Set up a route in your Laravel application to handle incoming calls:
use Illuminate\Http\Request; Route::post('/ivr', function (Request $request) { // Handle incoming call and respond with IVR menu options });
Step 2: Use Laravel's session or database drivers to store the state of the IVR session and handle user input.
Step 3: Define IVR menu options and handle user input accordingly:
use Nexmo\Call\NCCO\Action\Talk; use Nexmo\Call\NCCO\Action\Input; $ncco = []; if ($request->input('dtmf')) { // Handle user input } else { // Play IVR menu options $ncco[] = new Talk('Welcome to the IVR system. Press 1 for sales, 2 for support, or 3 for billing.'); $ncco[] = new Input('dtmf'); } return response()->json($ncco);
With these steps, you can create interactive IVR systems in Laravel applications, allowing users to navigate through menus and perform actions using voice or keypad inputs.
Libraries or Packages for Building Text Interfaces in Laravel
Building text interfaces in Laravel allows users to interact with your application through text-based channels like SMS, chat, or command-line interfaces. Laravel provides various libraries and packages that make it easy to build text interfaces.
Some popular libraries and packages for building text interfaces in Laravel include:
1. BotMan: BotMan is a PHP library that provides a framework-agnostic way to build chatbots and conversational agents. It supports multiple messaging platforms, including Facebook Messenger, Slack, and Telegram.
2. Inertia.js: Inertia.js is a server-side rendering (SSR) framework that allows you to build single-page applications (SPAs) with Laravel and Vue.js. It provides a seamless way to create text interfaces by rendering views on the server and updating the page content dynamically.
3. Laravel Zero: Laravel Zero is a micro-framework for building console applications with Laravel. It simplifies the process of building command-line interfaces (CLIs) by providing a clean and intuitive API.
Related Article: How to Fix Error 419 Page Expired in Laravel Post Request
Additional Resources
- Setting Up Stripe with Laravel Cashier