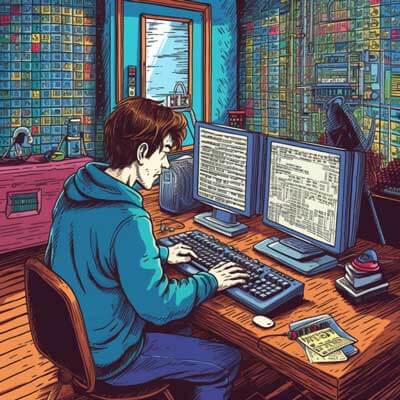
Table of Contents
Laravel Deployment Strategies
Laravel is a popular PHP framework that allows developers to create web applications quickly and efficiently. Once your Laravel application is complete, the next step is to deploy it to a production environment. There are several deployment strategies you can use to ensure a smooth and efficient deployment process.
Related Article: How to Use the PHP Random String Generator
Laravel Production Environment Setup
Before deploying your Laravel application, it's important to set up a production environment that is optimized for performance and security. This involves configuring your web server, database, and other server components to ensure your application runs smoothly in a production environment.
One important aspect of setting up a production environment is configuring your environment variables. Laravel uses a .env file to store sensitive information such as database credentials and API keys. In a production environment, it's important to secure these variables and prevent unauthorized access. One way to do this is by using a tool like Laravel Vault, which encrypts your environment variables and securely stores them.
Another important aspect of setting up a production environment is optimizing your server configuration. This involves configuring your web server, such as Apache or Nginx, to handle the load of your application. You can optimize your server configuration by enabling caching, compressing assets, and using a content delivery network (CDN) to serve static files.
Envoy Deployment for Laravel
Envoy is a task runner built specifically for Laravel that allows you to define and run deployment tasks for your application. With Envoy, you can automate the deployment process and easily deploy your Laravel application to multiple servers.
To use Envoy for deployment, you first need to install it globally using Composer. Once installed, you can create an Envoy.blade.php file in the root of your Laravel project. This file will contain the deployment tasks and configuration for your application.
Here is an example of a simple Envoy deployment task:
@servers(['web' => 'user@your-server']) @task('deploy', ['on' => 'web']) cd /path/to/your/laravel/project git pull origin master composer install --no-dev php artisan migrate --force @endtask
In this example, the deploy
task pulls the latest changes from the master
branch, installs the production dependencies, and runs the database migrations. To deploy your application, you can simply run the following command:
envoy run deploy
Laravel Forge Deployment
Laravel Forge is a server management and deployment platform that simplifies the process of deploying Laravel applications. With Forge, you can easily provision servers, configure your environment, and deploy your Laravel application with just a few clicks.
To deploy a Laravel application using Forge, you first need to create a server and provision it with Forge. Once your server is ready, you can connect your Git repository to Forge and configure the deployment settings for your application.
Forge supports both manual and automatic deployment options. With manual deployment, you can deploy your application with a single click from the Forge dashboard. With automatic deployment, Forge can automatically deploy your application whenever you push changes to your Git repository.
Related Article: How to Fix Error 419 Page Expired in Laravel Post Request
Laravel Vapor Deployment
Laravel Vapor is a serverless deployment platform for Laravel applications. It allows you to deploy your Laravel application to AWS Lambda and other serverless infrastructure, eliminating the need for managing servers and scaling infrastructure manually.
To deploy a Laravel application using Vapor, you first need to install the Vapor CLI. Once installed, you can configure your Laravel application to work with Vapor by running the following command:
php artisan vapor:install
This command will generate a vapor.yml
file in the root of your Laravel project. This file contains the configuration for your Vapor deployment.
To deploy your application, you can run the following command:
php artisan vapor deploy
Vapor will package and upload your application to AWS Lambda, and automatically handle scaling and provisioning of the serverless infrastructure.
Horizontal Scaling Laravel
Horizontal scaling is a technique used to handle increased traffic and load on your Laravel application by adding more servers to your infrastructure. By distributing the workload across multiple servers, you can improve the performance and reliability of your application.
To horizontally scale a Laravel application, you need to set up a load balancer that distributes incoming requests across multiple servers. This ensures that each server receives an equal share of the workload and prevents any single server from becoming overloaded.
There are several load balancing strategies you can use with Laravel, including round-robin, least connections, and IP hash. These strategies determine how requests are distributed among the servers in your infrastructure.
One popular tool for setting up load balancing in Laravel is AWS Elastic Load Balancer (ELB). ELB is a fully managed load balancing service provided by AWS that automatically distributes incoming traffic across multiple EC2 instances.
To set up load balancing with AWS ELB, you first need to create a load balancer and configure it to distribute traffic to your EC2 instances. You can then associate your Laravel application with the load balancer and configure the necessary health checks and routing rules.
Another option for load balancing in Laravel is using a reverse proxy server like Nginx or Apache. These servers can act as a load balancer and distribute incoming requests to multiple backend servers.
To set up load balancing with Nginx, you need to configure the upstream block in your Nginx configuration file. Here is an example:
http { upstream backend { server backend1.example.com; server backend2.example.com; server backend3.example.com; } server { listen 80; server_name example.com; location / { proxy_pass http://backend; proxy_set_header Host $host; } } }
In this example, the upstream
block defines the backend servers that Nginx will distribute requests to. The proxy_pass
directive specifies the URL to proxy requests to, and the proxy_set_header
directive sets the Host
header to the original request host.
Optimizing Laravel for Production
Optimizing your Laravel application for production involves several techniques and best practices to improve performance, scalability, and security.
Configuring and Optimizing Laravel for Production Environments
When deploying your Laravel application to a production environment, there are several configuration options you can optimize to improve performance and security.
One important configuration option is the APP_ENV
variable in your .env
file. This variable specifies the environment mode for your application, which can be either local
, production
, or testing
. Setting this variable to production
enables performance optimizations and disables debug mode for your application.
Another important configuration option is the APP_DEBUG
variable. By default, Laravel sets this variable to true
in the development environment, which enables detailed error reporting. In a production environment, it's recommended to set this variable to false
to disable error reporting and improve performance.
You can also optimize your database configuration by enabling query caching and optimizing database queries. Laravel provides a query builder that allows you to write database queries using a fluent and intuitive syntax. By using the query builder and optimizing your queries, you can improve the performance of your application.
Related Article: How to Echo or Print an Array in PHP
Deployment Strategies for Laravel Apps using Envoy, Forge, and Vapor
There are several deployment strategies you can use to deploy your Laravel application, depending on your requirements and infrastructure.
If you prefer a simple and lightweight deployment strategy, you can use Envoy. Envoy allows you to define and run deployment tasks for your Laravel application, making it easy to automate the deployment process.
If you are looking for a more comprehensive server management and deployment platform, Laravel Forge is a great choice. Forge simplifies the process of deploying Laravel applications by providing a user-friendly interface for server provisioning, configuration, and deployment.
If you want to take advantage of serverless infrastructure and eliminate the need for managing servers, Laravel Vapor is the way to go. Vapor allows you to deploy your Laravel application to AWS Lambda and other serverless infrastructure, providing automatic scaling and provisioning.
Horizontally Scaling a Laravel App
To horizontally scale a Laravel application, you need to distribute the workload across multiple servers. This can be achieved by setting up a load balancer that distributes incoming requests among the servers in your infrastructure.
There are several load balancing strategies you can use with Laravel, including round-robin, least connections, and IP hash. These strategies determine how requests are distributed among the servers and can be configured based on your specific requirements.
In addition to load balancing, you can also use caching to improve the performance and scalability of your Laravel application. Laravel provides a built-in caching system that allows you to cache database queries, views, and other expensive operations. By caching frequently accessed data, you can reduce the load on your servers and improve the response time of your application.
Load Balancing Strategies for Laravel
Load balancing is an essential technique for distributing incoming requests across multiple servers in order to improve the performance and reliability of a Laravel application. There are several load balancing strategies you can use with Laravel, including round-robin, least connections, and IP hash.
The round-robin strategy is the simplest load balancing strategy, where each request is distributed to the next server in a circular order. This ensures that each server receives an equal share of the workload.
The least connections strategy distributes requests to the server with the fewest active connections. This strategy is useful when the workload on each server is not uniform, as it helps to evenly distribute the load.
The IP hash strategy distributes requests based on the client's IP address. This ensures that requests from the same client are always sent to the same server. This strategy is useful when you need to maintain session state or when you have specific requirements for routing requests.
To implement load balancing in Laravel, you can use a reverse proxy server like Nginx or Apache. These servers can act as a load balancer and distribute incoming requests to multiple backend servers.
Best Practices for Deploying Laravel in a Production Environment
Deploying a Laravel application to a production environment requires careful planning and adherence to best practices. Here are some best practices to follow when deploying Laravel in a production environment:
1. Use a version control system: Use a version control system like Git to manage your codebase and track changes. This allows you to easily roll back to previous versions in case of issues.
2. Implement a CI/CD pipeline: Set up a continuous integration and continuous deployment (CI/CD) pipeline to automate the deployment process. This ensures that your application is always up to date and reduces the risk of human error during deployment.
3. Use environment variables: Store sensitive information like database credentials and API keys in environment variables. This prevents the exposure of sensitive information in your codebase.
4. Secure your application: Implement security measures such as SSL/TLS encryption, CSRF protection, and input validation to protect your application from attacks.
5. Monitor your application: Set up monitoring and logging tools to track the performance and health of your application. This allows you to identify and fix issues before they impact your users.
6. Regularly backup your data: Implement a backup strategy to regularly back up your data and ensure that you can recover from data loss or system failures.
Related Article: How To Get The Full URL In PHP
Advantages of Using Laravel Forge for Deployment
Laravel Forge is a server management and deployment platform that simplifies the process of deploying Laravel applications. Here are some advantages of using Laravel Forge for deployment:
1. Easy server provisioning: Laravel Forge allows you to easily provision servers on popular cloud providers like AWS, DigitalOcean, and Linode. It takes care of the server setup and configuration, allowing you to focus on your application.
2. User-friendly interface: Forge provides a user-friendly web interface for managing your servers and deploying your Laravel applications. It simplifies complex server management tasks and makes them accessible to developers of all skill levels.
3. One-click deployment: With Forge, you can deploy your Laravel application with just a single click. Forge automatically configures your server, installs the necessary dependencies, and deploys your application.
4. Automatic SSL/TLS certificate management: Forge integrates with Let's Encrypt to automatically provision and renew SSL/TLS certificates for your domains. This ensures that your application is secure and accessible over HTTPS.
5. Built-in monitoring and alerts: Forge provides built-in monitoring and alerting capabilities. You can easily track the performance and health of your application and receive alerts when issues arise.
Introduction to Laravel Vapor and its Deployment
Laravel Vapor is a serverless deployment platform for Laravel applications. It allows you to deploy your Laravel application to AWS Lambda and other serverless infrastructure, eliminating the need for managing servers and scaling infrastructure manually.
Vapor seamlessly integrates with Laravel and provides a simplified deployment process for serverless applications. Instead of provisioning and managing servers, Vapor automatically scales your application based on demand and provisions the necessary infrastructure.
To deploy a Laravel application using Vapor, you first need to install the Vapor CLI. Once installed, you can configure your Laravel application to work with Vapor by running the following command:
php artisan vapor:install
This command will generate a vapor.yml
file in the root of your Laravel project. This file contains the configuration for your Vapor deployment, including the AWS services and resources to be provisioned.
To deploy your application, you can run the following command:
php artisan vapor deploy
Vapor will package and upload your application to AWS Lambda, and automatically handle scaling and provisioning of the serverless infrastructure.
Steps to Optimize Laravel for High Traffic Production Environments
Optimizing a Laravel application for high traffic production environments involves several steps to improve performance, scalability, and reliability. Here are some steps to optimize Laravel for high traffic production environments:
1. Use caching: Implement caching for frequently accessed data, such as database queries and views. Laravel provides a built-in caching system that allows you to cache data using various drivers, such as Redis or Memcached.
2. Optimize database queries: Use Laravel's query builder to write efficient and optimized database queries. Avoid unnecessary joins, use indexes where appropriate, and paginate large result sets to improve performance.
3. Enable opcode caching: Enable opcode caching in your PHP configuration to cache the compiled bytecode of your PHP scripts. This reduces the overhead of compiling PHP code on each request and improves performance.
4. Use a content delivery network (CDN): Implement a CDN to serve static assets, such as images, CSS, and JavaScript files. This reduces the load on your servers and improves the response time for users in different geographical locations.
5. Scale horizontally: Distribute the workload across multiple servers by implementing load balancing. This ensures that each server receives an equal share of the workload and prevents any single server from becoming a bottleneck.
6. Monitor and optimize performance: Implement monitoring and profiling tools to identify performance bottlenecks and optimize your application. Use tools like New Relic or Blackfire to track the performance of your application and identify areas for improvement.
Tools and Techniques for Load Balancing a Laravel Application
Load balancing is a critical technique for distributing incoming requests across multiple servers in order to improve the performance and reliability of a Laravel application. Here are some tools and techniques for load balancing a Laravel application:
1. AWS Elastic Load Balancer (ELB): AWS ELB is a fully managed load balancing service provided by Amazon Web Services. It automatically distributes incoming traffic across multiple EC2 instances and provides advanced features such as SSL termination and session stickiness.
2. Nginx: Nginx is a popular web server and reverse proxy server that can also act as a load balancer. It supports various load balancing algorithms, including round-robin, least connections, and IP hash. Nginx can be configured to distribute incoming requests to multiple backend servers.
3. HAProxy: HAProxy is an open-source load balancer and proxy server that provides high availability and load balancing for TCP and HTTP-based applications. It supports various load balancing algorithms and can be configured to distribute requests to multiple backend servers.
4. Apache HTTP Server: Apache HTTP Server is a widely used web server that can also act as a load balancer. It supports various load balancing algorithms and can be configured to distribute requests across multiple backend servers using the mod_proxy_balancer module.
5. Kubernetes: Kubernetes is a container orchestration platform that provides built-in load balancing capabilities. It can automatically distribute incoming requests to containers running your Laravel application and scale the number of containers based on demand.
6. Docker Swarm: Docker Swarm is a native clustering and orchestration solution for Docker. It allows you to create a swarm of Docker nodes and distribute incoming requests to containers running your Laravel application.
These are just a few examples of tools and techniques for load balancing a Laravel application. The choice of tool or technique depends on your specific requirements and infrastructure. Make sure to evaluate and choose the most suitable option for your application.
Related Article: How to Use PHP Curl for HTTP Post
Additional Resources
- How to configure and optimize Laravel for production environments?