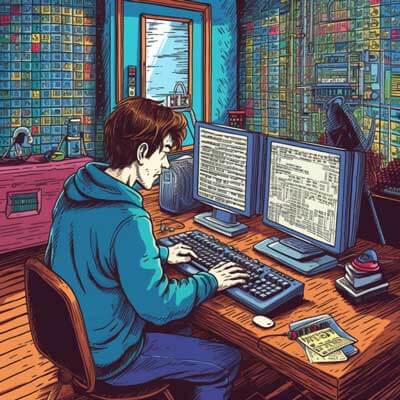
Table of Contents
How to fix the 'TypeError: _ is not defined' error
When working with ReactJS, you may encounter the error message "TypeError: _ is not defined". This error typically occurs when you are trying to use a variable or function that has not been defined or imported correctly. To fix this error, follow these steps:
1. Check for typos: Double-check the spelling and case sensitivity of the variable or function name. Make sure you are using the exact same name as defined.
2. Import the module or file: If the variable or function is defined in another module or file, make sure you have imported it correctly. Use the import statement to bring in the necessary code. Here is an example:
// File: utils.js export const addNumbers = (a, b) => { return a + b; } // File: App.js import { addNumbers } from './utils'; console.log(addNumbers(2, 3)); // Output: 5
In the above example, we define the addNumbers
function in a separate utils.js
file and import it in App.js
using the correct path. This way, we can use the addNumbers
function in our App
component without any errors.
3. Ensure proper scope: If the variable or function is defined within a specific scope, make sure you are accessing it from the correct scope. For example, within a class component, you need to use the this
keyword to access class methods and properties.
class MyComponent extends React.Component { myMethod() { console.log(this.props); // Accessing props within the class method } render() { return ( <div> <button onClick={this.myMethod}>Click me</button> </div> ); } }
In the above example, the myMethod
class method can access the props
using this.props
. Make sure you are using the correct scope to access the variable or function.
Related Article: Exploring Buffer Usage in ReactJS
What does 'undefined' mean
In JavaScript, the value undefined
represents a variable that has been declared but has not been assigned a value. It is one of the primitive data types in JavaScript. When you see undefined
in your code or console, it means that the variable or property does not have a defined value.
Here are some scenarios where undefined
can occur:
1. Declared but not assigned: If you declare a variable without assigning a value to it, the variable will have the value undefined
by default.
let myVariable; console.log(myVariable); // Output: undefined
In the above example, myVariable
is declared but not assigned a value, so its value is undefined
.
2. Missing function arguments: If you call a function with fewer arguments than expected, the missing arguments will have the value undefined
.
function greet(name) { console.log(`Hello, ${name}!`); } greet(); // Output: Hello, undefined!
In the above example, the greet
function expects an argument name
, but when we call it without any arguments, the name
parameter is undefined
.
3. Non-existent object properties: When you try to access a property that does not exist on an object, the result will be undefined
.
const person = { name: 'John', age: 30 }; console.log(person.location); // Output: undefined
In the above example, the person
object does not have a location
property, so accessing it will result in undefined
.
It's important to handle undefined
values in your code to prevent potential errors or unexpected behavior. You can use conditional statements or optional chaining to handle cases where a value may be undefined
.
Why am I getting a 'null is not an object' error
The error message "null is not an object" typically occurs when you try to access a property or method on a variable that is null
. In JavaScript, null
is a special value that represents the intentional absence of any object value. It is different from undefined
, which represents the absence of a value.
To fix this error, you need to ensure that the variable is not null
before accessing its properties or methods. You can use conditional statements or optional chaining to handle cases where a variable may be null
.
Here is an example of how this error can occur and how to fix it:
const person = null; console.log(person.name); // Error: TypeError: null is not an object // Fix: Check if person is not null before accessing its properties if (person !== null) { console.log(person.name); }
In the above example, we try to access the name
property of the person
variable, which is null
. This results in a TypeError
because null
is not an object and does not have any properties. To fix this, we can check if the person
variable is not null
before accessing its properties.
It's important to handle null
values in your code to prevent potential errors. Always check if a variable is not null
before accessing its properties or methods.
How to declare and use variables
In JavaScript, variables are used to store data that can be accessed and manipulated later in the program. To declare a variable, you use the var
, let
, or const
keyword followed by the variable name.
Here are the different ways to declare and use variables in JavaScript:
1. Using the var
keyword (legacy way):
var age = 30; console.log(age); // Output: 30 age = 40; // You can reassign the value of a variable console.log(age); // Output: 40 var name = 'John'; // You can declare and assign a value in the same line console.log(name); // Output: John
In the above example, we declare a variable age
using the var
keyword and assign it the value 30
. We can later reassign the variable to a different value. We can also declare and assign a value in the same line.
2. Using the let
keyword (recommended for block-scoped variables):
let age = 30; console.log(age); // Output: 30 age = 40; console.log(age); // Output: 40 let name = 'John'; console.log(name); // Output: John
In the above example, we use the let
keyword to declare the variable age
. The behavior is similar to var
, but let
is block-scoped, meaning it is only accessible within its block or nested blocks. This helps prevent unintended variable hoisting and reduces the risk of naming conflicts.
3. Using the const
keyword (recommended for constants):
const age = 30; console.log(age); // Output: 30 // Error: Cannot reassign a constant variable age = 40; const name = 'John'; console.log(name); // Output: John
In the above example, we use the const
keyword to declare the constant age
. Constants cannot be reassigned once they are initialized. They are useful for values that should never change, such as mathematical constants or configuration values.
Variables can store different types of data, including numbers, strings, booleans, objects, and arrays. You can perform various operations and manipulations on variables, such as arithmetic operations, concatenation, and accessing object properties.
Related Article: How to Implement Reactstrap in ReactJS
Difference between a function and a component
In ReactJS, both functions and components play crucial roles in building user interfaces. Understanding the difference between a function and a component is important when working with React.
1. Function: In the context of React, a function refers to a JavaScript function that takes in some input (props) and returns a React element. These functions are also known as functional components or stateless components.
Here is an example of a simple function that returns a React element:
function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } // Usage: <Greeting name="John" />
In the above example, the Greeting
function takes in props
as its input and returns a React element, which is a JSX expression. The props
allow us to pass data from the parent component to the child component.
2. Component: In React, a component is a reusable, self-contained piece of code that encapsulates UI logic and can be composed together to build complex user interfaces. Components can be created using classes or functions. Class components are also referred to as stateful components.
Here is an example of a simple class component:
class Greeting extends React.Component { render() { return <h1>Hello, {this.props.name}!</h1>; } } // Usage: <Greeting name="John" />
In the above example, the Greeting
class extends the React.Component
base class and overrides the render
method to return a React element. The props
can be accessed using this.props
within the class.
The main difference between a function and a component lies in their syntax and usage. Functions are simpler and easier to write, especially for simple components. Components, on the other hand, offer more flexibility and additional features, such as lifecycle methods and local state management.
Both functions and components are used to create reusable UI elements in React, and the choice between them depends on the complexity and requirements of the specific component you are building.
How to pass props to a component
In React, props (short for properties) are used to pass data from a parent component to a child component. Props are passed as attributes to the child component when it is being used or rendered. The child component can then access and use the passed props within its own logic.
Here is an example of how to pass props to a component:
function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } // Usage: <Greeting name="John" />
In the above example, we define a functional component Greeting
that takes in props
as its input. We pass the name
prop with the value "John" when using the Greeting
component. The child component can access the prop value using props.name
within its logic and render it as part of the returned JSX.
Props can be any valid JavaScript value, including strings, numbers, booleans, objects, or even functions. Here is an example of passing an object prop:
function UserInfo(props) { return ( <div> <h2>{props.user.name}</h2> <p>Email: {props.user.email}</p> </div> ); } // Usage: const user = { name: 'John Doe', email: 'john.doe@example.com' }; <UserInfo user={user} />
In the above example, we pass an object prop user
with the properties name
and email
. The child component UserInfo
can access the object properties using props.user.name
and props.user.email
within its logic.
Props are read-only and should not be modified within the child component. If you need to change the state or behavior of a component, you should use state instead.
How to manage state
In React, state is used to manage the internal data and behavior of a component. It allows components to keep track of changing data and update the UI accordingly. State is typically used in class components, although functional components with hooks can also manage state.
Here is an example of how to manage state in a class component:
class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } increment() { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.increment()}>Increment</button> </div> ); } } // Usage: <Counter />
In the above example, we define a class component Counter
with an initial state object containing a count
property. We increment the count and update the state using the setState
method. The updated state triggers a re-render of the component, updating the UI with the new count value.
Functional components can also manage state using hooks, specifically the useState
hook. Here is an example:
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); } return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> ); } // Usage: <Counter />
In the above example, we use the useState
hook to declare a state variable count
and a function setCount
to update the state. The increment
function updates the state by calling setCount
with the new count value.
Managing state allows components to respond to user interactions, API responses, or any other changes that require updating the UI. It is an important concept in React and helps create dynamic and interactive user interfaces.
What does the 'render' method do
In React, the render
method is a required method in class components that defines what should be rendered or displayed by the component. It returns a React element or null, which represents the output of the component.
Here is an example of a class component with the render
method:
class Greeting extends React.Component { render() { return <h1>Hello, {this.props.name}!</h1>; } } // Usage: <Greeting name="John" />
In the above example, the Greeting
class component has a render
method that returns a JSX expression <h1>Hello, {this.props.name}!</h1>
. The name
prop is accessed using this.props.name
within the render
method and rendered as part of the returned JSX.
The render
method is automatically called whenever the component needs to be re-rendered, such as when the component's props or state change. It is responsible for determining what the component should display based on its current data.
It's important to note that React components should be pure functions of their props and state, meaning the render
method should not have any side effects or modify the component's state or props directly. Any data manipulation or side effects should be handled in other lifecycle methods or separate functions.
Related Article: How to Style Components in ReactJS
Why am I getting '_ is not defined' error when calling a function
The error message "_ is not defined" typically occurs when you are trying to call a function that has not been defined or imported correctly. This error can happen for various reasons, such as misspelling the function name, not importing the function, or incorrectly referencing the function.
To fix this error, follow these steps:
1. Check for typos: Double-check the spelling and case sensitivity of the function name. Make sure you are using the exact same name as defined.
2. Import the module or file: If the function is defined in another module or file, make sure you have imported it correctly. Use the import statement to bring in the necessary code. Here is an example:
// File: utils.js export function addNumbers(a, b) { return a + b; } // File: App.js import { addNumbers } from './utils'; console.log(addNumbers(2, 3)); // Output: 5
In the above example, we define the addNumbers
function in a separate utils.js
file and import it in App.js
using the correct path. This way, we can call the addNumbers
function in our code without any errors.
3. Ensure proper scope: If the function is defined within a specific scope, make sure you are calling it from the correct scope. For example, within a class component, you need to use the this
keyword to call class methods.
class MyComponent extends React.Component { myMethod() { console.log('Hello!'); } render() { return ( <div> <button onClick={() => this.myMethod()}>Click me</button> </div> ); } }
In the above example, the myMethod
class method is called within the render
method using this.myMethod()
. Make sure you are using the correct scope to call the function.
How to debug 'undefined' errors
When encountering 'undefined' errors in your ReactJS code, it's essential to debug and identify the source of the issue. Here are some techniques to help you debug and fix 'undefined' errors:
1. Check the variable or property: If you are getting an 'undefined' error, start by checking the variable or property that is causing the error. Make sure it is defined and assigned a value before using it.
2. Use console.log: Insert console.log statements in your code to print the values of variables or properties at different stages of execution. This can help you identify where the 'undefined' value is coming from and track down the source of the error.
console.log(myVariable); // Check the value of myVariable
3. Check function arguments: If the 'undefined' error is related to a function, check the arguments you are passing. Make sure they are defined and have the expected values.
4. Step through your code: Use a debugger tool to step through your code line by line and inspect the values of variables and properties at different stages. This can help you pinpoint the exact location where the 'undefined' value is being introduced.
5. Review the code flow: Analyze the flow of your code and check if there are any logical errors or missing assignments that could lead to 'undefined' values. Make sure you are handling asynchronous operations correctly, as they can often introduce 'undefined' errors.
6. Use conditional statements: If you suspect that a variable or property might be 'undefined', use conditional statements to handle such cases. You can use the logical OR operator (||
) to provide a default value in case of 'undefined'.
const myVariable = undefined; const value = myVariable || 'default value'; console.log(value); // Output: default value
Additional Resources
- Passing props to a child component in ReactJS