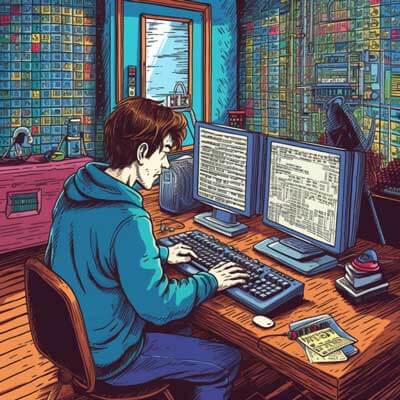
Table of Contents
Elasticsearch Query Optimization Techniques
When working with Elasticsearch, optimizing query performance is crucial for achieving efficient and fast search results. Here are some techniques to optimize Elasticsearch queries:
Related Article: How to Use Embedded JavaScript (EJS) in Node.js
1. Use Match Queries Instead of Term Queries
The term query in Elasticsearch is used to match exact terms in a field, while the match query analyzes the input text and performs a full-text search. In most cases, the match query provides better search results and is more flexible. Here's an example of using the match query:
GET /my_index/_search { "query": { "match": { "title": "elasticsearch" } } }
This query will return documents where the "title" field contains the term "elasticsearch".
2. Use Filtered Queries for Filtering
Filtering is a common operation in Elasticsearch where documents are filtered based on specific criteria. Instead of using the query context, which affects the relevance score, it is recommended to use the filter context. The filter context doesn't calculate relevance scores and is more efficient for filtering purposes. Here's an example of using a filtered query:
GET /my_index/_search { "query": { "filtered": { "filter": { "range": { "price": { "gte": 50, "lte": 100 } } } } } }
This query will return documents where the "price" field is between 50 and 100.
Elasticsearch Performance Monitoring Tools and Best Practices
Monitoring the performance of Elasticsearch is essential to ensure its optimal operation. Here are some tools and best practices for monitoring Elasticsearch performance:
Related Article: 16 Amazing Python Libraries You Can Use Now
1. Elasticsearch Monitoring Cluster API
Elasticsearch provides a Monitoring Cluster API that allows you to retrieve various performance metrics and statistics about your cluster. You can use this API to monitor the health of your cluster, track resource usage, and identify potential bottlenecks. Here's an example of using the Monitoring Cluster API:
GET /_cluster/stats
This API call will return detailed statistics about your Elasticsearch cluster.
2. Elasticsearch Monitoring Plugins
There are several monitoring plugins available for Elasticsearch that provide additional monitoring capabilities. One popular plugin is the Elastic Stack, which includes Elasticsearch, Logstash, and Kibana. The Elastic Stack allows you to collect, analyze, and visualize your Elasticsearch metrics in real-time. Here's an example of installing the Elastic Stack:
sudo apt-get update sudo apt-get install elasticsearch logstash kibana
After installing the Elastic Stack, you can configure it to collect and visualize Elasticsearch metrics.
Elasticsearch Query Profiling for Identifying Performance Bottlenecks
Profiling Elasticsearch queries can help identify performance bottlenecks and optimize query execution. Elasticsearch provides a Query Profiler API that allows you to profile individual queries and analyze their performance. Here's how you can use the Query Profiler API:
1. Enable Query Profiling
To enable query profiling, you need to set the "profile" parameter to "true" in your search request. Here's an example:
GET /my_index/_search { "profile": true, "query": { "match": { "title": "elasticsearch" } } }
This will return detailed profiling information for the query execution.
Related Article: How to Use the Regex Negation (Not) Operator
2. Analyze Query Profile
The profiling information returned by the Query Profiler API includes various metrics such as the time taken for each phase of query execution, the number of documents examined, and the memory used. By analyzing this information, you can identify potential performance bottlenecks and optimize your queries accordingly.
Elasticsearch Query Benchmarking Strategies
Benchmarking Elasticsearch queries is crucial for measuring their performance and identifying areas for improvement. Here are some strategies for benchmarking Elasticsearch queries:
1. Generate Realistic Test Data
To accurately benchmark Elasticsearch queries, it is important to generate realistic test data that resembles your production data. This will ensure that the benchmark results reflect the actual performance of your queries in a real-world scenario.
2. Use a Benchmarking Tool
There are several benchmarking tools available for Elasticsearch that can help you simulate heavy query workloads and measure their performance. One popular tool is Rally, which is an open-source benchmarking framework specifically designed for Elasticsearch. Rally allows you to define custom benchmark configurations, execute benchmark races, and analyze the results.
Related Article: How to Implement HTML Select Multiple As a Dropdown
Identifying Slow Queries in Elasticsearch
Identifying slow queries in Elasticsearch is crucial for optimizing query performance. Here are some techniques to identify slow queries:
1. Enable Slow Query Logging
Enabling slow query logging in Elasticsearch allows you to capture queries that exceed a certain threshold. By analyzing the slow query logs, you can identify queries that are taking longer to execute and optimize them accordingly. To enable slow query logging, you need to configure the "index.search.slowlog" setting in the Elasticsearch configuration file. Here's an example configuration:
index.search.slowlog.threshold.query.warn: 10s index.search.slowlog.threshold.query.info: 5s
This configuration will log queries that take longer than 5 seconds at the info level and queries that take longer than 10 seconds at the warn level.
2. Use the Search Profiler API
The Search Profiler API in Elasticsearch allows you to profile individual queries and analyze their execution time. By profiling your queries, you can identify slow queries and optimize their performance. Here's an example of using the Search Profiler API:
GET /my_index/_search { "profile": true, "query": { "match": { "title": "elasticsearch" } } }
This will return profiling information for the query execution, including the time taken for each phase of the execution.
Enabling Query Logging in Elasticsearch
Enabling query logging in Elasticsearch is essential for capturing and analyzing queries executed on your cluster. Here's how you can enable query logging:
Related Article: How to Use the aria-label Attribute in HTML
1. Configure the Logging Settings
To enable query logging, you need to configure the logging settings in the Elasticsearch configuration file. You can specify the log level, log format, and log file location. Here's an example configuration:
logger.index.search.slowlog: TRACE, index_search_slow_log_file logger.index.search.slowlog.appenderRef.index_search_slow_log_file.ref: index_search_slow_log_file appender.index_search_slow_log_file.type: dailyRollingFile appender.index_search_slow_log_file.file: /path/to/slowlog.log appender.index_search_slow_log_file.layout.type: pattern appender.index_search_slow_log_file.layout.pattern: "[%d{ISO8601}][%-5p][%-25c{1.}] %marker%m%n"
This configuration will log the slow queries to the "/path/to/slowlog.log" file.
2. Analyze the Query Logs
Once query logging is enabled, Elasticsearch will log the executed queries to the specified log file. By analyzing the query logs, you can gain insights into the query performance and identify areas for optimization.
Techniques for Analyzing Elasticsearch Queries
Analyzing Elasticsearch queries is crucial for understanding their performance and identifying areas for optimization. Here are some techniques for analyzing Elasticsearch queries:
1. Explain API
The Explain API in Elasticsearch allows you to analyze how a specific document matches a query. By providing a document ID and a query, the Explain API returns detailed information about the scoring and matching of the document. Here's an example of using the Explain API:
GET /my_index/_explain/1 { "query": { "match": { "title": "elasticsearch" } } }
This will return an explanation of how the document with ID 1 matches the query.
Related Article: How to Restrict HTML File Input to Only PDF and XLS
2. Kibana Dev Tools Console
Kibana's Dev Tools Console provides a useful interface for analyzing Elasticsearch queries. You can execute queries, view the results, and analyze the query performance. The Dev Tools Console also allows you to use various debugging features, such as formatting and highlighting query syntax.
Tips for Tuning Elasticsearch Queries
Tuning Elasticsearch queries is crucial for achieving optimal performance. Here are some tips for tuning Elasticsearch queries:
1. Use Query Caching
Query caching in Elasticsearch allows you to cache the results of frequently executed queries. By enabling query caching, you can improve query performance and reduce the load on your cluster. To enable query caching, you need to set the "request_cache" parameter to "true" in your search request. Here's an example:
GET /my_index/_search { "request_cache": true, "query": { "match": { "title": "elasticsearch" } } }
This will cache the results of the query and improve its performance for subsequent executions.
2. Use Field Data Loading
Field data loading in Elasticsearch allows you to load field data into memory for faster query execution. By loading frequently accessed fields into memory, you can significantly improve query performance. To enable field data loading, you need to set the "fielddata" parameter to "true" in your search request. Here's an example:
GET /my_index/_search { "fielddata": true, "query": { "match": { "title": "elasticsearch" } } }
This will load the "title" field data into memory and improve the query performance.
Related Article: How to Use Abstraction in Object Oriented Programming (OOP)
Measuring Execution Time of Elasticsearch Queries
Measuring the execution time of Elasticsearch queries is crucial for understanding their performance and identifying areas for optimization. Here are some techniques for measuring the execution time of Elasticsearch queries:
1. Use the Profile API
The Profile API in Elasticsearch allows you to profile the execution time of individual queries and analyze their performance. By profiling your queries, you can identify potential performance bottlenecks and optimize their execution. Here's an example of using the Profile API:
GET /my_index/_search { "profile": true, "query": { "match": { "title": "elasticsearch" } } }
This will return profiling information for the query execution, including the time taken for each phase of the execution.
2. Use the Explain API
The Explain API in Elasticsearch allows you to analyze how a specific document matches a query. By providing a document ID and a query, the Explain API returns detailed information about the scoring and matching of the document. By analyzing the explain output, you can gain insights into the execution time of the query. Here's an example of using the Explain API:
GET /my_index/_explain/1 { "query": { "match": { "title": "elasticsearch" } } }
This will return an explanation of how the document with ID 1 matches the query, including the execution time.
Understanding the Impact of Query Latency on Elasticsearch Performance
Query latency, or the time taken for a query to execute, has a significant impact on Elasticsearch performance. Here's how query latency can affect Elasticsearch performance:
Related Article: How to Ignore Case Sensitivity with Regex (Case Insensitive)
1. Increased Response Times
Queries with high latency can significantly increase the response times of your Elasticsearch cluster. When a query takes longer to execute, it increases the waiting time for other queries, resulting in slower response times for all queries.
2. Resource Utilization
Queries with high latency consume more resources, such as CPU and memory, during their execution. This increased resource utilization can impact the overall performance of your Elasticsearch cluster and lead to resource contention.
3. Scalability
High query latency can limit the scalability of your Elasticsearch cluster. As the number of queries increases, the latency of individual queries can increase, leading to reduced throughput and overall cluster performance.
Additional Resources
- Monitoring Performance Metrics of Elasticsearch Queries