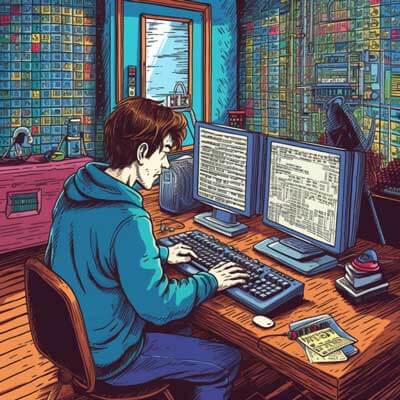
Table of Contents
Introduction to the Comparison
PHP and JavaScript are two popular programming languages used in web development. While PHP is primarily a server-side language, JavaScript is mainly used for client-side scripting. In this article, we will compare and contrast the features, capabilities, and use cases of PHP and JavaScript to help you understand the strengths and weaknesses of each language.
Related Article: How to Work with Arrays in PHP (with Advanced Examples)
Ecosystem Analysis
The PHP ecosystem is mature and extensive, with a wide range of frameworks, libraries, and tools available. One of the most popular frameworks is Laravel, which provides a robust and elegant way to build web applications. Here is an example of connecting to a MySQL database using PHP:
connect_error) { die("Connection failed: " . $conn->connect_error); } echo "Connected successfully"; ?>
On the other hand, JavaScript has a vibrant and rapidly evolving ecosystem. Node.js allows JavaScript to be used on the server-side, enabling full-stack JavaScript development. Express.js is a popular framework for building web applications with Node.js. Here is an example of a basic server setup using Express.js:
const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello World!'); }); app.listen(port, () => { console.log(`Server listening at http://localhost:${port}`); });
Web Development Contrast
PHP and JavaScript have different approaches to web development. PHP is a server-side language, meaning that the code is executed on the server before being sent to the client. This allows PHP to handle server-side tasks such as interacting with databases and processing form data. JavaScript, on the other hand, is primarily a client-side language, running in the browser. It is responsible for enhancing the user interface and handling client-side interactions.
PHP Example: User Authentication
Authentication is a critical aspect of web development. Here is an example of user authentication using PHP:
Related Article: Tutorial: Building a Laravel 9 Real Estate Listing App
JavaScript Example: User Authentication
JavaScript can also handle user authentication on the client-side. Here is an example using JavaScript and JSON Web Tokens (JWT):
// Login API endpoint app.post('/login', (req, res) => { const { username, password } = req.body; // Database query to check username and password if (username === "admin" && password === "password") { const token = jwt.sign({ username }, 'secret_key'); res.json({ token }); } else { res.status(401).json({ error: 'Invalid credentials' }); } }); // Protected route app.get('/protected', authenticateToken, (req, res) => { res.send('Protected data'); }); function authenticateToken(req, res, next) { const token = req.headers['authorization']; if (token == null) { return res.sendStatus(401); } jwt.verify(token, 'secret_key', (err, user) => { if (err) { return res.sendStatus(403); } req.user = user; next(); }); }
Active Libraries in Focus
Both PHP and JavaScript have a vast collection of libraries and frameworks that enhance development productivity. These libraries provide additional functionality and simplify common tasks.
PHP Library: Symfony
Symfony is a popular PHP framework that follows the Model-View-Controller (MVC) architectural pattern. It provides a set of reusable components and tools for building web applications. Here is an example of routing in Symfony:
// routes.yaml home: path: / controller: App\Controller\HomeController::index // HomeController.php <?php namespace App\Controller; use Symfony\Component\HttpFoundation\Response; class HomeController { public function index() { return new Response('Hello World!'); } }
JavaScript Library: React
React is a widely used JavaScript library for building user interfaces. It allows developers to create reusable UI components and efficiently update the UI based on changes in data. Here is an example of a basic React component:
import React from 'react'; function App() { return ( <div> <h1>Hello World!</h1> </div> ); } export default App;
Related Article: How to Use the Now Function in PHP
Productivity Assessment
When comparing PHP and JavaScript in terms of productivity, several factors come into play, including the ease of development, availability of tools and libraries, and the efficiency of the development workflow.
PHP Example: Data Validation
Data validation is an essential aspect of web development. Here is an example of data validation using PHP:
0) { foreach ($errors as $error) { echo $error . '<br>'; } } else { // Process data } ?>
JavaScript Example: Data Validation
JavaScript also provides built-in functions and libraries for data validation. Here is an example using the validator.js library:
const validator = require('validator'); const name = req.body.name; const email = req.body.email; const errors = []; if (validator.isEmpty(name)) { errors.push('Name is required'); } if (!validator.isEmail(email)) { errors.push('Invalid email address'); } if (errors.length > 0) { res.status(400).json({ errors }); } else { // Process data }
Performance Examination
Performance is a critical consideration in web development. Both PHP and JavaScript have their own performance characteristics and optimizations.
Related Article: How To Check If A PHP String Contains A Specific Word
PHP Example: Database Queries
Efficient database queries can significantly impact the performance of a web application. Here is an example of executing a prepared statement in PHP:
prepare("SELECT * FROM users WHERE id = ?"); $stmt->bind_param("i", $id); $stmt->execute(); $result = $stmt->get_result(); while ($row = $result->fetch_assoc()) { echo $row['name']; } $stmt->close(); ?>
JavaScript Example: Database Queries
In JavaScript, database queries can be performed using libraries such as Sequelize for SQL databases or MongoDB for NoSQL databases. Here is an example using Sequelize:
const User = require('../models/user'); User.findOne({ where: { id: 1 } }).then(user => { console.log(user.name); });
Third-Party Tools Overview
Both PHP and JavaScript have a wide range of third-party tools and services that can be integrated into web applications to enhance functionality and improve development workflow.
PHP Example: Mailgun
Mailgun is a popular email delivery service that provides APIs for sending and receiving emails. Here is an example of sending an email using the Mailgun PHP SDK:
messages()->send('YOUR_DOMAIN', [ 'from' => 'noreply@example.com', 'to' => 'recipient@example.com', 'subject' => 'Hello', 'text' => 'This is a test email' ]); ?>
Related Article: How to Fix Error 419 Page Expired in Laravel Post Request
JavaScript Example: Stripe
Stripe is a popular payment processing platform that provides APIs for accepting payments online. Here is an example of processing a payment using the Stripe JavaScript SDK:
const stripe = require('stripe')('YOUR_SECRET_KEY'); stripe.charges.create({ amount: 2000, currency: 'usd', source: 'tok_visa', description: 'Example charge' }).then(charge => { console.log(charge); });
Security Considerations
When it comes to web development, security is of utmost importance. Both PHP and JavaScript have their own security considerations and best practices.
PHP Example: User Input Sanitization
Sanitizing user input is crucial to prevent security vulnerabilities such as SQL injection and cross-site scripting (XSS) attacks. Here is an example of sanitizing user input in PHP:
JavaScript Example: Cross-Site Scripting Prevention
JavaScript also provides functions to prevent cross-site scripting (XSS) attacks. Here is an example:
const name = req.body.name; const email = req.body.email; const sanitizedName = DOMPurify.sanitize(name); const sanitizedEmail = DOMPurify.sanitize(email);
Related Article: PHP vs Java: A Practical Comparison
Scalability Comparison
Scalability is a crucial factor to consider when building web applications. Both PHP and JavaScript offer tools and techniques to scale applications efficiently.
PHP Example: Load Balancing
Load balancing distributes incoming requests across multiple servers to improve performance and handle higher traffic loads. Here is an example of load balancing using PHP and Nginx:
// Nginx configuration upstream backend { server backend1.example.com; server backend2.example.com; } server { listen 80; server_name example.com; location / { proxy_pass http://backend; } }
JavaScript Example: Horizontal Scaling
In JavaScript, horizontal scaling can be achieved using technologies like Docker and Kubernetes. Here is an example of deploying a Node.js application using Docker:
# Dockerfile FROM node:12 WORKDIR /app COPY package*.json ./ RUN npm install COPY . . EXPOSE 3000 CMD ["npm", "start"]
Use Case: E-commerce Platforms
E-commerce platforms have specific requirements and challenges. Let's explore how PHP and JavaScript can be used for building e-commerce platforms.
Related Article: How to Delete An Element From An Array In PHP
PHP Example: Shopping Cart Functionality
Building a shopping cart functionality is a common requirement in e-commerce platforms. Here is an example of managing a shopping cart using PHP:
JavaScript Example: Real-Time Inventory Updates
Real-time inventory updates are crucial in e-commerce platforms to prevent overselling. Here is an example of real-time inventory updates using JavaScript and WebSocket:
// Server-side code using Node.js and WebSocket const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080 }); wss.on('connection', ws => { ws.on('message', message => { // Update inventory in the database based on the received message // Send updated inventory to all connected clients wss.clients.forEach(client => { if (client.readyState === WebSocket.OPEN) { client.send(updatedInventory); } }); }); });
Best Practice: Code Organization
Code organization is essential for maintainability and readability. Let's explore best practices for organizing PHP and JavaScript code.
PHP Best Practice: MVC Architecture
The Model-View-Controller (MVC) architectural pattern is widely used in PHP web development. It separates the application logic into three components: the model, view, and controller. Here is an example of organizing code using the MVC pattern:
// Model class User { // User-related logic and database interactions } // View class UserView { // User-related HTML templates and rendering logic } // Controller class UserController { private $userModel; private $userView; public function __construct(User $userModel, UserView $userView) { $this->userModel = $userModel; $this->userView = $userView; } public function show($id) { $user = $this->userModel->getUserById($id); $this->userView->renderUser($user); } }
Related Article: How To Get The Full URL In PHP
JavaScript Best Practice: Modularization with ES Modules
ES Modules provide a standardized way to modularize JavaScript code. Modules encapsulate functionality and allow for better code organization. Here is an example of organizing JavaScript code using ES Modules:
// user.js export class User { // User-related logic and API interactions } // userView.js export class UserView { // User-related DOM manipulation and rendering logic } // userController.js import { User } from './user.js'; import { UserView } from './userView.js'; export class UserController { constructor() { this.userModel = new User(); this.userView = new UserView(); } show(id) { const user = this.userModel.getUserById(id); this.userView.renderUser(user); } }
Real World Example: Social Media Applications
Social media applications have unique requirements and challenges. Let's explore how PHP and JavaScript can be used to build social media applications.
PHP Example: User Follow System
Implementing a user follow system is a common requirement in social media applications. Here is an example of managing user follows using PHP and a relational database:
JavaScript Example: Real-Time Notifications
Real-time notifications play a crucial role in keeping users engaged in social media applications. Here is an example of implementing real-time notifications using JavaScript and WebSockets:
// Server-side code using Node.js and WebSocket const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080 }); wss.on('connection', ws => { ws.on('message', message => { const userId = getUserIdFromMessage(message); // Check for new notifications for the user in the database const notifications = getNotificationsForUser(userId); ws.send(JSON.stringify(notifications)); }); });
Related Article: PHP Date and Time Tutorial
Performance Consideration: Load Times
Load times significantly impact user experience and search engine rankings. Both PHP and JavaScript offer techniques to improve load times.
PHP Performance Consideration: Caching
Caching is a technique to store frequently accessed data in memory to improve performance. Here is an example of caching using PHP and the Memcached extension:
addServer('localhost', 11211); $key = 'cache_key'; $data = $memcached->get($key); if (!$data) { // Perform expensive database query or computation $data = fetchDataFromDatabase(); // Store the data in cache for future use $memcached->set($key, $data, 3600); } // Use the cached data echo $data; ?>
JavaScript Performance Consideration: Minification
Minification is the process of removing unnecessary characters from code to reduce file size and improve load times. Here is an example of minifying JavaScript using a tool like UglifyJS:
// Original JavaScript code function sum(a, b) { return a + b; } // Minified JavaScript code function sum(a,b){return a+b;}
Advanced Technique: Asynchronous Operations
Asynchronous operations are essential for handling tasks that require waiting for a response, such as making API calls or performing long-running operations. Both PHP and JavaScript provide mechanisms for handling asynchronous operations.
Related Article: How to Work with PHP Arrays: a Complete Guide
PHP Advanced Technique: Asynchronous I/O with ReactPHP
ReactPHP is a library for event-driven, non-blocking I/O operations in PHP. It allows for building asynchronous applications that can handle high concurrency. Here is an example of making an asynchronous HTTP request using ReactPHP:
request('GET', 'http://example.com'); $request->on('response', function (\React\Http\Message\Response $response) { $response->on('data', function ($chunk) { echo $chunk; }); }); $request->end(); $loop->run(); ?>
JavaScript Advanced Technique: Async/Await
Async/await is a modern JavaScript feature that simplifies handling asynchronous operations using promises. It allows for writing asynchronous code in a synchronous-like manner. Here is an example of making an asynchronous API call using async/await:
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } catch (error) { console.error(error); } } fetchData();
Code Snippet: Database Connection
Establishing a database connection is a fundamental aspect of web development. Here is an example of establishing a database connection using PHP and the PDO extension:
setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); echo "Connected successfully"; } catch(PDOException $e) { echo "Connection failed: " . $e->getMessage(); } ?>
Code Snippet: User Authentication
User authentication is a critical aspect of web applications. Here is an example of user authentication using JavaScript and JSON Web Tokens (JWT):
// Login API endpoint app.post('/login', (req, res) => { const { username, password } = req.body; // Database query to check username and password if (username === "admin" && password === "password") { const token = jwt.sign({ username }, 'secret_key'); res.json({ token }); } else { res.status(401).json({ error: 'Invalid credentials' }); } }); // Protected route app.get('/protected', authenticateToken, (req, res) => { res.send('Protected data'); }); function authenticateToken(req, res, next) { const token = req.headers['authorization']; if (token == null) { return res.sendStatus(401); } jwt.verify(token, 'secret_key', (err, user) => { if (err) { return res.sendStatus(403); } req.user = user; next(); }); }
Related Article: Preventing Redundant MySQL Queries in PHP Loops
Code Snippet: Data Validation
Data validation is crucial to ensure the integrity and security of web applications. Here is an example of data validation using PHP:
0) { foreach ($errors as $error) { echo $error . '<br>'; } } else { // Process data } ?>
Code Snippet: API Call
Making API calls is a common requirement in web development. Here is an example of making an API call using JavaScript and the fetch API:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error(error));
Code Snippet: Error Handling
Error handling is essential for providing meaningful error messages and handling exceptional conditions. Here is an example of error handling in PHP:
try { // Code that may throw an exception } catch (Exception $e) { echo 'An error occurred: ' . $e->getMessage(); }
Use Case: Content Management Systems
Content Management Systems (CMS) allow users to create, manage, and publish digital content. Let's explore how PHP and JavaScript can be used for building CMS.
Related Article: How to Display PHP Errors: A Step-by-Step Guide
PHP Example: Page Management
Managing pages is a core functionality of content management systems. Here is an example of managing pages using PHP and a relational database:
JavaScript Example: WYSIWYG Editor
WYSIWYG (What You See Is What You Get) editors allow users to create and edit content visually. Here is an example of integrating a WYSIWYG editor using JavaScript and a library like CKEditor:
<textarea id="editor"></textarea> ClassicEditor .create(document.querySelector('#editor')) .then(editor => { console.log('Editor was initialized', editor); }) .catch(error => { console.error(error); });
Best Practice: Testing and Debugging
Testing and debugging are essential for ensuring the quality and reliability of web applications. Let's explore best practices for testing and debugging PHP and JavaScript code.
PHP Best Practice: Unit Testing with PHPUnit
PHPUnit is a widely used testing framework for PHP applications. It allows for writing and executing unit tests to verify the correctness of individual units of code. Here is an example of writing a unit test using PHPUnit:
add(2, 3); $this->assertEquals(5, $result); } } ?>
Related Article: How to Use Laravel Folio: Tutorial with Advanced Examples
JavaScript Best Practice: Testing with Jest
Jest is a popular testing framework for JavaScript applications. It provides a simple and intuitive API for writing and executing tests. Here is an example of writing a test using Jest:
test('add() function adds two numbers correctly', () => { const calculator = new Calculator(); const result = calculator.add(2, 3); expect(result).toBe(5); });
Real World Example: News Websites
News websites have unique requirements, such as displaying real-time updates and managing content. Let's explore how PHP and JavaScript can be used for building news websites.
PHP Example: Article Management
Managing articles is a core functionality of news websites. Here is an example of managing articles using PHP and a relational database:
JavaScript Example: Real-Time Updates
Real-time updates are crucial in news websites to display breaking news and live updates. Here is an example of implementing real-time updates using JavaScript and WebSockets:
// Server-side code using Node.js and WebSocket const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080 }); wss.on('connection', ws => { ws.on('message', message => { // Check for new articles in the database const articles = getNewArticles(); ws.send(JSON.stringify(articles)); }); });
Related Article: How To Convert A String To A Number In PHP
Performance Consideration: Multithreading
Multithreading allows for handling multiple tasks concurrently, improving performance and responsiveness. Let's explore multithreading in PHP and JavaScript.
PHP Performance Consideration: Asynchronous PHP with Swoole
Swoole is an event-driven, high-performance PHP extension that provides support for asynchronous programming and multithreading. Here is an example of using Swoole to handle multiple requests concurrently:
on('Request', function ($request, $response) { $response->header("Content-Type", "text/plain"); $response->end("Hello World\n"); }); $server->start(); ?>
JavaScript Performance Consideration: Web Workers
Web Workers allow JavaScript code to run in the background, separate from the main execution thread. They enable concurrent processing and offload CPU-intensive tasks. Here is an example of using a web worker in JavaScript:
// main.js const worker = new Worker('worker.js'); worker.onmessage = function(event) { console.log('Received message from worker:', event.data); }; worker.postMessage('Hello from main.js'); // worker.js self.onmessage = function(event) { console.log('Received message from main.js:', event.data); // Perform heavy computations self.postMessage('Finished computations'); };
Advanced Technique: Caching Strategies
Caching strategies optimize performance by storing frequently accessed data for faster retrieval. Let's explore caching strategies in PHP and JavaScript.
Related Article: How to Fix the 404 Not Found Error in Errordocument in PHP
PHP Advanced Technique: Redis for Caching
Redis is an in-memory data structure store that can be used as a caching solution. It provides high-performance caching and supports various data structures. Here is an example of caching using Redis in PHP:
connect('127.0.0.1', 6379); $key = 'cache_key'; $data = $redis->get($key); if (!$data) { // Perform expensive database query or computation $data = fetchDataFromDatabase(); // Store the data in cache for future use $redis->set($key, $data); $redis->expire($key, 3600); } // Use the cached data echo $data; ?>
JavaScript Advanced Technique: Service Workers
Service workers are a JavaScript feature that enables caching and offline functionality in web applications. They allow for intercepting and handling network requests, enabling caching strategies. Here is an example of caching using a service worker in JavaScript:
// service-worker.js self.addEventListener('install', event => { event.waitUntil( caches.open('my-cache').then(cache => { return cache.addAll([ '/', '/styles.css', '/script.js', '/image.jpg' ]); }) ); }); self.addEventListener('fetch', event => { event.respondWith( caches.match(event.request).then(response => { return response || fetch(event.request); }) ); });
Error Handling: Common Issues and Solutions
Error handling is crucial for diagnosing and resolving issues in web applications. Let's explore common issues and solutions for error handling in PHP and JavaScript.
PHP Error Handling: Exception Handling
Exception handling allows for catching and handling errors in PHP. Here is an example of exception handling in PHP:
try { // Code that may throw an exception } catch (Exception $e) { echo 'An error occurred: ' . $e->getMessage(); }
Related Article: PHP vs Python: How to Choose the Right Language
JavaScript Error Handling: Try/Catch
Try/catch blocks can be used to catch and handle errors in JavaScript. Here is an example of try/catch error handling in JavaScript:
try { // Code that may throw an error } catch (error) { console.error('An error occurred:', error); }
Use Case: Real-Time Applications
Real-time applications require handling real-time data updates and providing instant feedback to users. Let's explore how PHP and JavaScript can be used for building real-time applications.
PHP Example: Chat Application
Building a chat application is a common use case for real-time applications. Here is an example of implementing real-time chat functionality using PHP and WebSocket:
// Server-side code using PHP and Ratchet WebSocket library use Ratchet\MessageComponentInterface; use Ratchet\ConnectionInterface; class Chat implements MessageComponentInterface { protected $clients; public function __construct() { $this->clients = new \SplObjectStorage; } public function onOpen(ConnectionInterface $conn) { $this->clients->attach($conn); echo "New connection: {$conn->resourceId}\n"; } public function onMessage(ConnectionInterface $from, $msg) { foreach ($this->clients as $client) { if ($client !== $from) { $client->send($msg); } } } public function onClose(ConnectionInterface $conn) { $this->clients->detach($conn); echo "Connection closed: {$conn->resourceId}\n"; } public function onError(ConnectionInterface $conn, \Exception $e) { echo "An error occurred: {$e->getMessage()}\n"; $conn->close(); } } $server = IoServer::factory( new HttpServer( new WsServer( new Chat() ) ), 8080 ); $server->run();
JavaScript Example: Real-Time Dashboard
Building a real-time dashboard is another use case for real-time applications. Here is an example of implementing a real-time dashboard using JavaScript and Socket.IO:
// Server-side code using Node.js and Socket.IO library const app = require('express')(); const http = require('http').createServer(app); const io = require('socket.io')(http); io.on('connection', socket => { console.log('New connection:', socket.id); socket.on('data', data => { // Process and broadcast the data to connected clients io.emit('data', data); }); socket.on('disconnect', () => { console.log('Connection closed:', socket.id); }); }); http.listen(8080, () => { console.log('Server listening on port 8080'); });
Related Article: Optimizing Laravel Apps: Deployment and Scaling Techniques
Best Practice: Security Measures
Implementing security measures is crucial for protecting web applications from vulnerabilities and attacks. Let's explore best practices for security in PHP and JavaScript.
PHP Best Practice: Prepared Statements
Prepared statements protect against SQL injection attacks by separating SQL code from user-supplied input. Here is an example of using prepared statements in PHP:
$stmt = $conn->prepare("SELECT * FROM users WHERE username = ?"); $stmt->bind_param("s", $username); $stmt->execute(); $result = $stmt->get_result(); while ($row = $result->fetch_assoc()) { echo $row['name']; } $stmt->close();
JavaScript Best Practice: Input Sanitization
Sanitizing user input is crucial to prevent security vulnerabilities such as cross-site scripting (XSS) attacks. Here is an example of input sanitization in JavaScript using a library like DOMPurify:
const name = req.body.name; const email = req.body.email; const sanitizedName = DOMPurify.sanitize(name); const sanitizedEmail = DOMPurify.sanitize(email);
Real World Example: Online Marketplaces
Online marketplaces have unique requirements and challenges, such as user authentication and product listings. Let's explore how PHP and JavaScript can be used for building online marketplaces.
Related Article: How To Convert A Php Object To An Associative Array
PHP Example: Product Listing
Listing products is a core functionality of online marketplaces. Here is an example of managing product listings using PHP and a relational database:
JavaScript Example: Shopping Cart
Implementing a shopping cart functionality is crucial for online marketplaces. Here is an example of managing a shopping cart using JavaScript:
class ShoppingCart { constructor() { this.items = []; } addItem(product, quantity) { this.items.push({ product, quantity }); } removeItem(productId) { this.items = this.items.filter(item => item.product.id !== productId); } getTotalPrice() { return this.items.reduce((total, item) => total + (item.product.price * item.quantity), 0); } } const shoppingCart = new ShoppingCart(); shoppingCart.addItem({ id: 1, name: 'Product 1', price: 10 }, 2); shoppingCart.addItem({ id: 2, name: 'Product 2', price: 20 }, 1); console.log(shoppingCart.getTotalPrice());
Performance Consideration: Database Queries
Efficient database queries are crucial for optimal performance in web applications. Let's explore performance considerations for database queries in PHP and JavaScript.
PHP Performance Consideration: Prepared Statements
Using prepared statements in PHP can improve performance by reducing the overhead of compiling and optimizing SQL queries. Here is an example of using prepared statements in PHP:
$stmt = $conn->prepare("SELECT * FROM users WHERE username = ?"); $stmt->bind_param("s", $username); $stmt->execute(); $result = $stmt->get_result(); while ($row = $result->fetch_assoc()) { echo $row['name']; } $stmt->close();
Related Article: How to Implement Asynchronous Code in PHP
JavaScript Performance Consideration: Database Indexing
Properly indexing database tables in JavaScript can significantly improve query performance. Here is an example of creating an index in MongoDB:
db.users.createIndex({ username: 1 });
Advanced Technique: Server-Side Rendering
Server-side rendering (SSR) allows for rendering web pages on the server and sending the fully rendered HTML to the client. Let's explore server-side rendering in PHP and JavaScript.
PHP Advanced Technique: Laravel Blade Templates
Laravel Blade is a templating engine that allows for server-side rendering in PHP. Here is an example of using Laravel Blade for server-side rendering:
// home.blade.php <title>Home</title> <h1>Welcome to the home page</h1> // route.php Route::get('/', function () { return view('home'); });
JavaScript Advanced Technique: Next.js
Next.js is a popular framework for server-side rendering in JavaScript. It allows for building server-rendered React applications with ease. Here is an example of using Next.js for server-side rendering:
// pages/index.js import React from 'react'; function HomePage() { return ( <div> <h1>Welcome to the home page</h1> </div> ); } export default HomePage;