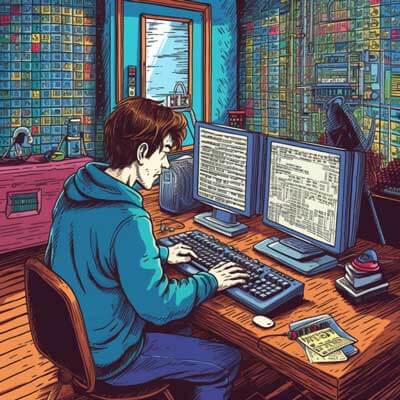
Table of Contents
Overview of Inequality
Inequality refers to the comparison between two values to determine if they are different or not. Python provides several comparison operators to perform inequality checks, including the "not equal" operator. This operator allows us to check if two values are not equal to each other.
Related Article: How to Append to Strings in Python
Comparison Operator: Not Equal
The "not equal" operator in Python is denoted by the symbol "!=". It is used to compare two values and returns True if they are not equal, and False otherwise. This operator can be used with various data types, such as numbers, strings, booleans, and even objects.
Let's take a look at some examples to understand how the "not equal" operator works.
# Numeric values x = 5 y = 10 print(x != y) # Output: True # String values name1 = "John" name2 = "Jane" print(name1 != name2) # Output: True # Boolean values is_true = True is_false = False print(is_true != is_false) # Output: True
In the above examples, we are comparing numeric values, string values, and boolean values using the "not equal" operator. The operator returns True when the values are not equal and False when they are equal.
Using Not Equal Operator in Conditional Statements
The "not equal" operator is commonly used in conditional statements to make decisions based on the inequality of values. Conditional statements allow us to execute different blocks of code based on certain conditions.
Here's an example that demonstrates the usage of the "not equal" operator in a conditional statement:
age = 18 if age != 18: print("You are not eligible to vote.") else: print("You are eligible to vote.")
In this example, we are checking if the value of the variable "age" is not equal to 18. If the condition is True, it means the person is not eligible to vote, and the corresponding message is printed. Otherwise, if the condition is False, it means the person is eligible to vote, and a different message is printed.
Boolean Expressions and Not Equal Operator
Boolean expressions are expressions that evaluate to either True or False. They are commonly used in conditional statements, loops, and other control structures. The "not equal" operator can be used in boolean expressions to compare two values and determine their inequality.
Let's consider a simple example where we compare the ages of two people using the "not equal" operator:
age1 = 25 age2 = 30 is_unequal = age1 != age2 print(is_unequal) # Output: True
In this example, we compare the ages of two people and assign the result of the comparison to the variable "is_unequal". The "not equal" operator evaluates to True because age1 and age2 are not equal. We then print the value of "is_unequal", which outputs True.
Related Article: How to Install Specific Package Versions With Pip in Python
Syntax for Not Equal Operator
The syntax for the "not equal" operator in Python is straightforward. It uses the "!=" symbol to compare two values.
value1 != value2
In the above syntax, "value1" and "value2" can be any valid Python values, including variables, literals, and expressions. The "not equal" operator compares these values and returns True if they are not equal, and False otherwise.
Code Snippet: Checking for Inequality
Let's take a look at a code snippet that demonstrates the usage of the "not equal" operator to check for inequality:
x = 10 y = 5 if x != y: print("x and y are not equal.") else: print("x and y are equal.")
In this code snippet, we compare the values of "x" and "y" using the "not equal" operator. If the values are not equal, the first block of code is executed and the message "x and y are not equal" is printed. Otherwise, if the values are equal, the second block of code is executed and the message "x and y are equal" is printed.
Applying Not Equal Operator in Conditional Statements
As mentioned earlier, the "not equal" operator is commonly used in conditional statements to make decisions based on the inequality of values. Let's consider an example where we use the "not equal" operator to check if a number is odd or even:
number = 9 if number % 2 != 0: print("The number is odd.") else: print("The number is even.")
In this example, we use the "not equal" operator to check if the remainder of "number" divided by 2 is not equal to 0. If the condition is True, it means the number is odd, and the corresponding message is printed. Otherwise, if the condition is False, it means the number is even, and a different message is printed.
Additional Resources
- Python Comparison Operators: Not Equal To