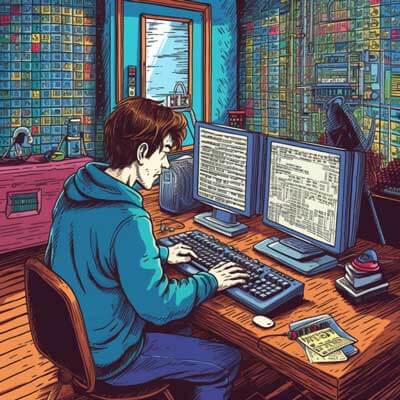
Table of Contents
Installing Rust and Setting Up the Development Environment
To get started with Rust programming for web development, the first step is to install Rust and set up your development environment. This chapter will guide you through the process of installing Rust and configuring your system to start coding in Rust.
Installing Rust
To install Rust, you need to download the Rustup installer, which is a toolchain manager for Rust. Rustup makes it easy to install and manage different versions of Rust on your system.
1. Open your web browser and navigate to the official Rust website: https://www.rust-lang.org/.
2. Click on the "Install" button, which will take you to the Rustup installation page.
3. Follow the instructions provided on the page to download and run the Rustup installer for your operating system.
Once the installer has finished, Rust and its package manager, Cargo, will be installed on your system.
Verifying the Installation
After the installation is complete, you can verify that Rust is installed correctly by opening a terminal or command prompt and running the following command:
$ rustc --version
This command will display the version of Rust installed on your system. If you see the version number, it means Rust has been installed successfully.
You can also verify the installation of Cargo, the Rust package manager, by running the following command:
$ cargo --version
If you see the version number, it means Cargo has been installed correctly.
Setting Up the Development Environment
Now that Rust is installed, it's time to set up your development environment. Rust has excellent tooling support that can enhance your productivity as a web developer.
One popular code editor for Rust is Visual Studio Code (VS Code). You can install the Rust extension for VS Code, which provides features like code completion, syntax highlighting, and debugging support.
To install the Rust extension for VS Code, follow these steps:
1. Open VS Code.
2. Click on the extensions icon in the sidebar (or press Ctrl+Shift+X
).
3. In the search bar, type "Rust" and press Enter.
4. Look for the "Rust" extension by rust-lang and click on the "Install" button.
Once the extension is installed, you can open a Rust project in VS Code and start coding.
Creating Your First Rust Project
To create a new Rust project, you can use Cargo, the Rust package manager. Cargo provides a set of commands to create, build, and manage Rust projects.
To create a new Rust project, open a terminal or command prompt and navigate to the directory where you want to create your project. Then run the following command:
$ cargo new my_project
This command will create a new directory named "my_project" with a basic Rust project structure.
Now you can open the project in your code editor and start writing Rust code for web development.
In this chapter, you learned how to install Rust and set up your development environment for web development. You also learned how to create a new Rust project using Cargo. In the next chapter, we will explore the basics of Rust programming and dive deeper into web development with Rust.
Installing Rust
Before we can start writing Rust code, we need to install the Rust programming language on our system. Rust provides an easy-to-use installer that works on various platforms, including Windows, macOS, and Linux. To install Rust, follow the instructions provided on the official Rust website: https://www.rust-lang.org/tools/install.
Hello, World!
Let's begin our journey with a classic "Hello, World!" example. Open your favorite text editor and create a new file called hello.rs
. In the file, write the following code:
fn main() { println!("Hello, World!"); }
Save the file and open a terminal or command prompt in the same directory as the hello.rs
file. To compile and run the code, enter the following command:
$ rustc hello.rs $ ./hello
You should see the output Hello, World!
printed to the console. Congratulations! You have just written and executed your first Rust program.
Syntax and Variables
Rust has a syntax similar to other curly brace languages like C and C++. The syntax is designed to be expressive and readable, allowing you to write code that is easy to understand and maintain. Let's take a look at a simple example that demonstrates the syntax and variable declaration in Rust:
fn main() { let message = "Hello, Rust!"; // String literal let number: u32 = 42; // Unsigned 32-bit integer println!("{}", message); println!("The answer is: {}", number); }
In this example, we declare two variables: message
and number
. The let
keyword is used to introduce a new variable. The type of the variable can be explicitly specified using a colon :
, as we did with number
. If the type is not explicitly specified, Rust can infer it from the value assigned to the variable.
The println!
macro is used to print the values of the variables. The {}
placeholder is used to indicate where the variable's value should be inserted. The exclamation mark !
indicates that println!
is a macro, rather than a regular function.
Data Types
Rust provides a rich set of data types, including integers, floating-point numbers, Booleans, characters, strings, arrays, tuples, and more. Here's a brief overview of some commonly used data types in Rust:
- **Integers**: Rust provides signed and unsigned integers of different sizes, such as i8
, i16
, i32
, i64
, u8
, u16
, u32
, and u64
.
- **Floating-Point Numbers**: Rust supports single-precision (f32
) and double-precision (f64
) floating-point numbers.
- **Booleans**: The bool
type represents a Boolean value, which can be either true
or false
.
- **Characters**: Rust's char
type represents a Unicode scalar value, denoted by single quotes, e.g., 'A'
.
- **Strings**: Rust's String
type represents a mutable, growable string, while string literals are of the &str
type.
- **Arrays**: Arrays in Rust have a fixed size and contain elements of the same type. They are denoted by square brackets, e.g., [1, 2, 3]
.
- **Tuples**: Tuples are collections of elements of different types. They are denoted by parentheses, e.g., (1, 2, 3)
.
Functions
Functions in Rust are declared using the fn
keyword, followed by the function name, a set of parentheses for parameters, and a return type specified after an arrow ->
. Here's an example of a simple function that adds two numbers and returns the result:
fn add_numbers(a: i32, b: i32) -> i32 { a + b } fn main() { let result = add_numbers(3, 4); println!("The result is: {}", result); }
In this example, the add_numbers
function takes two parameters of type i32
and returns an i32
value. The function body consists of a single expression a + b
, which is automatically returned without using the return
keyword.
Control Flow
Rust provides various control flow constructs, including if
expressions, loop
, while
, for
loops, and match
expressions. Here's an example that demonstrates the usage of some of these constructs:
fn main() { let number = 42; if number < 0 { println!("Negative"); } else if number > 0 { println!("Positive"); } else { println!("Zero"); } let mut count = 0; while count < 5 { println!("Count: {}", count); count += 1; } for i in 0..5 { println!("Value: {}", i); } match number { 0 => println!("Zero"), 1..=10 => println!("Between 1 and 10"), _ => println!("Other"), } }
In this example, we use an if
expression to check the value of number
and print the corresponding message. The while
loop is used to iterate until the count
variable reaches 5, printing the current value at each iteration. The for
loop iterates over a range from 0 to 4, printing the value of i
. Finally, the match
expression matches the value of number
against different patterns and prints the corresponding message.
Rust Syntax
Rust has a syntax that is both expressive and flexible. It is designed to be easy to read and write, while still maintaining the performance benefits of a statically-typed language. Here are a few key aspects of Rust's syntax:
Variables and Constants
Variables in Rust are declared using the let
keyword, followed by the variable name and its type. Rust is a statically-typed language, which means that you need to specify the type of every variable at compile-time. Here's an example of declaring a variable:
let x: i32 = 42;
Constants, on the other hand, are declared using the const
keyword. Unlike variables, constants must have a type annotation and their values cannot be changed. Here's an example of declaring a constant:
const PI: f64 = 3.14159;
Functions
Functions in Rust are declared using the fn
keyword, followed by the function name, arguments, return type, and the function body. Here's an example of declaring a simple function that adds two numbers:
fn add_numbers(x: i32, y: i32) -> i32 { return x + y; }
Control Flow
Rust provides various control flow statements such as if
, else
, for
, and while
loops. Here's an example of an if
statement in Rust:
let number = 42; if number > 0 { println!("The number is positive"); } else if number < 0 { println!("The number is negative"); } else { println!("The number is zero"); }
Data Types in Rust
Rust has a rich set of built-in data types that can be used to store and manipulate values. Here are some of the most commonly used data types in Rust:
Primitive Types
Rust has several primitive types, including integers, floating-point numbers, booleans, characters, and tuples. Here's an example of declaring variables with different primitive types:
let number: i32 = 42; let pi: f64 = 3.14159; let is_true: bool = true; let letter: char = 'a'; let tuple: (i32, f64, bool) = (42, 3.14159, true);
Arrays and Slices
Arrays and slices are used to store a fixed-size sequence of elements of the same type. Arrays have a fixed length, while slices can have a variable length. Here's an example of declaring an array and a slice:
let numbers: [i32; 5] = [1, 2, 3, 4, 5]; let slice: &[i32] = &numbers[1..3];
Strings
Rust's string type, String
, represents a growable, mutable sequence of characters. Here's an example of creating a string:
let greeting: String = String::from("Hello, Rust!");
Declaring Variables
To declare a variable in Rust, we use the let
keyword followed by the variable name. Rust is a statically typed language, which means we must also specify the type of the variable.
Here's an example of declaring a variable of type i32
(32-bit signed integer) and assigning it a value:
let x: i32 = 5;
We can also declare a variable without assigning an initial value. In this case, Rust assigns a default value based on the variable's type. For example:
let y: bool; // Defaults to false let z: Option<i32>; // Defaults to None
Immutable and Mutable Variables
By default, variables in Rust are immutable, meaning their values cannot be changed once assigned. This helps prevent subtle bugs and makes code easier to reason about.
To create a mutable variable, we use the mut
keyword:
let mut count = 0; count = 1; // Valid - count is mutable
Constants
Constants are similar to variables, but their values cannot be changed. They are declared using the const
keyword and must be annotated with a type:
const PI: f32 = 3.14;
Unlike variables, constants can be declared in any scope, including the global scope.
Functions
Functions in Rust are declared using the fn
keyword, followed by the function name, parameters, return type (if any), and body. Here's an example of a basic function that adds two numbers:
fn add(a: i32, b: i32) -> i32 { a + b }
Functions can have multiple parameters and return values, and the return type can be omitted if the function doesn't return anything.
We can call functions by using their name followed by parentheses and passing in the arguments:
let result = add(2, 3);
Managing Control Flow in Rust Programs
Control flow is a fundamental aspect of programming that allows us to determine the order in which statements are executed. In Rust, we have several constructs to manage control flow, including conditional statements and loops.
Conditional Statements
Conditional statements are used to make decisions in our code based on certain conditions. In Rust, we have the if
, else if
, and else
keywords to create conditional branches.
Here's an example that demonstrates the usage of conditional statements:
fn main() { let number = 7; if number < 5 { println!("The number is less than 5"); } else if number == 5 { println!("The number is equal to 5"); } else { println!("The number is greater than 5"); } }
In this example, the program checks if the number
variable is less than 5. If it is, it prints "The number is less than 5". Otherwise, it checks if the number is equal to 5. If it is, it prints "The number is equal to 5". If neither condition is true, it executes the code inside the else
block, which prints "The number is greater than 5".
Loops
Loops allow us to execute a block of code repeatedly until a certain condition is met. Rust provides several loop constructs, including loop
, while
, and for
.
The loop
keyword creates an infinite loop that continues until explicitly stopped:
fn main() { let mut counter = 0; loop { println!("The counter is: {}", counter); counter += 1; if counter == 5 { break; } } }
In this example, the program creates an infinite loop using the loop
keyword. Inside the loop, it prints the value of the counter
variable and increments it by 1. If the counter reaches 5, the break
keyword is used to exit the loop.
The while
keyword allows us to create a loop that continues as long as a certain condition is true:
fn main() { let mut counter = 0; while counter < 5 { println!("The counter is: {}", counter); counter += 1; } }
In this example, the program creates a loop using the while
keyword. As long as the counter
variable is less than 5, it prints the value of the counter and increments it by 1.
The for
keyword is used to iterate over a collection or a range of values:
fn main() { let numbers = [1, 2, 3, 4, 5]; for number in numbers.iter() { println!("The number is: {}", number); } }
In this example, the program creates a loop using the for
keyword. It iterates over each element in the numbers
array and prints its value.
Control Flow Keywords
Rust also provides control flow keywords like break
, continue
, and return
to modify the flow of execution within loops and functions.
The break
keyword is used to exit a loop prematurely:
fn main() { let mut counter = 0; while counter < 10 { if counter == 5 { break; } println!("The counter is: {}", counter); counter += 1; } }
In this example, the program exits the loop when the counter reaches 5 using the break
keyword.
The continue
keyword is used to skip the rest of the current iteration and move to the next one:
fn main() { for number in 1..=5 { if number == 3 { continue; } println!("The number is: {}", number); } }
In this example, the program skips printing the number 3 and continues to the next iteration using the continue
keyword.
The return
keyword is used to exit a function early and return a value:
fn multiply(a: i32, b: i32) -> i32 { if a == 0 || b == 0 { return 0; } a * b }
In this example, the function multiply
returns 0 if either a
or b
is 0. Otherwise, it multiplies a
and b
and returns the result.
Understanding and effectively managing control flow in Rust programs is essential for writing robust and efficient code. With conditional statements and loops, we can create complex logic and handle different scenarios in our web development projects.
Error Handling with the Result Type
Rust uses the Result type to handle recoverable errors. The Result type is an enumeration that has two variants: Ok and Err. The Ok variant represents success and contains the result value, while the Err variant represents failure and contains an error value.
To handle errors using the Result type, you can use the match expression to match the returned Result and handle each variant accordingly. Here's an example:
use std::fs::File; use std::io::Read; fn read_file_contents(file_path: &str) -> Result<String, std::io::Error> { let mut file = File::open(file_path)?; let mut contents = String::new(); file.read_to_string(&mut contents)?; Ok(contents) } fn main() { match read_file_contents("example.txt") { Ok(contents) => println!("File contents: {}", contents), Err(error) => eprintln!("Error reading file: {}", error), } }
In the above example, the read_file_contents
function attempts to open a file and read its contents. If successful, it returns an Ok variant containing the file contents. If an error occurs, it returns an Err variant containing the error.
The main function uses a match expression to handle the returned Result. If the Result is Ok, it prints the file contents. If the Result is Err, it prints the error message.
Propagating Errors with the ? Operator
The ? operator is a shorthand syntax provided by Rust to propagate errors automatically. It can be used inside functions that return a Result type to simplify error handling.
Here's an example that demonstrates the usage of the ? operator:
use std::fs::File; use std::io::{self, Read}; fn read_file_contents(file_path: &str) -> Result<String, io::Error> { let mut file = File::open(file_path)?; let mut contents = String::new(); file.read_to_string(&mut contents)?; Ok(contents) } fn process_file(file_path: &str) -> Result<(), io::Error> { let contents = read_file_contents(file_path)?; // Process the file contents Ok(()) } fn main() { match process_file("example.txt") { Ok(()) => println!("File processed successfully"), Err(error) => eprintln!("Error processing file: {}", error), } }
In the above example, the read_file_contents
function uses the ? operator to propagate any errors that occur when opening and reading the file. The process_file
function also uses the ? operator to propagate errors from read_file_contents
. This simplifies the error handling code, as any errors are automatically propagated up the call stack.
Handling Panics
In some cases, you may encounter unrecoverable errors or unexpected conditions that you want to handle with a panic. A panic is a runtime error that causes the program to abort and display an error message.
To handle panics, Rust provides the panic!
macro. You can use it to panic the program with a custom error message or use the unreachable!
macro to indicate that a particular code path should never be executed.
Here's an example that demonstrates the usage of the panic!
macro:
fn divide(a: i32, b: i32) -> i32 { if b == 0 { panic!("Attempted to divide by zero"); } a / b } fn main() { let result = divide(10, 0); println!("Result: {}", result); }
In the above example, the divide
function panics if the divisor is zero. When the program encounters a panic, it aborts and displays the panic message.
It's important to note that panicking should be used sparingly and only in cases where the program cannot recover from the error condition.
Introduction to Rust's Ownership System
Rust's ownership system is one of its most distinctive and powerful features. It allows you to write safe and efficient code by enforcing strict rules around memory management. In this chapter, we will explore the concept of ownership in Rust and learn how it helps prevent common memory-related bugs like null pointer dereferences and memory leaks.
Understanding Ownership
In Rust, every value has a unique owner. The owner is responsible for deallocating the memory used by the value when it is no longer needed. This ensures that there are no dangling pointers or memory leaks in your code.
Let's take a look at an example to understand how ownership works in Rust:
fn main() { let name = String::from("Alice"); let length = calculate_length(name); println!("The length of the name is {}", length); } fn calculate_length(s: String) -> usize { s.len() }
In this example, we create a String
called name
and pass it to the calculate_length
function. Ownership of the name
value is transferred to the calculate_length
function, which calculates the length of the string and returns it. The ownership is then transferred back to the main
function, where we print the length.
Moving and Copying
When a value is passed to a function or assigned to another variable, ownership is transferred. This is known as moving. After the move, the original variable is no longer valid and cannot be used.
fn main() { let name1 = String::from("Alice"); let name2 = name1; // ownership of name1 is moved to name2 println!("The name is {}", name1); // This will cause a compile-time error }
In this example, the ownership of name1
is moved to name2
, and we cannot use name1
anymore. If we try to use name1
after the move, the Rust compiler will throw a compile-time error.
However, there are some types in Rust that are copied instead of moved. These are types that have a fixed size known at compile-time, such as integers and booleans. When a copy of a value is made, both the original and the copy are valid and can be used independently.
fn main() { let x = 5; let y = x; // x is copied to y println!("x = {}, y = {}", x, y); // This will work fine }
In this example, the value of x
is copied to y
, so both x
and y
are valid and can be used independently.
Borrowing and References
Sometimes, instead of transferring ownership, we want to allow a function to temporarily borrow a value without taking ownership. This can be achieved using references in Rust.
fn main() { let name = String::from("Alice"); let length = calculate_length(&name); // Pass a reference to name println!("The length of the name is {}", length); } fn calculate_length(s: &String) -> usize { s.len() }
In this example, we pass a reference to name
instead of the value itself to the calculate_length
function. The function accepts a reference using the &
symbol, and the reference is borrowed for the duration of the function call. This allows the function to access the value without taking ownership.
Borrowing
Borrowing is the act of temporarily lending a value to another part of your code. In Rust, borrowing is enforced by the ownership system to ensure memory safety. When you borrow a value, you can use it but you cannot modify it. This prevents multiple parts of your code from simultaneously changing the same value, avoiding data races.
There are two types of borrowing in Rust: immutable borrowing and mutable borrowing. Immutable borrowing, denoted by the &
symbol, allows you to read the value but not modify it. Mutable borrowing, denoted by the &mut
symbol, allows you to both read and modify the value.
Let's take a look at an example of borrowing in Rust:
fn main() { let mut x = 5; let y = &x; // Immutable borrowing let z = &mut x; // Mutable borrowing // Do something with y and z }
In this example, we create a mutable variable x
with the value 5. We then borrow it immutably with let y = &x
, which allows us to read the value of x
through y
. Finally, we borrow it mutably with let z = &mut x
, which allows us to both read and modify the value of x
through z
.
References
References are a way to refer to a value without taking ownership of it. They are created by borrowing a value using the &
symbol. References can be used to pass values to functions or to access values within data structures, without actually moving the value.
In web development, references are commonly used when passing data between functions or when working with data structures such as vectors or hash maps. By using references instead of moving values, you can avoid unnecessary copying and improve performance.
Here's an example of using references in Rust:
fn print_message(message: &str) { println!("{}", message); } fn main() { let greeting = "Hello, world!"; print_message(&greeting); }
In this example, we define a function print_message
that takes a reference to a string slice (&str
) as an argument. We then create a variable greeting
with the value "Hello, world!" and pass a reference to it as an argument to print_message
using &greeting
. The function can read the value of greeting
without taking ownership of it.
Defining Structs in Rust
In Rust, a struct is a way to create custom data types that can hold multiple values of different types. It is similar to a class in other programming languages, but without the ability to define methods or inherit from other structs. Structs are useful for organizing related data and can be used to represent objects in a web application.
To define a struct in Rust, you use the struct
keyword followed by the name of the struct and a list of fields with their types. Here's an example of a simple struct representing a user in a web application:
struct User { username: String, email: String, age: u32, }
In this example, we define a struct named User
with three fields: username
, email
, and age
. The fields are of type String
and u32
, which represent a string and an unsigned 32-bit integer, respectively.
You can create an instance of a struct by using the struct's name followed by curly braces and providing values for each field:
let user = User { username: String::from("john_doe"), email: String::from("john@example.com"), age: 25, };
In this code snippet, we create a new User
instance named user
with the given values for each field. Note that we use String::from
to create a new String
instance for the username
and email
fields.
Accessing Struct Fields
Once you have an instance of a struct, you can access its fields using dot notation. Here's an example:
println!("Username: {}", user.username); println!("Email: {}", user.email); println!("Age: {}", user.age);
In this code snippet, we use dot notation to access each field of the user
instance and print its value.
Defining Enums in Rust
An enum in Rust is a type that represents a value that can be one of several possible variants. Enums are useful when you have a fixed set of values that a variable can take, such as different states of a web application.
To define an enum in Rust, you use the enum
keyword followed by the name of the enum and a list of variants. Each variant can optionally have associated data. Here's an example of an enum representing different HTTP methods in a web application:
enum HttpMethod { Get, Post(String), Put(u32), Delete, }
In this example, we define an enum named HttpMethod
with four variants: Get
, Post
, Put
, and Delete
. The Post
variant has an associated String
data, and the Put
variant has an associated u32
data.
You can create an instance of an enum variant by using the enum's name followed by the variant's name and providing any associated data:
let get_method = HttpMethod::Get; let post_method = HttpMethod::Post(String::from("https://example.com")); let put_method = HttpMethod::Put(42); let delete_method = HttpMethod::Delete;
In this code snippet, we create instances of the HttpMethod
enum with different variants. Note that we use String::from
to create a new String
instance for the Post
variant.
Matching Enum Variants
To work with an enum, you often need to match against its variants to perform different actions based on the value. You can use the match
expression in Rust to do this. Here's an example:
fn process_http_method(method: HttpMethod) { match method { HttpMethod::Get => println!("Processing GET request"), HttpMethod::Post(url) => println!("Processing POST request to {}", url), HttpMethod::Put(id) => println!("Processing PUT request with ID {}", id), HttpMethod::Delete => println!("Processing DELETE request"), } }
In this code snippet, we define a function named process_http_method
that takes an HttpMethod
as a parameter. Inside the function, we use the match
expression to match against each variant of the enum and perform different actions based on the value.
To use this function, you can pass an HttpMethod
instance to it:
let method = HttpMethod::Post(String::from("https://example.com")); process_http_method(method);
This code snippet creates an instance of the HttpMethod
enum with the Post
variant and calls the process_http_method
function with this instance.
Traits
Traits in Rust allow us to define shared behavior that can be implemented by different types. They are similar to interfaces in other programming languages. By using traits, we can define a set of methods that a type must implement to be considered as implementing that trait.
To define a trait, we use the trait
keyword followed by the trait name. Inside the trait block, we can define methods that the implementing types should have. Let's take a look at an example:
trait Sound { fn make_sound(&self); } struct Dog; struct Cat; impl Sound for Dog { fn make_sound(&self) { println!("Woof!"); } } impl Sound for Cat { fn make_sound(&self) { println!("Meow!"); } } fn main() { let dog = Dog; let cat = Cat; dog.make_sound(); // Output: Woof! cat.make_sound(); // Output: Meow! }
In this example, we define a Sound
trait with a single method make_sound()
. We then implement this trait for the Dog
and Cat
structs. The make_sound()
method is different for each type, but they both fulfill the contract of the Sound
trait.
Generics
Generics in Rust allow us to write code that can work with multiple types. They provide a way to write functions, structs, and enums that can be used with different types without sacrificing type safety.
To define a generic function, we use angle brackets <T>
after the function name. The T
is a type parameter that represents any type that will be passed to the function. Let's see an example:
fn print_type<T>(value: T) { println!("The type of value is: {:?}", std::any::type_name::<T>()); } fn main() { print_type(42); // Output: The type of value is: i32 print_type("Hello"); // Output: The type of value is: &str print_type(vec![1, 2, 3]); // Output: The type of value is: std::vec::Vec<i32> }
In this example, we define a generic function print_type()
that takes a value of any type T
. Inside the function, we use the std::any::type_name()
function to print the name of the type. We can call this function with different types, and Rust will infer the type for us.
Combining Traits and Generics
Traits and generics can be combined to create even more powerful abstractions. By using traits with generic types, we can write code that is both flexible and reusable.
Let's take a look at an example where we define a trait for a generic data structure called Container
:
trait Container<T> { fn add(&mut self, value: T); fn get(&self) -> Option<&T>; } struct Stack<T> { data: Vec<T>, } impl<T> Container<T> for Stack<T> { fn add(&mut self, value: T) { self.data.push(value); } fn get(&self) -> Option<&T> { self.data.last() } } fn main() { let mut stack = Stack::<i32> { data: vec![] }; stack.add(42); stack.add(123); if let Some(value) = stack.get() { println!("The last value in the stack is: {}", value); } }
In this example, we define a generic trait Container
that has two methods: add()
and get()
. We then implement this trait for the Stack
struct, which internally uses a Vec
to store the data. The type of the data is specified when creating an instance of Stack
.
By combining traits and generics, we can create reusable code that works with different types while enforcing a common behavior.
Creating a Module
To create a module in Rust, we use the mod
keyword followed by the name of the module. Modules can be nested within other modules to create a hierarchical structure. Let's create a simple module called math
that contains some mathematical functions:
// math.rs pub mod math { pub fn add(a: i32, b: i32) -> i32 { a + b } pub fn subtract(a: i32, b: i32) -> i32 { a - b } }
In the example above, we define a module called math
and two functions add
and subtract
within it. The pub
keyword before each function makes them accessible outside of the module.
Using a Module
To use a module in another file, we need to bring it into scope using the use
keyword. Let's create a new file called main.rs
and use the math
module we created earlier:
// main.rs use math::math; fn main() { let result = math::add(5, 3); println!("The result is: {}", result); }
In the example above, we import the math
module using the use
keyword and then call the add
function from the module.
Module Visibility
By default, items within a module are private and can only be accessed within the module itself. To make an item (such as a function or struct) accessible outside of the module, we need to use the pub
keyword. For example:
// math.rs pub mod math { pub fn add(a: i32, b: i32) -> i32 { a + b } fn multiply(a: i32, b: i32) -> i32 { a * b } }
In the example above, the add
function is accessible outside of the module because it is marked as pub
, but the multiply
function is not.
Re-exporting Modules
Sometimes, we want to make a module available through another module without directly exposing its implementation details. We can achieve this by using the pub use
syntax. Let's see an example:
// main.rs pub mod math { pub fn add(a: i32, b: i32) -> i32 { a + b } fn multiply(a: i32, b: i32) -> i32 { a * b } } pub use math::add as math_add; fn main() { let result = math_add(5, 3); println!("The result is: {}", result); }
In the example above, we re-export the add
function from the math
module as math_add
in the main
module. This allows us to use math_add
directly without importing the math
module.
Writing Test Cases
Writing test cases is crucial for maintaining code quality and preventing bugs. In Rust, we can use the built-in testing framework called cargo test
to write and execute tests.
To write a test case, we create a separate module inside our code file and annotate it with the #[cfg(test)]
attribute. Within this module, we define functions with the #[test]
attribute to denote them as test cases.
Let's take a look at an example of a simple test case for a function that adds two numbers:
#[cfg(test)] mod tests { #[test] fn test_add_numbers() { assert_eq!(add_numbers(2, 3), 5); assert_eq!(add_numbers(-1, 1), 0); assert_eq!(add_numbers(10, -5), 5); } }
In this example, we define the test_add_numbers
function and use the assert_eq!
macro to check if the result of add_numbers
is as expected. If the assertions fail, the test will fail, indicating that there might be an issue with the add_numbers
function.
To run the tests, we can use the following command in the terminal:
cargo test
Rust's testing framework provides various assertion macros like assert!
, assert_eq!
, and assert_ne!
to check different conditions and values. These macros help in writing expressive and meaningful test cases.
Debugging Rust Code
Despite our best efforts, bugs can still find their way into our code. When faced with a bug, debugging becomes an invaluable skill. Rust provides a powerful debugging experience with the help of the println!
macro and the Rust debugger, commonly known as gdb
.
The simplest way to debug Rust code is by using the println!
macro to print out the values of variables at different points in the code. By strategically placing these print statements, we can narrow down the source of the bug and identify unexpected values or conditions.
Here's an example of using println!
for debugging:
fn divide_numbers(a: i32, b: i32) -> Option<f64> { if b == 0 { println!("Error: Division by zero!"); return None; } let result = a as f64 / b as f64; println!("Result: {}", result); Some(result) }
In this example, we use println!
to print the result of the division operation. By inspecting the output, we can verify if the division is happening correctly and if the condition for division by zero is being handled appropriately.
Apart from using println!
, we can also use gdb
, a powerful debugger, to step through the code and inspect variables at runtime. gdb
provides a command-line interface to interact with the debugger and offers features like breakpoints, stepping, and variable inspection.
To use gdb
with Rust, we need to compile our code with the debug flag. We can do this using the --debug
flag with cargo build
or cargo run
:
cargo build --debug
Once the code is compiled with debug information, we can use gdb
with the executable produced by Cargo:
gdb target/debug/my_program
With gdb
, we can set breakpoints at specific lines of code, step through the code, inspect variables, and much more. This powerful debugging tool can help us identify and fix complex bugs in our Rust code.
Rust's Package Manager and Dependency Management
Rust, being a modern programming language, has its own package manager called Cargo. Cargo is not only a package manager but also a build system for Rust projects. It helps you manage your project's dependencies, build your project, and run tests. In this chapter, we will explore how to use Cargo effectively for managing dependencies in your Rust web development projects.
Initializing a New Rust Project with Cargo
To start a new Rust project with Cargo, you need to open a terminal or command prompt and navigate to the directory where you want to create your project. Then, run the following command:
cargo new my_project
This will create a new directory called "my_project" with the basic structure for a Rust project. Inside the "my_project" directory, you will find a "Cargo.toml" file, which is the manifest file for your project, and a "src" directory, where you will write your Rust code.
Adding Dependencies to Your Project
To add dependencies to your Rust project, you need to edit the "Cargo.toml" file. Open the "Cargo.toml" file in a text editor and add the desired dependencies under the "dependencies" section. Each dependency should be specified with its name and version number. For example:
[dependencies] actix-web = "3.3.2" tokio = { version = "1", features = ["full"] }
In the above example, we are adding two dependencies: "actix-web" version 3.3.2 and "tokio" version 1 with the "full" feature enabled.
After adding the dependencies, save the "Cargo.toml" file. Now, when you run any Cargo command, it will automatically download and manage the specified dependencies for you.
Updating Dependencies
As you continue working on your project, you may want to update your dependencies to their latest versions. Cargo makes it easy to update dependencies with a single command. Simply open a terminal or command prompt, navigate to your project directory, and run the following command:
cargo update
This will update all the dependencies listed in your "Cargo.toml" file to their latest versions, respecting any version constraints you have specified.
Building and Running Your Project
Cargo provides a convenient way to build and run your Rust project. To build your project, open a terminal or command prompt, navigate to your project directory, and run the following command:
cargo build
This will compile your Rust code and generate an executable binary file inside the "target/debug" directory. You can then run the compiled binary by running the following command:
cargo run
Cargo will take care of compiling your code, resolving dependencies, and running your project.
Running Tests
Cargo also provides built-in support for running tests in your Rust project. To run tests, open a terminal or command prompt, navigate to your project directory, and run the following command:
cargo test
This will compile your tests and run them, displaying the test results in the terminal.
Introduction to Web Development with Rust
Rust is a powerful and modern programming language that provides a safe and efficient way to build web applications. In this chapter, we will explore the basics of web development with Rust, including how to handle HTTP requests, serve static files, and interact with databases.
Handling HTTP Requests
When building web applications, handling HTTP requests is a fundamental task. Rust provides several libraries to handle incoming requests and route them to the appropriate handlers. One popular library is Actix Web.
Actix Web is a high-performance, asynchronous web framework built with Rust. It allows you to define routes and handlers using a declarative syntax. Here's an example of a simple Actix Web server that responds with "Hello, World!" for any incoming request:
use actix_web::{get, App, HttpResponse, HttpServer, Responder}; #[get("/")] async fn index() -> impl Responder { HttpResponse::Ok().body("Hello, World!") } #[actix_web::main] async fn main() -> std::io::Result<()> { HttpServer::new(|| { App::new().service(index) }) .bind("127.0.0.1:8000")? .run() .await }
In this example, the index
function is annotated with #[get("/")]
, which tells Actix Web to handle GET requests to the root URL ("/") with this function. The index
function returns an impl Responder
, which can be any type that implements the Responder
trait. In this case, we return an HttpResponse
with the "Hello, World!" body.
Serving Static Files
Web applications often need to serve static files such as HTML, CSS, and JavaScript. Actix Web provides a middleware called Files
that makes it easy to serve static files from a specified directory. Here's an example that serves files from the "static" directory:
use actix_files::Files; use actix_web::{App, HttpServer}; #[actix_web::main] async fn main() -> std::io::Result<()> { HttpServer::new(|| { App::new() .service(Files::new("/static", "static").show_files_listing()) }) .bind("127.0.0.1:8000")? .run() .await }
In this example, the Files
middleware is mounted at the "/static" URL prefix and serves files from the "static" directory. The show_files_listing
method enables directory listing for easier development.
Interacting with Databases
Most web applications require some form of data storage. Rust has a variety of libraries for interacting with databases, both SQL and NoSQL. One popular library for working with SQL databases is Diesel.
Diesel is a safe and ergonomic way to interact with databases in Rust. It provides a type-safe query builder and a powerful ORM (Object-Relational Mapping) framework. Here's an example of using Diesel to query a PostgreSQL database:
#[macro_use] extern crate diesel; use diesel::prelude::*; use dotenv::dotenv; use std::env; #[derive(Queryable)] struct User { id: i32, name: String, email: String, } fn main() { dotenv().ok(); let database_url = env::var("DATABASE_URL").expect("DATABASE_URL must be set"); let connection = PgConnection::establish(&database_url).expect("Failed to connect to database"); let users = users::table.load::<User>(&connection).expect("Error loading users"); for user in users { println!("{} ({}): {}", user.name, user.email, user.id); } }
In this example, we define a User
struct and use the #[derive(Queryable)]
attribute to automatically generate the necessary code for querying the database. We establish a connection to the database using the DATABASE_URL
environment variable, load all users from the users
table, and print their names, emails, and IDs.
Setting Up a Rust Web Development Project
Setting up a Rust web development project involves a few steps to ensure that you have the necessary tools and dependencies installed. In this chapter, we will guide you through the process of setting up a Rust web development project from scratch.
Step 1: Install Rust
Before you can start developing web applications with Rust, you need to have Rust installed on your machine. If you haven't installed Rust yet, you can do so by following the official installation guide available at https://www.rust-lang.org/tools/install. This guide provides platform-specific instructions for installing Rust on Windows, macOS, and Linux.
Once Rust is installed, you can verify the installation by opening a terminal or command prompt and running the following command:
$ rustc --version
If the command prints the version of Rust installed on your machine, you're ready to move on to the next step.
Step 2: Choose a Web Framework
Rust has several web frameworks available for building web applications. Some popular options include Rocket, Actix, and Warp. Each framework has its own strengths and features, so choose the one that best suits your needs.
For the purpose of this chapter, we will be using Rocket, a web framework that focuses on simplicity and ease of use. You can add Rocket as a dependency to your project by adding the following line to your Cargo.toml
file:
[dependencies] rocket = "0.5.0-rc.1"
Step 3: Create a New Rust Project
To create a new Rust project, open a terminal or command prompt and navigate to the directory where you want to create the project. Then, run the following command:
$ cargo new my_project_name
This command creates a new directory named my_project_name
and initializes it as a Rust project. Replace my_project_name
with the desired name for your project.
Step 4: Configure the Project
After creating the project, navigate to its directory by running the following command:
$ cd my_project_name
Next, open the Cargo.toml
file in a text editor and add the necessary dependencies for your web project. For example, if you're using Rocket, your Cargo.toml
file should look like this:
[dependencies] rocket = "0.5.0-rc.1"
Save the file and exit the text editor.
Step 5: Build and Run the Project
To build and run the project, use the cargo run
command:
$ cargo run
This command compiles the project and starts a local development server. You should see output indicating that the server is running.
Open a web browser and navigate to http://localhost:8000
, or the port specified by your web framework. You should see the default page provided by the framework.
Congratulations! You have successfully set up a Rust web development project. You can now start building your web application using Rust.
In this chapter, we covered the steps necessary to set up a Rust web development project. We installed Rust, chose a web framework, created a new Rust project, configured the project, and built and ran the project. Now you're ready to dive into web development with Rust.
The Hyper Crate
To handle HTTP requests and responses in Rust, we will be using the Hyper crate. Hyper is a fast and robust HTTP library for Rust that provides a high-level API for building HTTP clients and servers.
To get started, add the following dependency to your Cargo.toml
file:
[dependencies] hyper = "0.14"
Next, open your project's main source file and import the necessary modules from Hyper:
use hyper::service::{make_service_fn, service_fn}; use hyper::{Body, Request, Response, Server};
Creating an HTTP Server
To handle incoming HTTP requests, we need to create an HTTP server. The Hyper crate provides a convenient way to create a server using the Server
struct.
#[tokio::main] async fn main() { // Create a new instance of the Hyper server let addr = ([127, 0, 0, 1], 3000).into(); let make_svc = make_service_fn(|_conn| async { Ok::<_, hyper::Error>(service_fn(handle_request)) }); let server = Server::bind(&addr).serve(make_svc); // Start the server and handle any errors if let Err(e) = server.await { eprintln!("server error: {}", e); } }
In the above code, we bind the server to the localhost on port 3000 and define a make_svc
closure that creates a new instance of a service for each incoming connection. The handle_request
function will be responsible for handling each request.
Handling Requests
To handle HTTP requests, we need to define a function that takes a Request
object as input and returns a Response
object. This function will be called for each incoming request.
async fn handle_request(req: Request<Body>) -> Result<Response<Body>, hyper::Error> { // Handle the request and build a response // ... // Return the response Ok(Response::new(Body::from("Hello, World!"))) }
Inside the handle_request
function, we can access the request method, headers, and body to perform any necessary processing. We then construct a Response
object and return it.
Working with Request Data
To access data sent in the request body, we can use the req.into_body().data().await
method, which returns a Result
containing the request body as a Bytes
object.
async fn handle_request(req: Request<Body>) -> Result<Response<Body>, hyper::Error> { // Access the request body let body_bytes = hyper::body::to_bytes(req.into_body()).await?; let body_string = String::from_utf8_lossy(&body_bytes).to_string(); // Process the request body // ... // Return the response Ok(Response::new(Body::from("Hello, World!"))) }
In the above code, we convert the request body to bytes and then convert those bytes into a string. We can then process the body data as needed.
Returning Responses
To send a response back to the client, we construct a Response
object and return it from the handle_request
function.
async fn handle_request(req: Request<Body>) -> Result<Response<Body>, hyper::Error> { // Handle the request and build a response let response_body = "Hello, World!"; let response = Response::new(Body::from(response_body)); // Return the response Ok(response) }
In the above code, we create a response body and then use it to construct a Response
object. We then return this response from the function.
Working with Databases in Rust Web Development
Rust is a powerful and expressive language that is gaining popularity in the web development community. One of the key aspects of web development is working with databases to store and retrieve data efficiently. In this chapter, we will explore how to work with databases in Rust web development.
Choosing a Database
Before diving into the specifics of working with databases in Rust, it's important to choose a database that fits your project requirements. Rust has a growing ecosystem of database libraries that provide support for various database systems. Some popular choices include:
- rust-postgres: A PostgreSQL client library for Rust.
- Diesel: A high-level ORM and query builder for Rust, with support for multiple database backends.
- Sled: An embedded database written purely in Rust, designed for speed and simplicity.
- MongoDB Rust Driver: A MongoDB driver for Rust.
Consider the specific features and performance requirements of your project when choosing a database.
Connecting to a Database
Once you have chosen a database, the first step is to establish a connection. Let's take a look at an example of connecting to a PostgreSQL database using the rust-postgres
library:
use postgres::{Client, NoTls}; fn main() { let mut client = Client::connect("host=localhost user=postgres", NoTls).unwrap(); // Perform database operations... }
The Client::connect
function establishes a connection to the PostgreSQL database running on localhost
with the postgres
user. You can provide additional connection parameters as needed.
Executing Queries
After establishing a connection, you can execute queries to retrieve or modify data in the database. Let's see an example of executing a simple query to fetch all rows from a users
table:
use postgres::{Client, NoTls}; fn main() { let mut client = Client::connect("host=localhost user=postgres", NoTls).unwrap(); for row in client.query("SELECT * FROM users", &[]).unwrap() { let id: i32 = row.get(0); let name: String = row.get(1); let email: String = row.get(2); println!("Id: {}, Name: {}, Email: {}", id, name, email); } }
In this example, we use the query
method of the Client
struct to execute a SQL query that fetches all rows from the users
table. We then retrieve the values of each column for each row using the get
method.
Working with ORM Libraries
If you prefer a more high-level approach to working with databases, Rust has several ORM (Object-Relational Mapping) libraries available. These libraries provide a convenient way to interact with the database using Rust structs and methods.
One popular ORM library for Rust is Diesel
. It provides a powerful query builder and supports multiple database backends. Here's an example of using Diesel
to define a simple users
table and perform CRUD operations:
#[macro_use] extern crate diesel; use diesel::prelude::*; use diesel::sqlite::SqliteConnection; #[derive(Queryable)] struct User { id: i32, name: String, email: String, } fn main() { let connection = SqliteConnection::establish("test.db").unwrap(); let users = users::table.load::<User>(&connection).unwrap(); for user in users { println!("Id: {}, Name: {}, Email: {}", user.id, user.name, user.email); } }
In this example, we define a User
struct using the #[derive(Queryable)]
attribute, which tells Diesel
to generate the necessary code for querying the users
table. We then establish a connection to an SQLite database and fetch all rows from the users
table using the load
method.
Introduction to Authentication and Authorization
Authentication and authorization are two crucial components of web development, ensuring the security and privacy of user data. In this chapter, we will explore how to implement authentication and authorization in Rust, using the powerful libraries and frameworks available.
Understanding Authentication
Authentication is the process of verifying the identity of a user or system. It ensures that the user is who they claim to be before granting access to protected resources. In web development, authentication is commonly performed using credentials such as usernames and passwords.
Implementing Authentication in Rust
To implement authentication in Rust, we can leverage the capabilities of the actix-web
framework along with other libraries. Let's take a look at a simple example:
use actix_web::{web, App, HttpResponse, HttpServer}; async fn login() -> HttpResponse { // Authenticate user credentials // Generate and return an authentication token HttpResponse::Ok().body("Login successful") } async fn protected_resource() -> HttpResponse { // Verify authentication token // Access protected resource HttpResponse::Ok().body("Access granted") } #[actix_web::main] async fn main() -> std::io::Result<()> { HttpServer::new(|| { App::new() .route("/login", web::get().to(login)) .route("/protected", web::get().to(protected_resource)) }) .bind("127.0.0.1:8080")? .run() .await }
In this example, we define two routes: /login
for the authentication process and /protected
for accessing a protected resource. The login
function handles the authentication logic, while the protected_resource
function verifies the authentication token.
Understanding Authorization
Authorization, on the other hand, determines what actions a user can perform after they have been authenticated. It establishes access control rules and ensures that users have the necessary permissions to perform specific actions.
Implementing Authorization in Rust
To implement authorization in Rust, we can use a library like casbin
, which provides a flexible and powerful access control model. Let's see how it can be integrated into our existing code:
use actix_web::{web, App, HttpResponse, HttpServer}; use casbin::prelude::*; async fn protected_resource(enforcer: web::Data<Enforcer>) -> HttpResponse { // Verify authentication token // Verify authorization using Casbin let user = "alice"; let resource = "/protected"; let action = "GET"; if enforcer .enforce((user, resource, action)) .unwrap_or_default() { // Access granted HttpResponse::Ok().body("Access granted") } else { // Access denied HttpResponse::Forbidden().body("Access denied") } } #[actix_web::main] async fn main() -> std::io::Result<()> { // Load Casbin model and policy let model = "path/to/model.conf"; let policy = "path/to/policy.csv"; let enforcer = Enforcer::new(model, policy).unwrap(); HttpServer::new(move || { App::new() .app_data(web::Data::new(enforcer.clone())) .route("/protected", web::get().to(protected_resource)) }) .bind("127.0.0.1:8080")? .run() .await }
In this updated example, we introduce the casbin
library to handle authorization. We define an access control model and policy, and then use the Enforcer
struct to enforce authorization rules. The protected_resource
function now checks if the user has the necessary permissions to access the protected resource.
Setting Up Rocket
Before we can start building APIs with Rocket, we need to set it up in our Rust project. To do this, we add the Rocket dependency to our Cargo.toml
file:
[dependencies] rocket = "0.5"
Next, we need to enable the async-graphql
feature for Rocket. This feature allows us to integrate GraphQL into our APIs. We can add it to the Cargo.toml
file as follows:
[dependencies] rocket = { version = "0.5", features = ["async-graphql"] }
After modifying the Cargo.toml
file, we need to run cargo build
to fetch the dependencies and build our project.
Creating an API Endpoint
To create an API endpoint with Rocket, we need to define a function and annotate it with the #[get]
attribute. This attribute tells Rocket that this function should handle HTTP GET requests. We can then define the route path for our endpoint using the &str
type.
Here's an example of a simple API endpoint that returns a JSON response:
use rocket::serde::json::Json; #[get("/hello")] fn hello() -> Json<&'static str> { Json("Hello, world!") }
In this example, the hello()
function returns a Json<&'static str>
type, which represents a JSON response with a static string message. We use the Json
type provided by Rocket to automatically convert our response into JSON.
Handling Request Parameters
API endpoints often need to handle request parameters. Rocket provides a convenient way to extract and parse these parameters using the FromParam
trait.
Let's say we want to create an API endpoint that takes an integer parameter and returns its square:
#[get("/square/<num>")] fn square(num: i32) -> String { let result = num * num; result.to_string() }
In this example, we define a route path with the <num>
parameter. Rocket automatically parses the parameter as an i32
type and makes it available as a function parameter. We then calculate the square of the number and return it as a String
.
Working with GraphQL
Rocket integrates well with GraphQL through the async-graphql
crate. To use GraphQL in our API, we need to define a GraphQL schema and a resolver.
Here's an example of a simple GraphQL schema and resolver using Rocket:
use rocket::async_graphql::{EmptyMutation, Schema}; #[rocket::main] async fn main() { let schema = Schema::build(QueryRoot, EmptyMutation, EmptySubscription).finish(); rocket::build() .manage(schema) .mount("/", rocket::routes![graphql]) .launch() .await .unwrap(); } #[rocket::get("/graphql?<request..>")] async fn graphql(schema: rocket::State<'_, Schema<QueryRoot, EmptyMutation, EmptySubscription>>, request: rocket::GraphQLRequest) -> rocket::GraphQLResponse { request.into_inner().execute(&schema).await.into() } struct QueryRoot; #[rocket::async_graphql::Object] impl QueryRoot { async fn hello(&self) -> String { "Hello, world!".to_owned() } }
In this example, we define a GraphQL schema using the Schema
type from async-graphql
. We also define a resolver struct QueryRoot
and associate it with the schema using the #[rocket::async_graphql::Object]
attribute.
The graphql()
function is annotated with #[rocket::get("/graphql?<request..>")]
to handle GraphQL requests. It takes the schema as a state parameter and the request as a query parameter. The function executes the request against the schema and returns a GraphQL response.
Deploying Rust Web Applications to Production
Deploying a Rust web application to production involves several steps to ensure that your application runs smoothly and efficiently. In this chapter, we will explore the process of deploying Rust web applications to production environments.
Choosing a Deployment Method
There are several deployment methods available for Rust web applications, and the best choice depends on your specific requirements. Here are some popular options:
1. Self-Contained Executables
Rust allows you to compile your web application into a self-contained executable, which includes all the necessary dependencies. This method simplifies deployment as you can easily distribute and run the executable on the target server. To create a self-contained executable, you can use tools like Cargo and Rust's build system.
2. Docker Containers
Docker is a popular containerization platform that allows you to package your application and its dependencies into a container. This method provides a consistent environment across different servers and simplifies deployment. You can create a Docker image for your Rust web application using a Dockerfile, which specifies the necessary dependencies and instructions for building the image.
Here's an example Dockerfile for a Rust web application:
# Use a Rust base image FROM rust:latest # Set the working directory WORKDIR /app # Copy the source code COPY . . # Build the application RUN cargo build --release # Expose the necessary port EXPOSE 8000 # Start the application CMD ["cargo", "run", "--release"]
3. Cloud Platforms
Cloud platforms like AWS, Google Cloud, and Azure provide managed services for deploying Rust web applications. These platforms offer various deployment options, such as virtual machines, containers, and serverless functions. You can choose the most suitable option based on your requirements and familiarity with the platform.
Configuring and Optimizing the Production Environment
When deploying your Rust web application to production, there are a few important considerations to optimize performance and ensure secure operation:
1. Configuration
Ensure that your application's configuration is properly set up for the production environment. This includes database connections, API keys, and other environment-specific settings. It's recommended to use environment variables to store sensitive information instead of hardcoding them in the codebase.
2. Performance Optimization
Optimize your Rust web application for performance by utilizing caching mechanisms, optimizing database queries, and utilizing asynchronous programming where appropriate. Rust's async/await syntax and libraries like Tokio and Actix can help you leverage the full potential of asynchronous programming.
3. Logging and Monitoring
Implement comprehensive logging and monitoring solutions to track the performance and health of your application in the production environment. Tools like Prometheus, Grafana, and Sentry can help you collect and analyze application logs and metrics.
4. Security Considerations
Take necessary security measures to protect your Rust web application from common vulnerabilities. This includes using HTTPS for secure communication, validating user input, implementing proper authentication and authorization mechanisms, and regularly applying security updates to dependencies.
Continuous Integration and Deployment
Implementing a robust continuous integration and deployment (CI/CD) pipeline is crucial for efficient and reliable deployment of your Rust web application. CI/CD tools like Jenkins, GitLab CI/CD, and Travis CI can help automate the build, test, and deployment processes.
It's recommended to set up automated tests to ensure the stability and correctness of your web application before deploying it to the production environment. These tests can include unit tests, integration tests, and end-to-end tests.
Example 1: Rocket Framework
One popular framework for web development in Rust is Rocket. Rocket is a web framework that allows developers to easily build secure, fast, and reliable web applications. It provides a simple and intuitive API that makes it easy to handle HTTP requests and responses.
Here's a basic example of how to use Rocket to create a simple "Hello, World!" web application:
// main.rs #[macro_use] extern crate rocket; #[get("/")] fn index() -> &'static str { "Hello, World!" } #[launch] fn rocket() -> _ { rocket::build().mount("/", routes![index]) }
In this example, we define a route handler function called index
that returns the string "Hello, World!" when the root URL ("/") is accessed. The #[get("/")]
attribute is used to associate the index
function with the GET HTTP method and root URL.
The rocket
function is the entry point of the Rocket application. It uses the rocket::build()
function to create a new Rocket instance and the mount
method to associate the index
route with the root URL ("/").
Example 2: Diesel ORM
Another powerful tool for web development in Rust is the Diesel ORM (Object-Relational Mapping). Diesel provides a type-safe and efficient way to interact with databases in Rust.
Here's an example of how to use Diesel to perform basic database operations:
// main.rs #[macro_use] extern crate diesel; use diesel::prelude::*; use dotenv::dotenv; use std::env; #[derive(Queryable)] struct User { id: i32, name: String, email: String, } fn main() { dotenv().ok(); let database_url = env::var("DATABASE_URL").expect("DATABASE_URL must be set"); let connection = SqliteConnection::establish(&database_url).expect("Failed to connect to database"); let results = users.load::<User>(&connection).expect("Failed to load users"); for user in results { println!("ID: {}, Name: {}, Email: {}", user.id, user.name, user.email); } }
In this example, we define a User
struct that represents a table in the database. We use the #[derive(Queryable)]
attribute to automatically generate code for querying the User
table.
The main
function establishes a connection to the database using the DATABASE_URL
environment variable. It then uses the load
method to retrieve all users from the User
table and prints their information.
Example 3: Actix Web Framework
Actix is another popular web framework in Rust that is known for its high performance and scalability. It leverages asynchronous programming to handle a large number of concurrent connections efficiently.
Here's a simple example of how to use Actix to create a basic web server:
// main.rs use actix_web::{web, App, HttpResponse, HttpServer}; async fn index() -> HttpResponse { HttpResponse::Ok().body("Hello, World!") } #[actix_web::main] async fn main() -> std::io::Result<()> { HttpServer::new(|| { App::new().route("/", web::get().to(index)) }) .bind("127.0.0.1:8000")? .run() .await }
In this example, we define an asynchronous function called index
that returns an HttpResponse
with the body "Hello, World!".
The main
function creates an HttpServer
instance and uses the App
builder to define routes. In this case, we associate the root URL ("/") with the index
function using the route
method.
Finally, we bind the server to the address "127.0.0.1:8000" and start it using the run
method.
Asynchronous Programming with async/await
Asynchronous programming is essential for building scalable web applications. Rust provides native support for asynchronous programming through the async
and await
keywords. By using these keywords, you can write non-blocking code that efficiently utilizes system resources.
Here's an example of using async
and await
to make an HTTP request using the reqwest
crate:
use reqwest; async fn make_request() -> Result<(), reqwest::Error> { let response = reqwest::get("https://example.com").await?; println!("{}", response.text().await?); Ok(()) }
In this example, the make_request
function is marked as async
, allowing us to use await
inside it. The await
keyword suspends the execution of the function until the awaited future completes, allowing other tasks to run in the meantime.
Error Handling with Result and Option
Error handling is a crucial aspect of any web application. Rust provides two built-in types, Result
and Option
, for handling errors and optional values, respectively.
The Result
type represents either a successful value (Ok
) or an error (Err
). By using the ?
operator, you can conveniently propagate errors up the call stack. Here's an example:
use std::fs::File; use std::io::Read; fn read_file() -> Result<String, std::io::Error> { let mut file = File::open("example.txt")?; let mut contents = String::new(); file.read_to_string(&mut contents)?; Ok(contents) }
In this example, the ?
operator is used to propagate any errors that occur during file manipulation operations.
The Option
type represents an optional value that can either be Some(value)
or None
. It is often used to handle cases where a value may or may not be present. Here's an example:
fn divide(a: i32, b: i32) -> Option<f32> { if b == 0 { None } else { Some(a as f32 / b as f32) } }
In this example, the divide
function returns None
if the divisor is zero, indicating that the division is not possible.
Testing with the Rust Testing Framework
Testing is an essential part of developing reliable web applications. Rust provides a built-in testing framework that allows you to write tests for your code.
To write tests in Rust, you can use the #[cfg(test)]
attribute to mark your test functions. Here's an example:
#[cfg(test)] mod tests { #[test] fn test_addition() { assert_eq!(2 + 2, 4); } }
In this example, the test_addition
function is marked with the #[test]
attribute, indicating that it is a test function. The assert_eq!
macro is used to assert that the addition operation produces the expected result.
You can run your tests by executing the cargo test
command in your project's root directory.
Security Best Practices
Web security is of utmost importance when developing web applications. Here are some best practices to follow when developing web applications in Rust:
- Validate and sanitize user input to prevent common security vulnerabilities such as SQL injection and cross-site scripting (XSS) attacks.
- Use secure cryptographic algorithms and libraries for handling sensitive data, such as passwords and authentication tokens.
- Implement proper authentication and authorization mechanisms to ensure that only authorized users can access sensitive resources.
- Regularly update dependencies to address any security vulnerabilities in the libraries you use.
- Use secure coding practices, such as avoiding buffer overflows and race conditions, to prevent common security vulnerabilities.