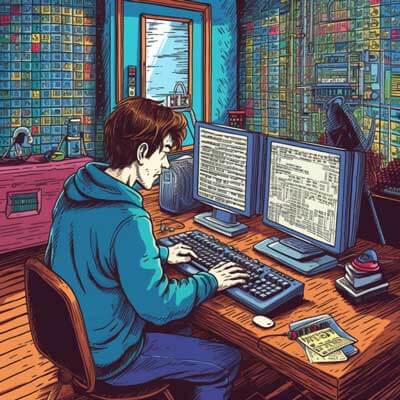
Table of Contents
Integrating Phoenix with Bootstrap
Phoenix is a useful web framework written in Elixir, and Bootstrap is a popular CSS framework that helps developers create responsive and visually appealing web applications. Integrating Phoenix with Bootstrap can greatly enhance the front-end of your Phoenix application and improve the user experience. In this section, we will explore how to integrate Phoenix with Bootstrap.
To integrate Phoenix with Bootstrap, follow these steps:
Step 1: Install Bootstrap
Start by adding Bootstrap to your Phoenix project. You can install Bootstrap using npm, a package manager for JavaScript. Open your terminal, navigate to your Phoenix project directory, and run the following command:
npm install bootstrap
This will install Bootstrap and its dependencies in your project.
Step 2: Import Bootstrap
Now that Bootstrap is installed, you need to import it into your Phoenix application. Open the app.css
file located in the assets/css
directory of your Phoenix project. Add the following line at the top of the file to import Bootstrap:
@import "bootstrap";
This will import all the stylesheets provided by Bootstrap into your Phoenix application.
Step 3: Add Bootstrap classes to your templates
With Bootstrap imported, you can now start using its classes in your Phoenix templates. For example, if you want to create a button with the primary style, add the btn btn-primary
classes to the <button>
element in your template:
<button class="btn btn-primary">Click me</button>
This will apply the primary button style provided by Bootstrap to the button element.
Step 4: Customize Bootstrap (optional)
If you want to customize the look and feel of Bootstrap to match your application's branding, you can override the default Bootstrap variables. Create a new file called _custom-variables.scss
in the assets/css
directory of your Phoenix project. In this file, you can define your custom variables and their values. For example, to change the primary color of Bootstrap, add the following line to _custom-variables.scss
:
$primary-color: #ff0000;
This will change the primary color of Bootstrap to red. Make sure to import your custom variables file after importing Bootstrap in the app.css
file:
@import "bootstrap"; @import "custom-variables";
Related Article: Internationalization & Encoding in Elixir Phoenix
Integrating Phoenix with Elasticsearch using the Tirexs library
Elasticsearch is a useful search engine that can be integrated with Phoenix to enable advanced search capabilities in your application. The Tirexs library provides a convenient way to interact with Elasticsearch in Elixir. In this section, we will explore how to integrate Phoenix with Elasticsearch using the Tirexs library.
To integrate Phoenix with Elasticsearch using the Tirexs library, follow these steps:
Step 1: Add Tirexs to your dependencies
Start by adding Tirexs to the mix.exs
file of your Phoenix project. Open the mix.exs
file, locate the deps
function, and add the following line:
{:tirexs, "~> 1.1"}
This will add Tirexs as a dependency to your project.
Step 2: Fetch and compile dependencies
After adding Tirexs to your dependencies, fetch and compile the dependencies by running the following command in your terminal:
mix deps.get
This will download and compile the required dependencies, including Tirexs.
Step 3: Configure Elasticsearch connection
Next, you need to configure the connection to your Elasticsearch cluster. Open the config/config.exs
file of your Phoenix project, locate the config :tirexs
section, and add the following lines:
config :tirexs, elasticsearch: [ url: "http://localhost:9200" ]
Replace http://localhost:9200
with the URL of your Elasticsearch cluster if it's running on a different host or port.
Step 4: Use Tirexs in your Phoenix application
With the configuration in place, you can now start using Tirexs in your Phoenix application. For example, let's say you want to perform a search query in one of your controllers. Open the desired controller file and add the following code inside the appropriate action:
def search(conn, params) do {:ok, result} = Tirexs.search("my_index", "my_type", %{ query: %{match: %{field: "title", query: params["q"]}} }) # Process the search result and render the appropriate view render(conn, "search.html", result: result) end
This code performs a search query against the my_index
index and the my_type
type, searching for documents whose title
field matches the query parameter q
. The search result is then passed to the view for rendering.
Configuring Phoenix with Ecto for MySQL, PostgreSQL, and MongoDB
Phoenix comes with Ecto, a flexible database wrapper and query generator, which allows you to easily work with various database systems, including MySQL, PostgreSQL, and MongoDB. In this section, we will explore how to configure Phoenix with Ecto for these three popular database systems.
Configuring Phoenix with Ecto for MySQL
To configure Phoenix with Ecto for MySQL, follow these steps:
Step 1: Install the necessary dependencies
Start by adding the mysql
and ecto_mysql
packages to the mix.exs
file of your Phoenix project. Open the mix.exs
file, locate the deps
function, and add the following lines:
{:mysql, "~> 2.0"}, {:ecto_mysql, "~> 0.5"}
This will add the MySQL driver and the Ecto adapter for MySQL as dependencies to your project.
Step 2: Configure the database connection
Next, open the config/config.exs
file of your Phoenix project and locate the config :my_app, MyApp.Repo
section. Replace the existing configuration with the following code:
config :my_app, MyApp.Repo, adapter: Ecto.Adapters.MySQL, username: "your_username", password: "your_password", database: "your_database", hostname: "localhost", pool_size: 10
Replace "your_username"
, "your_password"
, and "your_database"
with the appropriate values for your MySQL setup. If your MySQL server is running on a different hostname or port, update the hostname
configuration accordingly.
Step 3: Create the database migration
To create a migration for your MySQL database, run the following command in your terminal:
mix ecto.gen.migration create_table_name
Replace table_name
with the name of the table you want to create. This will generate a new migration file in the priv/repo/migrations
directory of your Phoenix project.
Step 4: Run the database migration
To run the migration and create the table in your MySQL database, run the following command in your terminal:
mix ecto.migrate
Ecto will execute the migration and create the table in your MySQL database.
Related Article: Phoenix Design Patterns: Actor Model, Repositories, and Events
Configuring Phoenix with Ecto for PostgreSQL
To configure Phoenix with Ecto for PostgreSQL, follow these steps:
Step 1: Install the necessary dependencies
Start by adding the postgrex
package to the mix.exs
file of your Phoenix project. Open the mix.exs
file, locate the deps
function, and add the following line:
{:postgrex, "~> 0.15"}
This will add the PostgreSQL driver as a dependency to your project.
Step 2: Configure the database connection
Next, open the config/config.exs
file of your Phoenix project and locate the config :my_app, MyApp.Repo
section. Replace the existing configuration with the following code:
config :my_app, MyApp.Repo, adapter: Ecto.Adapters.Postgres, database: "your_database", username: "your_username", password: "your_password", hostname: "localhost", pool_size: 10
Replace "your_username"
, "your_password"
, and "your_database"
with the appropriate values for your PostgreSQL setup. If your PostgreSQL server is running on a different hostname or port, update the hostname
configuration accordingly.
Step 3: Create the database migration
To create a migration for your PostgreSQL database, run the following command in your terminal:
mix ecto.gen.migration create_table_name
Replace table_name
with the name of the table you want to create. This will generate a new migration file in the priv/repo/migrations
directory of your Phoenix project.
Step 4: Run the database migration
To run the migration and create the table in your PostgreSQL database, run the following command in your terminal:
mix ecto.migrate
Ecto will execute the migration and create the table in your PostgreSQL database.
Configuring Phoenix with Ecto for MongoDB
To configure Phoenix with Ecto for MongoDB, follow these steps:
Step 1: Install the necessary dependencies
Start by adding the mongodb
and mongodb_ecto
packages to the mix.exs
file of your Phoenix project. Open the mix.exs
file, locate the deps
function, and add the following lines:
{:mongodb, ">= 0.0.0"}, {:mongodb_ecto, "~> 1.2"}
This will add the MongoDB driver and the Ecto adapter for MongoDB as dependencies to your project.
Step 2: Configure the database connection
Next, open the config/config.exs
file of your Phoenix project and locate the config :my_app, MyApp.Repo
section. Replace the existing configuration with the following code:
config :my_app, MyApp.Repo, adapter: Mongo.Ecto, database: "your_database", username: "your_username", password: "your_password", hostname: "localhost", pool_size: 10
Replace "your_username"
, "your_password"
, and "your_database"
with the appropriate values for your MongoDB setup. If your MongoDB server is running on a different hostname or port, update the hostname
configuration accordingly.
Step 3: Create the database migration
To create a migration for your MongoDB database, run the following command in your terminal:
mix ecto.gen.migration create_table_name
Replace table_name
with the name of the collection you want to create. This will generate a new migration file in the priv/repo/migrations
directory of your Phoenix project.
Step 4: Run the database migration
To run the migration and create the collection in your MongoDB database, run the following command in your terminal:
mix ecto.migrate
Ecto will execute the migration and create the collection in your MongoDB database.
Third-party tools commonly used in Elixir
Elixir, being a useful and flexible programming language, has a thriving ecosystem with a wide range of third-party tools that can greatly enhance your development experience and productivity. In this section, we will explore some of the most commonly used third-party tools in the Elixir community.
Phoenix LiveView
Phoenix LiveView is a cutting-edge library that enables real-time, interactive web applications without the need for writing JavaScript. It leverages the power of the Phoenix framework and the Erlang VM to provide a seamless and efficient development experience. With LiveView, you can build highly dynamic and responsive web applications with ease.
To use Phoenix LiveView in your Phoenix application, follow these steps:
Step 1: Add the phoenix_live_view
package to the mix.exs
file of your Phoenix project. Open the mix.exs
file, locate the deps
function, and add the following line:
{:phoenix_live_view, "~> 0.16"}
This will add Phoenix LiveView as a dependency to your project.
Step 2: Update your application endpoint file (lib/my_app_web/endpoint.ex
) to include the LiveView endpoint. Add the following line to the socket/1
function:
socket "/live", Phoenix.LiveView.Socket
This will mount the LiveView socket at the /live
endpoint.
Step 3: Create a new LiveView module. Run the following command in your terminal:
mix phx.gen.live MyModule MyModel my_models
Replace MyModule
with the desired module name, MyModel
with the name of the Ecto model you want to associate with the LiveView, and my_models
with the name of the database table.
This will generate a new LiveView module in the lib/my_app_web/live
directory of your Phoenix project.
Step 4: Update your router file (lib/my_app_web/router.ex
) to include the LiveView routes. Add the following line to the scope/2
function:
live "/my_models", MyModule
This will mount the LiveView module at the /my_models
route.
Step 5: Start the Phoenix server with LiveView support by running the following command in your terminal:
mix phx.server
You can now access the LiveView at http://localhost:4000/my_models
.
Related Article: Building Real-Time Apps with Phoenix Channels & WebSockets
ExUnit
ExUnit is Elixir's built-in testing framework, which provides a rich set of tools and functionalities for writing and running tests. It allows you to write unit tests, integration tests, and even acceptance tests for your Elixir code. With ExUnit, you can ensure the correctness and reliability of your codebase.
To use ExUnit for testing in your Elixir project, follow these steps:
Step 1: Create a new test file. In your terminal, navigate to the directory of the module you want to test and run the following command:
mix test.new
This will generate a new test file in the test
directory of your Elixir project.
Step 2: Write your test cases. Open the generated test file and write your test cases using the ExUnit syntax. For example, to test a function that adds two numbers, you can write the following test case:
defmodule MyApp.MyModuleTest do use ExUnit.Case test "add/2 adds two numbers" do assert MyApp.MyModule.add(2, 3) == 5 end end
Step 3: Run your tests. In your terminal, navigate to the root directory of your Elixir project and run the following command:
mix test
ExUnit will run all the tests in your project and display the results.
Popular open source tools used in Elixir development
The Elixir community is known for its vibrant open source ecosystem, which offers a wide range of tools and libraries to enhance the development experience and productivity. In this section, we will explore some of the most popular open source tools used in Elixir development.
Phoenix Framework
Phoenix is a web framework written in Elixir that enables developers to build high-performance, reliable, and scalable web applications. It follows the model-view-controller (MVC) architectural pattern and provides a rich set of features, including real-time capabilities with Phoenix LiveView. Phoenix is widely used in the Elixir community and is known for its ease of use, performance, and developer-friendly conventions.
To install Phoenix, follow the official installation guide on the Phoenix website: Phoenix Framework Installation Guide
Ecto
Ecto is a flexible database wrapper and query generator for Elixir. It provides a unified API to work with various database systems, including SQL databases like PostgreSQL and MySQL, as well as NoSQL databases like MongoDB. Ecto simplifies database interactions and provides useful features such as migrations, database transactions, and query composition. It is widely used in Elixir projects for database management and integration.
To install Ecto, add the ecto
package to the mix.exs
file of your Elixir project:
{:ecto, "~> 3.7"}
Then, fetch and compile the dependencies by running the following command in your terminal:
mix deps.get
For more information on how to use Ecto, refer to the official Ecto documentation: Ecto Documentation
Related Article: Exploring Phoenix: Umbrella Project Structures,Ecto & More
Plug
Plug is a specification and library for building composable web modules in Elixir. It provides a simple and flexible way to build web applications and APIs by composing small, reusable modules. Plug is used by many Elixir web frameworks, including Phoenix, and provides a unified interface for handling HTTP requests and responses. It allows developers to easily handle routing, middleware, and other aspects of web development.
To install Plug, add the plug
package to the mix.exs
file of your Elixir project:
{:plug, "~> 1.11"}
Then, fetch and compile the dependencies by running the following command in your terminal:
mix deps.get
For more information on how to use Plug, refer to the official Plug documentation: Plug Documentation
Enhancing the front-end of Phoenix using Bootstrap
The front-end of a web application plays a crucial role in providing an engaging user experience. Bootstrap, a popular CSS framework, can greatly enhance the front-end of your Phoenix application by providing a responsive and visually appealing design. In this section, we will explore how to enhance the front-end of Phoenix using Bootstrap.
To enhance the front-end of Phoenix using Bootstrap, follow these steps:
Step 1: Install Bootstrap
Start by adding Bootstrap to your Phoenix project. You can install Bootstrap using npm, a package manager for JavaScript. Open your terminal, navigate to your Phoenix project directory, and run the following command:
npm install bootstrap
This will install Bootstrap and its dependencies in your project.
Step 2: Import Bootstrap
Now that Bootstrap is installed, you need to import it into your Phoenix application. Open the app.css
file located in the assets/css
directory of your Phoenix project. Add the following line at the top of the file to import Bootstrap:
@import "bootstrap";
This will import all the stylesheets provided by Bootstrap into your Phoenix application.
Step 3: Use Bootstrap classes in your templates
With Bootstrap imported, you can now start using its classes in your Phoenix templates. For example, if you want to create a button with the primary style, add the btn btn-primary
classes to the <button>
element in your template:
<button class="btn btn-primary">Click me</button>
This will apply the primary button style provided by Bootstrap to the button element.
Step 4: Customize Bootstrap (optional)
If you want to customize the look and feel of Bootstrap to match your application's branding, you can override the default Bootstrap variables. Create a new file called _custom-variables.scss
in the assets/css
directory of your Phoenix project. In this file, you can define your custom variables and their values. For example, to change the primary color of Bootstrap, add the following line to _custom-variables.scss
:
$primary-color: #ff0000;
This will change the primary color of Bootstrap to red. Make sure to import your custom variables file after importing Bootstrap in the app.css
file:
@import "bootstrap"; @import "custom-variables";
Benefits of integrating Phoenix with Elasticsearch
Integrating Phoenix, a useful web framework written in Elixir, with Elasticsearch, a distributed search and analytics engine, can bring several benefits to your application. Elasticsearch offers advanced search capabilities and real-time data analysis, while Phoenix provides a solid foundation for building scalable and reliable web applications. In this section, we will explore the benefits of integrating Phoenix with Elasticsearch.
Advanced search capabilities
Elasticsearch is known for its useful search capabilities. By integrating Phoenix with Elasticsearch, you can leverage these capabilities in your application and provide advanced search functionality to your users. Elasticsearch supports full-text search, fuzzy search, and advanced filtering options, allowing users to find relevant information quickly and efficiently. Whether you are building a search engine, an e-commerce platform, or a content management system, integrating Phoenix with Elasticsearch can greatly enhance the search experience for your users.
Related Article: Phoenix Core Advanced: Contexts, Plugs & Telemetry
Real-time data analysis
Elasticsearch excels at real-time data analysis. By integrating Phoenix with Elasticsearch, you can easily index and analyze data in real-time, enabling you to make informed decisions based on the latest information. Whether you need to monitor application logs, track user behavior, or analyze system metrics, Elasticsearch provides useful tools for aggregating, filtering, and visualizing data. By integrating Phoenix with Elasticsearch, you can build real-time dashboards, generate insightful reports, and gain valuable insights from your data.
Scalability and performance
Both Phoenix and Elasticsearch are designed to be highly scalable and performant. By integrating the two, you can take advantage of their scalability features and build applications that can handle a large number of concurrent users and process massive amounts of data. Elasticsearch's distributed nature allows you to horizontally scale your application as your data grows, while Phoenix's underlying Erlang VM provides lightweight processes and efficient concurrency management. Integrating Phoenix with Elasticsearch enables you to build highly scalable and performant applications that can handle the demands of modern web development.
Flexible data modeling
Phoenix and Elasticsearch both offer flexible data modeling capabilities. Phoenix's underlying Ecto library provides a convenient way to work with various database systems, while Elasticsearch's schema-less nature allows you to index and search unstructured data easily. By integrating Phoenix with Elasticsearch, you can leverage the strengths of both frameworks and build applications that can handle diverse data models. Whether you are working with relational data, JSON documents, or unstructured logs, integrating Phoenix with Elasticsearch provides a flexible and scalable solution.
Alternative libraries for integrating Phoenix with Elasticsearch
While the Tirexs library is a popular choice for integrating Phoenix with Elasticsearch, there are also alternative libraries available that provide similar functionality. In this section, we will explore two alternative libraries for integrating Phoenix with Elasticsearch: ExElasticsearch and Elasticsearch Elixir.
Related Article: Elixir’s Phoenix Security: Token Auth & CSRF Prevention
ExElasticsearch
ExElasticsearch is a library that provides a lightweight and idiomatic Elixir API for interacting with Elasticsearch. It is built on top of the official Elasticsearch REST API and offers a simple and intuitive API for indexing, searching, and managing data in Elasticsearch. ExElasticsearch aims to provide a low-level API that closely mirrors the Elasticsearch API, making it easy to leverage the full power of Elasticsearch in your Phoenix application.
To use ExElasticsearch in your Phoenix application, follow these steps:
Step 1: Add ExElasticsearch to your dependencies
Start by adding ExElasticsearch to the mix.exs
file of your Phoenix project. Open the mix.exs
file, locate the deps
function, and add the following line:
{:ex_elasticsearch, "~> 0.9"}
This will add ExElasticsearch as a dependency to your project.
Step 2: Fetch and compile dependencies
After adding ExElasticsearch to your dependencies, fetch and compile the dependencies by running the following command in your terminal:
mix deps.get
This will download and compile the required dependencies, including ExElasticsearch.
Step 3: Use ExElasticsearch in your Phoenix application
With ExElasticsearch installed, you can now start using it in your Phoenix application. Refer to the ExElasticsearch documentation for examples and usage instructions: ExElasticsearch Documentation
Elasticsearch Elixir
Elasticsearch Elixir is another library that provides a high-level API for interacting with Elasticsearch in Elixir. It aims to provide a more idiomatic and Elixir-like API compared to the low-level REST API provided by Elasticsearch. Elasticsearch Elixir offers features such as query builders, index management, and pagination, making it easy to work with Elasticsearch in your Phoenix application.
To use Elasticsearch Elixir in your Phoenix application, follow these steps:
Step 1: Add Elasticsearch Elixir to your dependencies
Start by adding Elasticsearch Elixir to the mix.exs
file of your Phoenix project. Open the mix.exs
file, locate the deps
function, and add the following line:
{:elasticsearch, "~> 1.3"}
This will add Elasticsearch Elixir as a dependency to your project.
Step 2: Fetch and compile dependencies
After adding Elasticsearch Elixir to your dependencies, fetch and compile the dependencies by running the following command in your terminal:
mix deps.get
This will download and compile the required dependencies, including Elasticsearch Elixir.
Step 3: Use Elasticsearch Elixir in your Phoenix application
With Elasticsearch Elixir installed, you can now start using it in your Phoenix application. Refer to the Elasticsearch Elixir documentation for examples and usage instructions: Elasticsearch Elixir Documentation
Configuring Phoenix with multiple databases like MySQL, PostgreSQL, and MongoDB
Phoenix, being a flexible web framework, allows you to easily configure and work with multiple databases in your application. Whether you need to connect to MySQL, PostgreSQL, MongoDB, or any other database system, Phoenix's underlying Ecto library provides a unified API to manage and interact with different databases. In this section, we will explore how to configure Phoenix with multiple databases like MySQL, PostgreSQL, and MongoDB.
To configure Phoenix with multiple databases, follow these steps:
Step 1: Configure the primary database
Start by configuring the primary database for your Phoenix application. Open the config/config.exs
file of your Phoenix project and locate the config :my_app, MyApp.Repo
section. Replace the existing configuration with the following code:
config :my_app, MyApp.Repo, adapter: Ecto.Adapters.Postgres, database: "primary_database", username: "your_username", password: "your_password", hostname: "localhost", pool_size: 10
Replace "your_username"
, "your_password"
, and "primary_database"
with the appropriate values for your PostgreSQL setup. If your PostgreSQL server is running on a different hostname or port, update the hostname
configuration accordingly.
Step 2: Configure the secondary databases
To configure additional databases, create a new file called secondary_database.exs
in the config
directory of your Phoenix project. In this file, add the following code:
use Mix.Config config :my_app, MyApp.SecondaryRepo, adapter: Ecto.Adapters.MySQL, database: "secondary_database", username: "your_username", password: "your_password", hostname: "localhost", pool_size: 10
Replace "your_username"
, "your_password"
, and "secondary_database"
with the appropriate values for your MySQL setup. If your MySQL server is running on a different hostname or port, update the hostname
configuration accordingly.
Step 3: Create the repository modules
Next, create the repository modules for each database in your Phoenix application. Open the lib/my_app/repo.ex
file and add the following code:
defmodule MyApp.Repo do use Ecto.Repo, otp_app: :my_app, adapter: Ecto.Adapters.Postgres end defmodule MyApp.SecondaryRepo do use Ecto.Repo, otp_app: :my_app, adapter: Ecto.Adapters.MySQL end
Make sure to replace :my_app
with the appropriate OTP application name for your Phoenix project.
Step 4: Use the repositories in your application
With the repositories configured, you can now use them in your Phoenix application. Open the desired module file and add the following code to use the repositories:
defmodule MyApp.MyModule do use MyApp.Web, :controller def index(conn, _params) do primary_data = MyApp.Repo.all(MyApp.PrimaryModel) secondary_data = MyApp.SecondaryRepo.all(MyApp.SecondaryModel) render(conn, "index.html", primary_data: primary_data, secondary_data: secondary_data) end end
Replace MyApp.PrimaryModel
and MyApp.SecondaryModel
with the appropriate Ecto model names for your application.
Integrating Phoenix with MongoDB
Phoenix, being a flexible web framework, allows you to integrate with various database systems, including MongoDB. MongoDB is a popular NoSQL database that provides a flexible and scalable solution for storing and retrieving data. In this section, we will explore how to integrate Phoenix with MongoDB.
To integrate Phoenix with MongoDB, follow these steps:
Step 1: Add the necessary dependencies
Start by adding the mongodb
and mongodb_ecto
packages to the mix.exs
file of your Phoenix project. Open the mix.exs
file, locate the deps
function, and add the following lines:
{:mongodb, ">= 0.0.0"}, {:mongodb_ecto, "~> 1.2"}
This will add the MongoDB driver and the Ecto adapter for MongoDB as dependencies to your project.
Step 2: Configure the database connection
Next, open the config/config.exs
file of your Phoenix project and locate the config :my_app, MyApp.Repo
section. Replace the existing configuration with the following code:
config :my_app, MyApp.Repo, adapter: Mongo.Ecto, database: "your_database", username: "your_username", password: "your_password", hostname: "localhost", pool_size: 10
Replace "your_username"
, "your_password"
, and "your_database"
with the appropriate values for your MongoDB setup. If your MongoDB server is running on a different hostname or port, update the hostname
configuration accordingly.
Step 3: Generate the MongoDB migration
To generate a migration for your MongoDB collection, run the following command in your terminal:
mix ecto.gen.migration create_collection_name
Replace collection_name
with the name of the collection you want to create. This will generate a new migration file in the priv/repo/migrations
directory of your Phoenix project.
Step 4: Run the database migration
To run the migration and create the collection in your MongoDB database, run the following command in your terminal:
mix ecto.migrate
Ecto will execute the migration and create the collection in your MongoDB database.
Related Article: Integrating Phoenix Web Apps with Payment, Voice & Text
Additional Resources
- Integrating Elasticsearch with Phoenix using Tirexs library