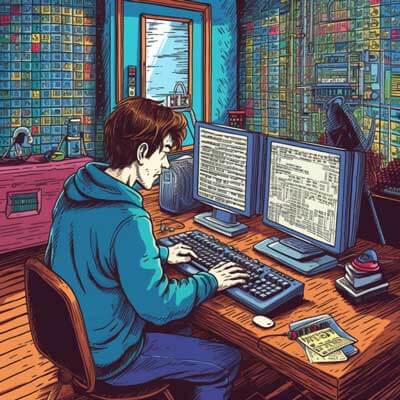
Table of Contents
React Router and Component Interaction
ReactJS is a popular JavaScript library for building user interfaces, and it provides a useful feature called React Router that allows us to create routes and navigate between different components in our application. Component interaction plays a vital role in building complex React applications, and understanding how to navigate between components is essential.
React Router is a third-party library that provides a declarative way to handle routing in React applications. It allows us to define routes for different components and render them based on the current URL. By using React Router, we can create a single-page application (SPA) with multiple views, each represented by a different component.
To use React Router in our application, we need to install it first. We can do this by running the following command:
npm install react-router-dom
Once React Router is installed, we can import it into our application and start creating routes. In our main component, we can import the necessary components from React Router, such as BrowserRouter, Route, and Link.
import { BrowserRouter, Route, Link } from 'react-router-dom';
The BrowserRouter component is a wrapper that provides the routing functionality to our application. We can wrap our entire application with BrowserRouter to enable routing.
ReactDOM.render( <BrowserRouter> <App /> </BrowserRouter>, document.getElementById('root') );
Inside the App component, we can define the routes using the Route component. The Route component takes two props: path and component. The path prop specifies the URL path for the component, and the component prop specifies the component to be rendered when the URL matches the path.
const App = () => { return ( <div> <nav> <ul> <li> <Link to="/">Home</Link> </li> <li> <Link to="/about">About</Link> </li> <li> <Link to="/contact">Contact</Link> </li> </ul> </nav> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> <Route path="/contact" component={Contact} /> </div> ); };
In the above example, we have defined three routes: one for the Home component, one for the About component, and one for the Contact component. The exact prop is used for the home route to match the exact URL path.
The Link component is used to create links between different components. It generates an anchor tag with the specified URL path. When clicked, it updates the URL in the browser and renders the corresponding component.
<Link to="/about">About</Link>
This code snippet generates a link to the About component with the path "/about". When clicked, it updates the URL to "/about" and renders the About component.
React Router provides a flexible and useful way to handle component interaction in React applications. By using the BrowserRouter, Route, and Link components, we can define routes and navigate between different components with ease.
Related Article: Handling Routing in React Apps with React Router
Handling onClick Events in React
Handling onClick events in React is a fundamental part of building interactive user interfaces. In React, we can attach event handlers to elements using the onClick prop. When the element is clicked, the event handler function is called.
const handleClick = () => { console.log('Button clicked!'); }; const Button = () => { return <button onClick={handleClick}>Click me</button>; };
In the above example, we have defined a handleClick function that logs a message to the console when the button is clicked. We then attach this function to the onClick prop of the button element.
We can also pass arguments to the event handler function by using an arrow function.
const handleClick = (name) => { console.log(`Hello, ${name}!`); }; const Button = () => { return ( <button onClick={() => handleClick('John')}> Click me </button> ); };
In this example, we pass the name 'John' to the handleClick function when the button is clicked. This allows us to customize the behavior of the event handler based on the specific context.
It's important to note that when using event handlers in React, we should avoid calling the function directly, as it will be called immediately when the component renders. Instead, we should pass a reference to the function by omitting the parentheses.
const handleClick = () => { console.log('Button clicked!'); }; const Button = () => { return <button onClick={handleClick}>Click me</button>; };
In this example, we pass the handleClick function without calling it immediately. React will automatically call the function when the button is clicked.
Handling onClick events in React is a simple and effective way to add interactivity to our components. By attaching event handlers to elements, we can respond to user actions and update the state of our application accordingly.
Rendering Components in React
In React, rendering components is a core concept that allows us to display UI elements on the screen. Components are reusable and self-contained pieces of code that encapsulate a specific functionality or visual representation.
To render a component in React, we need to define a function or a class that returns JSX (JavaScript XML) code. JSX is a syntax extension for JavaScript that allows us to write HTML-like code within our JavaScript files.
Here's an example of a functional component that renders a simple button:
import React from 'react'; const Button = () => { return <button>Click me</button>; }; export default Button;
In this example, we define a functional component called Button that returns a button element with the text "Click me". We export the component using the export default syntax so that it can be imported and used in other parts of our application.
To use the Button component, we can import it into another component and include it in the JSX code:
import React from 'react'; import Button from './Button'; const App = () => { return ( <div> <h1>Welcome to my app</h1> <Button /> </div> ); }; export default App;
In this example, we import the Button component and include it within the JSX code of the App component. When the App component is rendered, the Button component is also rendered and displayed on the screen.
In addition to functional components, we can also render class components in React. Class components are defined as ES6 classes that extend the React.Component class and implement a render method.
Here's an example of a class component that renders a simple message:
import React from 'react'; class Message extends React.Component { render() { return <p>Hello, world!</p>; } } export default Message;
In this example, we define a class component called Message that extends the React.Component class. The render method returns a paragraph element with the text "Hello, world!". We export the component so that it can be imported and used in other parts of our application.
To use the Message component, we can import it into another component and include it in the JSX code:
import React from 'react'; import Message from './Message'; const App = () => { return ( <div> <h1>Welcome to my app</h1> <Message /> </div> ); }; export default App;
In this example, we import the Message component and include it within the JSX code of the App component. When the App component is rendered, the Message component is also rendered and displayed on the screen.
Rendering components in React is a fundamental concept that allows us to build complex user interfaces. By defining and using components, we can create reusable and modular code that makes our applications easier to develop and maintain.
Managing State in React
In React, state is an important concept that allows us to store and manage data within a component. State represents the mutable values that are specific to a component and can change over time. By managing state, we can control the behavior and appearance of our components.
To use state in a functional component, we can use the useState hook provided by React. The useState hook returns a pair: the current state value and a function to update the state.
Here's an example of a functional component that uses state to manage a counter:
import React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; const decrement = () => { setCount(count - 1); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> <button onClick={decrement}>Decrement</button> </div> ); }; export default Counter;
In this example, we import the useState hook from React and use it to define a state variable called count. We initialize count with a value of 0. We also define two functions, increment and decrement, that update the count state using the setCount function.
Inside the JSX code, we display the current value of count using curly braces: {count}. We also attach the increment and decrement functions to the onClick event of the buttons.
When the buttons are clicked, the increment and decrement functions are called, which update the count state and trigger a re-render of the component.
In addition to functional components, we can also manage state in class components using the this.state object and the this.setState method.
Here's an example of a class component that manages a counter:
import React from 'react'; class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } increment() { this.setState({ count: this.state.count + 1 }); } decrement() { this.setState({ count: this.state.count - 1 }); } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={this.increment.bind(this)}>Increment</button> <button onClick={this.decrement.bind(this)}>Decrement</button> </div> ); } } export default Counter;
In this example, we define a class component called Counter that extends the React.Component class. We initialize the count state in the constructor and define the increment and decrement methods that update the count state using this.setState.
Inside the render method, we display the current value of count using this.state.count. We also bind the increment and decrement methods to the onClick event of the buttons.
When the buttons are clicked, the increment and decrement methods are called, which update the count state and trigger a re-render of the component.
Managing state in React is a useful feature that allows us to build dynamic and interactive user interfaces. By using the useState hook in functional components or the this.state object in class components, we can store and update data within our components.
Related Article: Executing Multiple Fetch Calls Simultaneously in ReactJS
Working with Props in React
In React, props are a way to pass data from a parent component to a child component. Props are read-only and cannot be modified by the child component. By using props, we can create reusable and configurable components.
To pass props to a child component, we can include them as attributes in the JSX code. The child component can then access the props using the props object.
Here's an example of a parent component that passes a name prop to a child component:
import React from 'react'; import ChildComponent from './ChildComponent'; const ParentComponent = () => { return <ChildComponent name="John" />; }; export default ParentComponent;
In this example, we import the ChildComponent and include it within the JSX code of the ParentComponent. We pass a name prop with the value "John" to the ChildComponent.
The ChildComponent can access the name prop using the props object:
import React from 'react'; const ChildComponent = (props) => { return <p>Hello, {props.name}</p>; }; export default ChildComponent;
In this example, we define the ChildComponent as a functional component that takes the props object as an argument. Inside the JSX code, we display the value of the name prop using {props.name}.
When the ParentComponent is rendered, the ChildComponent is also rendered with the name prop value "John". The ChildComponent displays the message "Hello, John".
In addition to passing simple values as props, we can also pass functions as props. This allows the child component to communicate with the parent component and trigger actions.
Here's an example of a parent component that passes a handleClick function as a prop to a child component:
import React from 'react'; import ChildComponent from './ChildComponent'; const ParentComponent = () => { const handleClick = () => { console.log('Button clicked!'); }; return <ChildComponent onClick={handleClick} />; }; export default ParentComponent;
In this example, we define a handleClick function in the ParentComponent. We pass this function as a prop called onClick to the ChildComponent.
The ChildComponent can access the onClick prop and call it as a function:
import React from 'react'; const ChildComponent = (props) => { return <button onClick={props.onClick}>Click me</button>; }; export default ChildComponent;
In this example, we define the ChildComponent as a functional component. Inside the JSX code, we attach the onClick prop to the button element.
When the button is clicked, the onClick prop is called as a function. In this case, it logs the message "Button clicked!" to the console.
Working with props in React allows us to create flexible and reusable components. By passing data and functions as props, we can customize the behavior and appearance of our components and create interactive user interfaces.
Conditionally Rendering Components in React
In React, we can conditionally render components based on certain conditions or values. This allows us to show or hide components dynamically and create more dynamic user interfaces.
To conditionally render components in React, we can use conditional statements such as if-else or the ternary operator within the JSX code.
Here's an example of a component that conditionally renders a message based on a boolean value:
import React from 'react'; const ConditionalComponent = ({ isLoggedIn }) => { if (isLoggedIn) { return <p>Welcome, user!</p>; } else { return <p>Please log in.</p>; } }; export default ConditionalComponent;
In this example, we define a functional component called ConditionalComponent that takes a prop called isLoggedIn. Inside the component, we use an if-else statement to conditionally render different messages based on the value of isLoggedIn.
When isLoggedIn is true, the component renders the message "Welcome, user!". When isLoggedIn is false, the component renders the message "Please log in.".
We can use the ConditionalComponent in another component and pass the isLoggedIn prop:
import React from 'react'; import ConditionalComponent from './ConditionalComponent'; const App = () => { const isLoggedIn = true; return ( <div> <h1>Welcome to my app</h1> <ConditionalComponent isLoggedIn={isLoggedIn} /> </div> ); }; export default App;
In this example, we import the ConditionalComponent and include it within the JSX code of the App component. We define a variable called isLoggedIn and pass it as the isLoggedIn prop to the ConditionalComponent.
When the App component is rendered, the ConditionalComponent is also rendered. Based on the value of isLoggedIn, the ConditionalComponent conditionally renders the appropriate message.
In addition to if-else statements, we can also use the ternary operator to conditionally render components. The ternary operator is a shorthand way of writing if-else statements in a single line.
Here's an example of a component that conditionally renders a message using the ternary operator:
import React from 'react'; const ConditionalComponent = ({ isLoggedIn }) => { return isLoggedIn ? <p>Welcome, user!</p> : <p>Please log in.</p>; }; export default ConditionalComponent;
In this example, we use the ternary operator to conditionally render different messages based on the value of isLoggedIn. If isLoggedIn is true, the component renders the message "Welcome, user!". If isLoggedIn is false, the component renders the message "Please log in.".
Conditionally rendering components in React allows us to create dynamic and interactive user interfaces. By using conditional statements or the ternary operator, we can show or hide components based on certain conditions or values.
Configuring Routes in React
In React, configuring routes allows us to define the different paths and components in our application. By using a routing library like React Router, we can create a single-page application with multiple views and navigate between them.
To configure routes in React, we need to set up a router that handles the routing functionality. React Router is a popular library that provides a declarative way to handle routing in React applications.
First, we need to install React Router in our project. We can do this by running the following command:
npm install react-router-dom
Once React Router is installed, we can import the necessary components from the react-router-dom package.
import { BrowserRouter, Route } from 'react-router-dom';
The BrowserRouter component is a wrapper that provides the routing functionality to our application. We can wrap our entire application with BrowserRouter to enable routing.
Next, we can define the routes using the Route component. The Route component takes two props: path and component. The path prop specifies the URL path for the component, and the component prop specifies the component to be rendered when the URL matches the path.
Here's an example of configuring routes in a React application:
import React from 'react'; import { BrowserRouter, Route } from 'react-router-dom'; import Home from './components/Home'; import About from './components/About'; import Contact from './components/Contact'; const App = () => { return ( <BrowserRouter> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> <Route path="/contact" component={Contact} /> </BrowserRouter> ); }; export default App;
In this example, we import the BrowserRouter and Route components from the react-router-dom package. We also import three components: Home, About, and Contact.
Inside the App component, we wrap the Route components with BrowserRouter. We define three routes: one for the Home component, one for the About component, and one for the Contact component. The exact prop is used for the home route to match the exact URL path.
When the URL matches a defined path, the corresponding component is rendered. For example, when the URL is "/about", the About component is rendered.
Configuring routes in React allows us to create a single-page application with multiple views. By using the BrowserRouter and Route components provided by React Router, we can define the routes and components in our application and handle navigation between them.
Understanding the Link Component in React Router
In React Router, the Link component is used to create links between different components. It generates an anchor tag with the specified URL path. When clicked, it updates the URL in the browser and renders the corresponding component.
To use the Link component in React Router, we need to import it from the react-router-dom package.
import { Link } from 'react-router-dom';
The Link component takes a prop called to, which specifies the URL path for the link.
Here's an example of using the Link component to create links between components:
import React from 'react'; import { Link } from 'react-router-dom'; const Navigation = () => { return ( <nav> <ul> <li> <Link to="/">Home</Link> </li> <li> <Link to="/about">About</Link> </li> <li> <Link to="/contact">Contact</Link> </li> </ul> </nav> ); }; export default Navigation;
In this example, we import the Link component from the react-router-dom package. Inside the Navigation component, we use the Link component to create links between different components.
The to prop of the Link component specifies the URL path for the link. For example, the first Link component has a to prop value of "/", which represents the home route.
When the links are clicked, the URL in the browser is updated to the specified path, and the corresponding component is rendered.
The Link component in React Router provides a declarative way to create links between components. By using the to prop, we can specify the URL path for the link and handle navigation in our application.
Related Article: Accessing Array Length in this.state in ReactJS
Creating Nested Routes in React
In React Router, nested routes allow us to create routes that are children of other routes. This allows us to build complex navigational structures and render different components based on the nested URL paths.
To create nested routes in React Router, we can define routes inside other routes. We can nest Route components within each other to create a hierarchical structure.
Here's an example of creating nested routes in React Router:
import React from 'react'; import { BrowserRouter, Route } from 'react-router-dom'; import Home from './components/Home'; import About from './components/About'; import Contact from './components/Contact'; import Profile from './components/Profile'; import Dashboard from './components/Dashboard'; const App = () => { return ( <BrowserRouter> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> <Route path="/contact" component={Contact} /> <Route path="/profile" component={Profile} /> <Route path="/dashboard" component={Dashboard} /> </BrowserRouter> ); }; export default App;
In this example, we define five routes: one for the Home component, one for the About component, one for the Contact component, one for the Profile component, and one for the Dashboard component.
The Profile and Dashboard components are nested routes because they are defined inside other routes. When the URL matches "/profile", the Profile component is rendered. When the URL matches "/dashboard", the Dashboard component is rendered.
Nested routes allow us to create more complex navigational structures in our React applications. By nesting Route components within each other, we can define routes that are children of other routes and render different components based on the nested URL paths.
Programmatic Navigation in React
In React, programmatic navigation allows us to navigate between different components programmatically, without relying on user interaction. This is useful when we want to redirect the user to a different page or component based on certain conditions or actions.
React Router provides a history object that we can use to programmatically navigate to different locations. The history object allows us to push, replace, or go back to a specific URL.
To access the history object in React Router, we can use the useHistory hook provided by the react-router-dom package.
Here's an example of using programmatic navigation in React Router:
import React from 'react'; import { useHistory } from 'react-router-dom'; const LoginForm = () => { const history = useHistory(); const handleSubmit = () => { // Perform login logic // Redirect to dashboard history.push('/dashboard'); }; return ( <form onSubmit={handleSubmit}> {/* Login form fields */} <button type="submit">Login</button> </form> ); }; export default LoginForm;
In this example, we import the useHistory hook from the react-router-dom package. Inside the LoginForm component, we use the useHistory hook to access the history object.
When the login form is submitted, the handleSubmit function is called. After performing the login logic, we can use the history.push method to navigate to a specific URL. In this case, we redirect the user to the dashboard component.
Programmatic navigation in React allows us to dynamically redirect users to different components based on certain conditions or actions. By using the useHistory hook provided by React Router, we can access the history object and use its methods to navigate programmatically.
Navigating to Another Component using React Router
In React Router, we can navigate to another component by updating the URL in the browser. This triggers the rendering of the corresponding component based on the defined routes.
To navigate to another component using React Router, we can use the Link component provided by the react-router-dom package. The Link component generates an anchor tag with the specified URL path. When clicked, it updates the URL in the browser and renders the corresponding component.
Here's an example of navigating to another component using React Router:
import React from 'react'; import { Link } from 'react-router-dom'; const Navigation = () => { return ( <nav> <ul> <li> <Link to="/about">About</Link> </li> <li> <Link to="/contact">Contact</Link> </li> </ul> </nav> ); }; export default Navigation;
In this example, we import the Link component from the react-router-dom package. Inside the Navigation component, we use the Link component to create links to the About and Contact components.
The to prop of the Link component specifies the URL path for the link. When the links are clicked, the URL in the browser is updated to the specified path, and the corresponding component is rendered.
Navigating to another component using React Router allows us to create a single-page application with multiple views. By using the Link component, we can define links between components and handle navigation in our application.
Handling onClick Event to Open Another Component in React
In React, we can handle the onClick event to open another component by updating the state of our application. By managing the state and conditionally rendering components, we can show or hide the desired component based on the user's interaction.
Here's an example of handling the onClick event to open another component in React:
import React, { useState } from 'react'; import ComponentToOpen from './ComponentToOpen'; const App = () => { const [isOpen, setIsOpen] = useState(false); const handleClick = () => { setIsOpen(true); }; return ( <div> <button onClick={handleClick}>Open Component</button> {isOpen && <ComponentToOpen />} </div> ); }; export default App;
In this example, we import the ComponentToOpen component and include it as a child component within the JSX code of the App component.
We define a state variable called isOpen and a function called setIsOpen using the useState hook. The initial value of isOpen is set to false.
When the button is clicked, the handleClick function is called, and it updates the isOpen state to true.
Inside the JSX code, we use the isOpen state to conditionally render the ComponentToOpen component. The ComponentToOpen component is only rendered when isOpen is true.
Related Article: Sharing Variables Between Components in ReactJS
The Process of Rendering a Component in React
The process of rendering a component in React involves several steps that React performs automatically. Understanding this process is important for building efficient and performant React applications.
When a component is rendered in React, the following steps are executed:
1. React creates a virtual representation of the component called a virtual DOM (VDOM). The virtual DOM is a lightweight copy of the actual DOM that React uses to perform efficient updates.
2. React compares the virtual DOM with the previous virtual DOM to determine the differences between them. This process is called reconciliation or diffing.
3. React updates the actual DOM based on the differences identified during the reconciliation process. Only the necessary changes are applied to the DOM, minimizing the amount of work required.
4. React calls the render method of the component to generate the JSX code for the component. The render method returns a React element, which is a lightweight description of what should be rendered on the screen.
5. React uses the JSX code to update the virtual DOM and perform the reconciliation process again if necessary. This allows React to efficiently handle updates and keep the actual DOM in sync with the virtual DOM.
6. React updates the actual DOM based on the changes identified during the reconciliation process. The updated components are displayed on the screen, and any necessary re-rendering is performed.
The process of rendering a component in React is optimized for efficiency and performance. By using the virtual DOM and performing a diffing process, React minimizes the amount of work required to update the actual DOM and keeps the application running smoothly.
Managing State in React to Open Another Component
In React, managing state is an important concept that allows us to store and update data within a component. By managing state, we can control the behavior and appearance of our components, including opening another component based on certain conditions or user interactions.
To manage state in React, we can use the useState hook provided by the React library. The useState hook allows us to define state variables and functions to update the state.
Here's an example of managing state in React to open another component:
import React, { useState } from 'react'; import ComponentToOpen from './ComponentToOpen'; const App = () => { const [isOpen, setIsOpen] = useState(false); const handleClick = () => { setIsOpen(true); }; return ( <div> <button onClick={handleClick}>Open Component</button> {isOpen && <ComponentToOpen />} </div> ); }; export default App;
In this example, we import the ComponentToOpen component and include it as a child component within the JSX code of the App component.
We define a state variable called isOpen and a function called setIsOpen using the useState hook. The initial value of isOpen is set to false.
When the button is clicked, the handleClick function is called, and it updates the isOpen state to true.
Inside the JSX code, we use the isOpen state to conditionally render the ComponentToOpen component. The ComponentToOpen component is only rendered when isOpen is true.
Understanding Props in React and Opening Another Component
In React, props are used to pass data from a parent component to a child component. Props are read-only and cannot be modified by the child component. By using props, we can customize the behavior and appearance of our components, including opening another component based on the passed props.
Here's an example of understanding props in React and opening another component:
import React from 'react'; import ComponentToOpen from './ComponentToOpen'; const ParentComponent = () => { const isOpen = true; return ( <div> <ComponentToOpen isOpen={isOpen} /> </div> ); }; export default ParentComponent;
In this example, we import the ComponentToOpen component and include it as a child component within the JSX code of the ParentComponent.
We define a variable called isOpen and pass it as a prop called isOpen to the ComponentToOpen component.
Inside the ComponentToOpen component, we can access the isOpen prop and use it to conditionally render the desired component:
import React from 'react'; import AnotherComponent from './AnotherComponent'; const ComponentToOpen = ({ isOpen }) => { return ( <div> {isOpen && <AnotherComponent />} </div> ); }; export default ComponentToOpen;
In this example, we import the AnotherComponent and include it as a child component within the JSX code of the ComponentToOpen component.
We define a functional component called ComponentToOpen that takes the isOpen prop as an argument. Inside the JSX code, we use the isOpen prop to conditionally render the AnotherComponent component. The AnotherComponent component is only rendered when isOpen is true.
Conditionally Rendering a Component in React
In React, conditionally rendering a component allows us to show or hide a component based on certain conditions or values. This allows us to create more dynamic and interactive user interfaces.
To conditionally render a component in React, we can use conditional statements or the ternary operator within the JSX code.
Here's an example of conditionally rendering a component in React using an if-else statement:
import React from 'react'; const ConditionalComponent = ({ isLoggedIn }) => { if (isLoggedIn) { return <p>Welcome, user!</p>; } else { return <p>Please log in.</p>; } }; export default ConditionalComponent;
In this example, we define a functional component called ConditionalComponent that takes a prop called isLoggedIn. Inside the component, we use an if-else statement to conditionally render different messages based on the value of isLoggedIn.
When isLoggedIn is true, the component renders the message "Welcome, user!". When isLoggedIn is false, the component renders the message "Please log in.".
We can use the ConditionalComponent in another component and pass the isLoggedIn prop:
import React from 'react'; import ConditionalComponent from './ConditionalComponent'; const App = () => { const isLoggedIn = true; return ( <div> <h1>Welcome to my app</h1> <ConditionalComponent isLoggedIn={isLoggedIn} /> </div> ); }; export default App;
In this example, we import the ConditionalComponent and include it within the JSX code of the App component. We define a variable called isLoggedIn and pass it as the isLoggedIn prop to the ConditionalComponent.
When the App component is rendered, the ConditionalComponent is also rendered. Based on the value of isLoggedIn, the ConditionalComponent conditionally renders the appropriate message.
Conditionally rendering a component in React allows us to show or hide components based on certain conditions or values. By using conditional statements or the ternary operator, we can create more dynamic and interactive user interfaces.
Related Article: ReactJS: How to Re-Render Post Localstorage Clearing
Configuring Routes in React to Open Another Component
In React, configuring routes allows us to define the different paths and components in our application. By using a routing library like React Router, we can create a single-page application with multiple views and navigate between them. Configuring routes in React allows us to open another component based on the defined routes and the URL path.
To configure routes in React, we need to set up a router that handles the routing functionality. React Router is a popular library that provides a declarative way to handle routing in React applications.
First, we need to install React Router in our project. We can do this by running the following command:
npm install react-router-dom
Once React Router is installed, we can import the necessary components from the react-router-dom package.
import { BrowserRouter, Route } from 'react-router-dom';
The BrowserRouter component is a wrapper that provides the routing functionality to our application. We can wrap our entire application with BrowserRouter to enable routing.
Next, we can define the routes using the Route component. The Route component takes two props: path and component. The path prop specifies the URL path for the component, and the component prop specifies the component to be rendered when the URL matches the path.
Here's an example of configuring routes in a React application to open another component:
import React from 'react'; import { BrowserRouter, Route } from 'react-router-dom'; import Home from './components/Home'; import About from './components/About'; import Contact from './components/Contact'; import AnotherComponent from './components/AnotherComponent'; const App = () => { return ( <BrowserRouter> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> <Route path="/contact" component={Contact} /> <Route path="/another" component={AnotherComponent} /> </BrowserRouter> ); }; export default App;
In this example, we import the BrowserRouter and Route components from the react-router-dom package. We also import three components: Home, About, and Contact.
Inside the App component, we wrap the Route components with BrowserRouter. We define three routes: one for the Home component, one for the About component, one for the Contact component, and one for the AnotherComponent component.
When the URL matches a defined path, the corresponding component is rendered. For example, when the URL is "/about", the About component is rendered. When the URL is "/another", the AnotherComponent component is rendered.
Configuring routes in React allows us to create a single-page application with multiple views. By using the BrowserRouter and Route components provided by React Router, we can define the routes and components in our application and handle navigation between them.
The Purpose of the Link Component in React Router
In React Router, the Link component is used to create links between different components. It generates an anchor tag with the specified URL path. When clicked, it updates the URL in the browser and renders the corresponding component.
The purpose of the Link component in React Router is to provide a declarative way to handle client-side navigation in a React application. Instead of manually updating the URL and reloading the page, we can use the Link component to navigate between different components.
To use the Link component in React Router, we need to import it from the react-router-dom package.
import { Link } from 'react-router-dom';
The Link component takes a prop called to, which specifies the URL path for the link.
Here's an example of using the Link component to create links between components:
import React from 'react'; import { Link } from 'react-router-dom'; const Navigation = () => { return ( <nav> <ul> <li> <Link to="/about">About</Link> </li> <li> <Link to="/contact">Contact</Link> </li> </ul> </nav> ); }; export default Navigation;
In this example, we import the Link component from the react-router-dom package. Inside the Navigation component, we use the Link component to create links to the About and Contact components.
The to prop of the Link component specifies the URL path for the link. When the links are clicked, the URL in the browser is updated to the specified path, and the corresponding component is rendered.
The Link component in React Router provides a declarative way to create links between components. By using the to prop, we can specify the URL path for the link and handle navigation in our application.
Creating Nested Routes in React to Open Another Component
In React, nested routes allow us to create routes that are children of other routes. This allows us to build complex navigational structures and render different components based on the nested URL paths. By configuring nested routes in React, we can open another component based on the defined routes and the URL path.
To create nested routes in React, we can define routes inside other routes. We can nest Route components within each other to create a hierarchical structure.
Here's an example of creating nested routes in React to open another component:
import React from 'react'; import { BrowserRouter, Route } from 'react-router-dom'; import Home from './components/Home'; import About from './components/About'; import Contact from './components/Contact'; import Profile from './components/Profile'; import Dashboard from './components/Dashboard'; import AnotherComponent from './components/AnotherComponent'; const App = () => { return ( <BrowserRouter> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> <Route path="/contact" component={Contact} /> <Route path="/profile" component={Profile} /> <Route path="/dashboard" component={Dashboard}> <Route path="/dashboard/another" component={AnotherComponent} /> </Route> </BrowserRouter> ); }; export default App;
In this example, we import the BrowserRouter and Route components from the react-router-dom package. We also import five components: Home, About, Contact, Profile, and Dashboard.
Inside the App component, we wrap the Route components with BrowserRouter. We define five routes: one for the Home component, one for the About component, one for the Contact component, one for the Profile component, and one for the Dashboard component.
The Profile and Dashboard components are nested routes because they are defined inside other routes. When the URL matches "/profile", the Profile component is rendered. When the URL matches "/dashboard", the Dashboard component is rendered. Additionally, when the URL matches "/dashboard/another", the AnotherComponent component is rendered.
Programmatic Navigation to Another Component in React
In React, programmatic navigation allows us to navigate to another component programmatically, without relying on user interaction. This is useful when we want to redirect the user to a different page or component based on certain conditions or actions. By handling programmatic navigation in React, we can open another component based on certain conditions or actions.
React Router provides a history object that we can use to perform programmatic navigation. The history object allows us to push, replace, or go back to a specific URL.
To access the history object in React Router, we can use the useHistory hook provided by the react-router-dom package.
Here's an example of programmatic navigation to another component in React:
import React from 'react'; import { useHistory } from 'react-router-dom'; const LoginForm = () => { const history = useHistory(); const handleSubmit = () => { // Perform login logic // Redirect to dashboard history.push('/dashboard'); }; return ( <form onSubmit={handleSubmit}> {/* Login form fields */} <button type="submit">Login</button> </form> ); }; export default LoginForm;
In this example, we import the useHistory hook from the react-router-dom package. Inside the LoginForm component, we use the useHistory hook to access the history object.
When the login form is submitted, the handleSubmit function is called. After performing the login logic, we can use the history.push method to navigate to a specific URL. In this case, we redirect the user to the dashboard component.
Programmatic navigation in React allows us to dynamically redirect users to different components based on certain conditions or actions. By using the useHistory hook provided by React Router, we can access the history object and use its methods to navigate programmatically.
Related Article: Handling State Persistence in ReactJS After Refresh
Additional Resources
- ReactJS Props vs State