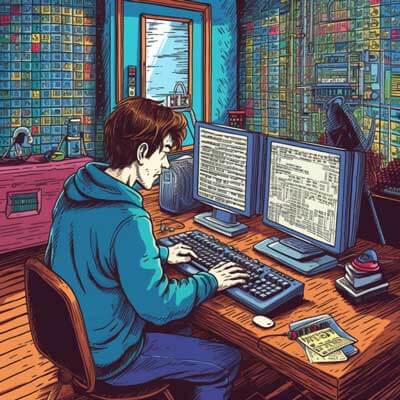
Table of Contents
The Phoenix framework, built on the Elixir language, provides a useful and flexible platform for building web applications. As with any software development project, it is important to follow best practices and utilize design patterns to ensure maintainability, scalability, and extensibility. In this article, we will explore three important design patterns for Phoenix: the Actor Model, Repositories, and Events.
Actor Model and its Role in Phoenix Design Patterns
The Actor Model is a concurrency model that provides a way to build concurrent and distributed systems by modeling them as interacting entities called actors. In Phoenix, the Actor Model is implemented using GenServers, which are processes that encapsulate state and behavior. Each GenServer can receive messages and respond to them, allowing for concurrent and asynchronous processing.
To demonstrate the Actor Model in action, let's consider a simple example of a chat application. We can create a GenServer to represent a chat room, which will handle incoming messages and broadcast them to all connected clients. Here's an example implementation:
defmodule ChatRoom do use GenServer def start_link do GenServer.start_link(__MODULE__, []) end def init(state) do {:ok, state} end def handle_cast({:join, user}, state) do {:noreply, [user | state]} end def handle_cast({:message, message}, state) do IO.puts("Received message: #{message}") {:noreply, state} end def broadcast(message, state) do Enum.each(state, fn user -> IO.puts("Broadcasting message to #{user}") end) end end
In this example, the ChatRoom
GenServer has two message handlers: one for when a user joins the chat room, and another for when a message is received. When a message is received, it is printed to the console. The broadcast/2
function is used to send the message to all connected users.
To start the ChatRoom
GenServer, we can use the start_link/0
function. Here's an example of how to start the GenServer and send messages:
{:ok, pid} = ChatRoom.start_link() GenServer.cast(pid, {:join, "User1"}) GenServer.cast(pid, {:message, "Hello, world!"})
This example demonstrates how the Actor Model can be used in Phoenix to handle concurrent and asynchronous tasks. By encapsulating state and behavior in GenServers, we can build scalable and fault-tolerant systems.
Related Article: Deployment Strategies and Scaling for Phoenix Apps
Repositories in Phoenix Design Patterns
The Repository pattern is a common design pattern used to abstract the data access layer of an application. In Phoenix, repositories are often used to interact with databases and provide a clean and consistent interface for accessing data.
To demonstrate the Repository pattern in Phoenix, let's consider an example of a blog application. We can create a PostRepository
module to handle operations related to blog posts, such as creating, updating, and retrieving posts. Here's an example implementation:
defmodule PostRepository do def create_post(attrs \\ %{}) do # Logic for creating a new post end def update_post(post_id, attrs) do # Logic for updating a post end def get_post(post_id) do # Logic for retrieving a post end end
In this example, the PostRepository
module provides functions for creating, updating, and retrieving posts. The specific implementation details will depend on the chosen database library and the requirements of the application.
To use the PostRepository
, we can call its functions from other parts of the application. Here's an example of how to create a new post:
PostRepository.create_post(%{title: "Hello, world!", content: "This is my first blog post."})
The Repository pattern helps to decouple the application from specific database implementations and provides a clear separation between the business logic and the data access layer.
Events and their Importance in Phoenix Design Patterns
Events play a crucial role in Phoenix design patterns as they enable loose coupling and allow for asynchronous communication between different parts of the system. Phoenix provides the PubSub module for implementing event-driven patterns.
To demonstrate the importance of events in Phoenix, let's consider an example of a real-time chat application. When a user sends a message, we want to broadcast that message to all connected clients. We can use the PubSub module to publish and subscribe to events. Here's an example implementation:
defmodule ChatPubSub do use Phoenix.PubSub def start_link do Phoenix.PubSub.start_link(__MODULE__, []) end def broadcast_message(message) do Phoenix.PubSub.broadcast(__MODULE__, :message, message) end def handle_event(:message, message, state) do IO.puts("Received message: #{message}") {:ok, state} end end
In this example, the ChatPubSub
module uses the PubSub module provided by Phoenix. The broadcast_message/1
function is used to publish the message event. When a message event is received, the handle_event/3
function is called, which simply prints the message to the console.
To subscribe to the message event, we can use the Phoenix.PubSub.subscribe/2
function. Here's an example of how to subscribe to the message event and receive messages:
{:ok, _} = ChatPubSub.start_link() Phoenix.PubSub.subscribe(ChatPubSub, :message)
This example demonstrates how events can be used in Phoenix to enable asynchronous communication and decoupling between different parts of the system. By publishing events and subscribing to them, we can build real-time and reactive applications.
Understanding the Elixir Language
Elixir is a functional programming language built on top of the Erlang virtual machine (BEAM). It is designed for building scalable and fault-tolerant applications. Elixir provides a useful set of features, including pattern matching, concurrency primitives, and metaprogramming capabilities.
To give you a taste of the Elixir language, let's look at some key features and syntax.
Pattern Matching
Pattern matching is a fundamental concept in Elixir. It allows you to match values against patterns and bind variables accordingly. Here's an example:
case {1, 2, 3} do {1, x, 3} -> IO.puts("Matched: #{x}") _ -> IO.puts("No match") end
In this example, the pattern {1, x, 3}
matches the tuple {1, 2, 3}
and binds the variable x
to the value 2
. The _
is a wildcard pattern that matches anything.
Concurrency Primitives
Elixir provides built-in concurrency primitives, including processes, message passing, and supervisors. Processes in Elixir are lightweight and isolated, and they communicate with each other by sending and receiving messages. Here's an example:
pid = spawn(fn -> IO.puts("Hello, world!") end) send(pid, :message) receive do :message -> IO.puts("Received message") end
In this example, we spawn a new process that prints "Hello, world!" and then send a message to that process. The receiving process matches on the :message
pattern and prints "Received message".
Metaprogramming
Elixir provides useful metaprogramming capabilities, allowing you to generate code dynamically at compile-time. This can be useful for generating boilerplate code or implementing domain-specific languages. Here's an example:
defmacro assert_positive(number) do quote do if unquote(number) < 0 do raise "Number must be positive" end end end defmodule MyModule do def my_function(number) do assert_positive(number) # Rest of the function end end
In this example, the assert_positive/1
macro generates code that checks if a given number is positive. The macro is then used in the my_function/1
function to ensure that the number passed to it is positive.
This is just a brief introduction to the Elixir language. To fully understand and utilize the power of Elixir, it is recommended to dive deeper into its documentation and explore the available libraries and frameworks.
Related Article: Integrating Phoenix Web Apps with Payment, Voice & Text
Exploring the Phoenix Framework
Phoenix is a web framework for building high-performance and fault-tolerant applications. It is built on top of the Elixir language and draws inspiration from frameworks such as Ruby on Rails. Phoenix provides a set of conventions and abstractions that make it easy to build scalable and maintainable web applications.
To get started with Phoenix, you will need to install the Phoenix framework and its dependencies. Here's a step-by-step guide to installing Phoenix:
Step 1: Install Elixir
Before installing Phoenix, you will need to install Elixir, the programming language on which Phoenix is built. Elixir provides precompiled packages for major operating systems, as well as instructions for building from source. You can find installation instructions on the Elixir website: https://elixir-lang.org/install.html
Step 2: Install Phoenix
Once you have Elixir installed, you can install Phoenix using the mix
package manager. Open a terminal and run the following command:
mix archive.install hex phx_new
This command installs the Phoenix Mix archive, which provides the phx.new
task for generating new Phoenix projects.
Step 3: Create a New Phoenix Project
To create a new Phoenix project, navigate to the directory where you want to create the project and run the following command:
mix phx.new my_app
This command generates a new Phoenix project in a directory named my_app
. It installs the necessary dependencies and sets up the project structure.
Step 4: Start the Phoenix Server
Once the project is generated, navigate to the project directory and start the Phoenix server by running the following command:
cd my_app mix phx.server
This command starts the Phoenix server and makes the application available at http://localhost:4000
.
Congratulations! You have successfully installed Phoenix and created a new Phoenix project. You can now start exploring the features and capabilities of the framework.
GenServer: A Key Component in Elixir
GenServer is a behavior provided by Elixir that allows you to define processes that encapsulate state and behavior. GenServers are a key component in Elixir for building concurrent and fault-tolerant systems.
To demonstrate how GenServers work, let's consider an example of a counter. We can create a GenServer to represent the counter, which will handle increment and decrement operations. Here's an example implementation:
defmodule Counter do use GenServer def start_link do GenServer.start_link(__MODULE__, 0) end def init(initial_value) do {:ok, initial_value} end def handle_call({:increment, value}, _from, state) do {:reply, state + value, state + value} end def handle_call({:decrement, value}, _from, state) do {:reply, state - value, state - value} end end
In this example, the Counter
GenServer has two call handlers: one for incrementing the counter and another for decrementing it. When a call is made to the GenServer, it replies with the updated counter value.
To start the Counter
GenServer, we can use the start_link/0
function. Here's an example of how to start the GenServer and make calls:
{:ok, pid} = Counter.start_link() GenServer.call(pid, {:increment, 5}) GenServer.call(pid, {:decrement, 3})
This example demonstrates how GenServers can be used to encapsulate state and behavior in concurrent systems. By handling calls and maintaining state, GenServers provide a useful abstraction for building fault-tolerant and scalable applications.
Implementing the Repository Pattern in Elixir
The Repository pattern is a common design pattern used to abstract the data access layer of an application. In Elixir, we can implement the Repository pattern using modules and functions that encapsulate the database operations.
To demonstrate the Repository pattern in Elixir, let's consider an example of a blog application. We can create a PostRepository
module to handle operations related to blog posts, such as creating, updating, and retrieving posts. Here's an example implementation:
defmodule PostRepository do def create_post(attrs \\ %{}) do # Logic for creating a new post end def update_post(post_id, attrs) do # Logic for updating a post end def get_post(post_id) do # Logic for retrieving a post end end
In this example, the PostRepository
module provides functions for creating, updating, and retrieving posts. The specific implementation details will depend on the chosen database library and the requirements of the application.
To use the PostRepository
, we can call its functions from other parts of the application. Here's an example of how to create a new post:
PostRepository.create_post(%{title: "Hello, world!", content: "This is my first blog post."})
The Repository pattern helps to decouple the application from specific database implementations and provides a clear separation between the business logic and the data access layer.
Utilizing the Service Pattern in Elixir
The Service pattern is a design pattern that allows you to encapsulate complex business logic into reusable and testable components. In Elixir, we can implement the Service pattern using modules and functions that represent the different services in the application.
To demonstrate the Service pattern in Elixir, let's consider an example of a shopping cart application. We can create a CartService
module to handle operations related to the shopping cart, such as adding items, removing items, and calculating the total price. Here's an example implementation:
defmodule CartService do def add_item(cart, item) do # Logic for adding an item to the cart end def remove_item(cart, item) do # Logic for removing an item from the cart end def calculate_total(cart) do # Logic for calculating the total price of the cart end end
In this example, the CartService
module provides functions for adding items, removing items, and calculating the total price of the cart. The specific implementation details will depend on the requirements of the application.
To use the CartService
, we can call its functions from other parts of the application. Here's an example of how to add an item to the cart:
cart = %{} item = %{name: "Product 1", price: 10} updated_cart = CartService.add_item(cart, item)
The Service pattern helps to encapsulate complex business logic into reusable components, making the code easier to read, test, and maintain.
Related Article: Elixir’s Phoenix Security: Token Auth & CSRF Prevention
The Concept of Event-Driven Programming
Event-driven programming is a programming paradigm that focuses on the flow of events and the reactions to those events. In event-driven programming, the program is structured around event sources, event handlers, and event loops.
In Elixir, event-driven programming can be implemented using the GenServer behavior and the PubSub module provided by the Phoenix framework. GenServers can handle events by implementing the handle_info/2
callback, while the PubSub module allows for publishing and subscribing to events.
To demonstrate event-driven programming in Elixir, let's consider an example of a real-time chat application. When a user sends a message, we want to broadcast that message to all connected clients. We can use the PubSub module to publish and subscribe to events. Here's an example implementation:
defmodule ChatPubSub do use Phoenix.PubSub def start_link do Phoenix.PubSub.start_link(__MODULE__, []) end def broadcast_message(message) do Phoenix.PubSub.broadcast(__MODULE__, :message, message) end def handle_info({:message, message}, state) do IO.puts("Received message: #{message}") {:noreply, state} end end
In this example, the ChatPubSub
module uses the PubSub module provided by Phoenix. The broadcast_message/1
function is used to publish the message event. When a message event is received, the handle_info/2
function is called, which simply prints the message to the console.
To subscribe to the message event, we can use the Phoenix.PubSub.subscribe/2
function. Here's an example of how to subscribe to the message event and receive messages:
{:ok, _} = ChatPubSub.start_link() Phoenix.PubSub.subscribe(ChatPubSub, :message)
This example demonstrates how event-driven programming can be used in Elixir to enable asynchronous communication and decoupling between different parts of the system. By publishing events and subscribing to them, we can build real-time and reactive applications.
Using PubSub in Phoenix for Event Communication
The PubSub module in Phoenix provides a useful mechanism for event communication between different parts of the system. It allows you to publish and subscribe to events, enabling loose coupling and asynchronous communication.
To use the PubSub module in Phoenix, you will need to start a PubSub server and then use the broadcast/3
and subscribe/3
functions to publish and subscribe to events, respectively.
Here's an example of how to use the PubSub module in Phoenix:
defmodule MyApp.PubSub do use Phoenix.PubSub def start_link do Phoenix.PubSub.start_link(__MODULE__, []) end end
In this example, we define a MyApp.PubSub
module that uses the Phoenix.PubSub
behavior. We also provide a start_link/0
function to start the PubSub server.
To publish an event, we can use the broadcast/3
function. Here's an example:
Phoenix.PubSub.broadcast(MyApp.PubSub, :my_event, %{data: "Hello, world!"})
In this example, we broadcast the :my_event
event with some data.
To subscribe to an event, we can use the subscribe/3
function. Here's an example:
Phoenix.PubSub.subscribe(MyApp.PubSub, :my_event)
In this example, we subscribe to the :my_event
event.
When an event is published, all subscribed processes will receive the event and can react to it accordingly. You can define event handlers using the handle_info/3
callback in your processes to handle the received events.
The PubSub module in Phoenix provides a flexible and scalable way of implementing event-driven communication in your applications. It allows for loose coupling and asynchronous communication, enabling you to build reactive and real-time systems.
Leveraging Concurrency in Elixir
Elixir is built on the Erlang virtual machine (BEAM), which is known for its lightweight processes and built-in concurrency primitives. Elixir provides a useful set of tools for leveraging concurrency and building highly concurrent and fault-tolerant systems.
To leverage concurrency in Elixir, you can use processes, message passing, and supervisors.
Processes
Processes in Elixir are lightweight and isolated units of execution. They are not operating system processes, but rather lightweight threads of execution managed by the BEAM. Elixir provides a simple syntax for spawning processes using the spawn/1
function. Here's an example:
pid = spawn(fn -> IO.puts("Hello, world!") end)
In this example, we spawn a new process that prints "Hello, world!".
Message Passing
Processes in Elixir communicate with each other by sending and receiving messages. Elixir provides the send/2
and receive/1
functions for message passing. Here's an example:
pid = spawn(fn -> receive do {:message, message} -> IO.puts("Received message: #{message}") end end) send(pid, {:message, "Hello, world!"})
In this example, we spawn a new process that waits for a message. We then send a message to that process, which matches on the {:message, message}
pattern and prints the message.
Supervisors
Supervisors in Elixir are responsible for starting, stopping, and monitoring processes. They ensure that processes are restarted in the event of failures, providing fault tolerance and self-healing capabilities. Elixir provides the Supervisor
module for defining and managing supervisors. Here's an example:
defmodule MyApp.Supervisor do use Supervisor def start_link do Supervisor.start_link(__MODULE__, []) end def init(_) do children = [ worker(MyApp.Worker, []) ] supervise(children, strategy: :one_for_one) end end
In this example, we define a supervisor for a worker process. The supervisor ensures that the worker process is restarted if it fails.
Understanding Fault Tolerance in Elixir
Fault tolerance is a crucial aspect of building reliable and resilient software systems. In Elixir, fault tolerance is achieved through the use of lightweight processes, supervision trees, and built-in error handling mechanisms.
Elixir's lightweight processes are isolated units of execution that can fail independently without affecting other processes. When a process fails, it can be restarted by a supervisor, which is responsible for managing the lifecycle of processes.
Supervisors in Elixir are defined using the Supervisor
behavior and are responsible for starting, stopping, and monitoring processes. They ensure that processes are restarted in the event of failures, providing fault tolerance and self-healing capabilities.
Here's an example of how to define a supervisor in Elixir:
defmodule MyApp.Supervisor do use Supervisor def start_link do Supervisor.start_link(__MODULE__, []) end def init(_) do children = [ worker(MyApp.Worker, []) ] supervise(children, strategy: :one_for_one) end end
In this example, we define a supervisor for a worker process. The supervisor ensures that the worker process is restarted if it fails. The supervise/2
function is used to specify the child processes and the restart strategy.
Related Article: Exploring Phoenix: Umbrella Project Structures,Ecto & More
Message Passing in Elixir: How it Works
Message passing is a fundamental concept in Elixir and is used for communication between processes. Elixir provides the send/2
and receive/1
functions for message passing.
When a process sends a message using the send/2
function, the message is put in the mailbox of the receiving process. The receiving process can then use the receive/1
function to pattern match on the messages in its mailbox.
Here's an example of how message passing works in Elixir:
pid = spawn(fn -> receive do {:message, message} -> IO.puts("Received message: #{message}") end end) send(pid, {:message, "Hello, world!"})
In this example, we spawn a new process that waits for a message. We then send a message to that process using the send/2
function. The receiving process matches on the {:message, message}
pattern and prints the message.
Elixir's message passing mechanism is asynchronous, meaning that the sender does not wait for the recipient to receive the message. This allows for concurrent and asynchronous communication between processes.
It's important to note that message passing in Elixir is done by copying the message data, not by sharing memory. This means that Elixir processes communicate by sending copies of their data, which ensures isolation and avoids shared mutable state.
Additional Resources
- OTP in Elixir