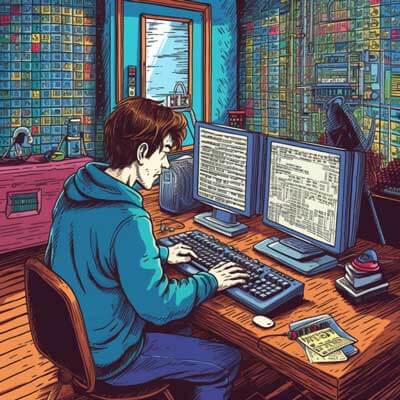
Table of Contents
Why Using Server-Side Rendering
Server-side rendering (SSR) is a technique that enables rendering web pages on the server and sending the fully rendered HTML to the client. This is in contrast to client-side rendering (CSR), where the HTML is initially empty and the web page is built on the client side using JavaScript.
There are several reasons why server-side rendering is important in web development:
1. Improved Performance: SSR improves the initial loading time of a web page. By rendering the HTML on the server, the browser can display the content to the user faster, even before the JavaScript and other assets are downloaded and executed.
2. Search Engine Optimization (SEO): Search engines often struggle with indexing JavaScript-heavy web applications. By providing pre-rendered HTML to search engines, SSR can improve the discoverability and ranking of your web pages.
3. Accessibility: SSR ensures that the content of your web page is available to users with limited or no JavaScript support. This is especially important for users with disabilities who rely on assistive technologies.
4. Social Media Sharing: When you share a link on social media platforms, they typically generate a preview of the shared content. SSR allows the preview to display the actual content of the web page, rather than just a loading spinner.
Now let's explore how to integrate React.js with Ruby on Rails to achieve server-side rendering.
Related Article: How to Implement Hover State in ReactJS with Inline Styles
Integrating React.js with Ruby on Rails
Ruby on Rails (Rails) is a popular web application framework that follows the Model-View-Controller (MVC) architectural pattern. React.js is a JavaScript library for building user interfaces. While Rails traditionally uses server-side rendering, it is possible to integrate React.js into a Rails application to leverage its useful client-side rendering capabilities.
To integrate React.js with Rails, you can follow these steps:
1. Set up a new Rails project or use an existing one.
2. Install the necessary dependencies. You will need to install both React.js and the React-Rails gem, which provides integration between Rails and React.js.
3. Create a new React component using JSX syntax. JSX allows you to write HTML-like code within your JavaScript files.
4. Use the React-Rails gem to render the React component on the server side. This involves creating a new controller action that renders the component to HTML.
5. Update your views to include the server-rendered React component. You can use the react_component
helper provided by the React-Rails gem to include the component in your views.
6. Update your JavaScript files to enable client-side rendering of the React component. This typically involves mounting the component to a specific DOM element on the client side.
Let's take a look at an example to illustrate the integration process.
First, install the React-Rails gem by adding it to your Gemfile and running the bundle install
command:
gem 'react-rails'
Next, generate a new React component using the React-Rails generator:
rails generate react:component HelloWorld greeting:string
This will generate a new file called hello_world.js.jsx
in the app/assets/javascripts/components
directory. Open the file and update it with the following code:
var HelloWorld = React.createClass({ render: function() { return ( <div> <h1>Hello, {this.props.greeting}!</h1> </div> ); } });
Now, let's create a new controller action that renders the React component to HTML. Open the corresponding controller file (e.g., app/controllers/welcome_controller.rb
) and add the following code:
class WelcomeController < ApplicationController def index @greeting = 'World' render react_component: 'HelloWorld', props: { greeting: @greeting } end end
In this example, we pass the @greeting
variable to the React component as a prop.
Finally, update your view file (e.g., app/views/welcome/index.html.erb
) to include the server-rendered React component:
<div id="hello-world"> <%= react_component 'HelloWorld', props: { greeting: @greeting } %> </div>
You will also need to update your JavaScript file (e.g., app/assets/javascripts/application.js
) to mount the React component on the client side:
//= require react //= require react_ujs //= require components $(document).ready(function() { ReactRailsUJS.mountComponents(); });
With these changes in place, when you visit the corresponding route in your Rails application, you should see the server-rendered React component with the appropriate greeting.
Now that we have integrated React.js with Ruby on Rails, let's dive into React on Rails, a gem that simplifies the process of server rendering in React.
Understanding React on Rails
React on Rails is a gem that provides seamless integration between React.js and Ruby on Rails. It simplifies the process of server rendering React components within a Rails application.
With React on Rails, you can achieve server-side rendering of React components by following a few simple steps:
1. Install the React on Rails gem by adding it to your Gemfile and running the bundle install
command:
gem 'react_on_rails'
2. Generate a new React component using the React on Rails generator:
rails generate react_on_rails:component HelloWorld greeting:string
This will generate a new file called hello_world.jsx
in the app/javascript/packs/components
directory. Open the file and update it with the following code:
import React from 'react'; const HelloWorld = (props) => { return ( <div> <h1>Hello, {props.greeting}!</h1> </div> ); }; export default HelloWorld;
3. Update your controller to render the React component as a server-rendered view. Open the corresponding controller file (e.g., app/controllers/welcome_controller.rb
) and add the following code:
class WelcomeController < ApplicationController def index @greeting = 'World' render react_component: 'HelloWorld', props: { greeting: @greeting } end end
4. Update your view file (e.g., app/views/welcome/index.html.erb
) to include the server-rendered React component:
<div id="hello-world"> <%= react_component 'HelloWorld', props: { greeting: @greeting } %> </div>
5. Mount the React components on the client side. Open the JavaScript file corresponding to your Rails application's main entry point (e.g., app/javascript/packs/application.js
) and add the following code:
import ReactOnRails from 'react-on-rails'; import HelloWorld from '../components/hello_world'; ReactOnRails.register({ HelloWorld, });
With these changes in place, you should now have server-rendered React components within your Ruby on Rails application.
React on Rails also provides additional features, such as server-side rendering of Redux stores, internationalization support, and more. Be sure to check out the official documentation for more details on how to leverage these features.
Now that we have explored the integration process of React.js with Ruby on Rails and understood React on Rails, let's delve into the benefits of server rendering in React.
Exploring the Benefits of Server Rendering in React
Server rendering in React offers several benefits that can greatly improve the performance and user experience of your web applications. Let's explore some of these benefits in detail.
1. Faster Initial Page Load: With server rendering, the HTML for your web page is generated on the server and sent to the client as a fully rendered page. This means that the user can see and interact with the content of the page much faster, as there is no need to wait for JavaScript to load and execute before rendering the page.
2. Improved SEO: Search engine crawlers have difficulty processing JavaScript-heavy web applications. By providing pre-rendered HTML to search engines, server rendering can improve the discoverability and ranking of your web pages. This can lead to increased organic traffic and better visibility for your website.
3. Enhanced User Experience: Server rendering ensures that the content of your web page is available to users with limited or no JavaScript support. This is particularly important for users who rely on assistive technologies, such as screen readers, as they can access the fully rendered content without any JavaScript dependencies.
4. Social Media Sharing: When you share a link on social media platforms, they often generate a preview of the shared content. Server rendering allows the preview to display the actual content of the web page, rather than just a loading spinner. This can make your shared links more compelling and increase engagement on social media.
5. Reduced Time to Interactive: With server rendering, the initial HTML is already rendered on the server, allowing the user to start interacting with the page immediately. This can significantly reduce the perceived page load time and provide a smoother user experience.
6. Graceful Degradation: In scenarios where JavaScript fails to load or execute, server rendering ensures that the user can still access and interact with the content of the web page. This provides a more robust and reliable experience for users in less-than-ideal network conditions or with older devices.
Now that we have explored the benefits of server rendering in React, let's move on to implementing server rendering in React.
Related Article: Adding a Newline in the JSON Property Render with ReactJS
Implementing Server Rendering in React
Implementing server rendering in React involves a few steps to configure your application and utilize the appropriate libraries. In this section, we will walk through the process of setting up server rendering in a React application using the Next.js framework.
Next.js is a popular React framework that provides built-in server rendering capabilities. It simplifies the process of setting up and configuring server rendering in React applications.
To implement server rendering in React using Next.js, follow these steps:
1. Set up a new Next.js project or use an existing one. You can start a new Next.js project by running the following command:
npx create-next-app my-app
2. Create a new page component in the pages
directory. This will be the entry point for your server-rendered React application. For example, create a file called index.js
in the pages
directory with the following code:
import React from 'react'; const Home = () => { return ( <div> <h1>Hello, world!</h1> </div> ); }; export default Home;
3. Start the development server by running the following command:
npm run dev
4. Open your browser and navigate to http://localhost:3000
. You should see the server-rendered React application with the "Hello, world!" message.
Congratulations! You have successfully implemented server rendering in your React application using Next.js. Next.js takes care of the server rendering process and provides a simple and intuitive API for building server-rendered React applications.
Now that we have implemented server rendering in React, let's compare the differences between client-side and server-side rendering in React.
Differences Between Client-Side and Server-Side Rendering in React
Client-side rendering (CSR) and server-side rendering (SSR) are two different approaches to rendering web pages in React. Understanding the differences between these two approaches is crucial for making informed decisions about which rendering strategy to choose for your React application.
1. Initial Page Load: In CSR, the initial HTML is minimal and often empty, as the web page is built on the client side using JavaScript. This means that the user initially sees a blank page, which is then populated with content as the JavaScript code is executed. In SSR, the server generates the fully rendered HTML, which is sent to the client for immediate display. This results in a faster initial page load, as the user can see the content without waiting for JavaScript to load and execute.
2. Time to Interactive: In CSR, the web page is not fully interactive until the JavaScript code is loaded and executed. This can lead to a delay in interactivity, especially on slower devices or networks. In SSR, the web page is fully interactive from the moment it is displayed, as the server has already rendered the HTML and attached any necessary event handlers. This provides a better user experience, particularly for users with limited network connectivity or older devices.
3. Search Engine Optimization (SEO): Search engines have difficulty indexing JavaScript-heavy web applications, as they often rely on JavaScript to generate content. In CSR, the initial HTML is minimal and lacks meaningful content, which can negatively impact SEO. In SSR, the server generates the fully rendered HTML, which includes all the content and metadata necessary for search engine crawlers to index. This improves the discoverability and ranking of your web pages in search engine results.
4. Code Complexity: CSR typically involves writing more JavaScript code, as the entire web page is built and rendered on the client side. This can result in more complex codebases and longer development cycles. SSR, on the other hand, simplifies the client-side JavaScript code, as the server takes care of rendering the initial HTML. This can lead to cleaner and more maintainable code.
5. Performance Trade-offs: CSR can provide a smoother user experience after the initial page load, as subsequent updates can be handled efficiently by the client-side JavaScript code. SSR, on the other hand, can be slower for subsequent navigations or updates, as the server needs to re-render the HTML for each request. However, this trade-off can be mitigated by using techniques such as incremental server rendering or client-side hydration.
Both client-side and server-side rendering have their own advantages and trade-offs. The choice between the two depends on the specific requirements and constraints of your application. It is also possible to combine both approaches to leverage the benefits of both client-side and server-side rendering.
Now that we have compared client-side and server-side rendering in React, let's discuss the limitations of server rendering in React.
Limitations of Server Rendering in React
While server rendering in React provides several benefits, it also has some limitations that you should be aware of. Understanding these limitations will help you make informed decisions about when and where to use server rendering in your React applications.
1. Increased Server Load: Server rendering requires the server to generate the fully rendered HTML for each request. This can put additional load on the server, especially for complex React applications with many components. It is important to consider the server's capacity and performance when implementing server rendering.
2. Slower Subsequent Navigations: Server rendering is typically slower for subsequent navigations, as the server needs to re-render the HTML for each request. This can result in a slower user experience compared to client-side rendering, where subsequent navigations can be handled more efficiently by the client-side JavaScript code.
3. Limited Client-Side Interactivity: Server rendering provides a fully interactive web page from the moment it is displayed. However, once the initial HTML is sent to the client, subsequent interactivity relies on client-side JavaScript execution. This means that certain interactions, such as animations or real-time updates, may not be as smooth or performant as with client-side rendering.
4. Increased Network Traffic: Server rendering requires sending the fully rendered HTML to the client, which can increase the amount of data transferred over the network compared to client-side rendering. This can have an impact on the overall performance and user experience, particularly for users with limited network connectivity.
5. Limited Component Reusability: Server rendering requires components to be rendered on the server, which may introduce limitations on component reusability. Some components may have dependencies on the client-side environment, such as the window or document objects, which are not available on the server. It is important to carefully design and test your components to ensure they can be rendered both on the server and the client.
Despite these limitations, server rendering in React can still provide significant benefits in terms of improved performance, SEO, accessibility, and user experience. It is important to evaluate the specific requirements and constraints of your application before deciding whether to use server rendering.
Now that we have discussed the limitations of server rendering in React, let's explore how to use server rendering with React and Ruby on Rails.
Using Server Rendering with React and Ruby on Rails
Using server rendering with React and Ruby on Rails involves integrating React.js into your Rails application and configuring the necessary components to enable server rendering.
To use server rendering with React and Ruby on Rails, you can follow these steps:
1. Install the necessary dependencies. You will need to install both React.js and the React-Rails gem, which provides integration between Rails and React.js. Add the following gems to your Gemfile and run the bundle install
command:
gem 'react-rails' gem 'webpacker'
2. Configure the React-Rails gem. Run the following command to generate the necessary configuration files:
rails generate react:install
This command will generate a config/webpacker.yml
file and a config/initializers/react_on_rails.rb
file.
3. Create a new React component. You can use the React-Rails generator to create a new React component. For example, run the following command to generate a new component called HelloWorld
:
rails generate react:component HelloWorld greeting:string
This will generate a new file called hello_world.js.jsx
in the app/javascript/components
directory. Open the file and update it with your desired React component code.
4. Render the React component on the server side. Open the controller action that corresponds to the route where you want to render the React component. Use the react_component
helper provided by the React-Rails gem to render the React component on the server side. For example, you can render the HelloWorld
component with the following code:
def index @greeting = 'Hello, World!' render react_component: 'HelloWorld', props: { greeting: @greeting } end
5. Update your view file to include the server-rendered React component. Use the react_component
helper in your view file to include the server-rendered React component. For example:
<%= react_component 'HelloWorld', props: { greeting: @greeting } %>
6. Update your JavaScript files to enable client-side rendering of the React component. Add the necessary JavaScript files and dependencies to your application. For example, you can use Webpacker to bundle your JavaScript files and import the necessary React components. Make sure to mount the React component on the client side. For example:
import ReactOnRails from 'react-on-rails'; import HelloWorld from '../components/hello_world'; ReactOnRails.register({ HelloWorld, });
With these changes in place, your Rails application should be set up to use server rendering with React. When you visit the corresponding route in your Rails application, you should see the server-rendered React component with the appropriate props.
Now that we have explored how to use server rendering with React and Ruby on Rails, let's discuss how to determine the necessity of server-side rendering for your React.js app.
Related Article: How to Integrate UseHistory from the React Router DOM
Determining the Necessity of Server-Side Rendering for Your React.js App
Determining whether server-side rendering (SSR) is necessary for your React.js app depends on various factors, such as the nature of your application, its performance requirements, and the specific use cases you are targeting.
Here are some considerations to help you determine the necessity of server-side rendering for your React.js app:
1. Performance Requirements: If your application requires fast initial page load times and a smooth user experience, server-side rendering can be beneficial. SSR allows the browser to display the content to the user faster, even before the JavaScript and other assets are downloaded and executed. This can improve perceived performance and user engagement.
2. Search Engine Optimization (SEO): If your application relies heavily on organic search traffic, server-side rendering can improve its discoverability and ranking in search engine results. Search engines often struggle with indexing JavaScript-heavy web applications. By providing pre-rendered HTML to search engines, SSR can make your web pages more accessible and SEO-friendly.
3. Accessibility: If accessibility is a priority for your application, server-side rendering can ensure that the content is available to users with limited or no JavaScript support. SSR allows the content to be rendered on the server and sent as fully rendered HTML to the client, making it accessible to assistive technologies and users with disabilities.
4. Social Media Sharing: If your application relies on social media sharing for marketing or user engagement, server-side rendering can provide a better user experience when sharing links on social media platforms. SSR ensures that the social media preview displays the actual content of the web page, rather than just a loading spinner or placeholder.
5. Complexity of User Interactions: If your application includes complex user interactions, such as real-time updates or animations, server-side rendering alone might not be sufficient. Client-side rendering (CSR) is better suited for handling such interactions. In this case, you can consider using a hybrid approach, where some parts of the application are server-rendered and others are client-rendered.
6. Development Effort and Maintenance: Implementing server-side rendering in React.js can require additional development effort and maintenance compared to client-side rendering. SSR introduces additional complexity and considerations, such as handling server-side state, ensuring component reusability, and managing server load. It is important to evaluate the trade-offs and consider whether the benefits outweigh the additional complexity.
Ultimately, the necessity of server-side rendering for your React.js app depends on your specific requirements and goals. It is important to carefully evaluate the benefits and limitations of SSR and consider the impact on performance, SEO, accessibility, and user experience.
Now that we have discussed determining the necessity of server-side rendering for your React.js app, let's move on to best practices for server rendering in React.
Best Practices for Server Rendering in React
Server rendering in React can offer significant benefits, but it also introduces additional considerations and best practices to ensure optimal performance and user experience. Here are some best practices to follow when implementing server rendering in React:
1. Keep Server-Side Rendering Lightweight: Server rendering can put additional load on the server, especially for complex React applications with many components. To ensure optimal performance, try to keep the server-side rendering process as lightweight as possible. Avoid rendering unnecessary components or data on the server side.
2. Minimize Server-Side State: Server-side rendering should ideally be stateless, meaning that the server does not maintain any application state. Instead, the server renders the initial HTML based on the provided props and data. Keeping the server-side stateless makes it easier to scale and cache the server-rendered HTML.
3. Optimize Network Requests: Server rendering often involves making network requests to fetch data for the initial render. To minimize the impact on performance, optimize these network requests. Use techniques such as data prefetching or server-side data fetching to ensure that the necessary data is available when rendering the initial HTML.
4. Handle Client-Side Hydration: After the server renders the initial HTML, it is important to handle the client-side hydration process. Hydration refers to the process of attaching event handlers and re-rendering the React components on the client side. Make sure to handle client-side hydration properly to ensure a seamless transition from the server-rendered HTML to the client-rendered React components.
5. Ensure Component Reusability: When implementing server rendering in React, it is important to ensure that your components are reusable both on the server and the client. Avoid introducing dependencies on client-side APIs or environments, such as the window or document objects. Design your components to be agnostic of the rendering environment.
6. Test and Debug Server Rendering: Server rendering introduces a new layer of complexity to the rendering process. Make sure to thoroughly test and debug server rendering in your React application. Use tools such as React Testing Library or Jest to write tests for your server-rendered components. Additionally, make use of server-side logging and error handling to identify and resolve issues with server rendering.