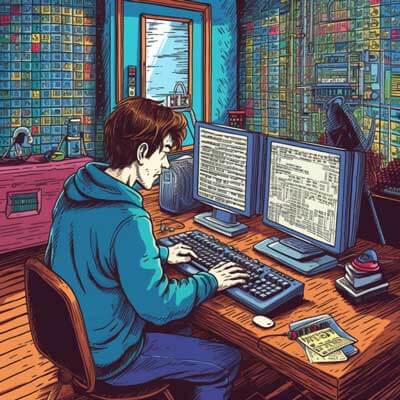
Table of Contents
What is the Go programming language?
The Go programming language, also known as Golang, is an open-source programming language developed by Google. It was designed to be efficient, readable, and scalable, making it popular among developers for building high-performance applications. Go has built-in support for concurrent programming, garbage collection, and a strong type system, making it ideal for managing large data volumes.
Related Article: Golang Tutorial for Backend Development
What is the Beego framework?
Beego is a full-featured and modular web framework for Go that follows the Model-View-Controller (MVC) architectural pattern. It provides a set of useful tools and features to help developers build web applications efficiently. Beego includes built-in support for URL routing, session management, form validation, caching, and internationalization, among other features. It is highly extensible and customizable, allowing developers to tailor it to their specific project requirements.
How does Go handle large volume data?
Go provides several features and tools that make it well-suited for handling large volumes of data efficiently. Some key features include:
1. Concurrency: Go has built-in support for goroutines, lightweight threads that allow for concurrent execution. Goroutines enable developers to process data concurrently, improving performance and scalability.
2. Channels: Go's channel construct allows goroutines to communicate and synchronize data. Channels provide a safe and efficient way to send and receive data between goroutines, making it easier to handle large volumes of data concurrently.
3. Garbage Collection: Go's garbage collector automatically manages memory, freeing up resources that are no longer in use. This helps prevent memory leaks and ensures efficient memory usage, even when dealing with large data volumes.
4. Efficient Data Structures: Go provides a rich set of built-in data structures, such as maps, slices, and arrays, that are optimized for performance. These data structures enable efficient manipulation and storage of large data volumes.
How can I use Beego for handling large datasets?
Beego provides several features and techniques that can be used to handle large datasets effectively. In this article, we will explore three key areas: stream processing, bulk data handling, and optimization techniques for pagination, filtering, and searching.
Related Article: Deployment and Monitoring Strategies for Gin Apps
Stream processing in Beego
Stream processing is a technique used to process data in real-time as it becomes available. Beego provides built-in support for stream processing through its robust request handling capabilities. By leveraging Beego's request handling features, developers can efficiently process and handle large volumes of data as it flows through the application.
Here's an example of how to implement stream processing in Beego:
package controllers import ( "github.com/astaxie/beego" ) type StreamController struct { beego.Controller } func (c *StreamController) Post() { // Read incoming data from the request body data := c.Ctx.Input.RequestBody // Process the data stream // ... // Return a response c.Data["json"] = map[string]interface{}{ "message": "Data processed successfully", } c.ServeJSON() }
In the above example, we define a StreamController
that handles POST requests. The incoming data is read from the request body using c.Ctx.Input.RequestBody
. The data can then be processed as required, and a response is sent back to the client.
Bulk data handling in Beego
Handling bulk data efficiently is crucial when dealing with large datasets. Beego provides features and techniques that make it easy to handle bulk data operations.
One approach is to use the orm
package, which is included with Beego, to interact with databases. The orm
package provides an Object-Relational Mapping (ORM) layer that simplifies database operations and improves performance. It supports bulk operations such as batch inserts, updates, and deletes, which are essential when dealing with large datasets.
Here's an example of using the orm
package for bulk data handling in Beego:
package models import ( "github.com/astaxie/beego/orm" ) type User struct { Id int Name string Age int } func InsertUsers(users []User) error { o := orm.NewOrm() // Begin a transaction err := o.Begin() if err != nil { return err } // Insert users in batches for i := 0; i < len(users); i += 1000 { end := i + 1000 if end > len(users) { end = len(users) } batch := users[i:end] // Insert the batch of users _, err := o.InsertMulti(len(batch), batch) if err != nil { o.Rollback() return err } } // Commit the transaction err = o.Commit() if err != nil { o.Rollback() return err } return nil }
In the above example, we define a User
struct to represent a user entity. The InsertUsers
function takes a slice of User
objects and inserts them in batches using the InsertMulti
function provided by the orm
package.
Pagination optimization in large datasets
When working with large datasets, pagination is a common technique used to retrieve and display data in smaller chunks. Beego provides built-in support for pagination, allowing developers to efficiently retrieve and display large datasets in a controlled manner.
Here's an example of pagination optimization using Beego:
package controllers import ( "github.com/astaxie/beego" "github.com/astaxie/beego/orm" ) type UserController struct { beego.Controller } func (c *UserController) List() { pageNum, _ := c.GetInt("page", 1) pageSize, _ := c.GetInt("size", 10) o := orm.NewOrm() // Query users with pagination var users []*User _, err := o.QueryTable("user").Limit(pageSize, (pageNum-1)*pageSize).All(&users) if err != nil { c.Data["json"] = map[string]interface{}{ "error": err.Error(), } } else { c.Data["json"] = users } c.ServeJSON() }
In the above example, we define a UserController
with a List
method that retrieves a list of users with pagination. The page
and size
query parameters are used to specify the page number and page size, respectively. The Limit
function is used to limit the number of results returned by the query, based on the specified page number and page size.
Filtering optimization in large datasets
Filtering large datasets efficiently is essential for delivering fast and relevant results to users. Beego provides various techniques for optimizing filtering operations on large datasets.
One approach is to use the orm
package's query builder to construct complex queries with filtering conditions. The query builder provides a fluent interface that allows developers to chain filtering conditions and apply them to the query.
Here's an example of filtering optimization using the orm
package in Beego:
package controllers import ( "github.com/astaxie/beego" "github.com/astaxie/beego/orm" ) type UserController struct { beego.Controller } func (c *UserController) List() { query := c.GetString("query") o := orm.NewOrm() // Query users with filtering conditions var users []*User qs := o.QueryTable("user") if query != "" { qs = qs.Filter("name__icontains", query) } _, err := qs.All(&users) if err != nil { c.Data["json"] = map[string]interface{}{ "error": err.Error(), } } else { c.Data["json"] = users } c.ServeJSON() }
In the above example, we define a UserController
with a List
method that retrieves a list of users with filtering conditions. The query
query parameter is used to specify the filtering condition, which in this case is a case-insensitive search for users whose name contains the specified query string. The Filter
function is used to add the filtering condition to the query.
Related Article: Intergrating Payment, Voice and Text with Gin & Golang
Asynchronous tasks in Beego
Asynchronous tasks are often necessary when dealing with large datasets to avoid blocking the main execution thread. Beego provides support for running asynchronous tasks through goroutines and channels.
Here's an example of running an asynchronous task in Beego:
package controllers import ( "github.com/astaxie/beego" ) type TaskController struct { beego.Controller } func (c *TaskController) Run() { go func() { // Perform time-consuming task asynchronously // ... // Update task status or send result // ... }() c.Data["json"] = map[string]interface{}{ "message": "Task started successfully", } c.ServeJSON() }
In the above example, we define a TaskController
with a Run
method that starts an asynchronous task. The task is executed in a goroutine, allowing the main execution thread to continue without waiting for the task to complete. This enables the application to handle other requests or perform other tasks concurrently.
Background job processing in Beego
Background job processing is a common requirement when dealing with large datasets or time-consuming tasks. Beego provides support for background job processing through various mechanisms such as message queues and task schedulers.
One popular approach for background job processing in Beego is to use a message queue system like RabbitMQ or Redis. The message queue can be used to enqueue background jobs, which are then processed by separate worker processes.
Here's an example of background job processing using a message queue in Beego:
package controllers import ( "github.com/astaxie/beego" "github.com/streadway/amqp" ) type JobController struct { beego.Controller } func (c *JobController) Enqueue() { conn, err := amqp.Dial("amqp://guest:guest@localhost:5672/") if err != nil { c.Data["json"] = map[string]interface{}{ "error": err.Error(), } c.ServeJSON() return } defer conn.Close() ch, err := conn.Channel() if err != nil { c.Data["json"] = map[string]interface{}{ "error": err.Error(), } c.ServeJSON() return } defer ch.Close() // Declare a queue to enqueue jobs queue, err := ch.QueueDeclare( "jobs", // Queue name false, // Durable false, // Delete when unused false, // Exclusive false, // No-wait nil, // Arguments ) if err != nil { c.Data["json"] = map[string]interface{}{ "error": err.Error(), } c.ServeJSON() return } // Publish a message to the queue err = ch.Publish( "", // Exchange queue.Name, // Routing key false, // Mandatory false, // Immediate amqp.Publishing{ ContentType: "text/plain", Body: []byte("job payload"), }) if err != nil { c.Data["json"] = map[string]interface{}{ "error": err.Error(), } } else { c.Data["json"] = map[string]interface{}{ "message": "Job enqueued successfully", } } c.ServeJSON() }
In the above example, we define a JobController
with an Enqueue
method that enqueues a background job. We use the amqp
package to establish a connection to a RabbitMQ server and publish a message to a queue named "jobs". The worker processes can then consume the messages from the queue and process the background jobs asynchronously.