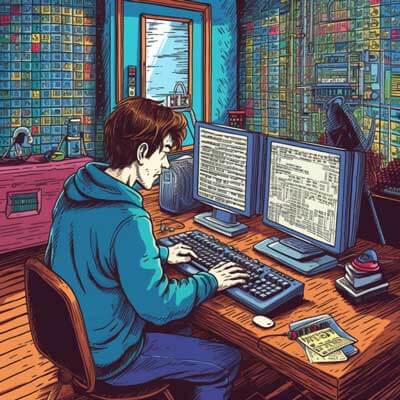
Table of Contents
Overview of Priority Queue in Python
In computer science, a priority queue is an abstract data type that is similar to a regular queue, but with each element having a priority associated with it. The priority determines the order in which the elements are processed, with higher priority elements being processed before lower priority elements.
Python provides several ways to implement a priority queue, with the most common approach being using a heap queue. This article will explore the implementation of a priority queue in Python using the heapq module, as well as discuss the role of binary heaps in priority queues.
Related Article: How To Access Index In Python For Loops
Heap Queue and Its Implementation
A heap queue, also known as a binary heap, is a complete binary tree where each node has a value greater than or equal to its children (in the case of a max heap) or less than or equal to its children (in the case of a min heap). This property allows for efficient insertion and removal of elements based on their priority.
To implement a heap queue in Python, we can use the heapq module, which provides functions to create and manipulate heap data structures. The heapq module uses a list to represent the binary heap, with the first element of the list being the root of the heap.
Let's take a look at an example of how to create and manipulate a heap queue using the heapq module:
import heapq # Create an empty heap heap = [] # Insert elements into the heap heapq.heappush(heap, 5) heapq.heappush(heap, 3) heapq.heappush(heap, 8) heapq.heappush(heap, 1) # Remove and return the smallest element from the heap smallest = heapq.heappop(heap) print(smallest) # Output: 1
In the example above, we create an empty heap using an empty list. We then use the heappush()
function to insert elements into the heap, and the heappop()
function to remove and return the smallest element from the heap.
Binary Heap and Its Role in Priority Queues
A binary heap is a complete binary tree that satisfies the heap property. In a max heap, for every node i
other than the root, the value of heap[i]
is greater than or equal to the values of its children. In a min heap, the value of heap[i]
is less than or equal to the values of its children.
Binary heaps are an efficient data structure for implementing priority queues because they allow for efficient insertion and removal of elements based on their priority. The heap property ensures that the highest (or lowest) priority element is always at the root of the heap, making it easy to access and remove.
Priority Queue Implementation using heapq Module
Python's heapq module provides functions to create and manipulate heap data structures, which can be used to implement a priority queue. The heappush()
function can be used to insert elements into the heap, and the heappop()
function can be used to remove and return the element with the highest (or lowest) priority.
Here's an example of how to implement a priority queue using the heapq module:
import heapq class PriorityQueue: def __init__(self): self.heap = [] def push(self, item, priority): heapq.heappush(self.heap, (priority, item)) def pop(self): priority, item = heapq.heappop(self.heap) return item def is_empty(self): return len(self.heap) == 0
In the example above, we define a PriorityQueue
class that uses a list as the underlying data structure for the heap. The push()
method takes an item and its priority as arguments, and inserts a tuple (priority, item)
into the heap using the heappush()
function. The pop()
method removes and returns the item with the highest (or lowest) priority from the heap. The is_empty()
method checks if the heap is empty.
Related Article: How to Access Python Data Structures with Square Brackets
Difference between Priority Queue and Regular Queue
While a regular queue follows the FIFO (First-In, First-Out) principle, a priority queue uses a priority value associated with each element to determine the order in which elements are processed. In a regular queue, elements are processed in the order they were added, whereas in a priority queue, elements are processed based on their priority.
A priority queue allows for efficient access and removal of the element with the highest (or lowest) priority, which can be useful in various applications such as task scheduling, event processing, and graph algorithms.
Using Binary Heap for Priority Queue Implementation
As mentioned earlier, a binary heap is a complete binary tree that satisfies the heap property, making it an efficient data structure for implementing a priority queue. The heap property ensures that the highest (or lowest) priority element is always at the root of the heap, allowing for efficient access and removal of elements.
Here's an example of how to use a binary heap for priority queue implementation:
import heapq # Create an empty heap heap = [] # Insert elements into the heap with priority heapq.heappush(heap, (5, 'Task 1')) heapq.heappush(heap, (3, 'Task 2')) heapq.heappush(heap, (8, 'Task 3')) heapq.heappush(heap, (1, 'Task 4')) # Remove and return the element with the highest priority highest_priority = heapq.heappop(heap) print(highest_priority) # Output: (1, 'Task 4')
In the example above, we create an empty heap and use the heappush()
function to insert elements into the heap with their respective priorities. The elements are tuples containing the priority and the task name. We then use the heappop()
function to remove and return the element with the highest priority, which in this case is (1, 'Task 4')
.
Additional Resources
- Implementing a Priority Queue in Python