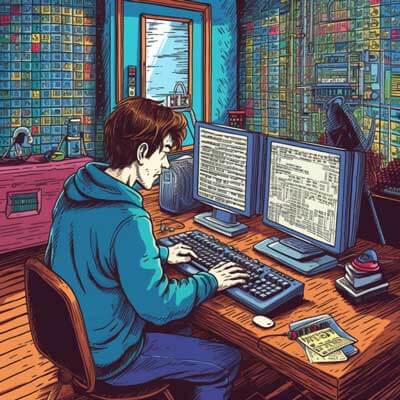
Table of Contents
Integrating Bootstrap with Ruby on Rails
Bootstrap is a popular front-end framework that allows developers to quickly build responsive and mobile-first websites. Integrating Bootstrap with Ruby on Rails can help streamline the process of designing and styling your Rails applications. In this section, we will explore how to integrate Bootstrap with Ruby on Rails and leverage its useful features.
To integrate Bootstrap with Ruby on Rails, you can make use of the bootstrap-sass gem. This gem provides Sass versions of Bootstrap CSS and JavaScript files, allowing you to easily customize and use Bootstrap components in your Rails application.
To get started, you will need to add the bootstrap-sass gem to your Gemfile:
gem 'bootstrap-sass'
After adding the gem, run the bundle command to install it:
bundle install
Next, you will need to import the Bootstrap stylesheets and JavaScript files in your Rails application. Open your app/assets/stylesheets/application.scss
file and add the following line to import the Bootstrap stylesheets:
@import "bootstrap-sprockets"; @import "bootstrap";
This will import the necessary Bootstrap stylesheets into your application. Additionally, you will need to include the Bootstrap JavaScript files. Open your app/assets/javascripts/application.js
file and add the following lines:
//= require jquery //= require bootstrap-sprockets
Now, you can use Bootstrap classes and components in your views. For example, to create a button with the Bootstrap styling, you can use the following code:
<%= link_to 'Click me', '#', class: 'btn btn-primary' %>
This will create a button with the class btn
for the base styling and btn-primary
for the blue color scheme.
Related Article: Ruby on Rails with WebSockets & Real-time Features
Using the Searchkick gem to integrate Elasticsearch with Rails
Elasticsearch is a useful search engine that provides fast and scalable full-text search capabilities. Integrating Elasticsearch with Ruby on Rails can greatly enhance the search functionality of your Rails applications. In this section, we will explore how to use the Searchkick gem to integrate Elasticsearch with Rails and perform advanced searches.
To integrate Elasticsearch with your Ruby on Rails application, you can make use of the Searchkick gem. This gem provides a simple and intuitive API for interacting with Elasticsearch and allows you to easily add full-text search capabilities to your Rails models.
To get started, you will need to add the searchkick gem to your Gemfile:
gem 'searchkick'
After adding the gem, run the bundle command to install it:
bundle install
Next, you will need to configure the Searchkick gem to connect to your Elasticsearch instance. Open your config/initializers/searchkick.rb
file and add the following lines:
Searchkick.client = Elasticsearch::Client.new(hosts: ['localhost:9200'])
This will configure the Searchkick gem to connect to Elasticsearch running on your local machine.
Now, you can use the Searchkick gem to perform advanced searches on your Rails models. For example, suppose you have a Product
model with a name
attribute. To perform a full-text search on the name
attribute, you can use the following code:
Product.search('iphone')
This will return all the products that have the word "iphone" in their name.
The Searchkick gem also provides additional features such as fuzzy search, autocomplete, and faceted search. You can refer to the Searchkick documentation for more details on how to use these features.
Popular databases for Ruby on Rails applications
When building Ruby on Rails applications, you have several options for choosing a database to store your application's data. In this section, we will discuss some of the popular databases used with Ruby on Rails and their advantages.
Advantages of using MySQL with Ruby on Rails
MySQL is one of the most widely used relational databases and is a popular choice for Ruby on Rails applications. It offers several advantages that make it a preferred choice for many developers.
- Performance: MySQL is known for its high performance and can handle large amounts of data efficiently. It is optimized for read-heavy workloads, making it suitable for applications with a high volume of read operations.
- Scalability: MySQL allows for easy horizontal scaling by adding more servers to handle increased traffic. It supports replication and clustering, making it a scalable choice for growing applications.
- Reliability: MySQL has a proven track record of reliability and stability. It is widely used in production environments and has a large community of users and developers who can provide support.
- Compatibility: MySQL is compatible with a wide range of platforms and programming languages, including Ruby on Rails. It has well-documented APIs and drivers, making it easy to integrate with Rails applications.
To use MySQL with Ruby on Rails, you will need to configure your database connection in your Rails application's config/database.yml
file. Here is an example configuration:
development: adapter: mysql2 encoding: utf8 database: myapp_development username: root password: password host: localhost port: 3306
Connecting Ruby on Rails with PostgreSQL
PostgreSQL is another popular choice for Ruby on Rails applications. It is a useful and feature-rich relational database that offers several advantages over other databases.
- Advanced features: PostgreSQL provides advanced features such as support for JSON data types, full-text search, and geospatial data. These features can be beneficial when building applications that require complex data processing and querying.
- Concurrency: PostgreSQL offers excellent support for concurrent access to the database, allowing multiple users to work simultaneously without conflicts. It uses a multi-version concurrency control (MVCC) system to achieve this.
- Extensibility: PostgreSQL allows you to define custom data types, operators, and functions, making it highly extensible. This can be useful when you need to store and query data that is not supported by the standard SQL data types.
- Reliability: PostgreSQL is known for its reliability and data integrity. It provides features such as transactions, ACID compliance, and crash recovery mechanisms, ensuring that your data remains consistent and safe.
To use PostgreSQL with Ruby on Rails, you will need to configure your database connection in your Rails application's config/database.yml
file. Here is an example configuration:
development: adapter: postgresql encoding: unicode database: myapp_development username: postgres password: password host: localhost port: 5432
Integrating MongoDB with Ruby on Rails
MongoDB is a popular NoSQL database that offers high scalability, flexibility, and performance. It is a document-oriented database that stores data in a flexible and schema-less format, making it suitable for applications with rapidly changing data models.
To integrate MongoDB with Ruby on Rails, you can use the Mongoid gem. Mongoid is an Object-Document Mapping (ODM) library for MongoDB that provides a simple and intuitive API for interacting with MongoDB from your Rails application.
To get started, you will need to add the mongoid gem to your Gemfile:
gem 'mongoid', '~> 7.0.5'
After adding the gem, run the bundle command to install it:
bundle install
Next, you will need to generate a configuration file for Mongoid. Run the following command to generate the configuration file:
rails generate mongoid:config
This will generate a config/mongoid.yml
file that you can use to configure your MongoDB connection.
To define your MongoDB models, you can use the Mongoid DSL. Here is an example of a User
model using Mongoid:
class User include Mongoid::Document field :name, type: String field :email, type: String end
With Mongoid, you can perform CRUD operations on your MongoDB models using familiar ActiveRecord-like methods. For example, to create a new user, you can use the following code:
User.create(name: 'John Doe', email: 'john@example.com')
Open source software tools for Ruby on Rails
Ruby on Rails has a vibrant ecosystem of open source tools and libraries that can help you streamline your development process and enhance your Rails applications. In this section, we will explore some popular open source software tools that can be used with Ruby on Rails.
Styling Ruby on Rails apps with Bootstrap
Bootstrap is a widely used open source front-end framework that provides a set of pre-styled components and a responsive grid system. It can be used to quickly style and design Ruby on Rails applications, making them visually appealing and user-friendly.
To integrate Bootstrap with Ruby on Rails, you can make use of the bootstrap-sass gem, as mentioned earlier. This gem provides Sass versions of Bootstrap CSS and JavaScript files, allowing you to easily customize and use Bootstrap components in your Rails application.
Modern frontend toolchains for Ruby on Rails
In recent years, modern frontend toolchains have gained popularity in the Ruby on Rails community. These toolchains, such as Webpacker and Stimulus, provide a modern approach to managing frontend assets and JavaScript in Rails applications.
Webpacker is a gem that allows you to use Webpack, a useful module bundler, to manage your frontend assets in Rails. It provides a seamless integration with Rails and allows you to use modern JavaScript frameworks and libraries in your Rails applications.
Stimulus is a JavaScript framework created by the team behind Basecamp that focuses on providing a lightweight and flexible way to add interactivity to your Rails views. It follows a "convention over configuration" approach and allows you to write JavaScript code in a declarative manner.
Related Article: Techniques for Handling Large Data with Ruby on Rails
Improving search functionality in Rails applications with the Searchkick gem
Search functionality is a crucial aspect of many Rails applications. The Searchkick gem, as mentioned earlier, provides a simple and useful way to integrate Elasticsearch with Ruby on Rails and enhance the search functionality of your applications.
In this section, we will explore some advanced features of the Searchkick gem that can help you improve the search functionality in your Rails applications.
Advanced search queries
The Searchkick gem allows you to perform advanced search queries using Elasticsearch's useful query DSL. You can use the search
method with a block to define custom search queries. Here is an example:
Product.search(query: { match: { name: 'iphone' } }) do highlight :name end
This will perform a search for products with the name "iphone" and include highlighting for the name field in the search results.
Auto-complete suggestions
The Searchkick gem also provides support for auto-complete suggestions, allowing you to provide real-time search suggestions to your users as they type. To enable auto-complete suggestions, you can use the suggest
method. Here is an example:
Product.search(query: { match: { name: 'ip' } }) do suggest :name_suggestions, { text: 'ip', term: { field: 'name', suggest_mode: 'always' } } end
This will perform a search for products with names containing "ip" and provide auto-complete suggestions based on the name field.
Additional Resources
- Using Sass with Rails