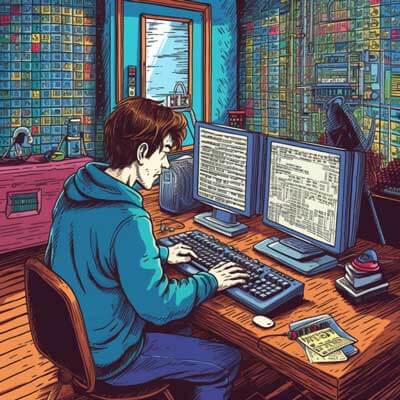
Table of Contents
Overview
The isnumeric() method is a built-in method in Python that allows us to check if a string consists of only numeric characters. It returns True if all the characters in the string are numeric, otherwise it returns False. The isnumeric() method is useful when we need to validate user input or perform operations based on numeric values.
Let's see an example of how to use the isnumeric() method:
number = "12345" print(number.isnumeric()) # Output: True word = "Hello" print(word.isnumeric()) # Output: False
In the above example, we first check if the string "12345" is numeric using the isnumeric() method, which returns True. Then we check if the string "Hello" is numeric, which returns False.
It's important to note that the isnumeric() method only returns True for positive integers. It does not consider negative numbers, decimal numbers, or complex numbers as numeric. If we need to validate such cases, we can use other methods like isdigit() or isdecimal().
Related Article: How to Run External Programs in Python 3 with Subprocess
Python's int Data Type
The int data type in Python represents whole numbers, both positive and negative. It is used to store and perform operations on integers. In Python, we can create an int object by assigning an integer value to a variable.
Here's an example of using the int data type:
number = 10 print(number) # Output: 10 negative_number = -5 print(negative_number) # Output: -5
In the above example, we create two variables, number
and negative_number
, and assign integer values to them. We can perform various operations on these variables using arithmetic operators such as addition, subtraction, multiplication, and division.
Exploring Python's float Data Type
The float data type in Python represents decimal numbers. It is used to store and perform operations on numbers with fractional parts. In Python, we can create a float object by assigning a decimal value to a variable.
Here's an example of using the float data type:
number = 3.14 print(number) # Output: 3.14 negative_number = -2.5 print(negative_number) # Output: -2.5
In the above example, we create two variables, number
and negative_number
, and assign float values to them. We can perform various operations on these variables using arithmetic operators.
It's important to note that floating-point arithmetic may not always be precise due to the nature of binary representation. Therefore, it's recommended to handle floating-point calculations with caution, especially when dealing with financial or critical calculations. Python provides the decimal
module for more precise decimal arithmetic if needed.
Python's complex Data Type
The complex data type in Python represents complex numbers. A complex number consists of a real part and an imaginary part. It is used to perform operations involving complex numbers, such as complex arithmetic and signal processing.
In Python, we can create a complex object by assigning a value with a real and imaginary part to a variable. The imaginary part is denoted by j
or J
.
Here's an example of using the complex data type:
number = 2 + 3j print(number) # Output: (2+3j) negative_number = -1 - 2j print(negative_number) # Output: (-1-2j)
In the above example, we create two variables, number
and negative_number
, and assign complex values to them. We can perform various operations on these variables using arithmetic operators.
Python provides built-in functions to extract the real and imaginary parts of a complex number, such as real()
and imag()
. We can also perform complex arithmetic operations, such as addition, subtraction, multiplication, and division, on complex numbers.
Related Article: Intro to Payment Processing in Django Web Apps
Checking if a String is Numeric in Python
In Python, we can check if a string is numeric using various methods, such as isnumeric()
, isdigit()
, isdecimal()
, and regular expressions.
We have already discussed the isnumeric()
method in a previous section. It returns True if all the characters in the string are numeric, otherwise it returns False.
The isdigit()
method is similar to isnumeric()
, but it only returns True for positive integers. It does not consider decimal numbers or complex numbers as numeric.
The isdecimal()
method is another method to check if a string consists of only decimal characters. It returns True if all the characters in the string are decimal, otherwise it returns False.
Here's an example of using these methods:
number = "12345" print(number.isnumeric()) # Output: True print(number.isdigit()) # Output: True print(number.isdecimal()) # Output: True decimal_number = "3.14" print(decimal_number.isnumeric()) # Output: False print(decimal_number.isdigit()) # Output: False print(decimal_number.isdecimal()) # Output: False negative_number = "-5" print(negative_number.isnumeric()) # Output: False print(negative_number.isdigit()) # Output: False print(negative_number.isdecimal()) # Output: False
In the above example, we first check if the string "12345" is numeric using the isnumeric()
, isdigit()
, and isdecimal()
methods, which all return True. Then, we check if the string "3.14" and "-5" are numeric using these methods, which all return False.
Regular expressions provide a more flexible way to check if a string is numeric. We can use the re
module in Python to match numeric patterns in strings.
Different Numeric Data Types in Python
Python provides several numeric data types to handle different types of numbers. The main numeric data types in Python are int, float, and complex.
The int data type is used to represent whole numbers, both positive and negative. It is stored as a fixed-size integer in memory.
The float data type is used to represent decimal numbers. It is stored as a floating-point number in memory.
The complex data type is used to represent complex numbers, which consist of a real part and an imaginary part. It is stored as a pair of floating-point numbers in memory.
In addition to these basic numeric data types, Python also provides other numeric data types such as decimal, fraction, and boolean. These data types are used for more specific purposes, such as precise decimal arithmetic, rational numbers, and logical values, respectively.
Converting a String to an Integer in Python
In Python, we can convert a string to an integer using the int()
function. The int()
function takes a string as an argument and returns an integer value.
Here's an example of converting a string to an integer:
number = "10" integer = int(number) print(integer) # Output: 10 print(type(integer)) # Output: <class 'int'>
In the above example, we convert the string "10" to an integer using the int()
function. The resulting integer value is then assigned to the variable integer
. We can verify the type of the variable using the type()
function.
It's important to note that if the string cannot be converted to an integer, a ValueError
exception is raised. Therefore, it's recommended to handle exceptions when converting strings to integers, especially when dealing with user input.
Performing Arithmetic Operations in Python
Python provides various arithmetic operators to perform numerical operations. These operators can be used with int, float, and complex data types to perform addition, subtraction, multiplication, division, and more.
Here's an example of performing arithmetic operations in Python:
a = 10 b = 5 addition = a + b print(addition) # Output: 15 subtraction = a - b print(subtraction) # Output: 5 multiplication = a * b print(multiplication) # Output: 50 division = a / b print(division) # Output: 2.0 modulo = a % b print(modulo) # Output: 0 exponentiation = a ** b print(exponentiation) # Output: 100000
In the above example, we perform various arithmetic operations on two variables, a
and b
. We use the addition operator (+) to add the values, subtraction operator (-) to subtract the values, multiplication operator (*) to multiply the values, division operator (/) to divide the values, modulo operator (%) to get the remainder of division, and exponentiation operator (**) to raise a
to the power of b
.
It's important to note that the result of division between two integers is always a float, even if the division is exact. If we want to perform integer division and get the quotient as an integer, we can use the double slash operator (//).
Related Article: Working with List of Lists in Python (Nested Lists)
Comparison of int, float, and complex Data Types
The int, float, and complex data types in Python have different characteristics and are used for different purposes.
The int data type is used to represent whole numbers, both positive and negative. It is stored as a fixed-size integer in memory. Integers are useful for counting, indexing, and performing integer arithmetic operations.
The float data type is used to represent decimal numbers. It is stored as a floating-point number in memory. Floats are useful for representing measurements, scientific calculations, and any situation that requires fractional values.
The complex data type is used to represent complex numbers, which consist of a real part and an imaginary part. It is stored as a pair of floating-point numbers in memory. Complex numbers are useful for mathematical calculations involving imaginary numbers, such as signal processing and electrical engineering.
Here's an example of comparing the int, float, and complex data types:
integer = 10 float_number = 3.14 complex_number = 2 + 3j print(type(integer)) # Output: <class 'int'> print(type(float_number)) # Output: <class 'float'> print(type(complex_number)) # Output: <class 'complex'>
In the above example, we create three variables, integer
, float_number
, and complex_number
, and assign values of different data types to them. We use the type()
function to get the type of each variable.
Additional Resources
- What are the different numeric data types in Python?
- How to convert a string to an integer in Python?
- How to perform arithmetic operations on numeric data types in Python?