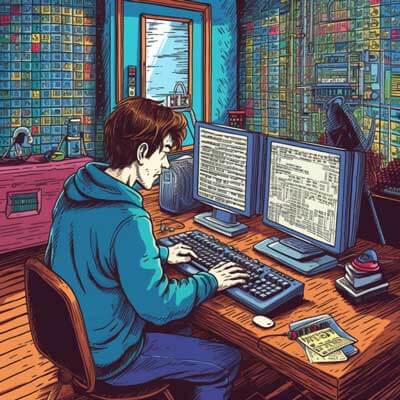
Table of Contents
Introduction to Main Function
The main function is a fundamental concept in Python programming. It serves as the entry point for the execution of a Python program. When a Python script is run, the interpreter starts executing the code from the top, line by line. However, only the code within the main function will be executed automatically.
Related Article: 19 Python Code Snippets for Everyday Issues
Main Function: Definition and Syntax
In Python, the main function is defined like any other function, using the def
keyword followed by the function name, parentheses, and a colon. The syntax for defining the main function is as follows:
def main(): # code to be executed
The name of the main function can be anything you prefer, but it is conventionally named main
to indicate its purpose.
Role of the Main Function in Code Execution
The main function plays a crucial role in the execution of a Python program. It serves as the starting point from which the interpreter begins executing the code. All statements and functions called within the main function will be executed in the order they are written.
By convention, the main function is typically placed at the end of the script, after all other functions and class definitions. This ensures that all necessary code has been defined before the main function is executed.
Best Practices for Using Main Function
To ensure clean and organized code, it is recommended to follow some best practices when using the main function in Python:
1. Keep the main function simple and focused: The main function should primarily serve as a coordinator, calling other functions and initiating the program's execution. Avoid placing complex or lengthy code directly within the main function.
2. Use helper functions: Break down your program's logic into smaller, reusable functions. This promotes code modularity and makes it easier to test and maintain.
3. Avoid global variables: Instead of relying on global variables, pass data through function parameters and return values. This improves code readability and reduces the risk of naming conflicts.
4. Use if __name__ == "__main__": guard: This conditional statement ensures that the code within it is only executed if the script is run directly, and not imported as a module. It prevents unintended execution when importing the script into another program.
Here's an example demonstrating these best practices:
def helper_function(): # code for a helper function def main(): # code for the main function helper_function() if __name__ == "__main__": main()
Related Article: How to End Python Programs
Real World Examples of Main Function Usage
Let's explore some real-world examples of how the main function is used in different contexts:
Use Case: Main Function in Data Analysis
In data analysis projects, the main function is often used to orchestrate the data processing pipeline. It calls functions for loading data, preprocessing, feature extraction, model training, and evaluation. Here's a simplified example:
def load_data(): # code to load data def preprocess_data(): # code to preprocess data def train_model(): # code to train model def evaluate_model(): # code to evaluate model def main(): data = load_data() preprocessed_data = preprocess_data(data) model = train_model(preprocessed_data) evaluate_model(model) if __name__ == "__main__": main()
Use Case: Main Function in Web Development
In web development, the main function is commonly used to start the web server and handle incoming requests. Here's a basic example using the Flask framework:
from flask import Flask, request app = Flask(__name__) @app.route("/") def index(): return "Hello, World!" def main(): app.run() if __name__ == "__main__": main()
Use Case: Main Function in Machine Learning
In machine learning projects, the main function is often responsible for loading a trained model and using it to make predictions on new data. Here's a simple example:
import joblib def load_model(): # code to load trained model def predict(model, data): # code to make predictions using the model def main(): model = load_model() data = load_new_data() predictions = predict(model, data) print(predictions) if __name__ == "__main__": main()
Related Article: How To Manually Raise An Exception In Python
Performance Considerations: Main Function in Large Programs
In large programs, it's important to consider the performance implications of the main function. When a program grows in size, the main function may become a bottleneck if it performs computationally expensive operations or has a complex control flow.
To mitigate the performance impact, consider the following techniques:
- Offload computationally expensive tasks to separate functions or modules.
- Use concurrency techniques, such as multi-threading or asynchronous programming, to parallelize tasks and improve overall performance.
- Employ efficient data structures and algorithms to optimize code execution time.
Advanced Techniques: Main Function in Recursive Algorithms
Recursive algorithms are algorithms that solve a problem by reducing it to smaller, similar subproblems. In such algorithms, the main function can be used to initiate the recursive calls and handle the termination condition. Here's an example of a recursive algorithm to calculate the factorial of a number:
def factorial(n): if n == 0: return 1 else: return n * factorial(n - 1) def main(): n = int(input("Enter a number: ")) result = factorial(n) print(f"The factorial of {n} is {result}") if __name__ == "__main__": main()
Advanced Techniques: Main Function in Multi-threading
Multi-threading allows a program to perform multiple tasks concurrently, utilizing multiple threads of execution. The main function can be used to spawn and manage multiple threads. Here's a simple example using the threading module:
import threading def worker(): # code for the worker thread def main(): threads = [] for _ in range(5): t = threading.Thread(target=worker) threads.append(t) t.start() for t in threads: t.join() if __name__ == "__main__": main()
Code Snippet: Basic Usage of Main Function
def main(): print("Hello, World!") if __name__ == "__main__": main()
Related Article: How To Fix ValueError: Invalid Literal For Int With Base 10
Code Snippet: Main Function in Class Methods
class MyClass: def __init__(self): pass def main(self): print("This is the main method of MyClass") if __name__ == "__main__": obj = MyClass() obj.main()
Code Snippet: Main Function with Command Line Arguments
import sys def main(): args = sys.argv[1:] print(f"Command line arguments: {args}") if __name__ == "__main__": main()
Code Snippet: Main Function in Recursive Code
def recursive_function(n): if n == 0: return else: print(n) recursive_function(n - 1) def main(): recursive_function(5) if __name__ == "__main__": main()
Code Snippet: Main Function in a Multi-threaded Environment
import threading def worker(): print("This is the worker thread") def main(): t = threading.Thread(target=worker) t.start() t.join() if __name__ == "__main__": main()
Related Article: How to Parse a YAML File in Python
Error Handling and the Main Function
Error handling is an important aspect of any program, including those that use the main function. By convention, error handling is often done within the main function to catch and handle exceptions gracefully. Here's an example:
def main(): try: # code that may raise an exception result = 10 / 0 print(result) except ZeroDivisionError: print("Cannot divide by zero") except Exception as e: print(f"An error occurred: {str(e)}") if __name__ == "__main__": main()
In this example, the code within the main function attempts to divide 10 by 0, which raises a ZeroDivisionError. The exception is caught within the main function, and an appropriate error message is displayed.