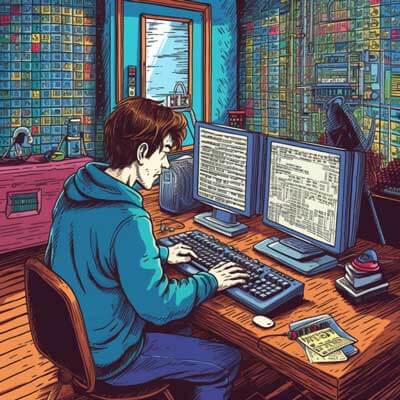
Table of Contents
Introduction to OAuth 2
OAuth 2 is an authorization framework that allows third-party applications to access user resources without the need for them to share their credentials. It provides a secure and standardized way for users to grant limited access to their data to other applications, without compromising their login credentials.
Related Article: Using Regular Expressions to Exclude or Negate Matches
How OAuth 2 Works
OAuth 2 works by separating the roles of the resource owner (the user), the client (the third-party application), and the authorization server (the service that hosts the user's resources). The process involves the exchange of tokens, which grant temporary access to the user's resources.
Example: Initial Authorization Request
GET /authorize? response_type=code &client_id=CLIENT_ID &redirect_uri=REDIRECT_URI &scope=SCOPE &state=STATE HTTP/1.1Host: authorization-server.com
Example: Access Token Request
POST /token HTTP/1.1Host: authorization-server.comContent-Type: application/x-www-form-urlencodedgrant_type=authorization_code&code=AUTHORIZATION_CODE&redirect_uri=REDIRECT_URI&client_id=CLIENT_ID&client_secret=CLIENT_SECRET
OAuth 2: The Basics
OAuth 2 introduces several key concepts and terminologies that are important to understand.
Tokens
Tokens are the central mechanism in OAuth 2. There are two main types of tokens: access tokens and refresh tokens. Access tokens are short-lived and are used to access protected resources on behalf of the user. Refresh tokens are long-lived and are used to obtain new access tokens when they expire.
Example: Access Token Usage
GET /api/resource HTTP/1.1Host: resource-server.comAuthorization: Bearer ACCESS_TOKEN
Example: Token Refresh
POST /token HTTP/1.1Host: authorization-server.comContent-Type: application/x-www-form-urlencodedgrant_type=refresh_token&refresh_token=REFRESH_TOKEN&client_id=CLIENT_ID&client_secret=CLIENT_SECRET
Related Article: How to Use the in Source Query Parameter in Elasticsearch
Client Types
OAuth 2 defines different client types based on their capabilities and level of trust. The client type determines the authentication and authorization mechanisms used during the OAuth 2 process.
Example: Public Client
Public clients are applications that cannot keep a client secret confidential, such as client-side JavaScript applications or mobile apps.
Example: Confidential Client
Confidential clients are applications capable of keeping a client secret confidential, such as server-side web applications or command-line tools.
Grant Types
OAuth 2 defines several grant types, which determine how the client obtains an access token. The choice of grant type depends on the client type and the specific requirements of the application.
Example: Authorization Code Grant
The authorization code grant type is the most common and secure way to obtain an access token. It involves a two-step process where the client first obtains an authorization code, and then exchanges it for an access token.
Example: Client Credentials Grant
The client credentials grant type is used by confidential clients to obtain an access token directly from the authorization server, without involving the user.
Use Case: Single Page Applications
Single Page Applications (SPAs) are web applications that load a single HTML page and dynamically update the content as the user interacts with the application. SPAs often rely on OAuth 2 for authentication and authorization.
Code Snippet: Initial Authorization Request
const authorize = () => { window.location.href = `https://authorization-server.com/authorize?response_type=token&client_id=CLIENT_ID&redirect_uri=REDIRECT_URI&scope=SCOPE&state=STATE`;};
Related Article: Troubleshooting 502 Bad Gateway Nginx
Code Snippet: Access Token Usage
fetch('https://api.example.com/resource', { headers: { Authorization: `Bearer ACCESS_TOKEN` }}).then(response => response.json()).then(data => { // Handle response data}).catch(error => { // Handle error});
Use Case: Mobile Applications
Mobile applications often require OAuth 2 to authenticate and access user resources. OAuth 2 provides a secure and standardized way for mobile apps to obtain access tokens and interact with APIs.
Code Snippet: Initial Authorization Request
String authorizationUrl = "https://authorization-server.com/authorize?" + "response_type=code&" + "client_id=CLIENT_ID&" + "redirect_uri=REDIRECT_URI&" + "scope=SCOPE&" + "state=STATE";Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(authorizationUrl));startActivity(intent);
Code Snippet: Access Token Request
String tokenUrl = "https://authorization-server.com/token";HttpURLConnection connection = (HttpURLConnection) new URL(tokenUrl).openConnection();connection.setRequestMethod("POST");connection.setDoOutput(true);String requestBody = "grant_type=authorization_code" + "&code=AUTHORIZATION_CODE" + "&redirect_uri=REDIRECT_URI" + "&client_id=CLIENT_ID" + "&client_secret=CLIENT_SECRET";OutputStream outputStream = connection.getOutputStream();outputStream.write(requestBody.getBytes());outputStream.flush();// Handle response
This is just a small portion of the content that can be covered in an article about OAuth 2. By diving deeper into each chapter, you can gain a comprehensive understanding of OAuth 2 and its various aspects.