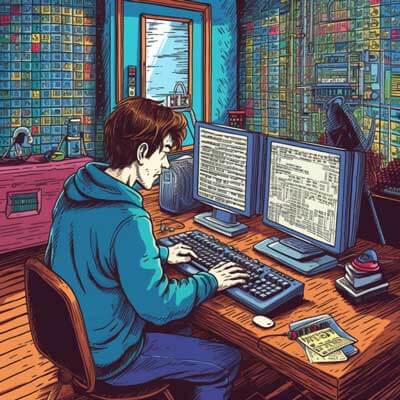
Table of Contents
What is webpack and why is it used?
Webpack is a useful open-source module bundler that is widely used in modern web development. It takes your JavaScript code, along with any other assets such as CSS, images, and fonts, and bundles them together into optimized static assets that can be easily served to the browser.
Webpack is used to simplify the development process by allowing developers to manage and bundle all the dependencies of a project into a single file or a set of files. This makes it easier to organize and maintain complex projects, as well as improve performance by reducing the number of requests needed to load a web page.
One of the main advantages of using webpack is its ability to handle modular JavaScript code. It uses a dependency graph to determine the order in which modules should be bundled, ensuring that all dependencies are resolved correctly. This allows developers to write modular code using popular JavaScript frameworks such as ReactJS and AngularJS.
Webpack also provides a wide range of features and optimizations, such as code splitting, lazy loading, and tree shaking, which can greatly improve the performance and loading time of web applications. Additionally, it supports a variety of loaders and plugins that can be used to preprocess and transform different types of files, such as CSS preprocessors, image optimization, and code minification.
Overall, webpack is a versatile and useful tool that plays a crucial role in modern web development, allowing developers to bundle and optimize their code for production, as well as providing a seamless development experience.
Related Article: Enhancing React Applications with Third-Party Integrations
How to install webpack in ReactJS
To install webpack in a ReactJS project, you need to follow a few simple steps:
Step 1: Create a new ReactJS project or navigate to an existing ReactJS project directory.
Step 2: Open a terminal or command prompt and navigate to the project directory.
Step 3: Run the following command to initialize a new npm project:
npm init -y
This will create a new package.json
file in your project directory.
Step 4: Install webpack and webpack-cli as dev dependencies by running the following command:
npm install webpack webpack-cli --save-dev
This will install the latest versions of webpack and webpack-cli in your project.
Step 5: Create a new file named webpack.config.js
in your project directory. This file will contain the configuration for webpack.
Step 6: Open the webpack.config.js
file in a text editor and add the following code:
const path = require('path'); module.exports = { entry: './src/index.js', output: { path: path.resolve(__dirname, 'dist'), filename: 'bundle.js', }, };
This code sets up the entry point for webpack (./src/index.js
) and specifies the output path and filename (./dist/bundle.js
).
Step 7: Save the webpack.config.js
file and close it.
Step 8: Open the package.json
file in a text editor and add the following code to the "scripts"
section:
"scripts": { "build": "webpack" }
This code adds a new script named "build" that runs the webpack command.
Step 9: Save the package.json
file and close it.
Step 10: Open a terminal or command prompt and run the following command to build your ReactJS project using webpack:
npm run build
Webpack will bundle your code and generate the output file in the specified output path.
Congratulations! You have successfully installed webpack in your ReactJS project.
The role of npm in webpack installation
npm (Node Package Manager) plays a crucial role in the installation and management of webpack in a ReactJS project. It is the default package manager for Node.js and is used to install, manage, and update third-party packages and dependencies.
When installing webpack in a ReactJS project, npm is used to download the webpack and webpack-cli packages from the npm registry. These packages are specified as dev dependencies in the project's package.json
file, which allows npm to manage and track them.
npm also provides a mechanism for defining and executing scripts in the package.json
file. In the case of webpack, a script named "build" is added to the package.json
file, which runs the webpack command to build the project.
Overall, npm plays a crucial role in the installation and management of webpack in a ReactJS project, providing a convenient and reliable way to install and manage dependencies and scripts.
Creating a webpack configuration file in ReactJS
To configure webpack in a ReactJS project, you need to create a webpack configuration file. This file defines various settings and options for webpack, such as the entry point, output path, loaders, and plugins.
Here is an example of a basic webpack configuration file for a ReactJS project:
const path = require('path'); module.exports = { entry: './src/index.js', output: { path: path.resolve(__dirname, 'dist'), filename: 'bundle.js', }, };
In this example, the configuration file sets up the entry point for webpack (./src/index.js
) and specifies the output path and filename (./dist/bundle.js
).
You can customize the configuration file based on your project's requirements. For example, you can add loaders to preprocess different types of files, such as CSS or images, or add plugins to perform additional optimizations.
Once you have created the webpack configuration file, you can run webpack with the --config
option to specify the path to the configuration file. For example:
webpack --config webpack.config.js
This will use the specified configuration file to build your ReactJS project.
Creating a webpack configuration file allows you to customize and fine-tune webpack based on your project's specific needs, providing a flexible and useful tool for managing and bundling your code.
Related Article: Implementing HTML Templates in ReactJS
Understanding loaders in webpack and their usage in ReactJS
Loaders are a key feature of webpack that allow you to preprocess and transform different types of files before they are included in the bundle. They are applied to files as they are loaded by webpack and can perform tasks such as transpiling JavaScript, compiling CSS, and optimizing images.
In a ReactJS project, loaders are commonly used to preprocess JSX files and transpile them into plain JavaScript that can be understood by the browser. This is typically done using the babel-loader
and the Babel transpiler.
To use loaders in webpack, you need to install them as dependencies in your project and configure them in the webpack configuration file. Here is an example of how to install and configure babel-loader
in a ReactJS project:
Step 1: Install babel-loader
and other required Babel dependencies as dev dependencies in your project:
npm install babel-loader @babel/core @babel/preset-react --save-dev
Step 2: Open the webpack.config.js
file and add the following code to the module rules section:
module: { rules: [ { test: /\.jsx?$/, exclude: /node_modules/, use: { loader: 'babel-loader', options: { presets: ['@babel/preset-react'], }, }, }, ], },
In this example, the babel-loader
is added as a rule to the module section of the webpack configuration. It specifies that the loader should be applied to files with the .jsx
or .js
extension, excluding files in the node_modules
directory. The loader is configured with the @babel/preset-react
preset, which enables JSX transpilation.
Once you have configured the loader, you can use JSX syntax in your ReactJS components and webpack will automatically apply the loader to preprocess the files.
Loaders in webpack are highly customizable and can be used for a wide range of tasks, such as preprocessing CSS, optimizing images, or even running custom scripts. By using loaders, you can extend the capabilities of webpack and customize the build process to suit your project's needs.
Exploring plugins in webpack and their usage in ReactJS
Plugins are another important feature of webpack that allow you to perform additional tasks and optimizations during the build process. They can be used to manipulate the bundle, modify or replace chunks, and apply various optimizations to the output.
In a ReactJS project, plugins are commonly used to perform tasks such as code minification, environment-specific configurations, and code splitting. Some commonly used plugins in ReactJS projects include html-webpack-plugin
, uglifyjs-webpack-plugin
, and dotenv-webpack
.
To use plugins in webpack, you need to install them as dependencies in your project and configure them in the webpack configuration file. Here is an example of how to install and configure the html-webpack-plugin
in a ReactJS project:
Step 1: Install html-webpack-plugin
as a dev dependency in your project:
npm install html-webpack-plugin --save-dev
Step 2: Open the webpack.config.js
file and add the following code at the top of the file to import the html-webpack-plugin
:
const HtmlWebpackPlugin = require('html-webpack-plugin');
Step 3: Add the following code to the plugins section of the webpack configuration:
plugins: [ new HtmlWebpackPlugin({ template: './public/index.html', }), ],
In this example, the html-webpack-plugin
is added as a plugin to the webpack configuration. It is configured with the template
option, which specifies the path to the HTML template file used to generate the output HTML file.
Once you have configured the plugin, webpack will automatically generate an HTML file based on the specified template file, including the generated bundle file as a script tag.
Plugins in webpack provide a wide range of functionality and can be used to perform various tasks, such as generating dynamic HTML files, optimizing assets, and injecting environment variables. By using plugins, you can extend the capabilities of webpack and customize the build process to suit your project's needs.
Specifying the entry point in webpack for ReactJS
The entry point is an important concept in webpack that specifies the starting point for building the dependency graph of a project. It is the file or files that webpack uses to begin bundling the code and resolving dependencies.
In a ReactJS project, the entry point typically refers to the main JavaScript file that initializes the React application. This file is often named index.js
or app.js
and is located in the src
directory of the project.
To specify the entry point in webpack for a ReactJS project, you need to configure the entry
option in the webpack configuration file. Here is an example:
module.exports = { entry: './src/index.js', // other configuration options... };
In this example, the entry
option is set to './src/index.js'
, which specifies that the index.js
file in the src
directory is the entry point for webpack.
If your ReactJS project has multiple entry points, you can specify them as an object with multiple key-value pairs. This allows you to create multiple bundles for different parts of your application. Here is an example:
module.exports = { entry: { main: './src/index.js', vendor: './src/vendor.js', }, // other configuration options... };
In this example, two entry points are specified: './src/index.js'
for the main application code and './src/vendor.js'
for third-party libraries or dependencies.
Specifying the entry point in webpack is crucial for building a ReactJS project, as it determines where webpack starts bundling the code and resolving the dependencies. By correctly configuring the entry point, you can ensure that your ReactJS application is built correctly and all the required modules are included in the bundle.
Defining the output path in webpack for a ReactJS project
The output path in webpack specifies the directory where webpack should generate the output files. These files are the result of the bundling process and include the bundled JavaScript code, CSS stylesheets, and any other assets used in the project.
In a ReactJS project, the output path is typically set to a directory named dist
(short for distribution) or build
in the project's root directory. This directory is often ignored by version control systems and contains the final build artifacts that can be deployed to a web server.
To define the output path in webpack for a ReactJS project, you need to configure the output
option in the webpack configuration file. Here is an example:
const path = require('path'); module.exports = { entry: './src/index.js', output: { path: path.resolve(__dirname, 'dist'), filename: 'bundle.js', }, // other configuration options... };
In this example, the output
option is configured with the path
and filename
options. The path
option specifies the absolute path to the output directory, using the path.resolve
method to resolve the path relative to the current directory. The filename
option specifies the name of the output file.
Defining the output path in webpack is important for organizing and managing the build artifacts of a ReactJS project. By specifying a separate output directory, you can keep the source code separate from the generated output files, making it easier to deploy and distribute the project.
Related Article: The Mechanisms Behind ReactJS’s High-Speed Performance
Understanding modules in webpack and their definition in ReactJS
Modules are a fundamental concept in webpack that allow you to organize and bundle your code into individual units of functionality. A module can be any file or resource that is part of your project, such as JavaScript files, CSS stylesheets, images, or fonts.
In a ReactJS project, modules are commonly used to represent individual components or functional units of the application. Each React component can be considered a module, which can include its own JavaScript code, CSS styles, and other related assets.
Webpack uses a dependency graph to determine the order in which modules should be bundled, ensuring that all dependencies are resolved correctly. This allows you to write modular code using popular JavaScript frameworks such as ReactJS, AngularJS, or Vue.js.
To define modules in a ReactJS project, you need to use the appropriate syntax and conventions of the framework. For example, in ReactJS, you can define a module by creating a new file that exports a React component.
Here is an example of a simple React component defined as a module:
// src/App.js import React from 'react'; function App() { return <div>Hello, World!</div>; } export default App;
In this example, the App
component is defined as a module by creating a new file named App.js
in the src
directory. The component is exported using the export default
syntax, allowing it to be imported and used in other parts of the application.
Once you have defined modules in your ReactJS project, webpack will analyze the dependencies between modules and bundle them together into a single file or a set of files. This allows you to organize and manage your code in a modular and maintainable way, making it easier to develop and maintain complex projects.
Using modules in webpack and ReactJS is a useful technique that allows you to write modular and reusable code, improving the maintainability and scalability of your application.
Using react-scripts instead of manual webpack configuration
React-scripts is a set of pre-configured scripts and tools that provide a zero-configuration development environment for ReactJS projects. It is a popular choice for bootstrapping React applications and comes bundled with Create React App, a popular React project generator.
React-scripts abstracts away the complexity of configuring webpack and other build tools, allowing developers to focus on writing code and building their applications. It provides a number of built-in features and optimizations, such as hot module replacement, code splitting, and automatic linting.
To use react-scripts in a ReactJS project, you need to install it as a dev dependency in your project:
npm install react-scripts --save-dev
Once installed, you can use the pre-configured scripts provided by react-scripts to start, build, and test your ReactJS application. For example:
- To start the development server and run your application in development mode, run the following command:
npm start
This will start the development server and open your application in the browser. Any changes you make to your code will be automatically reloaded in the browser.
- To build your application for production, run the following command:
npm run build
This will create a production-ready build of your application in the build
directory.
- To run the tests for your application, run the following command:
npm test
This will run the test suite and provide feedback on any failures or errors.
Using react-scripts eliminates the need for manual webpack configuration, as all the necessary build configurations are handled by react-scripts behind the scenes. This allows you to quickly set up a development environment for your ReactJS project without having to worry about the complexities of webpack.
However, it's important to note that react-scripts provides a set of pre-configured options and may not cover all the advanced use cases and customizations that can be achieved with manual webpack configuration. If you require more fine-grained control over the webpack configuration, you may need to eject from react-scripts and manually configure webpack.
Additional Resources
- Getting Started with React and Webpack