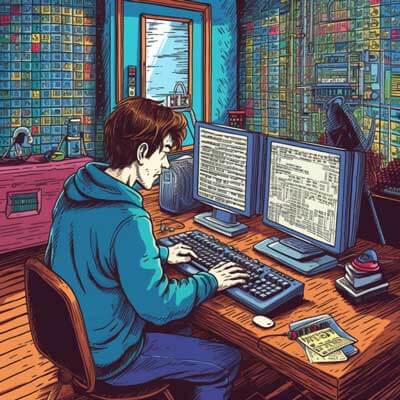
Table of Contents
ActiveRecord
ActiveRecord is an Object-Relational Mapping (ORM) library in Ruby that provides an interface between Ruby objects and relational databases. It allows developers to interact with databases using Ruby code, abstracting away the complexities of SQL queries and database connections. ActiveRecord follows the active record pattern, where each database table is represented by a Ruby class, and each instance of that class represents a row in the table.
To use ActiveRecord in your Ruby application, you first need to install the activerecord
gem. You can do this by adding the following line to your Gemfile:
gem 'activerecord'
Then, run bundle install
to install the gem.
Next, you need to establish a database connection in your application. ActiveRecord supports multiple database adapters, including MySQL, PostgreSQL, SQLite, and more. To establish a connection, you need to configure the database settings in your application's configuration file. Here's an example configuration for a SQLite database:
require 'active_record' ActiveRecord::Base.establish_connection( adapter: 'sqlite3', database: 'path/to/database.sqlite3' )
Once you have established a database connection, you can define ActiveRecord models to represent your database tables. A model is a Ruby class that inherits from ActiveRecord::Base
. Let's say you have a users
table in your database. Here's how you can define a User
model:
class User < ActiveRecord::Base end
With the User
model defined, you can now perform CRUD (Create, Read, Update, Delete) operations on the users
table using ActiveRecord methods. For example, to retrieve all users from the database, you can use the all
method:
users = User.all
To create a new user, you can use the create
method:
user = User.create(name: 'John Doe', email: 'john@example.com')
To update an existing user, you can use the update
method:
user.update(name: 'Jane Doe')
And to delete a user, you can use the destroy
method:
user.destroy
These are just some basic examples of how to use ActiveRecord in Ruby. The library provides many more features and methods for querying and manipulating data in your databases. You can refer to the official ActiveRecord documentation for more information and examples.
Related Article: Ruby on Rails with SSO, API Versioning & Audit Trails
Working with Databases in Ruby
Ruby provides several libraries and frameworks for working with databases. In addition to ActiveRecord, which we discussed in the previous section, there are other popular libraries that can be used for interacting with databases in Ruby, such as Sequel and DataMapper.
Sequel is a lightweight and flexible ORM library that supports a wide range of database adapters. It provides a clean and intuitive API for querying and manipulating data in databases. To use Sequel in your Ruby application, you need to install the sequel
gem by adding the following line to your Gemfile:
gem 'sequel'
Then, run bundle install
to install the gem.
To establish a database connection with Sequel, you can use the Sequel.connect
method and pass in the appropriate database URL or connection settings. Here's an example for connecting to a PostgreSQL database:
require 'sequel' DB = Sequel.connect('postgres://username:password@localhost/mydatabase')
Once you have established a database connection, you can define models in Sequel using the Sequel::Model
class. Let's say you have a books
table in your database. Here's how you can define a Book
model:
class Book < Sequel::Model end
With the Book
model defined, you can perform CRUD operations on the books
table using Sequel methods. For example, to retrieve all books from the database, you can use the all
method:
books = Book.all
To create a new book, you can use the create
method:
book = Book.create(title: 'Ruby Programming', author: 'John Doe')
To update an existing book, you can use the update
method:
book.update(title: 'Ruby Programming 101')
And to delete a book, you can use the delete
method:
book.delete
Sequel also provides a useful query DSL (Domain-Specific Language) for constructing complex SQL queries. You can use methods like where
, order
, limit
, and offset
to filter, sort, and paginate data. Here's an example:
books = Book.where(author: 'John Doe').order(:title).limit(10).offset(0)
This will retrieve the first 10 books written by John Doe, ordered by title.
These are just some basic examples of how to work with databases in Ruby using the Sequel library. Sequel offers many more features and options for database interactions. You can refer to the official Sequel documentation for more information and examples.
Implementing Pagination in Ruby
Pagination is a common technique used to divide large volumes of data into smaller, more manageable pages. It allows users to navigate through the data easily and improves the performance of your application by reducing the amount of data retrieved from the database at once.
In Ruby, there are several libraries and techniques available for implementing pagination, such as Kaminari, will_paginate, and endless page.
Kaminari
Kaminari is a popular pagination library for Ruby on Rails applications. It provides a simple and flexible API for implementing pagination in your views. To use Kaminari in your Rails application, you need to add the kaminari
gem to your Gemfile:
gem 'kaminari'
Then, run bundle install
to install the gem.
Once you have installed the Kaminari gem, you can use the paginate
method in your controller to paginate the data. Here's an example:
def index @users = User.order(:name).paginate(page: params[:page], per_page: 10) end
In the above example, the paginate
method is called on the User
model with the page
parameter set to the current page number and the per_page
parameter set to the number of records to display per page (in this case, 10).
In your view, you can use the paginate
helper to display the pagination links. Here's an example:
<%= paginate @users %>
This will render the pagination links based on the current page and the total number of pages.
Kaminari also provides additional features, such as customizing the appearance of the pagination links, handling AJAX requests, and more. You can refer to the official Kaminari documentation for more information and examples.
Related Article: Ruby on Rails with Internationalization and Character Encoding
will_paginate
will_paginate is another popular pagination library for Ruby on Rails applications. It provides a similar API to Kaminari and is widely used in older Rails applications. To use will_paginate in your Rails application, you need to add the will_paginate
gem to your Gemfile:
gem 'will_paginate'
Then, run bundle install
to install the gem.
Once you have installed the will_paginate gem, you can use the paginate
method in your controller to paginate the data. Here's an example:
def index @users = User.order(:name).paginate(page: params[:page], per_page: 10) end
In the above example, the paginate
method is called on the User
model with the page
parameter set to the current page number and the per_page
parameter set to the number of records to display per page (in this case, 10).
In your view, you can use the will_paginate
helper to display the pagination links. Here's an example:
<%= will_paginate @users %>
This will render the pagination links based on the current page and the total number of pages.
will_paginate also provides additional features, such as customizing the appearance of the pagination links, handling AJAX requests, and more. You can refer to the official will_paginate documentation for more information and examples.
Endless Page
Endless Page is a technique for implementing infinite scrolling pagination in Ruby on Rails applications. It allows you to load more data as the user scrolls down the page, without the need for explicit pagination links.
To implement endless page in your Rails application, you can use the will_paginate
gem along with some JavaScript code. Here's an example:
In your controller, you can use the paginate
method as usual:
def index @users = User.order(:name).paginate(page: params[:page], per_page: 10) end
In your view, you can render the initial set of data:
<%= render @users %>
Then, you can add JavaScript code to your view to detect when the user reaches the bottom of the page and load more data using AJAX:
$(window).scroll(function() { if ($(window).scrollTop() + $(window).height() >= $(document).height()) { var nextPage = <%= @users.current_page + 1 %>; var url = '<%= users_path %>?page=' + nextPage; $.get(url, function(data) { $('#users').append(data); }); } });
This JavaScript code listens for the scroll
event on the window and checks if the user has reached the bottom of the page. If so, it makes an AJAX request to the next page of data and appends it to the existing data.
In your controller, you need to handle the AJAX request and render the partial view for the next page:
def index @users = User.order(:name).paginate(page: params[:page], per_page: 10) respond_to do |format| format.html format.js { render 'index.js.erb' } end end
This will render the index.js.erb
template, which contains the JavaScript code to append the next page of data.
Endless Page provides a seamless and interactive pagination experience for users, as they can continue scrolling to load more data without having to click on pagination links. You can refer to the official Endless Page documentation for more information and examples.
Utilizing Batch Processing in Ruby
Batch processing is a technique used to process large volumes of data in smaller, manageable chunks. It allows you to perform operations on a subset of data at a time, reducing memory usage and improving performance.
In Ruby, there are several approaches and libraries available for implementing batch processing, such as the in_batches
method in ActiveRecord, the find_each
method in ActiveRecord, and the each_slice
method in Ruby's Enumerable module.
Using the in_batches
method in ActiveRecord
The in_batches
method in ActiveRecord allows you to process records in batches based on a specified batch size. It is useful when you need to perform an operation on a large dataset without loading all the records into memory at once.
Here's an example of how to use the in_batches
method:
User.in_batches(of: 1000).each do |batch| batch.each do |user| # Perform operation on user end end
In the above example, the in_batches
method is called on the User
model with the of
option set to 1000, which specifies that each batch should contain 1000 records. The each
method is then called on each batch to iterate over the records and perform the desired operation.
The in_batches
method also provides additional options, such as start
, finish
, and load
. You can refer to the official ActiveRecord documentation for more information on these options.
Related Article: Ruby on Rails with Bootstrap, Elasticsearch and Databases
Using the find_each
method in ActiveRecord
The find_each
method in ActiveRecord is similar to the in_batches
method, but it processes records one by one instead of in batches. It is useful when you need to perform an operation on each record individually, without loading all the records into memory at once.
Here's an example of how to use the find_each
method:
User.find_each do |user| # Perform operation on user end
In the above example, the find_each
method is called on the User
model, and the given block is executed for each record. The records are fetched from the database in batches, with each batch containing a certain number of records (the default batch size is 1000, but you can customize it using the batch_size
option).
The advantage of using the find_each
method is that it automatically takes care of the batch processing logic for you, so you don't have to worry about memory issues or performance optimizations. However, it may be slower than using the in_batches
method if you need to perform bulk operations on the records.
Using the each_slice
method in Ruby's Enumerable module
If you're not using ActiveRecord or prefer a more generic approach, you can use the each_slice
method in Ruby's Enumerable module to process data in batches. This method allows you to split an enumerable object (such as an array or a range) into smaller chunks and iterate over them.
Here's an example of how to use the each_slice
method:
users = User.all.to_a users.each_slice(1000) do |batch| batch.each do |user| # Perform operation on user end end
In the above example, the each_slice
method is called on the users
array with the argument 1000
, which specifies that each batch should contain 1000 records. The each
method is then called on each batch to iterate over the records and perform the desired operation.
The each_slice
method is not limited to arrays; it can also be used with other enumerable objects like ranges or custom iterators. This makes it a versatile option for batch processing in Ruby.
These are just some examples of how to utilize batch processing in Ruby. Depending on your specific use case and requirements, you may choose a different approach or library. It's important to consider factors such as memory usage, performance, and ease of implementation when implementing batch processing in your applications.
Exploring Data Streaming Techniques in Ruby
Data streaming is a technique used to process and transmit data in a continuous and non-blocking manner. It allows you to handle large volumes of data efficiently, without having to load the entire dataset into memory at once.
In Ruby, there are several techniques and libraries available for implementing data streaming, such as using the Enumerator class, the Streamio library, and the CSV library.
Using the Enumerator class
The Enumerator class in Ruby provides a way to generate and iterate over a sequence of values. It can be used to implement data streaming by generating and yielding data on-the-fly, instead of loading it all into memory at once.
Here's an example of how to use the Enumerator class for data streaming:
stream = Enumerator.new do |yielder| File.open('data.txt', 'r').each_line do |line| yielder << line.chomp end end stream.each do |data| # Process data end
In the above example, an Enumerator object is created using the Enumerator.new
method. Inside the block, we read lines from a file and yield each line as data. The data is then processed in the outer each
loop.
The advantage of using the Enumerator class is that it allows you to generate and process data on-the-fly, without having to load the entire dataset into memory. This is especially useful when dealing with large files or external data sources.
Related Article: Ruby on Rails Performance Tuning and Optimization
Using the Streamio library
The Streamio library is a Ruby gem that provides a simple and efficient way to stream data from files or external sources. It is built on top of the Enumerator class and provides additional features for handling different types of data, such as binary data or audio/video streams.
To use the Streamio library, you need to install the streamio-ffmpeg
gem by adding the following line to your Gemfile:
gem 'streamio-ffmpeg'
Then, run bundle install
to install the gem.
Once you have installed the Streamio gem, you can use its API to stream data. Here's an example:
require 'streamio-ffmpeg' stream = Streamio::FFMPEG::Reader.open('video.mp4') stream.each_frame do |frame| # Process frame data end
In the above example, we open a video file using the Streamio::FFMPEG::Reader.open
method and iterate over each frame using the each_frame
method. Inside the block, we can process the frame data as needed.
The Streamio library provides support for streaming various types of data, such as audio, video, images, and more. You can refer to the official Streamio documentation for more information and examples.
Using the CSV library
The CSV library in Ruby provides a way to read and write CSV (Comma-Separated Values) files. It can also be used for data streaming by reading data from a CSV file line by line, instead of loading the entire file into memory.
Here's an example of how to use the CSV library for data streaming:
require 'csv' CSV.foreach('data.csv') do |row| # Process row data end
In the above example, we use the CSV.foreach
method to read rows from a CSV file and process each row in the given block. This allows us to handle large CSV files efficiently, without having to load the entire file into memory.
The CSV library provides various options and methods for customizing the behavior of CSV parsing, such as specifying the field separator, handling headers, and more. You can refer to the official CSV documentation for more information and examples.
These are just some examples of how to explore data streaming techniques in Ruby. Depending on your specific use case and requirements, you may choose a different approach or library. It's important to consider factors such as data size, performance, and ease of implementation when implementing data streaming in your applications.
Implementing Data Archival in Ruby
Data archival is a process of moving data from the primary storage to a secondary storage for long-term retention. It is often used to free up space in the primary storage, improve database performance, and comply with data retention policies.
In Ruby, there are several techniques and libraries available for implementing data archival, such as using database queries, creating backup files, and using third-party archival services.
Using database queries
One way to implement data archival in Ruby is by using database queries to move data from the primary storage to an archival storage. This can be done by selecting the data to be archived based on certain criteria, such as a date range or specific attributes, and then inserting the selected data into a separate archival table or database.
Here's an example of how to implement data archival using database queries in Ruby:
archived_users = User.where('created_at < ?', 1.year.ago) archived_users.each do |user| ArchivedUser.create(name: user.name, email: user.email) user.destroy end
In the above example, we select all users who were created more than one year ago using the where
method with a condition on the created_at
attribute. We then iterate over each archived user, create a corresponding record in the ArchivedUser
table, and delete the original user record.
This approach allows you to move the data from the primary storage to a separate archival table or database, effectively archiving the data while keeping it accessible if needed in the future.
Related Article: Ruby on Rails with Modular Rails, ActiveRecord & Testing
Creating backup files
Another way to implement data archival in Ruby is by creating backup files of the data to be archived. This can be done by exporting the data to a file format like CSV or JSON, compressing the file, and storing it in a secondary storage.
Here's an example of how to implement data archival by creating backup files in Ruby:
require 'csv' archived_users = User.where('created_at < ?', 1.year.ago) CSV.open('archive.csv', 'w') do |csv| archived_users.each do |user| csv << [user.name, user.email] user.destroy end end
In the above example, we select all users who were created more than one year ago using the where
method with a condition on the created_at
attribute. We then iterate over each archived user, write their data to a CSV file using the CSV.open
method, and delete the original user record.
This approach allows you to create backup files of the data, which can be stored in a secondary storage like a file server or cloud storage. The backup files can then be restored if needed in the future.
Using third-party archival services
Alternatively, you can implement data archival in Ruby by using third-party archival services. These services provide a scalable and secure way to archive data, often with features like automatic data retention policies, redundancy, and data encryption.
One popular third-party archival service is Amazon S3 (Simple Storage Service). S3 provides an API that allows you to store and retrieve data in the cloud. You can use the AWS SDK for Ruby (aws-sdk-s3 gem) to interact with S3 and implement data archival.
Here's an example of how to implement data archival using Amazon S3 in Ruby:
require 'aws-sdk-s3' s3 = Aws::S3::Resource.new(region: 'us-east-1') bucket = s3.bucket('my-bucket') archived_users = User.where('created_at < ?', 1.year.ago) archived_users.each do |user| object = bucket.object("archive/#{user.id}.json") object.put(body: { name: user.name, email: user.email }.to_json) user.destroy end
In the above example, we create an instance of the AWS S3 resource using the Aws::S3::Resource.new
method and specify the region and bucket name. We then select all users who were created more than one year ago using the where
method with a condition on the created_at
attribute. We iterate over each archived user, create an S3 object with a unique key based on the user ID, put the user data as a JSON object in the object, and delete the original user record.
This approach allows you to leverage the scalability and durability of Amazon S3 for data archival, ensuring the long-term retention and accessibility of the archived data.
These are just some examples of how to implement data archival in Ruby. Depending on your specific use case and requirements, you may choose a different approach or library. It's important to consider factors such as data size, performance, security, and compliance when implementing data archival in your applications.
Understanding Data Partitioning in Ruby
Data partitioning is a technique used to divide a large dataset into smaller, more manageable partitions. Each partition contains a subset of the data and is stored separately, allowing for improved performance and scalability.
In Ruby, there are several approaches and libraries available for implementing data partitioning, such as using database partitioning, sharding, and using third-party partitioning tools.
Using database partitioning
One way to implement data partitioning in Ruby is by using database partitioning techniques provided by database management systems (DBMS). Database partitioning allows you to divide a table into smaller logical units called partitions, based on a specific criteria such as a range of values or a hash function.
Here's an example of how to implement data partitioning using database partitioning in Ruby with PostgreSQL:
class User < ActiveRecord::Base self.partition_key = :created_at self.partition_type = :range partition_by_range :created_at, start_date: Date.new(2022, 1, 1), interval: '1 month' end
In the above example, we define a User
model in Ruby using ActiveRecord and specify the partition key as created_at
, which means the table will be partitioned based on the created_at
attribute. We also specify the partition type as range
and provide additional options for partitioning by range, such as the start date and the interval (in this case, one month).
With database partitioning set up, the data will be automatically distributed across multiple partitions based on the specified criteria. This allows for efficient data retrieval and improved performance, especially when dealing with large datasets.
It's worth noting that the specific syntax and features for implementing database partitioning may vary depending on the DBMS you are using. It's recommended to consult the documentation of your DBMS for more information on how to implement data partitioning.
Related Article: Ruby on Rails with WebSockets & Real-time Features
Using sharding
Another way to implement data partitioning in Ruby is by using sharding techniques. Sharding involves splitting a dataset across multiple database servers, also known as shards, based on a specific criteria such as a hash function or a consistent hashing algorithm.
Here's an example of how to implement data partitioning using sharding in Ruby:
class User < ActiveRecord::Base def self.shard_key(user_id) user_id % 4 end end
In the above example, we define a User
model in Ruby using ActiveRecord and define a shard_key
method that takes a user_id
as input and returns the shard key. In this case, we use a simple modulo operation to determine the shard key based on the user ID.
With sharding set up, the data will be distributed across multiple shards based on the shard key. This allows for horizontal scaling and improved performance, as each shard can handle a subset of the data.
It's important to note that sharding introduces additional complexity, such as managing multiple database connections and handling data consistency across shards. There are also libraries and frameworks available for implementing sharding in Ruby, such as octopus
and sharding-by-key
. These libraries provide abstractions and tools to simplify the sharding process and handle common challenges.
Using third-party partitioning tools
Alternatively, you can implement data partitioning in Ruby by using third-party partitioning tools or libraries. These tools provide a higher-level abstraction for managing and distributing data across multiple partitions or shards.
One popular third-party partitioning tool is Apache Kafka. Kafka is a distributed streaming platform that allows you to publish and subscribe to streams of records in a fault-tolerant and scalable manner. It provides built-in partitioning and replication mechanisms, allowing you to distribute data across multiple Kafka brokers.
To use Apache Kafka for data partitioning in Ruby, you need to install the ruby-kafka
gem by adding the following line to your Gemfile:
gem 'ruby-kafka'
Then, run bundle install
to install the gem.
Once you have installed the Ruby Kafka gem, you can use its API to produce and consume data from Kafka topics. Here's an example:
require 'kafka' kafka = Kafka.new(['localhost:9092'], client_id: 'my-application') producer = kafka.producer producer.produce('my-topic', key: 'key1', value: 'value1') producer.deliver_messages consumer = kafka.consumer(group_id: 'my-group') consumer.subscribe('my-topic') consumer.each_message do |message| puts "Received message: #{message.value}" end
In the above example, we create a Kafka producer and produce a message to the my-topic
topic. We then create a Kafka consumer, subscribe to the my-topic
topic, and process each received message in the given block.
Apache Kafka provides a scalable and fault-tolerant solution for data partitioning, allowing you to distribute data across multiple brokers and handle high-throughput data streams.
These are just some examples of how to implement data partitioning in Ruby. Depending on your specific use case and requirements, you may choose a different approach or library. It's important to consider factors such as data size, performance, scalability, and ease of implementation when implementing data partitioning in your applications.
Exploring Data Warehousing Solutions in Ruby
Data warehousing is a technique used to store and analyze large volumes of data from various sources in a centralized repository. It provides a way to consolidate and integrate data from different systems, making it easier to perform complex analytics and generate meaningful insights.
In Ruby, there are several techniques and libraries available for implementing data warehousing, such as using relational databases, columnar databases, and third-party data warehousing services.
Using relational databases
One way to implement data warehousing in Ruby is by using relational databases, such as PostgreSQL or MySQL. Relational databases provide a structured and efficient way to store and query data, making them suitable for data warehousing applications.
To implement data warehousing using a relational database, you need to design a schema that can accommodate the data from different sources and support complex queries. This typically involves creating tables, defining relationships between tables, and optimizing the database schema for analytics queries.
Here's an example of how to implement data warehousing using a relational database in Ruby with PostgreSQL:
class User < ActiveRecord::Base establish_connection( adapter: 'postgresql', host: 'localhost', database: 'mydatawarehouse' ) end
In the above example, we define a User
model in Ruby using ActiveRecord and establish a connection to a PostgreSQL database. This database will serve as the data warehouse for storing and querying data.
With the database connection established, you can define additional models and tables to represent the data from different sources. You can also define relationships between tables using foreign keys or join tables to support complex queries.
Using a relational database for data warehousing provides flexibility, scalability, and the ability to handle complex queries. However, it may require careful database design and optimization to ensure optimal performance for analytics queries.
Using columnar databases
Another way to implement data warehousing in Ruby is by using columnar databases, such as Apache Cassandra or Amazon Redshift. Columnar databases store data in columns rather than rows, allowing for efficient compression and faster data retrieval for analytics queries.
To implement data warehousing using a columnar database, you need to design a schema that can accommodate the data from different sources and support columnar storage. This typically involves creating tables with appropriate column definitions, defining data types, and optimizing the database schema for analytics queries.
Here's an example of how to implement data warehousing using a columnar database in Ruby with Apache Cassandra:
require 'cassandra' cluster = Cassandra.cluster(hosts: ['localhost']) session = cluster.connect('mydatawarehouse') session.execute("CREATE TABLE users (id UUID PRIMARY KEY, name TEXT, email TEXT)")
In the above example, we establish a connection to an Apache Cassandra database using the Cassandra.cluster
method and create a session for executing queries. We then execute a CREATE TABLE statement to create a table named users
with the specified columns.
With the database connection established and the table created, you can insert data into the table and perform queries using the session object.
Using a columnar database for data warehousing provides fast data retrieval, efficient storage, and the ability to scale horizontally. However, it may require specialized knowledge and tools for managing and optimizing the database.
Using third-party data warehousing services
Alternatively, you can implement data warehousing in Ruby by using third-party data warehousing services. These services provide a fully managed and scalable solution for storing and analyzing large volumes of data, without the need for managing infrastructure or database administration.
One popular third-party data warehousing service is Amazon Redshift. Redshift is a fully managed data warehousing service that allows you to analyze large datasets using SQL queries. It provides high performance, scalability, and integration with other AWS services.
To use Amazon Redshift for data warehousing in Ruby, you need to install the aws-sdk-redshift
gem by adding the following line to your Gemfile:
gem 'aws-sdk-redshift'
Then, run bundle install
to install the gem.
Once you have installed the AWS Redshift gem, you can use its API to interact with Redshift. Here's an example:
require 'aws-sdk-redshift' client = Aws::Redshift::Client.new(region: 'us-east-1') response = client.create_cluster({ cluster_identifier: 'my-cluster', node_type: 'dc2.large', cluster_type: 'single-node', publicly_accessible: true, master_username: 'admin', master_user_password: 'password', db_name: 'mydatawarehouse' })
In the above example, we create an instance of the AWS Redshift client using the Aws::Redshift::Client.new
method and specify the region. We then use the create_cluster
method to create a Redshift cluster with the specified configuration.
With the Redshift cluster created, you can connect to it using a SQL client and perform data warehousing tasks, such as creating tables, loading data, and running analytics queries.
Using a third-party data warehousing service like Amazon Redshift provides a scalable and fully managed solution for data warehousing, allowing you to focus on data analysis rather than infrastructure management.
These are just some examples of how to explore data warehousing solutions in Ruby. Depending on your specific use case and requirements, you may choose a different approach or service. It's important to consider factors such as data size, performance, scalability, cost, and ease of implementation when implementing data warehousing in your applications.