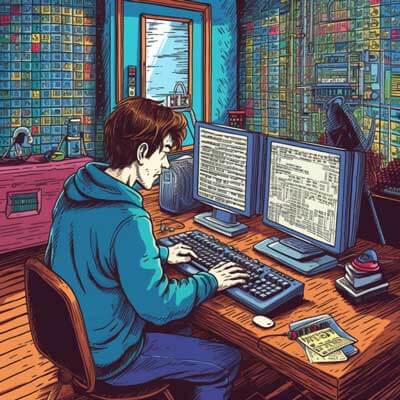
Table of Contents
Overview of the Ceiling Function
The ceiling function is a mathematical function that allows you to round up a number to the nearest integer. The resulting integer will always be greater than or equal to the original number. This can be useful in various scenarios, such as when you need to calculate the number of items needed for a certain quantity or when you want to ensure that a value is always rounded up to avoid any discrepancies.
The ceiling function is part of the math module in Python, which provides a set of mathematical functions and constants. To use the ceiling function, you need to import the math module into your Python script or interactive session.
Related Article: How to Run External Programs in Python 3 with Subprocess
How to Use the math.ceil Function
To use the ceiling function in Python, you need to call the math.ceil() method and pass in the number that you want to round up. The method will return the rounded up value as an integer.
Here's an example of how to use the math.ceil() function:
import math x = 4.2 rounded_up = math.ceil(x) print(rounded_up) # Output: 5
In this example, we import the math module and assign the value 4.2 to the variable x
. We then call the math.ceil() function and pass in x
as the argument. The returned value is assigned to the variable rounded_up
, which is then printed to the console.
Differences Between math.ceil and math.floor
While both math.ceil() and math.floor() functions in Python are used for rounding numbers, they have a key difference. The ceil() function always rounds a number up to the nearest integer, while the floor() function always rounds a number down to the nearest integer.
Let's see an example to understand the difference between the two functions:
import math x = 4.2 rounded_up = math.ceil(x) rounded_down = math.floor(x) print(rounded_up) # Output: 5 print(rounded_down) # Output: 4
In this example, we use the same value x = 4.2
and apply the math.ceil() and math.floor() functions. The ceil() function rounds up the number to 5, while the floor() function rounds it down to 4.
Code Snippet: Rounding Up Using the Ceiling Function
To round up a number using the ceiling function in Python, you simply need to call the math.ceil() function and pass in the number you want to round up. The function will return the rounded up value as an integer.
Here's a code snippet that demonstrates rounding up using the ceiling function:
import math def round_up(number): return math.ceil(number) x = 4.2 rounded_up = round_up(x) print(rounded_up) # Output: 5
In this code snippet, we define a function called round_up()
that takes a number as an argument. Inside the function, we call the math.ceil() function and pass in the number to round it up. The rounded up value is then returned by the function.
We then assign the value 4.2 to the variable x
and call the round_up()
function with x
as the argument. The returned value is assigned to the variable rounded_up
and printed to the console.
Related Article: How to Improve the Security of Flask Web Apps
The Mathematical Ceiling and Ceiling Value
In mathematics, the ceiling function is also known as the "ceiling value" or "smallest integer not less than" function. It is denoted by the symbol ⌈x⌉ or ceil(x).
The ceiling function rounds a number up to the nearest integer that is greater than or equal to the original number. This means that if the number is already an integer, the ceiling function will return the same value.
For example:
- ceil(4.2) = 5
- ceil(7) = 7
The ceiling function is commonly used in various fields, such as finance, statistics, and computer science. It helps ensure accuracy and precision in calculations where rounding up is necessary.
In Python, the math.ceil() function provides a convenient way to apply the ceiling function to numbers. By using this function, you can easily round up numbers and perform calculations with confidence.
Additional Resources
- math.ceil() in Python