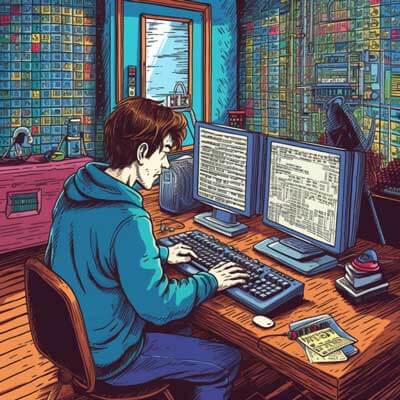
Table of Contents
Overview
The isalpha()
function is a built-in method in Python that is used to check if all the characters in a given string are alphabetic. In other words, it returns True
if all the characters in the string are alphabets (lowercase or uppercase), and False
otherwise.
This function is particularly useful when you need to validate user input or perform string manipulation tasks that involve checking for alphabetic characters. By using the isalpha()
function, you can easily determine whether a string consists only of letters, without the need for complex regular expressions or loops.
Related Article: Comparing Substrings in Python
Syntax and Parameters
The syntax for using the isalpha()
function is straightforward:
string.isalpha()
Here, string
refers to the string object that you want to check for alphabetic characters. It is important to note that the isalpha()
function is a method that belongs to the string class, so it can only be called on string objects.
The isalpha()
function does not take any additional parameters. It simply checks whether all the characters in the string are alphabetic.
How to Use isalpha to Check Alphabetic Characters
To use the isalpha()
function, you simply need to call it on the string that you want to check. The function will return True
if all the characters in the string are alphabetic, and False
otherwise.
Here is an example that demonstrates how to use the isalpha()
function:
name = "John" if name.isalpha(): print("The name contains only alphabetic characters.") else: print("The name contains non-alphabetic characters.")
In this example, the isalpha()
function is called on the name
string. Since the string consists only of alphabetic characters, the condition name.isalpha()
evaluates to True
, and the corresponding message is printed.
Examples
Let's consider a scenario where you need to validate user input for a name field in a form. The name should only contain alphabetic characters, and any non-alphabetic characters should be considered as invalid input.
Here is an example that demonstrates how to use the isalpha()
function to validate user input:
def validate_name(name): if name.isalpha(): return True else: return False user_input = input("Enter your name: ") if validate_name(user_input): print("Valid name.") else: print("Invalid name. Please enter only alphabetic characters.")
In this example, the validate_name()
function takes a string as input and checks if it contains only alphabetic characters using the isalpha()
function. If the input is valid, the function returns True
, indicating that the name is valid. Otherwise, it returns False
, indicating that the name is invalid.
The user is prompted to enter their name using the input()
function, and the input is passed to the validate_name()
function. Depending on the result, the appropriate message is displayed.
Related Article: How To Handle Ambiguous Truth Values In Python Arrays
Common Mistakes When Using isalpha
When using the isalpha()
function, it is important to be aware of some common mistakes that can lead to unexpected results:
1. Not considering whitespace: The isalpha()
function returns False
if the string contains any non-alphabetic characters, including whitespace. If you want to allow whitespace in addition to alphabetic characters, you need to handle it separately.
2. Treating special characters as alphabetic: The isalpha()
function only considers alphabetic characters from the English alphabet. It does not recognize special characters or accented letters as alphabetic. If you need to handle such characters, you may need to use additional libraries or functions.
3. Not handling empty strings: The isalpha()
function returns False
for empty strings, as they do not contain any alphabetic characters. If you want to consider empty strings as valid, you need to handle this case separately.
Best Practices
To ensure efficient and accurate usage of the isalpha()
function, consider the following best practices:
1. Validate input before using isalpha()
: Before calling the isalpha()
function, it is recommended to validate the input string for any special requirements or constraints specific to your use case. This can help prevent unexpected results or errors.
2. Handle whitespace and special characters separately: If you want to allow whitespace or special characters in addition to alphabetic characters, consider using additional functions or regular expressions to handle these cases.
3. Consider language-specific requirements: The isalpha()
function only considers alphabetic characters from the English alphabet. If you are working with strings in other languages that have additional alphabetic characters, consider using language-specific libraries or functions for accurate validation.
4. Use isalpha()
in combination with other string methods: The isalpha()
function can be used in combination with other string methods to perform more complex string manipulation tasks. For example, you can use isalpha()
to check if a string consists only of alphabetic characters before converting it to uppercase or lowercase using the upper()
or lower()
methods.
Additional Resources
- Python isalpha method - Programiz