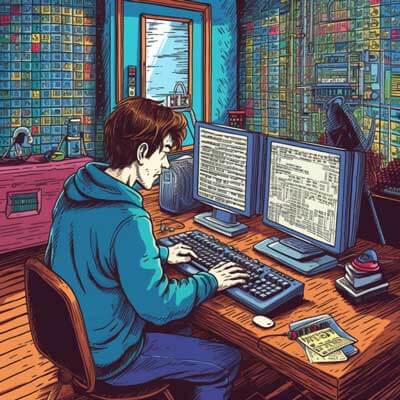
Table of Contents
Overview of Numeric Data Types
Numeric data types are used to store numerical values. These data types are essential for performing arithmetic operations and mathematical calculations. Python provides several built-in numeric data types, each with its own characteristics and use cases. The most commonly used numeric data types in Python are integers, floating-point numbers, and complex numbers.
Related Article: Python Set Intersection Tutorial
Integers
Integers, or int, are whole numbers without a fractional component. They can be positive, negative, or zero. In Python, integers are represented using the int class. For example, x = 10
assigns the integer value 10 to the variable x.
Floating-Point Numbers
Floating-point numbers, or float, are numbers with a fractional component. They can be positive, negative, or zero. In Python, floating-point numbers are represented using the float class. For example, y = 3.14
assigns the floating-point value 3.14 to the variable y.
Complex Numbers
Complex numbers, or complex, are numbers with both a real and imaginary part. They are represented as a + bj
, where a is the real part and b is the imaginary part. In Python, complex numbers are represented using the complex class. For example, z = 2 + 3j
assigns the complex value 2 + 3j to the variable z.
Related Article: Tutorial: Django + MongoDB, ElasticSearch & Message Brokers
Numeric Operations in Python
Python provides a wide range of built-in operators and functions for performing numeric operations. These operations can be used with different numeric data types to perform arithmetic calculations, comparisons, and other mathematical manipulations. Some of the common numeric operations in Python include addition, subtraction, multiplication, division, modulus, exponentiation, and more.
Addition (+)
The addition operator (+) is used to perform addition between two numbers. It can be used with both integers and floating-point numbers. For example:
x = 5 y = 3 result = x + y print(result) # Output: 8
Subtraction (-)
The subtraction operator (-) is used to perform subtraction between two numbers. It can be used with both integers and floating-point numbers. For example:
x = 5 y = 3 result = x - y print(result) # Output: 2
Multiplication (*)
The multiplication operator (*) is used to perform multiplication between two numbers. It can be used with both integers and floating-point numbers. For example:
x = 5 y = 3 result = x * y print(result) # Output: 15
Related Article: How To Merge Dictionaries In Python
Division (/)
The division operator (/) is used to perform division between two numbers. It always returns a floating-point result, even if the inputs are integers. For example:
x = 10 y = 3 result = x / y print(result) # Output: 3.3333333333333335
Modulus (%)
The modulus operator (%) is used to find the remainder of a division operation. It can be used with both integers and floating-point numbers. For example:
x = 10 y = 3 result = x % y print(result) # Output: 1
Exponentiation (**)
The exponentiation operator (**) is used to raise a number to a power. It can be used with both integers and floating-point numbers. For example:
x = 2 y = 3 result = x ** y print(result) # Output: 8
Using the isnumeric() Method
The isnumeric() method is a built-in method in Python that can be used to check if a string contains only numeric characters. It returns True if all the characters in the string are numeric, and False otherwise. This method can be useful when validating user input or processing data that should only contain numbers.
To use the isnumeric() method, simply call it on a string object. For example:
num1 = "12345" num2 = "12.34" num3 = "abc123" print(num1.isnumeric()) # Output: True print(num2.isnumeric()) # Output: False print(num3.isnumeric()) # Output: False
In the above example, num1 contains only numeric characters, so its isnumeric() method returns True. However, num2 contains a decimal point, and num3 contains alphabetic characters, so their isnumeric() methods return False.
It's important to note that the isnumeric() method only considers characters from the Unicode character set as numeric. This means that certain characters, such as superscripts, subscripts, and fractions, may not be considered numeric by the method.
Related Article: How to Work with Encoding & Multiple Languages in Django
Performing Numeric Operations in Python
To perform numeric operations in Python, you can simply use the appropriate operators or functions for the desired operation. Python's rich set of numeric operators allows you to perform complex calculations and manipulate numeric data with ease.
Here are some examples of performing numeric operations in Python:
Example 1: Addition
x = 5 + 3 print(x) # Output: 8
In this example, the addition operator (+) is used to add the numbers 5 and 3, and the result 8 is stored in the variable x.
Example 2: Subtraction
x = 5 - 3 print(x) # Output: 2
In this example, the subtraction operator (-) is used to subtract the number 3 from 5, and the result 2 is stored in the variable x.
Example 3: Multiplication
x = 5 * 3 print(x) # Output: 15
In this example, the multiplication operator (*) is used to multiply the numbers 5 and 3, and the result 15 is stored in the variable x.
Related Article: How to Use Inline If Statements for Print in Python
Example 4: Division
x = 10 / 3 print(x) # Output: 3.3333333333333335
In this example, the division operator (/) is used to divide the number 10 by 3, and the result 3.3333333333333335 is stored in the variable x.
Example 5: Modulus
x = 10 % 3 print(x) # Output: 1
In this example, the modulus operator (%) is used to find the remainder of dividing 10 by 3, and the result 1 is stored in the variable x.
Example 6: Exponentiation
x = 2 ** 3 print(x) # Output: 8
In this example, the exponentiation operator (**) is used to raise the number 2 to the power of 3, and the result 8 is stored in the variable x.
Different Numeric Data Types
Python provides different numeric data types to handle various types of numerical values. Understanding the differences between these data types is crucial for writing accurate and efficient code.
Related Article: How to Remove a Key from a Python Dictionary
Integers
Integers are used to represent whole numbers without a fractional component. In Python, integers are represented using the int class. They can be positive, negative, or zero. Integers have a fixed size and can be used for precise calculations. However, they have a limited range compared to other numeric data types.
Floating-Point Numbers
Floating-point numbers are used to represent numbers with a fractional component. In Python, floating-point numbers are represented using the float class. They can be positive, negative, or zero. Floating-point numbers have a larger range compared to integers, but they have limited precision due to the way they are stored in memory.
Complex Numbers
Complex numbers are used to represent numbers with both a real and imaginary part. In Python, complex numbers are represented using the complex class. They can be positive, negative, or zero. Complex numbers are mainly used for mathematical calculations involving complex arithmetic, such as signal processing and electrical engineering.
Additional Resources
- Numeric Data Types in Python