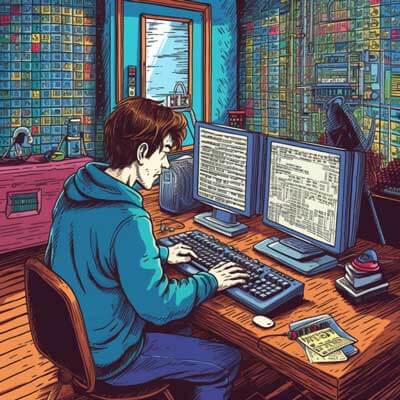
Table of Contents
Passing Parameters to Components
In ReactJS, passing parameters to components is a fundamental concept that allows us to customize and configure our components based on specific requirements. It enables us to create reusable and flexible components that can be used in different scenarios.
Related Article: How to Set Up Your First ReactJS Project
Pass a Parameter to a React Component
To pass a parameter to a React component, we can use props. Props, short for properties, are used to pass data from a parent component to its child components. Props can be any JavaScript value, such as strings, numbers, objects, or functions.
Here's an example of how to pass a parameter to a React component:
// ParentComponent.tsx import React from 'react'; import ChildComponent from './ChildComponent'; const ParentComponent = () => { const parameter = 'Hello, World!'; return ( <div> <ChildComponent parameter={parameter} /> </div> ); }; export default ParentComponent; // ChildComponent.tsx import React from 'react'; type Props = { parameter: string; }; const ChildComponent = (props: Props) => { return <div>{props.parameter}</div>; }; export default ChildComponent;
In this example, the ParentComponent
passes the parameter
variable as a prop to the ChildComponent
. The ChildComponent
receives the prop through its function parameter and displays it within a div
element.
React Component Props
React component props are a way to pass data from parent components to child components. Props are immutable and can only be passed from parent to child, not vice versa. They allow us to customize the behavior and appearance of components based on specific requirements.
Using Props in React Component
To use props in a React component, we can access them through the function parameter or by using the this.props
object in a class component.
Here's an example of how to use props in a React component:
// GreetingComponent.tsx import React from 'react'; type Props = { name: string; }; const GreetingComponent = (props: Props) => { return <div>Hello, {props.name}!</div>; }; export default GreetingComponent;
In this example, the GreetingComponent
receives the name
prop through its function parameter and displays a greeting message using the prop value.
Related Article: Adding a Newline in the JSON Property Render with ReactJS
React TypeScript Component Parameter
In React with TypeScript, we can define the type of component parameters using TypeScript interfaces or types. This helps us ensure type safety and avoid potential errors when passing parameters to components.
Pass a Parameter to a React Component in TypeScript
To pass a parameter to a React component in TypeScript, we can define the type of the parameter using an interface or type.
Here's an example of how to pass a parameter to a React component in TypeScript:
// ParentComponent.tsx import React from 'react'; import ChildComponent from './ChildComponent'; interface Props { parameter: string; } const ParentComponent: React.FC<Props> = ({ parameter }) => { return ( <div> <ChildComponent parameter={parameter} /> </div> ); }; export default ParentComponent; // ChildComponent.tsx import React from 'react'; interface Props { parameter: string; } const ChildComponent: React.FC<Props> = ({ parameter }) => { return <div>{parameter}</div>; }; export default ChildComponent;
In this example, we define the type of the parameter
prop using the Props
interface in both the ParentComponent
and ChildComponent
. The prop types are then used in the function parameters of the components.
React Component Props in TypeScript
In TypeScript, React component props can be defined using interfaces or types. These props allow us to specify the types of the props and ensure type safety when passing data between components.
Using Props in React Component with TypeScript
To use props in a React component with TypeScript, we can access them through the function parameter or by using the this.props
object in a class component, specifying the types of the props using interfaces or types.
Here's an example of how to use props in a React component with TypeScript:
// GreetingComponent.tsx import React from 'react'; interface Props { name: string; } const GreetingComponent: React.FC<Props> = ({ name }) => { return <div>Hello, {name}!</div>; }; export default GreetingComponent;
In this example, the GreetingComponent
receives the name
prop through its function parameter and displays a greeting message using the prop value. The prop type is specified using the Props
interface.
Related Article: Inserting Plain Text into an Array Using ReactJS
React Component Parameter Example
Here's an example of a React component with a parameter:
// CounterComponent.tsx import React from 'react'; type Props = { initialValue: number; }; const CounterComponent = (props: Props) => { const [count, setCount] = React.useState(props.initialValue); const increment = () => { setCount(count + 1); }; const decrement = () => { setCount(count - 1); }; return ( <div> <div>Count: {count}</div> <button onClick={increment}>Increment</button> <button onClick={decrement}>Decrement</button> </div> ); }; export default CounterComponent;
In this example, the CounterComponent
receives an initialValue
prop and uses it to initialize the state variable count
. The component provides buttons to increment and decrement the count value.
Pass a Parameter to a React Component Example
Here's an example of how to pass a parameter to a React component:
// ParentComponent.tsx import React from 'react'; import ChildComponent from './ChildComponent'; const ParentComponent = () => { const parameter = 'Hello, World!'; return ( <div> <ChildComponent parameter={parameter} /> </div> ); }; export default ParentComponent; // ChildComponent.tsx import React from 'react'; type Props = { parameter: string; }; const ChildComponent = (props: Props) => { return <div>{props.parameter}</div>; }; export default ChildComponent;
In this example, the ParentComponent
passes the parameter
variable as a prop to the ChildComponent
. The ChildComponent
receives the prop through its function parameter and displays it within a div
element.
What are Props in React Component?
Props in React components are a way to pass data from parent components to child components. They allow us to customize and configure components based on specific requirements. Props are immutable and can only be passed from parent to child, not vice versa.
How to Pass a Parameter to a React Component?
To pass a parameter to a React component, we can use props. Props are passed from parent components to child components and can be any JavaScript value. To pass a parameter, simply assign the value to a prop in the parent component and access it in the child component.
Related Article: Enhancing React Applications with Third-Party Integrations
Can I Pass Multiple Parameters to a React Component?
Yes, you can pass multiple parameters to a React component by assigning multiple props in the parent component and accessing them in the child component. Each prop will represent a different parameter that can be used to customize the behavior and appearance of the component.
Difference Between Props and State in React
In React, props and state are both used to manage data in components, but they have different purposes and characteristics.
- Props: Props are used to pass data from parent components to child components. They are immutable and cannot be modified by the child components. Props are passed down the component tree and allow components to be reusable and customizable.
- State: State is used to manage the internal data of a component. It is mutable and can be modified by the component itself. State allows components to maintain and update their own data, triggering re-renders when the state changes.
Accessing Passed Parameter in a React Component
To access a passed parameter in a React component, we can use the props object or the function parameter in a functional component. In a class component, we can access the passed parameter using this.props.parameter
.
Here's an example of how to access a passed parameter in a React component:
// ChildComponent.tsx import React from 'react'; type Props = { parameter: string; }; const ChildComponent = (props: Props) => { return <div>{props.parameter}</div>; }; export default ChildComponent;
In this example, the ChildComponent
receives the parameter
prop through its function parameter and displays it within a div
element.
Mutability of Props in React
In React, props are immutable, which means they cannot be modified by the child components. Once a prop is passed to a component, it cannot be changed from within that component. This helps maintain the data flow and ensures that components are predictable and reusable.
Related Article: How to Use a For Loop Inside Render in ReactJS
Changing the Value of a Prop in a React Component
Since props are immutable in React, we cannot directly change the value of a prop within a component. If we need to update the value of a prop, we should do it in the parent component and pass the updated value as a new prop to the child component.
Here's an example of how to update the value of a prop in a React component:
// ParentComponent.tsx import React from 'react'; import ChildComponent from './ChildComponent'; const ParentComponent = () => { const [parameter, setParameter] = React.useState('Hello, World!'); const updateParameter = () => { setParameter('Hello, React!'); }; return ( <div> <button onClick={updateParameter}>Update Parameter</button> <ChildComponent parameter={parameter} /> </div> ); }; export default ParentComponent; // ChildComponent.tsx import React from 'react'; type Props = { parameter: string; }; const ChildComponent = (props: Props) => { return <div>{props.parameter}</div>; }; export default ChildComponent;
In this example, the ParentComponent
maintains the parameter
state and provides a button to update its value. When the button is clicked, the updateParameter
function is called, updating the state value. The updated value is then passed as a prop to the ChildComponent
.
Passing Parameters to a React Component using TypeScript
In TypeScript, we can define the types of component parameters using interfaces or types. This helps ensure type safety and avoid potential errors when passing parameters to React components.
Here's an example of how to pass parameters to a React component using TypeScript:
// ParentComponent.tsx import React from 'react'; import ChildComponent from './ChildComponent'; interface Props { parameter1: string; parameter2: number; } const ParentComponent: React.FC<Props> = ({ parameter1, parameter2 }) => { return ( <div> <ChildComponent parameter1={parameter1} parameter2={parameter2} /> </div> ); }; export default ParentComponent; // ChildComponent.tsx import React from 'react'; interface Props { parameter1: string; parameter2: number; } const ChildComponent: React.FC<Props> = ({ parameter1, parameter2 }) => { return ( <div> <div>{parameter1}</div> <div>{parameter2}</div> </div> ); }; export default ChildComponent;
In this example, we define the types of the parameter1
and parameter2
props using the Props
interface in both the ParentComponent
and ChildComponent
. The prop types are then used in the function parameters of the components.
Limitations of Passing Parameters to React Components in TypeScript
When passing parameters to React components in TypeScript, there are a few limitations to keep in mind:
1. Prop types must match the defined types: The types of the props passed to a component must match the defined types in the component's interface or type. Otherwise, TypeScript will throw an error.
2. Immutable props: Just like in regular React, props in TypeScript are immutable and cannot be modified within the component. If you need to update a prop value, you should do it in the parent component and pass the updated value as a new prop.
3. Prop drilling: When passing props through multiple levels of components, it can become tedious and result in prop drilling. Prop drilling is the process of passing props through intermediate components that don't use the props themselves. This can make the code harder to maintain and lead to unnecessary re-renders.
4. TypeScript setup: To use TypeScript with React, you need to set up the necessary TypeScript configuration files and dependencies. This can be an additional step compared to regular JavaScript development.
Despite these limitations, using TypeScript with React provides the benefits of type safety and improved code maintainability.
Passing an Object as a Parameter to a React Component
In React, we can pass an object as a parameter to a component by using object destructuring in the component's function parameter or by accessing the object directly through props.
Here's an example of how to pass an object as a parameter to a React component:
// ParentComponent.tsx import React from 'react'; import ChildComponent from './ChildComponent'; const ParentComponent = () => { const parameter = { name: 'John', age: 25 }; return ( <div> <ChildComponent {...parameter} /> </div> ); }; export default ParentComponent; // ChildComponent.tsx import React from 'react'; type Props = { name: string; age: number; }; const ChildComponent = ({ name, age }: Props) => { return ( <div> <div>Name: {name}</div> <div>Age: {age}</div> </div> ); }; export default ChildComponent;
In this example, the ParentComponent
passes an object parameter
as props to the ChildComponent
using object spread syntax ({...parameter}
). The ChildComponent
then destructures the props object to access the name
and age
properties. The component displays the values of these properties within div
elements.
Passing an object as a parameter allows us to pass multiple related values to a component in a structured way.
Related Article: Handling State Persistence in ReactJS After Refresh
Additional Resources
- React Components and Props in TypeScript