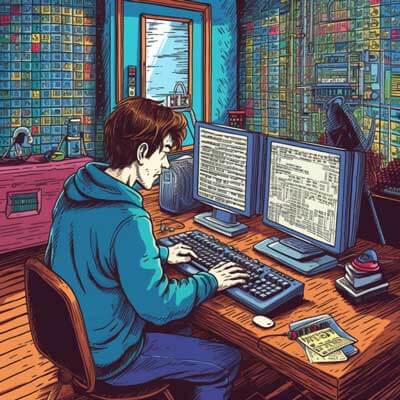
Table of Contents
Introduction
Ruby on Rails is a useful web application framework that allows developers to build robust and scalable applications. However, as your Rails application grows and handles more traffic, it's important to optimize its performance to ensure smooth and efficient operation. In this article, we will explore various techniques and best practices for performance tuning and optimization.
Related Article: Ruby on Rails with Modular Rails, ActiveRecord & Testing
ActiveRecord Query Optimization
One of the key aspects of performance tuning in Ruby on Rails is optimizing the database queries generated by ActiveRecord. By optimizing your queries, you can reduce the load on your database and improve the overall performance of your application.
Lazy Loading and Eager Loading
When working with ActiveRecord associations, it's important to understand the concepts of lazy loading and eager loading. Lazy loading is the default behavior in Rails, where associated records are loaded from the database only when they are accessed. While this can be convenient, it can also lead to the N+1 query problem, where multiple queries are executed to fetch associated records.
To address the N+1 query problem, Rails provides eager loading. Eager loading allows you to specify the associations that should be loaded upfront, reducing the number of queries executed. Let's consider an example:
# User model class User < ApplicationRecord has_many :posts end # Post model class Post < ApplicationRecord belongs_to :user end # Inefficient code using lazy loading users = User.all users.each do |user| puts user.posts.count end # Efficient code using eager loading users = User.includes(:posts).all users.each do |user| puts user.posts.count end
In the inefficient code snippet, a separate query is executed for each user to fetch their posts. In the efficient code snippet, we use the includes
method to eager load the associated posts, reducing the number of queries executed.
Query Optimization Techniques
In addition to eager loading, there are several other techniques you can use to optimize your ActiveRecord queries:
1. Select only the required columns: By selecting only the necessary columns, you can reduce the amount of data fetched from the database, improving query performance. For example:
# Inefficient query fetching all columns users = User.all # Efficient query fetching only required columns users = User.select(:name, :email)
2. Use joins
instead of includes
for read-only associations: When you have read-only associations, such as fetching comments for a blog post, you can use joins
instead of includes
to avoid loading unnecessary data. For example:
# Inefficient query using includes post = Post.includes(:comments).find(params[:id]) # Efficient query using joins post = Post.joins(:comments).find(params[:id])
3. Use pluck
for fetching specific columns: If you only need specific columns from a table, you can use the pluck
method to fetch those columns directly, instead of fetching the entire record. This can significantly reduce the amount of data transferred from the database. For example:
# Inefficient query fetching all columns user_emails = User.all.map(&:email) # Efficient query fetching only email column user_emails = User.pluck(:email)
Related Article: Ruby on Rails with WebSockets & Real-time Features
Database Optimization Techniques
Indexing
Indexing is a crucial technique for optimizing database performance. Indexes are data structures that allow the database to efficiently retrieve and search for records. By creating appropriate indexes on your database tables, you can speed up query execution and improve overall performance.
In Rails, you can define indexes using migrations. Let's consider an example where we want to add an index on the email
column of the users
table:
class AddIndexToUsersEmail < ActiveRecord::Migration[6.0] def change add_index :users, :email end end
In this example, we use the add_index
method to add an index on the email
column of the users
table. This index will improve the performance of queries that involve searching or sorting by email.
It's important to carefully consider which columns to index, as indexes come with some overhead in terms of storage and maintenance. Indexing columns that are frequently used in queries, such as foreign keys and columns involved in search conditions, can significantly improve query performance.
Denormalization
Denormalization is another technique that can improve database performance by reducing the number of joins required for fetching data. In a normalized database schema, data is organized into multiple tables to eliminate redundancy and ensure data integrity. However, joining multiple tables can be expensive in terms of performance.
To address this, denormalization involves storing redundant data in a single table to eliminate the need for joins. This can improve query performance, especially for read-heavy applications. However, denormalization also introduces data redundancy and the need for data synchronization.
Consider a scenario where you have a users
table and a posts
table, and you frequently need to fetch the user's name along with the post. In a normalized schema, you would need to join the users
and posts
table to retrieve the required data. However, by denormalizing the data and storing the user's name directly in the posts
table, you can eliminate the need for a join.
While denormalization can improve performance, it should be used judiciously and with caution. It's important to consider the trade-offs between performance and data redundancy, and ensure proper synchronization mechanisms are in place to maintain data integrity.
Front-End Performance Optimization
Related Article: Techniques for Handling Large Data with Ruby on Rails
Turbolinks
Turbolinks is a feature built into Rails that improves front-end performance by reducing page load times. It achieves this by replacing full page reloads with AJAX requests, allowing only the changed content to be updated.
To enable Turbolinks in your Rails application, you need to include the turbolinks
gem in your Gemfile and include it in your JavaScript manifest file.
# Gemfile gem 'turbolinks' # app/assets/javascripts/application.js //= require turbolinks
Once Turbolinks is enabled, any links or forms within your Rails application will be intercepted and handled using AJAX, resulting in faster page loads and a more responsive user experience.
Webpacker
Webpacker is a gem that integrates the webpack build system into Rails, providing useful front-end asset management capabilities. With Webpacker, you can easily manage and bundle your JavaScript, CSS, and other front-end assets, improving performance and reducing page load times.
To use Webpacker in your Rails application, you need to include the webpacker
gem in your Gemfile and run the installation command.
# Gemfile gem 'webpacker' # Terminal bundle install rails webpacker:install
Once Webpacker is installed, you can configure your front-end assets using webpack and leverage its features, such as code splitting, asset minification, and caching. This can greatly improve the performance of your application by reducing the size of assets and optimizing their delivery.
CDN Integration
Integrating a Content Delivery Network (CDN) into your Rails application can significantly improve front-end performance. A CDN is a network of servers located around the world that caches and delivers your static assets, such as images, CSS, and JavaScript files, from the server closest to the user's location.
To integrate a CDN into your Rails application, you can use popular CDN providers like Cloudflare, AWS CloudFront, or Fastly. The specific steps for integration will depend on the CDN provider you choose. Typically, you will need to configure your CDN to point to your application's static assets, and update your application's asset URLs to use the CDN domain.
Rails Caching Strategies
Related Article: Ruby on Rails with Bootstrap, Elasticsearch and Databases
Page Caching
Page caching is a technique where the entire HTML output of a page is cached and served directly from the cache, bypassing the application server and database. This can significantly improve the performance of static pages that don't change frequently.
In Rails, you can enable page caching by using the caches_page
method in your controllers. Let's consider an example:
class PagesController < ApplicationController caches_page :home def home # ... end end
In this example, the home
action of the PagesController
is cached, and subsequent requests to the same page will be served directly from the cache.
It's important to note that page caching is not suitable for pages that contain dynamic content, such as user-specific data or forms. In such cases, you can use other caching strategies, such as fragment caching or action caching.
Fragment Caching
Fragment caching is a technique where specific parts of a page are cached and served from the cache, while the rest of the page is generated dynamically. This is useful for pages that have dynamic content but also contain sections that don't change frequently.
In Rails, you can enable fragment caching by using the cache
helper method in your views. Let's consider an example:
<% cache('sidebar') do %> <div id="sidebar"> <!-- Sidebar content --> </div> <% end %>
In this example, the contents of the div
with the id "sidebar" will be cached using the key 'sidebar'. Subsequent requests to the same page will serve the cached fragment directly, improving performance.
You can also specify a time-to-live (TTL) for the cache, after which the fragment will be regenerated. This allows you to balance performance and freshness of the cached content.
Action Caching
Action caching is a technique where the output of an entire controller action is cached and served from the cache, bypassing the application server and database. This is useful for actions that have expensive calculations or database queries and don't change frequently.
In Rails, you can enable action caching by using the caches_action
method in your controllers. Let's consider an example:
class ProductsController < ApplicationController caches_action :index, :show def index @products = Product.all end def show @product = Product.find(params[:id]) end end
In this example, the index
and show
actions of the ProductsController
are cached, and subsequent requests to the same actions will be served directly from the cache.
It's important to note that action caching is not suitable for actions that contain user-specific data or forms. In such cases, you can use other caching strategies, such as fragment caching or low-level caching.
Server-Side Performance Tuning
Related Article: Ruby on Rails with SSO, API Versioning & Audit Trails
Memory Profiling
Profiling memory usage is an important aspect of server-side performance tuning. By analyzing the memory usage of your Rails application, you can identify memory leaks, inefficient memory usage, and optimize your application for better performance.
There are several tools and gems available for memory profiling in Rails, such as:
1. rack-mini-profiler: This gem provides detailed performance profiling information, including memory usage, query timings, and CPU usage. It can be easily integrated into your Rails application for real-time performance analysis.
2. memory_profiler: This gem allows you to profile the memory usage of specific methods or blocks of code in your Rails application. It provides detailed reports on memory allocations, object counts, and memory growth over time.
Caching Strategies
Caching is an effective strategy for improving server-side performance in Ruby. By caching the results of expensive computations or database queries, you can avoid unnecessary work and improve the response time of your application.
In addition to the caching strategies mentioned earlier, Rails provides a low-level caching API that allows you to cache arbitrary data. You can use the Rails.cache
object to store and retrieve data from the cache. Let's consider an example:
# Store data in the cache Rails.cache.write('key', 'value') # Retrieve data from the cache value = Rails.cache.read('key')
In this example, we store the value 'value' in the cache with the key 'key'. Subsequent reads of the same key will retrieve the cached value.
You can also specify a TTL for the cached data, after which it will be considered expired and evicted from the cache. This allows you to balance performance and freshness of the cached data.
Best Practices for Ruby Performance
When it comes to optimizing Ruby performance in a Rails application, there are several best practices to keep in mind:
1. Avoid unnecessary object allocations: Ruby creates objects for almost everything, and excessive object allocations can impact performance. Minimize object allocations by reusing objects, using symbols instead of strings where appropriate, and avoiding unnecessary array or hash creations.
2. Use efficient data structures: Choose the most appropriate data structures for your use case. For example, use sets instead of arrays for membership checks, and use hashes for quick lookups.
3. Optimize loops and iterations: Loops and iterations can be a performance bottleneck. Minimize the number of iterations and avoid unnecessary computations within loops. Consider using built-in Ruby methods like map
, reduce
, and select
for efficient data processing.
4. Be mindful of memory usage: Ruby's garbage collector can have an impact on performance. Minimize memory usage by releasing unnecessary references, avoiding large object allocations, and using efficient data structures.
5. Profile and benchmark your code: Use tools like benchmarking libraries and profilers to identify performance bottlenecks in your code. This will help you focus your optimization efforts on the areas that have the most impact.
Recommended Approach for Server-Side Performance Tuning in Rails
When it comes to server-side performance tuning in Rails, it's important to take a systematic approach to identify and address performance bottlenecks. Here's a recommended approach:
1. Identify performance bottlenecks: Start by profiling your application to identify the areas that have the most impact on performance. Use tools like profilers, benchmarking libraries, and monitoring tools to gather performance data.
2. Analyze the data: Once you have gathered performance data, analyze it to identify the specific areas that are causing performance issues. Look for slow database queries, memory leaks, excessive object allocations, or any other bottlenecks.
3. Optimize database queries: Optimize your ActiveRecord queries by using eager loading, selecting only the required columns, and adding appropriate indexes. Consider denormalization where it makes sense to reduce the number of joins.
4. Use caching strategically: Identify areas of your application that can benefit from caching and implement the appropriate caching strategy. Consider page caching, fragment caching, or action caching based on the nature of your application and the specific use cases.
5. Optimize Ruby code: Follow best practices for Ruby performance, such as minimizing object allocations, using efficient data structures, optimizing loops and iterations, and being mindful of memory usage.
6. Benchmark and iterate: Benchmark your code after making optimizations to measure the impact on performance. Iterate on the optimization process, focusing on the areas that have the most impact.
Related Article: Ruby on Rails with Internationalization and Character Encoding
Common Pitfalls to Avoid in Database Performance in Ruby
When it comes to database performance in Ruby, there are several common pitfalls to avoid:
1. N+1 query problem: The N+1 query problem occurs when you execute multiple queries to fetch associated records, leading to poor performance. Use eager loading to fetch associated records upfront and reduce the number of queries executed.
2. Lack of indexes: Indexes are crucial for efficient database performance. Analyze your query patterns and add appropriate indexes to improve query execution time.
3. Inefficient queries: Avoid fetching unnecessary columns or rows from the database. Use the select
method to fetch only the required columns and use conditions to limit the number of rows fetched.
4. Missing database constraints: Ensure that your database tables have appropriate constraints, such as foreign key constraints and unique constraints. This will improve data integrity and query performance.
5. Not using database-specific features: Different databases have unique features and optimizations. Take advantage of database-specific features like stored procedures, materialized views, and database-specific query optimizations to improve performance.
Tools and Gems for Memory Profiling in Rails
There are several tools and gems available for memory profiling in Rails:
1. rack-mini-profiler: This gem provides detailed performance profiling information, including memory usage, query timings, and CPU usage. It can be easily integrated into your Rails application for real-time performance analysis.
2. memory_profiler: This gem allows you to profile the memory usage of specific methods or blocks of code in your Rails application. It provides detailed reports on memory allocations, object counts, and memory growth over time.
3. ruby-prof: This gem provides a complete suite of profiling tools for Ruby, including memory profiling. It allows you to profile specific methods or blocks of code and provides detailed reports on memory allocations, object counts, and memory growth.
4. derailed_benchmarks: This gem provides a set of benchmarks and profiling tools specifically designed for Rails applications. It allows you to measure the performance of your application and identify bottlenecks.