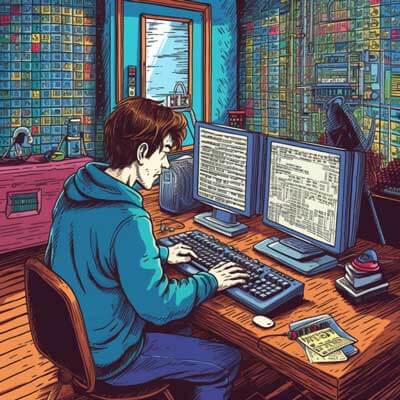
Table of Contents
Overview of the Python Deleter Functionality
The deleter
is a special method that allows you to define the behavior of deleting an attribute of an object. It is part of the property decorator, which provides a way to encapsulate the access to attributes of a class. By using the deleter
, you can define what happens when an attribute is deleted, allowing you to perform any necessary cleanup or custom actions.
The deleter
function is defined within a property decorator and has the same name as the property it is associated with. It takes a single parameter, self
, which refers to the instance of the class the property belongs to. Inside the deleter
function, you can define the actions to be taken when the attribute is deleted.
Using the deleter
functionality can help make your code more organized and maintainable. It allows you to define the behavior of deleting an attribute in a centralized location, making it easier to manage and update.
Related Article: How To Handle Ambiguous Truth Values In Python Arrays
Deleting Files in Python
Deleting files in Python often requires careful handling to avoid accidentally deleting important data. Luckily, Python provides an easy way to delete files using the os
module.
To delete a file in Python, you can use the os.remove()
function. This function takes a single parameter, which is the path to the file you want to delete. Here's an example:
import os file_path = "/path/to/file.txt" os.remove(file_path)
Make sure to provide the correct file path to the os.remove()
function. If the file does not exist or the path is incorrect, an error will be raised.
Deleting Directories
Deleting directories in Python follows a similar approach to deleting files. However, since directories can contain multiple files and subdirectories, you need to use a different function from the os
module.
To delete a directory in Python, you can use the os.rmdir()
function. This function takes a single parameter, which is the path to the directory you want to delete. Here's an example:
import os dir_path = "/path/to/directory" os.rmdir(dir_path)
Just like with deleting files, make sure to provide the correct directory path to the os.rmdir()
function. If the directory does not exist or the path is incorrect, an error will be raised. Additionally, the directory must be empty for the deletion to succeed. If the directory contains any files or subdirectories, you will need to delete them first before deleting the parent directory.
Removing Items from a List
Python provides several ways to remove an item from a list. The method you choose depends on the specific requirements of your code.
One common way to remove an item from a list is to use the remove()
method. This method takes a single parameter, which is the value of the item you want to remove. Here's an example:
my_list = [1, 2, 3, 4, 5] my_list.remove(3) print(my_list) # [1, 2, 4, 5]
The remove()
method removes the first occurrence of the specified value from the list. If the value is not found in the list, a ValueError
will be raised. If you need to remove all occurrences of a value, you can use a loop or a list comprehension.
Another way to remove an item from a list is to use the del
statement. This statement allows you to delete an item by its index. Here's an example:
my_list = [1, 2, 3, 4, 5] del my_list[2] print(my_list) # [1, 2, 4, 5]
The del
statement removes the item at the specified index from the list. If the index is out of range, a IndexError
will be raised.
Related Article: PHP vs Python: How to Choose the Right Language
Deleting Keys from a Dictionary
Dictionaries are a useful data structure that allows you to store key-value pairs. To delete a key from a dictionary, you can use the del
statement or the pop()
method.
Using the del
statement, you can remove a key-value pair from a dictionary by specifying the key you want to delete. Here's an example:
my_dict = {'name': 'John', 'age': 30, 'city': 'New York'} del my_dict['age'] print(my_dict) # {'name': 'John', 'city': 'New York'}
The del
statement removes the key-value pair with the specified key from the dictionary. If the key is not found in the dictionary, a KeyError
will be raised.
Alternatively, you can use the pop()
method to remove a key-value pair from a dictionary. This method takes a single parameter, which is the key you want to remove. Here's an example:
my_dict = {'name': 'John', 'age': 30, 'city': 'New York'} my_dict.pop('age') print(my_dict) # {'name': 'John', 'city': 'New York'}
The pop()
method removes the key-value pair with the specified key from the dictionary and returns the value. If the key is not found in the dictionary, a KeyError
will be raised.
Deleting Specific Elements from Lists
In addition to removing a specific item from a list, you may also need to delete multiple items that meet certain conditions. Python provides several ways to achieve this.
One way to delete specific elements from a list is to use a list comprehension. A list comprehension allows you to create a new list based on the elements of an existing list that satisfy a certain condition. Here's an example:
my_list = [1, 2, 3, 4, 5] my_list = [x for x in my_list if x != 3] print(my_list) # [1, 2, 4, 5]
In this example, the list comprehension iterates over each element of my_list
and adds it to the new list if it is not equal to 3. This effectively removes the element with the value 3 from the list.
Another way to delete specific elements from a list is to use the filter()
function. This function takes two parameters: a function that determines whether an element should be included in the filtered list, and the list to be filtered. Here's an example:
my_list = [1, 2, 3, 4, 5] my_list = list(filter(lambda x: x != 3, my_list)) print(my_list) # [1, 2, 4, 5]
In this example, the lambda
function checks if an element is not equal to 3, and the filter()
function applies this function to each element of my_list
. The resulting filtered list contains only the elements that satisfy the condition.
Code Snippet: Deleting a File in Python
import os file_path = "/path/to/file.txt" os.remove(file_path)
This code snippet demonstrates how to delete a file in Python using the os.remove()
function. Make sure to provide the correct file path to the function to avoid errors.
Code Snippet: Removing an Item from a List in Python
my_list = [1, 2, 3, 4, 5] my_list.remove(3) print(my_list) # [1, 2, 4, 5]
This code snippet shows how to remove a specific item from a list using the remove()
method. The specified item is removed from the list, and the resulting list is printed.
Related Article: Real-Time Features with Flask-SocketIO and WebSockets
Code Snippet: Deleting a Key from a Dictionary in Python
my_dict = {'name': 'John', 'age': 30, 'city': 'New York'} del my_dict['age'] print(my_dict) # {'name': 'John', 'city': 'New York'}
This code snippet demonstrates how to delete a key from a dictionary using the del
statement. The specified key is removed along with its corresponding value, and the resulting dictionary is printed.
Additional Resources
- Deleting Files in Python