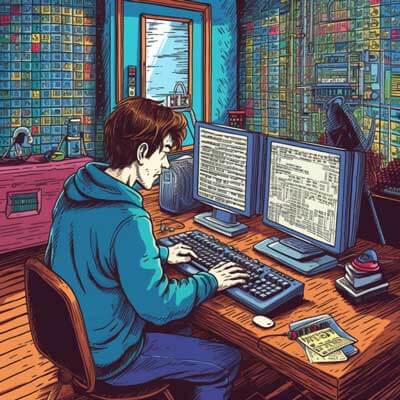
Table of Contents
Overview of Word Embeddings in NLP
Word embeddings play a crucial role in natural language processing (NLP) tasks, as they provide a numerical representation of words that can be used as input to machine learning models. These embeddings capture the semantic and syntactic relationships between words, allowing models to better understand and interpret text data.
One popular technique for generating word embeddings is Word2Vec, which is based on the idea that words appearing in similar contexts tend to have similar meanings. Word2Vec can be trained on large corpora of text data to learn word embeddings, which can then be used in various NLP tasks.
Another commonly used technique is GloVe (Global Vectors for Word Representation), which combines global co-occurrence counts with local context window information to generate word embeddings. GloVe embeddings capture both the semantic and the syntactic relationships between words, making them suitable for a wide range of NLP tasks.
In recent years, deep learning methods, particularly those based on neural networks, have also gained popularity in the field of NLP. Models such as recurrent neural networks (RNNs) and transformers can be used to learn word embeddings in an end-to-end manner, allowing for more accurate and context-aware representations of words.
Related Article: Overview of PyTorch Ecosystem and Libraries
Using PyTorch for Text Classification
Text classification is a fundamental task in NLP, where the goal is to assign predefined categories or labels to text documents. PyTorch provides useful tools and libraries that can be used to build text classification models efficiently.
To illustrate the process of text classification using PyTorch, let's consider a simple example of sentiment analysis, where the goal is to classify movie reviews as either positive or negative.
First, we need to preprocess the text data by tokenizing the sentences and converting the words into numerical representations. This can be done using PyTorch's torchtext
library, which provides convenient functions for data preprocessing and loading.
Once the data is preprocessed, we can build our text classification model. For sentiment analysis, a common approach is to use a recurrent neural network (RNN) or a convolutional neural network (CNN) to capture the sequential or spatial dependencies in the text, respectively.
Here's an example of how to define a simple RNN-based text classification model using PyTorch:
import torch import torch.nn as nn class RNNClassifier(nn.Module): def __init__(self, embedding_dim, hidden_dim, output_dim): super(RNNClassifier, self).__init__() self.embedding = nn.Embedding(vocab_size, embedding_dim) self.rnn = nn.RNN(embedding_dim, hidden_dim, batch_first=True) self.fc = nn.Linear(hidden_dim, output_dim) def forward(self, x): embedded = self.embedding(x) output, hidden = self.rnn(embedded) last_hidden = hidden.squeeze(0) logits = self.fc(last_hidden) return logits
In this example, the model takes as input a sequence of word indices, which are then embedded into dense vectors using an embedding layer. The embedded sequence is then passed through an RNN layer, and the final hidden state of the RNN is used to make predictions using a fully connected layer.
Once the model is defined, we can train it using PyTorch's optimization and training utilities. The training process involves computing the loss between the predicted labels and the ground truth labels, and updating the model's parameters using gradient descent. PyTorch provides easy-to-use functions for computing gradients and performing optimization, making the training process straightforward.
Implementing Sequence Labeling with PyTorch
Sequence labeling is another common NLP task, where the goal is to assign labels to each element in a sequence of tokens. This task is often used in named entity recognition (NER), part-of-speech tagging, and other similar applications.
PyTorch provides several tools and libraries that can be used to implement sequence labeling models efficiently. One popular approach is to use a conditional random field (CRF) layer on top of a neural network, which allows for modeling the dependencies between the labels in the sequence.
To illustrate the process of sequence labeling using PyTorch, let's consider the task of named entity recognition (NER), where the goal is to identify and classify named entities in text.
First, we need to prepare the data by tokenizing the text and assigning labels to each token. Next, we can build our sequence labeling model using PyTorch.
Here's an example of how to define a simple CRF-based NER model using PyTorch:
import torch import torch.nn as nn import torch.optim as optim from torchcrf import CRF class NERModel(nn.Module): def __init__(self, vocab_size, embedding_dim, hidden_dim, num_labels): super(NERModel, self).__init__() self.embedding = nn.Embedding(vocab_size, embedding_dim) self.lstm = nn.LSTM(embedding_dim, hidden_dim, bidirectional=True, batch_first=True) self.hidden2label = nn.Linear(hidden_dim * 2, num_labels) self.crf = CRF(num_labels) def forward(self, x): embedded = self.embedding(x) lstm_output, _ = self.lstm(embedded) logits = self.hidden2label(lstm_output) return logits model = NERModel(vocab_size, embedding_dim, hidden_dim, num_labels) optimizer = optim.Adam(model.parameters(), lr=learning_rate) criterion = nn.CrossEntropyLoss() # Training loop for epoch in range(num_epochs): optimizer.zero_grad() outputs = model(inputs) loss = criterion(outputs, targets) loss.backward() optimizer.step()
In this example, the model takes as input a sequence of word indices, which are then embedded into dense vectors using an embedding layer. The embedded sequence is then passed through an LSTM layer, and the output of the LSTM is used to make predictions using a linear layer. Finally, the CRF layer is used to model the dependencies between the labels and compute the loss.
The training process is similar to text classification, where we compute the loss between the predicted labels and the ground truth labels, and update the model's parameters using gradient descent.
Applying PyTorch for Sentiment Analysis
Sentiment analysis is a common NLP task that involves determining the sentiment expressed in a piece of text, such as positive, negative, or neutral. PyTorch provides useful tools and libraries that can be used to build sentiment analysis models efficiently.
To perform sentiment analysis using PyTorch, we can use techniques such as word embeddings and neural networks to capture the semantic and contextual information in the text.
Here's an example of how to implement a simple sentiment analysis model using PyTorch:
import torch import torch.nn as nn class SentimentAnalysisModel(nn.Module): def __init__(self, embedding_dim, hidden_dim, output_dim): super(SentimentAnalysisModel, self).__init__() self.embedding = nn.Embedding(vocab_size, embedding_dim) self.lstm = nn.LSTM(embedding_dim, hidden_dim, batch_first=True) self.fc = nn.Linear(hidden_dim, output_dim) def forward(self, x): embedded = self.embedding(x) lstm_output, _ = self.lstm(embedded) last_hidden = lstm_output[:, -1, :] logits = self.fc(last_hidden) return logits model = SentimentAnalysisModel(embedding_dim, hidden_dim, output_dim) criterion = nn.CrossEntropyLoss() optimizer = torch.optim.Adam(model.parameters(), lr=learning_rate) # Training loop for epoch in range(num_epochs): optimizer.zero_grad() outputs = model(inputs) loss = criterion(outputs, targets) loss.backward() optimizer.step()
In this example, the model takes as input a sequence of word indices, which are then embedded into dense vectors using an embedding layer. The embedded sequence is then passed through an LSTM layer, and the final hidden state of the LSTM is used to make predictions using a linear layer.
The training process involves computing the loss between the predicted labels and the ground truth labels, and updating the model's parameters using gradient descent.
Related Article: Practical Guide to PyTorch Model Deployment
Exploring Named Entity Recognition in NLP with PyTorch
Named Entity Recognition (NER) is a task in NLP that involves identifying and classifying named entities in text, such as person names, organization names, locations, and more. PyTorch provides useful tools and libraries that can be used to build NER models efficiently.
To perform NER using PyTorch, we can use techniques such as word embeddings, recurrent neural networks (RNNs), and conditional random fields (CRFs) to capture the contextual and sequential information in the text.
Here's an example of how to implement a simple NER model using PyTorch:
import torch import torch.nn as nn import torch.optim as optim from torchcrf import CRF class NERModel(nn.Module): def __init__(self, vocab_size, embedding_dim, hidden_dim, num_labels): super(NERModel, self).__init__() self.embedding = nn.Embedding(vocab_size, embedding_dim) self.lstm = nn.LSTM(embedding_dim, hidden_dim, bidirectional=True, batch_first=True) self.hidden2label = nn.Linear(hidden_dim * 2, num_labels) self.crf = CRF(num_labels) def forward(self, x): embedded = self.embedding(x) lstm_output, _ = self.lstm(embedded) logits = self.hidden2label(lstm_output) return logits model = NERModel(vocab_size, embedding_dim, hidden_dim, num_labels) optimizer = optim.Adam(model.parameters(), lr=learning_rate) criterion = nn.CrossEntropyLoss() # Training loop for epoch in range(num_epochs): optimizer.zero_grad() outputs = model(inputs) loss = criterion(outputs, targets) loss.backward() optimizer.step()
In this example, the model takes as input a sequence of word indices, which are then embedded into dense vectors using an embedding layer. The embedded sequence is then passed through an LSTM layer, and the output of the LSTM is used to make predictions using a linear layer. Finally, the CRF layer is used to model the dependencies between the labels and compute the loss.
The training process involves computing the loss between the predicted labels and the ground truth labels, and updating the model's parameters using gradient descent.
PyTorch Usage for Text Classification in NLP
Text classification is a fundamental task in natural language processing (NLP) that involves assigning predefined categories or labels to text documents. PyTorch provides useful tools and libraries that can be used to build text classification models efficiently.
To perform text classification using PyTorch, we can use techniques such as word embeddings, recurrent neural networks (RNNs), and convolutional neural networks (CNNs) to capture the semantic and contextual information in the text.
Here's an example of how to implement a simple text classification model using PyTorch:
import torch import torch.nn as nn class TextClassificationModel(nn.Module): def __init__(self, embedding_dim, hidden_dim, output_dim): super(TextClassificationModel, self).__init__() self.embedding = nn.Embedding(vocab_size, embedding_dim) self.lstm = nn.LSTM(embedding_dim, hidden_dim, batch_first=True) self.fc = nn.Linear(hidden_dim, output_dim) def forward(self, x): embedded = self.embedding(x) lstm_output, _ = self.lstm(embedded) last_hidden = lstm_output[:, -1, :] logits = self.fc(last_hidden) return logits model = TextClassificationModel(embedding_dim, hidden_dim, output_dim) criterion = nn.CrossEntropyLoss() optimizer = torch.optim.Adam(model.parameters(), lr=learning_rate) # Training loop for epoch in range(num_epochs): optimizer.zero_grad() outputs = model(inputs) loss = criterion(outputs, targets) loss.backward() optimizer.step()
In this example, the model takes as input a sequence of word indices, which are then embedded into dense vectors using an embedding layer. The embedded sequence is then passed through an LSTM layer, and the final hidden state of the LSTM is used to make predictions using a linear layer.
The training process involves computing the loss between the predicted labels and the ground truth labels, and updating the model's parameters using gradient descent.
Popular Applications of PyTorch in NLP
PyTorch, a popular deep learning framework, has been widely used in various applications of natural language processing (NLP). Some of the popular applications of PyTorch in NLP include:
1. Text Classification: PyTorch provides tools and libraries for building text classification models, which can be used to classify text documents into predefined categories or labels. These models can be trained on large datasets to achieve high accuracy in tasks such as sentiment analysis, spam detection, and topic classification.
2. Named Entity Recognition (NER): PyTorch can be used to build NER models that can identify and classify named entities in text, such as person names, organization names, locations, and more. These models can be trained on labeled datasets to extract useful information from unstructured text data.
3. Sentiment Analysis: PyTorch enables the development of sentiment analysis models that can determine the sentiment expressed in a piece of text, such as positive, negative, or neutral. These models can be trained on large datasets to classify text based on sentiment, which is useful in applications such as social media monitoring and customer feedback analysis.
4. Machine Translation: PyTorch can be used to build machine translation models that can automatically translate text from one language to another. These models leverage neural networks and attention mechanisms to capture the contextual and semantic information in the text, resulting in accurate translations.
5. Question Answering: PyTorch enables the development of question answering models that can answer questions based on a given context or passage. These models can be trained on large datasets to understand the context and extract relevant information to provide accurate answers.
These are just a few examples of the popular applications of PyTorch in NLP. With its flexibility and efficiency, PyTorch continues to be a preferred choice for researchers and practitioners in the field.
PyTorch Capabilities for Named Entity Recognition in NLP
Named Entity Recognition (NER) is a task in natural language processing (NLP) that involves identifying and classifying named entities in text, such as person names, organization names, locations, and more. PyTorch provides useful capabilities for building NER models efficiently.
PyTorch's flexible and dynamic computational graph allows for easy implementation of complex models for NER. Models can be built using PyTorch's neural network modules, which provide a wide range of layers and activation functions that can be combined to create custom architectures.
PyTorch also provides tools and libraries for data preprocessing and loading, making it easy to prepare the data for NER tasks. The torchtext
library, for example, provides functions for tokenizing text, converting words into numerical representations, and batching the data for efficient training.
Furthermore, PyTorch offers a variety of optimization algorithms and training utilities that can be used to train NER models effectively. The torch.optim
module provides implementations of popular optimization algorithms, such as stochastic gradient descent (SGD) and Adam, which can be used to update the model's parameters based on the computed gradients.
Additionally, PyTorch supports the use of conditional random fields (CRFs) for modeling the dependencies between the labels in sequence labeling tasks like NER. The torchcrf
library provides a CRF layer that can be easily integrated into PyTorch models, allowing for more accurate modeling of label dependencies and improved performance in NER tasks.
Related Article: Creating Custom Datasets and Dataloaders in PyTorch