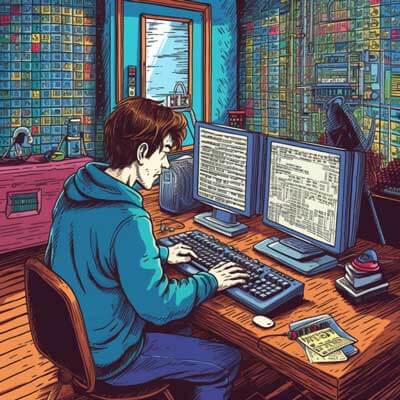
Table of Contents
Query parameters are a way to pass data in the URL of a web page. They are commonly used to provide additional information to a server or to share data between different pages or components.
In React, query parameters can be accessed using the react-router-dom
library, which provides routing capabilities.
To use query parameters in React, you need to install the react-router-dom
library as a dependency in your project:
npm install react-router-dom
Once installed, you can define routes in your application and access query parameters using the useLocation
hook from react-router-dom
.
Here is an example of accessing query parameters in React:
import React from 'react'; import { useLocation } from 'react-router-dom'; function UserProfile() { const location = useLocation(); const searchParams = new URLSearchParams(location.search); const username = searchParams.get('username'); return <h1>Welcome, {username}!</h1>; } export default UserProfile;
In this example, the useLocation
hook is used to access the current location object from the react-router-dom
library. The search
property of the location object contains the query parameters.
The URLSearchParams
constructor is used to parse the query parameters and create a SearchParams
object. The get
method of the SearchParams
object is then used to retrieve the value of the username
query parameter.
To pass query parameters in a URL, you can use the Link
component from react-router-dom
:
import React from 'react'; import { Link } from 'react-router-dom'; function HomePage() { return ( <div> <h1>Welcome to my website!</h1> <Link to="/user-profile?username=john">Go to John's profile</Link> </div> ); } export default HomePage;
In this example, the Link
component is used to create a link to the UserProfile
component with the username
query parameter set to "john".
Query parameters can also be accessed in class components using the this.props.location
object:
import React from 'react'; class UserProfile extends React.Component { render() { const searchParams = new URLSearchParams(this.props.location.search); const username = searchParams.get('username'); return <h1>Welcome, {username}!</h1>; } } export default UserProfile;
In this example, the location
object is accessed from the props
object in the render
method of the component.
React Components
React components are the building blocks of a React application. They are reusable, self-contained pieces of code that can be rendered to the DOM. Each component can have its own logic, state, and properties. React components can be divided into two types: functional components and class components.
Functional components are simpler and easier to understand. They are JavaScript functions that take in props as input and return JSX (JavaScript XML) as output. Here is an example of a functional component:
import React from 'react'; function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } export default Greeting;
Class components are more useful and allow for more complex logic. They are JavaScript classes that extend the React.Component
class. They have a render method that returns JSX. Here is an example of a class component:
import React from 'react'; class Greeting extends React.Component { render() { return <h1>Hello, {this.props.name}!</h1>; } } export default Greeting;
Both functional and class components can be used interchangeably in a React application. However, functional components are recommended for most use cases as they are simpler and easier to test.
Related Article: Implementing HTML Templates in ReactJS
React Props
In React, props (short for properties) are used to pass data from a parent component to a child component. Props are read-only and cannot be directly modified by the child component. They are passed down as function arguments in functional components and as properties of the this.props
object in class components.
Here is an example of a parent component passing a prop to a child component:
import React from 'react'; import Greeting from './Greeting'; function App() { return <Greeting name="John" />; } export default App;
In the above example, the parent component App
passes the prop name
with the value "John" to the Greeting
component. The Greeting
component can then access this prop using the props
object:
import React from 'react'; function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } export default Greeting;
The Greeting
component receives the prop name
and uses it to render the greeting "Hello, John!".
Props can be any JavaScript value, including strings, numbers, booleans, objects, and even functions. They can also be used to pass event handlers from parent components to child components.
React State
In React, state is used to manage component-specific data that can change over time. Unlike props, state is mutable and can be updated within a component. Changes to state trigger a re-render of the component, updating the UI to reflect the new state.
State is declared and initialized in the constructor of a class component using the this.state
object. Here is an example:
import React from 'react'; class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.setState({ count: this.state.count + 1 })}> Increment </button> </div> ); } } export default Counter;
In the above example, the Counter
component has a state property count
initialized to 0. The current count is rendered in the UI using {this.state.count}
. When the "Increment" button is clicked, the onClick
event handler updates the state by calling this.setState({ count: this.state.count + 1 })
.
It's important to note that state should be treated as immutable and updated using the setState
method. Directly modifying the state object can lead to unexpected behavior.
Functional components can also have state using React Hooks, which we will cover later in this article.
React Context
React Context is a feature that allows data to be passed down the component tree without explicitly passing props at every level. It provides a way to share data between components that are not directly connected in the component hierarchy.
Context consists of two components: Provider
and Consumer
. The Provider
component wraps the parent component and provides the data to be shared. The Consumer
component is used by child components to access the shared data.
Here is an example of using React Context:
import React from 'react'; // Create a new context const ThemeContext = React.createContext(); // Wrap the parent component with the Provider function App() { return ( <ThemeContext.Provider value="dark"> <Toolbar /> </ThemeContext.Provider> ); } // Child component consuming the context value function Toolbar() { return ( <ThemeContext.Consumer> {theme => <div>Current theme: {theme}</div>} </ThemeContext.Consumer> ); } export default App;
In the above example, the App
component wraps the Toolbar
component with the ThemeContext.Provider
component. The value "dark" is passed as the context value.
The Toolbar
component uses the ThemeContext.Consumer
component to access the context value, which is then rendered in the UI.
Context can be useful when passing down data such as user authentication, theme settings, or language preferences to multiple components without having to pass props through every intermediate component.
Related Article: Adding a Newline in the JSON Property Render with ReactJS
React Event Handling
React provides a simple and intuitive way to handle events in components. Event handlers are functions that are invoked when a specific event occurs, such as a button click or a form submission.
In React, event handlers are defined as methods within a class component.
Here is an example of handling a button click event:
import React from 'react'; class Button extends React.Component { handleClick() { console.log('Button clicked!'); } render() { return <button onClick={this.handleClick}>Click me</button>; } } export default Button;
In the above example, the Button
component has a handleClick
method that logs a message to the console when the button is clicked. The onClick
event handler is set to the handleClick
method.
Event handlers can also be defined inline using arrow functions:
import React from 'react'; class Button extends React.Component { render() { return <button onClick={() => console.log('Button clicked!')}>Click me</button>; } } export default Button;
In this example, the onClick
event handler is defined inline as an arrow function.
Event handlers can also receive parameters and access the event object:
import React from 'react'; class Button extends React.Component { handleClick(event) { console.log('Button clicked!', event.target); } render() { return <button onClick={this.handleClick}>Click me</button>; } } export default Button;
In this example, the handleClick
method receives the event
object as a parameter and logs the target element when the button is clicked.
React event handling follows the same conventions as standard HTML event handling. The event
object contains useful information about the event, such as the target element, event type, and event modifiers.
React Lifecycle Methods
React lifecycle methods are special methods that are automatically called at different stages of a component's lifecycle. They allow you to hook into these stages and perform certain actions, such as initializing state, fetching data, or cleaning up resources.
Class components have several lifecycle methods that can be overridden to control the component's behavior. The most commonly used lifecycle methods are:
- componentDidMount
: Called after the component is rendered for the first time. It is commonly used to fetch data from an API or initialize subscriptions.
- componentDidUpdate
: Called after the component's updates have been applied to the DOM. It is commonly used to perform side effects based on changes in props or state.
- componentWillUnmount
: Called before the component is removed from the DOM. It is commonly used to clean up resources, such as cancelling timers or unsubscribing from subscriptions.
Here is an example of using the componentDidMount
lifecycle method:
import React from 'react'; class DataFetcher extends React.Component { componentDidMount() { fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error(error)); } render() { return <div>Loading data...</div>; } } export default DataFetcher;
In the above example, the DataFetcher
component fetches data from an API using the componentDidMount
lifecycle method. The fetched data is then logged to the console.
Lifecycle methods can also be used to optimize performance by preventing unnecessary re-renders. The shouldComponentUpdate
method can be overridden to control whether the component should update or not based on changes in props or state.
It's important to note that with the introduction of React Hooks, the use of lifecycle methods has been reduced in favor of using hooks like useEffect
to handle side effects and lifecycle-like behavior in functional components.
React Router
React Router is a popular library for handling routing in React applications. It allows you to define routes and map them to specific components, enabling navigation between different views or pages in a single-page application.
To use React Router, you need to install it as a dependency in your project:
npm install react-router-dom
Once installed, you can define routes using the BrowserRouter
component:
import React from 'react'; import { BrowserRouter, Route, Link } from 'react-router-dom'; import Home from './Home'; import About from './About'; import Contact from './Contact'; function App() { return ( <BrowserRouter> <nav> <ul> <li> <Link to="/">Home</Link> </li> <li> <Link to="/about">About</Link> </li> <li> <Link to="/contact">Contact</Link> </li> </ul> </nav> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> <Route path="/contact" component={Contact} /> </BrowserRouter> ); } export default App;
In the above example, the BrowserRouter
component wraps the routes and provides the necessary routing functionality. The Link
component is used to create navigation links.
The Route
component is used to define the routes. The path
prop specifies the URL path, and the component
prop specifies the component to render when the path matches.
React Router also supports dynamic routing using URL parameters:
import React from 'react'; import { BrowserRouter, Route, Link } from 'react-router-dom'; import User from './User'; function App() { return ( <BrowserRouter> <nav> <ul> <li> <Link to="/user/1">User 1</Link> </li> <li> <Link to="/user/2">User 2</Link> </li> </ul> </nav> <Route path="/user/:id" component={User} /> </BrowserRouter> ); } export default App;
In this example, the User
component is rendered when the path matches "/user/:id", where ":id" is a URL parameter that can be accessed in the User
component.
React Router provides many more features for handling navigation, such as nested routes, redirects, and route guards. It is a useful tool for building complex routing in React applications.
React Redux
React Redux is a library that integrates Redux with React, providing a predictable state container for managing application state. Redux is a popular state management library that follows the principles of Flux architecture.
To use React Redux, you need to install it as a dependency in your project:
npm install react-redux
Once installed, you can use the Provider
component to wrap your root component and provide the Redux store:
import React from 'react'; import { Provider } from 'react-redux'; import { createStore } from 'redux'; import rootReducer from './reducers'; import App from './App'; const store = createStore(rootReducer); function Root() { return ( <Provider store={store}> <App /> </Provider> ); } export default Root;
In this example, the Redux store is created using the createStore
function and the root reducer. The Provider
component wraps the App
component and provides the store to all components in the application.
To connect components to the Redux store and access state or dispatch actions, you can use the connect
function from React Redux:
import React from 'react'; import { connect } from 'react-redux'; function Counter({ count, increment }) { return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> ); } const mapStateToProps = state => ({ count: state.count }); const mapDispatchToProps = dispatch => ({ increment: () => dispatch({ type: 'INCREMENT' }) }); export default connect(mapStateToProps, mapDispatchToProps)(Counter);
In this example, the Counter
component is connected to the Redux store using the connect
function. The mapStateToProps
function maps the state from the Redux store to component props, and the mapDispatchToProps
function maps dispatch actions to component props.
React Redux provides many more features for managing application state, such as selectors, middleware, and async actions. It is a useful tool for building scalable and maintainable React applications.
Related Article: How to Implement Hover State in ReactJS with Inline Styles
React Form Handling
In React, form handling follows the same principles as handling events. You can use event handlers to capture user input and update the component's state accordingly. React also provides some additional features to make form handling easier.
Here is an example of a simple form with form handling in React:
import React from 'react'; class LoginForm extends React.Component { constructor(props) { super(props); this.state = { username: '', password: '' }; } handleChange(event) { this.setState({ [event.target.name]: event.target.value }); } handleSubmit(event) { event.preventDefault(); console.log('Submit:', this.state); } render() { return ( <form onSubmit={this.handleSubmit}> <label> Username: <input type="text" name="username" value={this.state.username} onChange={this.handleChange} /> </label> <label> Password: <input type="password" name="password" value={this.state.password} onChange={this.handleChange} /> </label> <button type="submit">Submit</button> </form> ); } } export default LoginForm;
In the above example, the LoginForm
component has two input fields for username and password. The component's state is updated whenever the input values change using the handleChange
method.
The form's onSubmit
event handler is set to the handleSubmit
method, which is called when the form is submitted. The handleSubmit
method prevents the default form submission behavior and logs the form data to the console.
React also provides additional features for form validation, such as setting input field validation rules, displaying error messages, and disabling the submit button until the form is valid. These features can be implemented using conditional rendering and state management.
How to Pass Data Between React Components?
In React, data can be passed between components using props. Props are used to pass data from a parent component to a child component. They allow you to create reusable and composable components by enabling components to be self-contained and independent.
To pass data from a parent component to a child component, you simply declare the prop in the parent component and pass the value as a prop when rendering the child component.
Here is an example:
import React from 'react'; import Greeting from './Greeting'; function App() { const name = 'John'; return <Greeting name={name} />; } export default App;
In this example, the App
component passes the name
prop with the value "John" to the Greeting
component. The Greeting
component can then access this prop using the props
object.
import React from 'react'; function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } export default Greeting;
The Greeting
component receives the name
prop and uses it to render the greeting "Hello, John!".
Props can also be passed to functional components using the destructuring syntax:
import React from 'react'; function Greeting({ name }) { return <h1>Hello, {name}!</h1>; } export default Greeting;
In this example, the name
prop is destructured from the props
object directly in the function signature.
Props can be any JavaScript value, including strings, numbers, booleans, objects, and even functions. They can also be used to pass event handlers from parent components to child components.
It's important to note that props are read-only and cannot be directly modified by the child component. If the child component needs to update the data, it should be done by invoking a callback function passed as a prop from the parent component.
What is the Difference Between React State and Props?
In React, both state and props are used to manage data within a component. While they serve similar purposes, there are some key differences between the two.
State is used to manage component-specific data that can change over time. It is declared and initialized within the component and can be updated using the setState
method. Changes to state trigger a re-render of the component, updating the UI to reflect the new state.
Props, on the other hand, are used to pass data from a parent component to a child component. They are read-only and cannot be directly modified by the child component. Props enable components to be reusable and composable by allowing components to be self-contained and independent.
Here are some key differences between state and props:
- Initialization: State is initialized within the component using the this.state
object in class components or the useState
hook in functional components. Props are passed to the component when it is rendered and accessed using the props
object in class components or destructuring in functional components.
- Mutability: State is mutable and can be updated within the component using the setState
method. Props are read-only and cannot be directly modified by the child component. If the child component needs to update the data, it should be done by invoking a callback function passed as a prop from the parent component.
- Scope: State is specific to a single component instance and is not accessible by other components. It is encapsulated within the component and can only be accessed and modified by that component. Props, on the other hand, are passed down from a parent component and can be accessed by child components.
- Updates: Changes to state trigger a re-render of the component, updating the UI to reflect the new state. Props, on the other hand, can only be updated by the parent component. Changes to props in the parent component will trigger a re-render of the child component with the new props.
In general, state should be used to manage data that is internal to the component and can change over time. Props should be used to pass data from a parent component to a child component and should not be modified within the child component.
What are React Lifecycle Methods and When to Use Them?
React lifecycle methods are special methods that are automatically called at different stages of a component's lifecycle. They allow you to hook into these stages and perform certain actions, such as initializing state, fetching data, or cleaning up resources.
Class components have several lifecycle methods that can be overridden to control the component's behavior. The most commonly used lifecycle methods are:
- componentDidMount
: Called after the component is rendered for the first time. It is commonly used to fetch data from an API, initialize subscriptions, or set up timers.
- componentDidUpdate
: Called after the component's updates have been applied to the DOM. It is commonly used to perform side effects based on changes in props or state, such as updating the DOM, fetching additional data, or logging.
- componentWillUnmount
: Called before the component is removed from the DOM. It is commonly used to clean up resources, such as cancelling timers, unsubscribing from subscriptions, or removing event listeners.
Here is an example of using lifecycle methods in a class component:
import React from 'react'; class DataFetcher extends React.Component { constructor(props) { super(props); this.state = { data: null }; } componentDidMount() { fetch('https://api.example.com/data') .then(response => response.json()) .then(data => this.setState({ data })) .catch(error => console.error(error)); } componentDidUpdate(prevProps, prevState) { if (prevState.data !== this.state.data) { console.log('Data changed:', this.state.data); } } componentWillUnmount() { console.log('Component unmounted'); } render() { return <div>Loading data...</div>; } } export default DataFetcher;
In this example, the DataFetcher
component fetches data from an API using the componentDidMount
lifecycle method. The fetched data is stored in the component's state using the setState
method.
The componentDidUpdate
lifecycle method compares the previous state with the current state and logs a message to the console when the data changes.
The componentWillUnmount
lifecycle method logs a message to the console when the component is unmounted.
Lifecycle methods can also be used to optimize performance by preventing unnecessary re-renders. The shouldComponentUpdate
method can be overridden to control whether the component should update or not based on changes in props or state.
With the introduction of React Hooks, the use of lifecycle methods has been reduced in favor of using hooks like useEffect
to handle side effects and lifecycle-like behavior in functional components.
Related Article: How to Solve “_ is not defined” Errors in ReactJS
Additional Resources
- How to Fetch Data in React