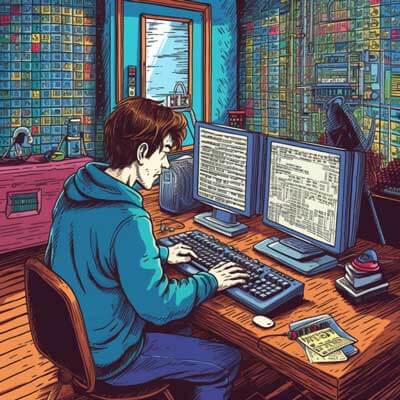
Table of Contents
Overview of String Reversal in Python
String reversal is a common operation in many programming languages, including Python. Reversing a string means changing the order of its characters so that the last character becomes the first, the second-to-last character becomes the second, and so on. In Python, there are several ways to reverse a string, ranging from simple and straightforward approaches to more advanced techniques. In this article, we will explore different methods for reversing a string in Python, including slicing, recursion, and using built-in library functions.
Related Article: How to Use Class And Instance Variables in Python
Code Snippet for Reversing a String using Slicing
One of the simplest and most efficient ways to reverse a string in Python is by using slicing. Slicing allows us to extract a portion of a string by specifying a range of indices.
Here's an example code snippet that demonstrates how to reverse a string using slicing:
def reverse_string(string): return string[::-1] original_string = "Hello, World!" reversed_string = reverse_string(original_string) print(reversed_string)
In this code, we define a function called reverse_string
that takes a string as input and returns the reversed string using slicing. The slicing syntax [start:end:step]
allows us to specify a negative step value -1
, which reverses the string. Finally, we call the reverse_string
function with an example string "Hello, World!" and print the reversed string.
Output:
!dlroW ,olleH
Using slicing to reverse a string is a concise and efficient approach, as it takes advantage of the built-in slicing functionality in Python.
Code Snippet for Reversing a String using Recursion
Another approach to reverse a string in Python is by using recursion. Recursion is a programming technique where a function calls itself to solve a smaller subproblem.
Here's an example code snippet that demonstrates how to reverse a string using recursion:
def reverse_string_recursive(string): if len(string) == 0: return "" else: return reverse_string_recursive(string[1:]) + string[0] original_string = "Hello, World!" reversed_string = reverse_string_recursive(original_string) print(reversed_string)
In this code, we define a function called reverse_string_recursive
that takes a string as input and returns the reversed string using recursion. The base case for the recursion is when the length of the string becomes zero, in which case an empty string is returned. Otherwise, the function calls itself with a substring starting from the second character (string[1:]
) and concatenates it with the first character (string[0]
).
Output:
!dlroW ,olleH
Using recursion to reverse a string provides a more intuitive and elegant solution, but it may not be as efficient as the slicing method for very long strings due to the overhead of function calls.
Using Python's Library for String Reversal
Python provides a built-in reverse()
function for lists that can also be used to reverse a string indirectly. Since strings in Python are immutable, we need to convert the string into a list, reverse the list using the reverse()
function, and then convert the list back into a string.
Here's an example code snippet that demonstrates how to reverse a string using the reverse()
function:
def reverse_string_library(string): string_list = list(string) string_list.reverse() reversed_string = "".join(string_list) return reversed_string original_string = "Hello, World!" reversed_string = reverse_string_library(original_string) print(reversed_string)
In this code, we define a function called reverse_string_library
that takes a string as input and returns the reversed string using the reverse()
function. We convert the string into a list using the list()
function, reverse the list using the reverse()
function, and finally join the reversed list elements back into a string using the join()
function.
Output:
!dlroW ,olleH
Using the built-in reverse()
function can be a convenient way to reverse a string, especially if you are already working with lists in your code.
Related Article: How To Limit Floats To Two Decimal Points In Python
Common Methods for Reversing a String in Python
In addition to the methods mentioned above, there are other common methods for reversing a string in Python. Here are a few examples:
Using a Loop: We can iterate through the characters of the string in reverse order and concatenate them to a new string.
def reverse_string_loop(string): reversed_string = "" for char in string[::-1]: reversed_string += char return reversed_string
Using the reversed()
function: We can use the reversed()
function to reverse the characters of the string and then join them back into a string.
def reverse_string_reversed(string): reversed_string = "".join(reversed(string)) return reversed_string
Using the join()
function with a generator expression: We can use a generator expression to reverse the characters of the string and then join them back into a string.
def reverse_string_generator(string): reversed_string = "".join(char for char in reversed(string)) return reversed_string
These methods provide alternative ways to reverse a string in Python, allowing you to choose the one that best fits your specific use case.