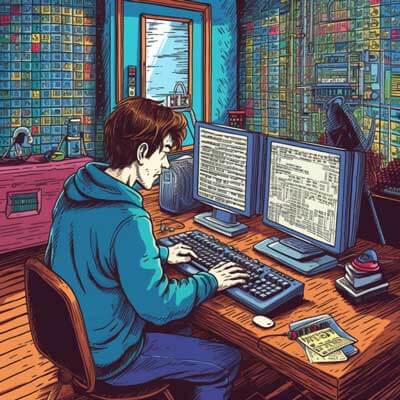
Table of Contents
Introduction to Do While Loop
The do-while loop is a control flow statement in Java that allows a block of code to be executed repeatedly until a certain condition is met. It is similar to the while loop, but with one key difference: the do-while loop guarantees that the block of code is executed at least once, even if the condition is initially false. This makes it particularly useful in situations where you want to ensure that a block of code is executed before checking the condition.
Here's the syntax of the do-while loop:
do { // code to be executed } while (condition);
The flow of execution in a do-while loop is as follows:
1. The block of code inside the do statement is executed.
2. After the code is executed, the condition is checked.
3. If the condition is true, the loop continues to the next iteration, repeating steps 1 and 2.
4. If the condition is false, the loop terminates and the program continues with the next statement after the loop.
Let's dive into some examples to better understand the do-while loop.
Related Article: Top Java Interview Questions and Answers
Syntax of Do While Loop
The syntax of the do-while loop is as follows:
do { // code to be executed } while (condition);
The code block inside the do
statement will be executed once initially, regardless of the condition. After the code block is executed, the condition is checked. If the condition is true, the loop will continue to execute the code block again. If the condition is false, the loop will terminate.
Here's an example that demonstrates the syntax of the do-while loop:
int i = 1; do { System.out.println("Count: " + i); i++; } while (i <= 5);
Output:
Count: 1 Count: 2 Count: 3 Count: 4 Count: 5
In this example, the code block inside the do statement is executed once initially, printing the value of i
. After each iteration, the value of i
is incremented. The condition i <= 5
is checked, and if it evaluates to true, the loop continues to the next iteration. This process is repeated until the condition becomes false.
Flow of Execution in Do While Loop
The flow of execution in a do-while loop is as follows:
1. The block of code inside the do statement is executed.
2. After the code is executed, the condition is checked.
3. If the condition is true, the loop continues to the next iteration, repeating steps 1 and 2.
4. If the condition is false, the loop terminates and the program continues with the next statement after the loop.
Let's consider an example to visualize the flow of execution:
int i = 1; do { System.out.println("Count: " + i); i++; } while (i <= 5); System.out.println("Loop completed");
Output:
Count: 1 Count: 2 Count: 3 Count: 4 Count: 5 Loop completed
In this example, the code block inside the do statement is executed once initially, printing the value of i
. After each iteration, the value of i
is incremented. The condition i <= 5
is checked, and if it evaluates to true, the loop continues to the next iteration. This process is repeated until the condition becomes false. Finally, the statement "Loop completed" is printed after the loop terminates.
Setting Up Your First Do While Loop
To set up your first do-while loop in Java, follow these steps:
1. Declare and initialize any necessary variables outside the loop.
2. Write the code block that you want to repeat inside the do statement.
3. Write the condition that determines when the loop should terminate after the do statement.
Here's an example that demonstrates setting up a simple do-while loop:
int count = 0; do { System.out.println("Count: " + count); count++; } while (count < 5);
Output:
Count: 0 Count: 1 Count: 2 Count: 3 Count: 4
In this example, we declare an integer variable count
and initialize it to 0. The code block inside the do statement prints the value of count
, and then increments it by 1. The condition count < 5
is checked, and if it evaluates to true, the loop continues to the next iteration. This process is repeated until the condition becomes false.
Related Article: How to Retrieve Current Date and Time in Java
Code Snippet: Basic Usage of Do While Loop
int i = 1; do { System.out.println("Count: " + i); i++; } while (i <= 5);
Output:
Count: 1 Count: 2 Count: 3 Count: 4 Count: 5
This code snippet demonstrates the basic usage of the do-while loop. The loop starts with i
initialized to 1. The code block inside the do statement prints the value of i
and increments it by 1. The loop continues until i
becomes greater than 5.
Code Snippet: Nested Do While Loop
int i = 1; int j = 1; do { do { System.out.println("i: " + i + ", j: " + j); j++; } while (j <= 3); i++; j = 1; } while (i <= 2);
Output:
i: 1, j: 1 i: 1, j: 2 i: 1, j: 3 i: 2, j: 1 i: 2, j: 2 i: 2, j: 3
This code snippet demonstrates a nested do-while loop. The outer loop iterates over i
from 1 to 2, and the inner loop iterates over j
from 1 to 3 for each value of i
. The code block inside the inner loop prints the values of i
and j
.