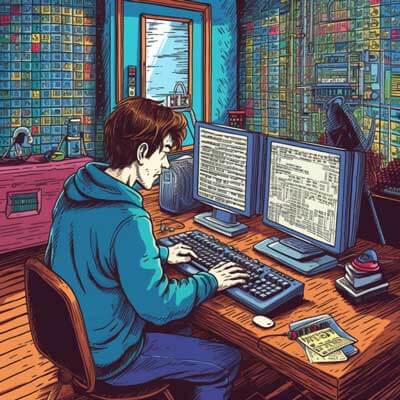
Table of Contents
Overview of Dictionary Sorting
A dictionary is an unordered collection of key-value pairs. While dictionaries are commonly used to store and retrieve data based on keys, there are times when we need to sort the dictionary based on either the keys or the values. Sorting a dictionary can be useful in a variety of scenarios, such as displaying data in a specific order or performing calculations based on sorted data.
Python provides several methods and techniques to sort a dictionary by either the keys or the values. In this tutorial, we will explore these methods and discuss best practices for dictionary sorting in Python.
Related Article: How to Use Class And Instance Variables in Python
Sorting Dictionary by Value
To sort a dictionary by its values, we can make use of the sorted()
function and the items()
method of the dictionary. The items()
method returns a list of tuples, where each tuple contains the key-value pair of the dictionary. By passing this list to the sorted()
function along with a lambda function to specify the sorting criteria, we can obtain a sorted list of key-value pairs.
Here is an example code snippet that demonstrates how to sort a dictionary by its values:
my_dict = {'apple': 3, 'banana': 1, 'orange': 2} sorted_dict = sorted(my_dict.items(), key=lambda x: x[1]) for key, value in sorted_dict: print(key, value)
Output:
banana 1 orange 2 apple 3
In the above example, we have a dictionary my_dict
with three key-value pairs. We use the sorted()
function to sort the dictionary by its values, using a lambda function to specify that the sorting should be based on the second element of each tuple (x[1]
). The sorted key-value pairs are then printed in the desired order.
Sorting Dictionary by Key
Similar to sorting by values, we can also sort a dictionary by its keys using the sorted()
function and the items()
method. By specifying the sorting criteria as the first element of each tuple (x[0]
), we can obtain a sorted list of key-value pairs based on the keys.
Here is an example code snippet that demonstrates how to sort a dictionary by its keys:
my_dict = {'apple': 3, 'banana': 1, 'orange': 2} sorted_dict = sorted(my_dict.items(), key=lambda x: x[0]) for key, value in sorted_dict: print(key, value)
Output:
apple 3 banana 1 orange 2
In the above example, we have the same dictionary my_dict
as before. We use the sorted()
function to sort the dictionary by its keys, using a lambda function to specify that the sorting should be based on the first element of each tuple (x[0]
). The sorted key-value pairs are then printed in the desired order.
Code Snippet: Sort Dictionary by Value
my_dict = {'apple': 3, 'banana': 1, 'orange': 2} sorted_dict = sorted(my_dict.items(), key=lambda x: x[1]) for key, value in sorted_dict: print(key, value)
Related Article: How to Write JSON Data to a File in Python
Code Snippet: Sort Dictionary by Key
my_dict = {'apple': 3, 'banana': 1, 'orange': 2} sorted_dict = sorted(my_dict.items(), key=lambda x: x[0]) for key, value in sorted_dict: print(key, value)
Common Pitfalls in Dictionary Sorting
When sorting a dictionary in Python, there are a few common pitfalls to be aware of:
1. Immutable Keys: If the keys of your dictionary are immutable objects, such as strings or numbers, you can sort the dictionary without any issues. However, if the keys are mutable objects, such as lists or dictionaries, you may encounter errors or unexpected results when trying to sort the dictionary.
2. Duplicate Keys: In a dictionary, each key must be unique. If you have duplicate keys in your dictionary, sorting by key may not yield the desired results. It is important to ensure that your dictionary has unique keys before attempting to sort it.
3. Performance: Sorting a dictionary can be computationally expensive, especially if the dictionary contains a large number of key-value pairs. Keep in mind the performance implications when sorting dictionaries, and consider whether sorting is necessary or if there are alternative approaches that can achieve the desired outcome.
Best Practices for Dictionary Sorting
To ensure smooth and accurate sorting of dictionaries in Python, it is recommended to follow these best practices:
1. Use the items()
method: When sorting a dictionary, it is helpful to convert it into a list of tuples using the items()
method. This allows for easier manipulation and sorting of the dictionary's key-value pairs.
2. Specify the sorting criteria: Use a lambda function or a custom sorting function to specify the sorting criteria. This ensures that the dictionary is sorted based on the desired element of each tuple, whether it is the key or the value.
3. Handle edge cases: Account for edge cases such as empty dictionaries, dictionaries with single key-value pairs, or dictionaries with duplicate keys. Handling these cases appropriately will prevent errors and ensure accurate sorting.
4. Consider alternatives: If sorting a dictionary is not necessary for your specific use case, consider alternative approaches that can achieve the desired outcome without the need for sorting. This can help improve performance and reduce code complexity.
Additional Resources
- Sorting a Dictionary in Python