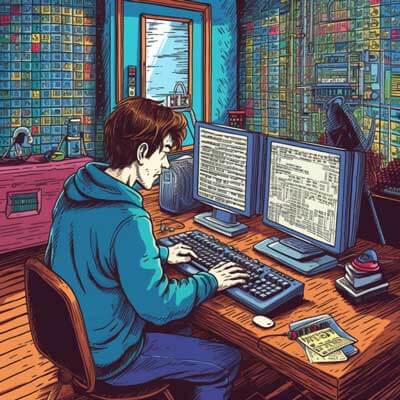
Table of Contents
Introduction to Date and Time in Programming
Date and time manipulation is a fundamental aspect of programming, as it allows us to work with temporal data and perform various operations such as formatting, comparison, and calculation. In PHP, the date and time functionality is provided by the built-in date
and time
functions, as well as other related functions and classes.
Related Article: Integrating Payment & Communication Features in Laravel
Example: Getting the Current Date and Time
To retrieve the current date and time in PHP, you can use the date
function with the appropriate format string. The format string specifies how the date and time should be formatted.
$currentDateTime = date('Y-m-d H:i:s'); echo $currentDateTime;
This will output the current date and time in the format YYYY-MM-DD HH:MM:SS
, such as 2022-01-01 14:30:45
.
Example: Calculating Future Dates
You can also perform calculations on dates and times using the strtotime
function along with the date
function. strtotime
allows you to parse a date or time string and convert it into a Unix timestamp, which is a numeric representation of the date and time.
$futureDate = strtotime('+1 week'); $formattedDate = date('Y-m-d', $futureDate); echo $formattedDate;
This code will calculate the date that is one week ahead of the current date and output it in the format YYYY-MM-DD
.
Establishing the Basics of Date and Time Functions
In this chapter, we will explore the basic functions for working with dates and times in PHP. These functions include date
, time
, strtotime
, mktime
, and more.
Related Article: PHP vs Python: How to Choose the Right Language
Example: Getting the Unix Timestamp
The time
function returns the current Unix timestamp, which represents the number of seconds that have elapsed since January 1, 1970 UTC.
$timestamp = time(); echo $timestamp;
This will output a numeric value representing the current timestamp.
Example: Converting a Timestamp to a Date
To convert a Unix timestamp to a human-readable date and time format, you can use the date
function along with the desired format string.
$timestamp = 1641023345; $formattedDate = date('Y-m-d H:i:s', $timestamp); echo $formattedDate;
This code will convert the given timestamp to the format YYYY-MM-DD HH:MM:SS
and output the formatted date and time.
Formatting Date and Time
Formatting date and time is often necessary to display them in a specific format that meets the requirements of your application or user preferences. In PHP, you can achieve this by using the date
function with a format string that specifies the desired format.
Example: Formatting Dates
To format a date, you can use various format characters that represent different components of the date, such as year, month, day, and more.
$date = '2022-01-01'; $formattedDate = date('F j, Y', strtotime($date)); echo $formattedDate;
This code will format the date as "January 1, 2022" and output the formatted date string.
Related Article: How to Use PHP Curl for HTTP Post
Example: Formatting Times
Formatting times involves specifying the desired format using format characters that represent different components of the time, such as hours, minutes, seconds, and more.
$time = '14:30:45'; $formattedTime = date('h:i A', strtotime($time)); echo $formattedTime;
This code will format the time as "02:30 PM" and output the formatted time string.
Working with Time in Seconds and Microseconds
In addition to working with dates and times in a human-readable format, PHP provides functions for dealing with time in seconds and microseconds. This can be useful for measuring elapsed time, calculating time differences, and performing other time-related operations.
Example: Calculating Time Difference
To calculate the difference between two points in time, you can subtract the timestamps representing those points and convert the result to the desired time unit.
$startTime = microtime(true); // Perform some time-consuming operation $endTime = microtime(true); $timeDiff = $endTime - $startTime; echo "Time taken: " . $timeDiff . " seconds";
This code measures the time taken to perform a time-consuming operation and outputs the result in seconds.
Example: Generating a Random Microsecond Value
If you need to generate a random microsecond value, you can use the microtime
function along with some additional calculations.
$microseconds = microtime(true); $randomMicroseconds = ($microseconds - floor($microseconds)) * 1000000; echo $randomMicroseconds;
This code generates a random microsecond value and outputs it.
Related Article: How to Work with PHP Arrays: a Complete Guide
Retrieving Current Date and Year
In many cases, you may need to retrieve the current date or specific components of the date, such as the year. PHP provides functions that allow you to easily retrieve the current date and extract specific components from it.
Example: Getting the Current Date
To retrieve the current date, you can use the date
function with the appropriate format string.
$currentDate = date('Y-m-d'); echo $currentDate;
This code will output the current date in the format YYYY-MM-DD
.
Example: Getting the Current Year
To retrieve the current year, you can use the date
function with the format character for the year.
$currentYear = date('Y'); echo $currentYear;
This code will output the current year as a four-digit number.
Manipulating Time and Date with strtotime
The strtotime
function in PHP allows you to parse a textual representation of a date or time and convert it into a Unix timestamp. This makes it easy to perform various operations on dates and times, such as adding or subtracting time intervals, finding specific dates, and more.
Related Article: How to Fix the 404 Not Found Error in Errordocument in PHP
Example: Adding Days to a Date
To add a specific number of days to a given date, you can use the strtotime
function along with the appropriate syntax.
$date = '2022-01-01'; $modifiedDate = strtotime($date . ' +1 week'); $formattedDate = date('Y-m-d', $modifiedDate); echo $formattedDate;
This code adds one week to the given date and outputs the modified date.
Example: Finding the Next Occurrence of a Day
To find the next occurrence of a specific day (e.g., Saturday) from a given date, you can use the strtotime
function along with the appropriate syntax.
$date = '2022-01-01'; $nextSaturday = strtotime('next Saturday', strtotime($date)); $formattedDate = date('Y-m-d', $nextSaturday); echo $formattedDate;
This code finds the next Saturday from the given date and outputs the resulting date.
Introduction to Timezones and Their Role
Timezones play a crucial role in accurately representing and converting dates and times across different regions of the world. Understanding timezones is essential to ensure correct handling of temporal data in global applications.
Example: Retrieving the Current Timezone
To retrieve the current timezone set in PHP, you can use the date_default_timezone_get
function.
$timezone = date_default_timezone_get(); echo $timezone;
This code will output the name of the current timezone.
Related Article: How To Convert A Php Object To An Associative Array
Example: Listing Available Timezones
To list all available timezones in PHP, you can use the timezone_identifiers_list
function.
$timezones = timezone_identifiers_list(); foreach ($timezones as $timezone) { echo $timezone . "<br>"; }
This code will output a list of all available timezones.
Adjusting Time Zones for Your Application
In many cases, you may need to adjust the timezone used by your application to match the timezone of your users or a specific region. PHP provides functions that allow you to easily change the default timezone and convert dates and times between different timezones.
Example: Changing the Default Timezone
To change the default timezone used by PHP, you can use the date_default_timezone_set
function.
date_default_timezone_set('America/New_York');
This code sets the default timezone to "America/New_York".
Example: Converting Timezones
To convert a date or time from one timezone to another, you can use the DateTime
class along with the setTimezone
method.
$date = new DateTime('2022-01-01', new DateTimeZone('America/New_York')); $date->setTimezone(new DateTimeZone('Europe/London')); $formattedDate = $date->format('Y-m-d H:i:s'); echo $formattedDate;
This code converts the given date from the "America/New_York" timezone to the "Europe/London" timezone and outputs the resulting date in the format YYYY-MM-DD HH:MM:SS
.
Related Article: How to Use Laravel Folio: Tutorial with Advanced Examples
Use Case: Displaying Current Date and Time
A common use case in web applications is to display the current date and time to the user. This provides a real-time reference and enhances the user experience.
Example: Displaying Current Date and Time
To display the current date and time in a web page, you can use PHP along with HTML.
$currentDateTime = date('Y-m-d H:i:s'); echo "Current date and time: " . $currentDateTime;
This code will output the current date and time in the format YYYY-MM-DD HH:MM:SS
along with the text "Current date and time: ".
Example: Automatically Updating the Displayed Time
To automatically update the displayed time without refreshing the entire page, you can use JavaScript along with PHP and HTML.
<p id="currentDateTime"></p> function updateDateTime() { var currentDateTime = new Date().toLocaleString(); document.getElementById("currentDateTime").innerHTML = "Current date and time: " + currentDateTime; } setInterval(updateDateTime, 1000);
This code displays the current date and time in a paragraph element and updates it every second using JavaScript.
Use Case: Scheduling Tasks Based on Date and Time
Scheduling tasks based on specific dates and times is a common requirement in many applications. PHP provides functions and techniques that allow you to easily implement task scheduling based on temporal conditions.
Related Article: How to Echo or Print an Array in PHP
Example: Sending Email Reminders
To send email reminders to users based on a specific date and time, you can use PHP along with a cron job or a task scheduler.
$reminderDate = '2022-01-01'; $reminderTime = '09:00:00'; $reminderDateTime = strtotime($reminderDate . ' ' . $reminderTime); $currentDateTime = time(); if ($currentDateTime >= $reminderDateTime) { // Send the reminder email echo "Reminder sent!"; }
This code checks if the current date and time have passed the specified reminder date and time, and if so, sends the reminder email.
Example: Running Scheduled Tasks at Regular Intervals
To run scheduled tasks at regular intervals, you can use a task scheduler or a cron job along with PHP.
// This code runs every hour if (date('i') == '00') { // Run the scheduled task echo "Task executed!"; }
This code checks if the current minute is zero (indicating the start of a new hour) and if so, executes the scheduled task.
Use Case: Timezone Conversion for Global Applications
When developing global applications that cater to users in different timezones, it is essential to handle timezone conversions correctly. PHP provides functions and techniques to ensure accurate timezone conversions and display the correct time to users based on their location.
Example: Displaying Local Time for Users
To display the local time for users based on their timezone, you can use PHP along with the DateTime
class and the user's timezone information.
// Assume the user's timezone is retrieved from the user's preferences or location $userTimezone = 'Asia/Kolkata'; $currentTime = new DateTime('now', new DateTimeZone($userTimezone)); $formattedTime = $currentTime->format('Y-m-d H:i:s'); echo "Local time: " . $formattedTime;
This code retrieves the user's timezone information and displays the current local time for the user.
Related Article: Tutorial: Building a Laravel 9 Real Estate Listing App
Example: Converting Timezone for User Input
When accepting user input for dates or times, it is important to convert the input to a consistent timezone before storing or processing it. This ensures that the input is accurately represented and can be compared or manipulated correctly.
// Assume the user input is in the user's timezone $userTimezone = 'America/New_York'; $userInput = '2022-01-01 14:30:45'; $userDateTime = new DateTime($userInput, new DateTimeZone($userTimezone)); $userDateTime->setTimezone(new DateTimeZone('UTC')); $convertedDateTime = $userDateTime->format('Y-m-d H:i:s'); echo "Converted date and time: " . $convertedDateTime;
This code converts the user input from the user's timezone to UTC (Coordinated Universal Time) and outputs the converted date and time.
Code Snippet: Format Date and Time
To format a date and time in PHP, you can use the date
function with the appropriate format string.
$date = '2022-01-01'; $time = '14:30:45'; $formattedDateTime = date('F j, Y h:i A', strtotime($date . ' ' . $time)); echo $formattedDateTime;
This code formats the given date and time as "January 1, 2022 02:30 PM" and outputs the formatted date and time string.
Code Snippet: Get Current Date and Year
To retrieve the current date and year in PHP, you can use the date
function with the appropriate format characters.
$currentDate = date('Y-m-d'); $currentYear = date('Y'); echo "Current date: " . $currentDate . "<br>"; echo "Current year: " . $currentYear;
This code retrieves the current date and year and outputs them.
Code Snippet: Calculate Time Difference
To calculate the time difference between two points in time, you can subtract the timestamps representing those points and convert the result to the desired time unit.
$startTime = microtime(true); // Perform some time-consuming operation $endTime = microtime(true); $timeDiff = $endTime - $startTime; echo "Time taken: " . $timeDiff . " seconds";
This code measures the time taken to perform a time-consuming operation and outputs the result in seconds.
Related Article: How To Make A Redirect In PHP
Code Snippet: Convert String to Date Using strtotime
To convert a string representation of a date or time to a Unix timestamp, you can use the strtotime
function.
$dateString = 'January 1, 2022'; $timestamp = strtotime($dateString); echo $timestamp;
This code converts the given date string to a Unix timestamp and outputs the result.
Code Snippet: Set and Get Timezone
To set and retrieve the timezone in PHP, you can use the date_default_timezone_set
and date_default_timezone_get
functions, respectively.
// Set the timezone date_default_timezone_set('America/New_York'); // Get the timezone $timezone = date_default_timezone_get(); echo $timezone;
This code sets the default timezone to "America/New_York" and retrieves the current timezone.
Best Practice: Validate and Sanitize Date Input
When accepting and processing date input from users, it is important to validate and sanitize the input to ensure its correctness and prevent potential security vulnerabilities.
Example: Validating Date Input
To validate a date input, you can use the DateTime
class and its createFromFormat
method along with appropriate error handling.
$dateString = $_POST['date']; try { $date = DateTime::createFromFormat('Y-m-d', $dateString); if ($date === false) { throw new Exception('Invalid date format'); } } catch (Exception $e) { echo "Error: " . $e->getMessage(); }
This code validates a date input in the format YYYY-MM-DD
and throws an exception if the input is invalid.
Related Article: How to Use Loops in PHP
Example: Sanitizing Date Input
To sanitize a date input, you can use PHP's filter_var
function with the FILTER_SANITIZE_STRING
filter.
$dateString = $_POST['date']; $sanitizedDate = filter_var($dateString, FILTER_SANITIZE_STRING); echo "Sanitized date: " . $sanitizedDate;
This code sanitizes a date input by removing any potentially harmful characters and outputs the sanitized date.
Best Practice: Handle Timezone Differences
When dealing with timezones in global applications, it is important to handle timezone differences correctly to ensure accurate representation and conversion of dates and times.
Example: Storing and Displaying Dates in UTC
A common practice is to store dates and times in UTC (Coordinated Universal Time) and convert them to the user's local timezone when displaying them.
// Store the date and time in UTC $userDateTime = new DateTime('2022-01-01 14:30:45', new DateTimeZone('UTC')); $storedDateTime = $userDateTime->format('Y-m-d H:i:s'); // Display the date and time in the user's local timezone $userTimezone = 'America/New_York'; $userDateTime->setTimezone(new DateTimeZone($userTimezone)); $formattedDateTime = $userDateTime->format('F j, Y h:i A'); echo "Stored date and time: " . $storedDateTime . "<br>"; echo "Displayed date and time: " . $formattedDateTime;
This code stores the date and time in UTC and converts it to the user's local timezone when displaying it.
Best Practice: Avoid Date and Time Manipulation Errors
When working with dates and times, it is important to avoid common manipulation errors that can lead to incorrect results. This includes handling daylight saving time changes, leap years, and other edge cases.
Related Article: How To Check If A PHP String Contains A Specific Word
Example: Handling Daylight Saving Time Changes
To handle daylight saving time changes correctly, you can use the DateTime
class along with the setTimezone
method.
$date = new DateTime('2022-03-13 02:30:00', new DateTimeZone('America/New_York')); $date->setTimezone(new DateTimeZone('UTC')); $formattedDate = $date->format('Y-m-d H:i:s'); echo $formattedDate;
This code handles the daylight saving time change that occurs on March 13, 2022, in the "America/New_York" timezone and outputs the converted date and time in UTC.
Handling Errors in Date and Time Functions
When working with date and time functions, it is important to handle potential errors and exceptions that may occur. This ensures the reliability and robustness of your code.
Example: Handling Invalid Date Formats
To handle invalid date formats gracefully, you can use appropriate error handling techniques, such as try-catch blocks.
$dateString = '2022-01-01'; try { $date = new DateTime($dateString); } catch (Exception $e) { echo "Error: " . $e->getMessage(); }
This code attempts to create a DateTime
object from a date string and catches any exceptions that may occur during the process.
Performance Consideration: Efficient Date and Time Formatting
When formatting dates and times, it is important to consider the performance implications, especially when dealing with large amounts of data or frequently updating timestamps.
Related Article: How to Work with Arrays in PHP (with Advanced Examples)
Example: Caching Formatted Dates
To improve performance when formatting dates that are frequently used, you can cache the formatted dates and reuse them instead of reformatting them every time.
$timestamp = time(); $formattedDate = date('Y-m-d', $timestamp); // Cache the formatted date cache_set('formatted_date', $formattedDate); // Retrieve the cached formatted date $cachedFormattedDate = cache_get('formatted_date'); echo $cachedFormattedDate;
This code caches the formatted date and retrieves it from the cache instead of reformatting it every time.
Performance Consideration: Timezone Setting and Conversion Efficiency
When working with timezones, it is important to consider the performance impact of setting and converting timezones, especially when dealing with large amounts of data or frequent conversions.
Example: Caching Timezone Conversions
To improve performance when converting dates and times between timezones that are frequently used, you can cache the converted dates and reuse them instead of converting them every time.
$userTimezone = 'America/New_York'; $storedDateTime = '2022-01-01 14:30:45'; // Convert the stored date and time to the user's timezone $cachedConvertedDateTime = cache_get('converted_date_time'); if ($cachedConvertedDateTime === null) { $storedDateTimeObject = new DateTime($storedDateTime, new DateTimeZone('UTC')); $userDateTimeObject = clone $storedDateTimeObject; $userDateTimeObject->setTimezone(new DateTimeZone($userTimezone)); $formattedDateTime = $userDateTimeObject->format('Y-m-d H:i:s'); // Cache the converted date and time cache_set('converted_date_time', $formattedDateTime); $cachedConvertedDateTime = $formattedDateTime; } echo $cachedConvertedDateTime;
This code caches the converted date and time and retrieves it from the cache instead of converting it every time.
Advanced Technique: Create Custom Date and Time Functions
In addition to the built-in date and time functions, PHP allows you to create custom functions to encapsulate complex or frequently used date and time operations.
Related Article: Processing MySQL Queries in PHP: A Detailed Guide
Example: Custom Function to Calculate Age
To calculate a person's age based on their birthdate, you can create a custom function that encapsulates the necessary calculations.
function calculateAge($birthdate) { $today = new DateTime(); $birthDate = new DateTime($birthdate); $age = $today->diff($birthDate)->y; return $age; } $birthdate = '1990-01-01'; $age = calculateAge($birthdate); echo "Age: " . $age;
This code defines a custom function calculateAge
that calculates a person's age based on their birthdate and outputs the result.
Advanced Technique: Leverage Built-in Functions for Complex Date and Time Operations
In addition to custom functions, PHP provides built-in functions that can be leveraged to perform complex date and time operations efficiently.
Example: Calculating the Number of Days Between Two Dates
To calculate the number of days between two dates, you can use the DateTime
class along with the diff
method.
$startDate = new DateTime('2022-01-01'); $endDate = new DateTime('2022-12-31'); $interval = $startDate->diff($endDate); $numberOfDays = $interval->days; echo "Number of days: " . $numberOfDays;
This code calculates the number of days between the start and end dates and outputs the result.
Real World Example: Building a Countdown Timer
A countdown timer is a common feature in various applications, such as event management systems, online sales, and more. PHP can be used to build a countdown timer that dynamically displays the remaining time until a specified date and time.
Related Article: How to Use the PHP Random String Generator
Example: Building a Countdown Timer
To build a countdown timer in PHP, you can use JavaScript along with PHP to calculate and update the remaining time dynamically.
<p id="countdown"></p> function updateCountdown() { var targetDate = new Date('2022-12-31 23:59:59'); var currentDate = new Date(); var remainingTime = targetDate - currentDate; // Calculate remaining days, hours, minutes, and seconds var days = Math.floor(remainingTime / (1000 * 60 * 60 * 24)); var hours = Math.floor((remainingTime % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60)); var minutes = Math.floor((remainingTime % (1000 * 60 * 60)) / (1000 * 60)); var seconds = Math.floor((remainingTime % (1000 * 60)) / 1000); // Display the remaining time document.getElementById("countdown").innerHTML = "Countdown: " + days + " days, " + hours + " hours, " + minutes + " minutes, " + seconds + " seconds"; } setInterval(updateCountdown, 1000);
This code builds a countdown timer that displays the remaining time until December 31, 2022, 23:59:59. The timer updates every second using JavaScript.
Real World Example: Creating a World Clock Application
A world clock application allows users to view the current time in various cities or timezones around the world. PHP can be used to build a world clock application that retrieves and displays the current time in different timezones.
Example: Creating a World Clock Application
To create a world clock application in PHP, you can use PHP along with JavaScript to retrieve and display the current time in different timezones.
New York London Tokyo <p id="currentTime"></p> function updateTime() { var selectedTimezone = document.getElementById("timezoneSelect").value; // Retrieve the current time in the selected timezone var xmlhttp = new XMLHttpRequest(); xmlhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { var currentTime = this.responseText; document.getElementById("currentTime").innerHTML = "Current time: " + currentTime; } }; xmlhttp.open("GET", "get_time.php?timezone=" + selectedTimezone, true); xmlhttp.send(); } // Update the time when the page loads updateTime();
This code creates a dropdown list of timezones and retrieves the current time in the selected timezone using an AJAX request to a PHP script (get_time.php
). The current time is then displayed on the page.
The get_time.php
script would handle the server-side logic to retrieve the current time in the specified timezone and return it as the response to the AJAX request.
Real World Example: Implementing a Scheduling System
A scheduling system allows users to schedule and manage events, appointments, and other time-based activities. PHP can be used to implement a scheduling system that handles date and time-related operations, such as creating, updating, and displaying scheduled events.
Related Article: How to Delete An Element From An Array In PHP
Example: Implementing a Scheduling System
To implement a scheduling system in PHP, you can use PHP along with a database to store and manage scheduled events.
// Assume a database connection is established // Create a scheduled event $eventDate = '2022-01-01'; $eventTime = '14:30:00'; $eventName = 'Meeting'; $query = "INSERT INTO events (date, time, name) VALUES ('$eventDate', '$eventTime', '$eventName')"; $result = mysqli_query($connection, $query); if ($result) { echo "Event created successfully!"; } else { echo "Error creating event: " . mysqli_error($connection); } // Retrieve and display scheduled events $query = "SELECT * FROM events"; $result = mysqli_query($connection, $query); if (mysqli_num_rows($result) > 0) { while ($row = mysqli_fetch_assoc($result)) { $eventDate = $row['date']; $eventTime = $row['time']; $eventName = $row['name']; echo "Event: " . $eventName . "<br>"; echo "Date: " . $eventDate . "<br>"; echo "Time: " . $eventTime . "<br><br>"; } } else { echo "No events found."; }
This code demonstrates the creation and retrieval of scheduled events. It creates an event with a specified date, time, and name, and then retrieves and displays all the scheduled events.
Note: The database connection and query execution code may vary depending on the database system being used.