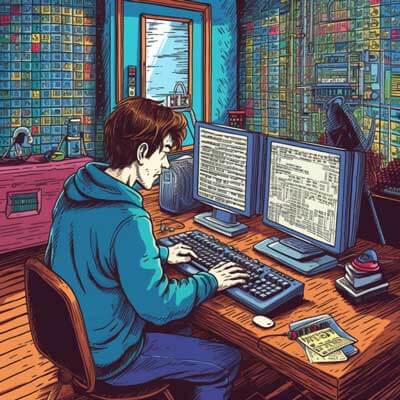
Table of Contents
The Reduce Function in Functional Programming
Functional programming is a coding paradigm that focuses on using pure functions and immutable data. One of the key functions in functional programming is the reduce function. The reduce function takes an iterable, applies a function to each element, and returns a single value by reducing the iterable to a single result.
The reduce function follows a simple pattern: it takes two arguments - the function to apply and the iterable to process. The function is applied to the first two elements of the iterable, then to the result and the next element, and so on, until all elements of the iterable are processed and a single result is obtained.
Let's take a look at an example to understand how the reduce function works in practice.
from functools import reduce # A simple example - summing all numbers in a list numbers = [1, 2, 3, 4, 5] sum = reduce(lambda x, y: x + y, numbers) print(sum) # Output: 15
In this example, we use the reduce function to sum all the numbers in the numbers
list. The lambda function (lambda x, y: x + y)
takes two arguments x
and y
and returns their sum. The reduce function applies this lambda function to the first two elements of the list (1
and 2
), then to the result (3
) and the next element (3
), and so on, until it obtains the final sum of 15
.
The reduce function is a useful tool in functional programming as it allows us to perform complex operations on iterables in a concise and elegant way. It can be used for a variety of tasks, such as finding the maximum or minimum value in a list, calculating the product of all numbers, or even performing string concatenation.
Related Article: Fixing 'Dataframe Constructor Not Properly Called' in Python
Lambda Functions
Lambda functions, also known as anonymous functions, are small, single-line functions that don't have a name. They are commonly used in conjunction with the reduce function to define the operation to be applied to each element of the iterable.
Lambda functions are defined using the lambda
keyword followed by the arguments and a colon, and then the expression to be evaluated. The result of the lambda function is the value of the expression.
Let's see an example of using a lambda function with the reduce function to find the maximum value in a list:
from functools import reduce numbers = [5, 8, 3, 2, 1] max_value = reduce(lambda x, y: x if x > y else y, numbers) print(max_value) # Output: 8
In this example, the lambda function (lambda x, y: x if x > y else y)
compares two numbers x
and y
, and returns the larger of the two. The reduce function applies this lambda function to the first two elements of the list (5
and 8
), then to the result (8
) and the next element (3
), and so on, until it obtains the maximum value of 8
.
Lambda functions are a handy way to define simple operations inline without the need to define a separate named function. They can be especially useful when working with the reduce function as they allow for concise and readable code.
Iterables
In the context of the reduce function, an iterable is any object that can be looped over, such as a list, tuple, or string. The reduce function applies the provided function to each element of the iterable in a sequential manner, reducing the iterable to a single result.
Iterables are essential for the reduce function as they provide the elements to be processed. Without iterables, the reduce function would have nothing to operate on.
Let's look at an example of using the reduce function with a string:
from functools import reduce string = "Hello, World!" concatenated = reduce(lambda x, y: x + y, string) print(concatenated) # Output: Hello, World!
In this example, the reduce function is used to concatenate all the characters in the string. The lambda function (lambda x, y: x + y)
takes two characters x
and y
and returns their concatenation. The reduce function applies this lambda function to the first two characters of the string (H
and e
), then to the result (He
) and the next character (l
), and so on, until it obtains the final concatenated string Hello, World!
.
Iterables provide a flexible way to work with different types of data structures and allow the reduce function to process them effectively.
Accumulators
Accumulators play a crucial role in the reduce process. An accumulator is a variable that stores the intermediate result of the reduce function as it iterates over the elements of the iterable.
The accumulator is initially set to the first element of the iterable and is updated with the result of applying the function to the current accumulator value and the next element in the iterable. This process continues until all elements of the iterable have been processed, and the final result is obtained.
Let's see an example of using an accumulator in the reduce function to calculate the product of all numbers in a list:
from functools import reduce numbers = [2, 3, 4, 5] product = reduce(lambda x, y: x * y, numbers) print(product) # Output: 120
In this example, the lambda function (lambda x, y: x * y)
multiplies two numbers x
and y
. The reduce function applies this lambda function to the first two elements of the list (2
and 3
), then to the result (6
) and the next element (4
), and so on, until it obtains the final product of 120
.
The accumulator, in this case, starts with the value 2
and is updated with the product of each subsequent element in the list. This allows the reduce function to accumulate the intermediate results and calculate the overall product.
Accumulators are a fundamental concept in the reduce process as they enable the function to keep track of the intermediate results and produce the final output.
Related Article: How to Print a Python Dictionary Line by Line
Higher-Order Functions
In functional programming, higher-order functions are functions that can take other functions as arguments or return functions as results. The reduce function is a higher-order function as it takes a function as an argument and applies it to each element of the iterable.
The use of higher-order functions enhances the flexibility and reusability of code. It allows us to separate the logic of the operation from the actual data being processed, promoting a more modular and composable approach to programming.
Let's see an example of using a higher-order function with the reduce function to find the sum of squares of all numbers in a list:
from functools import reduce numbers = [1, 2, 3, 4, 5] sum_of_squares = reduce(lambda x, y: x + y, map(lambda x: x**2, numbers)) print(sum_of_squares) # Output: 55
In this example, we use the map function to apply a lambda function (lambda x: x**2)
to each element of the numbers
list. The map function returns a new iterable with the squared values. Then, the reduce function applies the lambda function (lambda x, y: x + y)
to the resulting iterable, summing all the squared values and obtaining the final result of 55
.
The higher-order function map is used to transform the data before it is processed by the reduce function. This separation of concerns allows for more modular and reusable code, where the operation and the data transformation are decoupled.
Comparison Between Reduce, Map, and Filter Functions
The reduce, map, and filter functions are three fundamental higher-order functions in Python that operate on iterables. While they have similarities, they serve different purposes and have distinct functionalities.
The map
function applies a given function to each element of an iterable and returns a new iterable with the results. It is commonly used to transform data by applying a specific operation to each element.
The filter
function applies a given function to each element of an iterable and returns a new iterable with only the elements for which the function returns True. It is commonly used to filter out elements based on a specific condition.
The reduce
function, as we have discussed earlier, applies a given function to each element of an iterable in a sequential manner, reducing the iterable to a single result. It is commonly used to perform calculations or aggregations on the elements of an iterable.
Let's see an example that demonstrates the differences between these three functions:
from functools import reduce numbers = [1, 2, 3, 4, 5] # Using map to square each number squared_numbers = map(lambda x: x**2, numbers) print(list(squared_numbers)) # Output: [1, 4, 9, 16, 25] # Using filter to get only even numbers even_numbers = filter(lambda x: x % 2 == 0, numbers) print(list(even_numbers)) # Output: [2, 4] # Using reduce to find the product of all numbers product = reduce(lambda x, y: x * y, numbers) print(product) # Output: 120
In this example, we use the map function to square each number in the numbers
list, the filter function to get only the even numbers, and the reduce function to find the product of all numbers.
Each of these functions serves a different purpose and provides a useful tool for working with iterables in Python.
Implementing the Reduce Function
To implement the reduce function in Python, we can use the functools
module, which provides a built-in implementation of the reduce function.
Here's an example of implementing the reduce function without using the built-in implementation:
def reduce_custom(func, iterable, initial=None): iterator = iter(iterable) if initial is None: try: initial = next(iterator) except StopIteration: raise TypeError('reduce() of empty sequence with no initial value') acc = initial for element in iterator: acc = func(acc, element) return acc numbers = [1, 2, 3, 4, 5] sum = reduce_custom(lambda x, y: x + y, numbers) print(sum) # Output: 15
In this implementation, we define a custom reduce_custom
function that takes three arguments: the function to apply, the iterable to process, and an optional initial value for the accumulator.
The function first checks if an initial value is provided. If not, it tries to get the first element of the iterable using the next()
function. If the iterable is empty and no initial value is provided, it raises a TypeError
.
The function then initializes the accumulator with the initial value and iterates over the remaining elements of the iterable, applying the function to the accumulator and each element.
Finally, the function returns the final value of the accumulator.
This custom implementation demonstrates the underlying mechanism of the reduce function and allows for a deeper understanding of its inner workings.
Additional Resources
- Documentation - functools.reduce