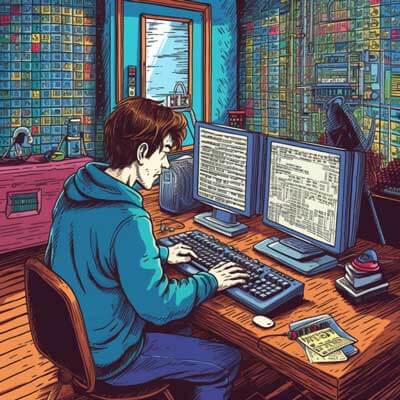
Table of Contents
In this article, we will explore the step-by-step process to deploy ReactJS apps, covering various deployment methods and platforms. We will also delve into the tools and technologies that play a crucial role in the deployment process, such as create-react-app, Webpack, Babel, npm, and yarn. Whether you are a seasoned ReactJS developer or just getting started, this comprehensive guide will help you navigate the deployment landscape and successfully deploy your ReactJS apps.
Introduction to create-react-app
Create React App (CRA) is a popular toolchain used to create ReactJS applications with zero configuration. It provides a pre-configured setup that includes all the necessary dependencies, build scripts, and development server to get started with ReactJS development quickly.
To create a new ReactJS app using create-react-app, follow these steps:
Step 1: Ensure that you have Node.js installed on your machine. You can download and install Node.js from the official website (https://nodejs.org).
Step 2: Open your terminal or command prompt and run the following command to install create-react-app globally:
npm install -g create-react-app
Step 3: Once the installation is complete, navigate to the directory where you want to create your ReactJS app and run the following command:
npx create-react-app my-app
Replace "my-app" with the desired name of your app. This command will create a new directory called "my-app" and initialize a new ReactJS project inside it.
Step 4: Navigate to the newly created directory by running the following command:
cd my-app
Step 5: To start the development server and run your ReactJS app locally, run the following command:
npm start
This will start the development server and open your app in your default web browser. You can now begin developing your ReactJS app and make changes to the code in real-time, with automatic reloading.
Create React App also provides a build command that generates an optimized production-ready build of your ReactJS app. To create a production build, run the following command:
npm run build
This command will generate a "build" folder in your project directory, containing all the necessary files to deploy your ReactJS app.
Related Article: How to Add Navbar Components for Different Pages in ReactJS
Example 1: Creating a new ReactJS app using create-react-app
To illustrate the process of creating a new ReactJS app using create-react-app, let's walk through an example. We'll create a simple "Hello World" app.
Step 1: Open your terminal or command prompt.
Step 2: Run the following command to install create-react-app globally:
npm install -g create-react-app
Step 3: Once the installation is complete, navigate to the directory where you want to create your ReactJS app. For example, if you want to create the app in a folder called "my-app", run the following command:
npx create-react-app my-app
Step 4: Navigate to the newly created directory:
cd my-app
Step 5: Start the development server:
npm start
This will start the development server and open your app in your default web browser. You should see a "Welcome to React" message on the screen.
Congratulations! You have successfully created a new ReactJS app using create-react-app.
Example 2: Creating a production build of a ReactJS app
To showcase the process of creating a production build of a ReactJS app using create-react-app, let's continue with the previous example.
Step 1: Open your terminal or command prompt.
Step 2: Navigate to the directory of your ReactJS app. For example, if your app is located in a folder called "my-app", run the following command:
cd my-app
Step 3: Create a production build of your ReactJS app:
npm run build
This command will generate a "build" folder in your project directory, containing the optimized production-ready build of your ReactJS app.
You can now deploy the contents of the "build" folder to a hosting platform or server of your choice to make your ReactJS app accessible to users.
Using Webpack
Webpack is a useful module bundler that plays a crucial role in the deployment of ReactJS apps. It allows developers to bundle and optimize their JavaScript, CSS, and other assets into a single file or a set of files, making it easier to deploy and serve the application.
Webpack works by creating a dependency graph of all the modules and assets in your application and generating a bundle that can be used by the browser. It also provides various loaders and plugins to handle different types of assets, such as CSS, images, and fonts.
To utilize Webpack in the deployment of ReactJS apps, you can either configure it manually or use tools like create-react-app, which abstracts away the complexity of Webpack configuration.
Related Article: Handling State Persistence in ReactJS After Refresh
Example 1: Manual Webpack configuration
To illustrate the process of manually configuring Webpack for a ReactJS app, let's walk through an example. We'll configure Webpack to bundle our ReactJS app and handle CSS and image assets.
Step 1: Create a new file called "webpack.config.js" in the root directory of your ReactJS app.
Step 2: Open the "webpack.config.js" file in a text editor and add the following code:
const path = require('path'); module.exports = { entry: './src/index.js', output: { path: path.resolve(__dirname, 'dist'), filename: 'bundle.js', }, module: { rules: [ { test: /\.(js|jsx)$/, exclude: /node_modules/, use: { loader: 'babel-loader', }, }, { test: /\.css$/, use: ['style-loader', 'css-loader'], }, { test: /\.(png|svg|jpg|jpeg|gif)$/, type: 'asset/resource', }, ], }, };
This configuration sets the entry point of the application to "./src/index.js" and specifies the output directory as "./dist" with the filename "bundle.js". It also configures loaders for JavaScript files (using babel-loader), CSS files (using style-loader and css-loader), and image files (using asset/resource).
Step 3: Install the necessary dependencies by running the following command in your terminal or command prompt:
npm install webpack webpack-cli babel-loader style-loader css-loader --save-dev
Step 4: Build your ReactJS app using Webpack:
npx webpack
This command will run Webpack and generate a bundled JavaScript file and handle CSS and image assets according to the configuration specified in "webpack.config.js".
You can now deploy the generated bundle and assets to a hosting platform or server of your choice to make your ReactJS app accessible to users.
Example 2: Using create-react-app with Webpack
create-react-app abstracts away the complexity of Webpack configuration and provides a pre-configured setup for building and deploying ReactJS apps. However, if you need to customize the Webpack configuration, create-react-app provides an eject command that exposes the underlying configuration files.
To illustrate the process of using create-react-app with Webpack, let's continue with the previous example.
Step 1: Open your terminal or command prompt.
Step 2: Navigate to the directory of your ReactJS app. For example, if your app is located in a folder called "my-app", run the following command:
cd my-app
Step 3: Eject create-react-app to expose the underlying Webpack configuration:
npm run eject
This command will generate the necessary configuration files (such as "webpack.config.js") in your project directory.
Step 4: Customize the Webpack configuration as needed by modifying the generated "webpack.config.js" file.
Step 5: Build your ReactJS app using the modified Webpack configuration:
npm run build
This command will generate a production-ready build of your ReactJS app, incorporating the customizations made to the Webpack configuration.
You can now deploy the generated build to a hosting platform or server of your choice to make your ReactJS app accessible to users.
Leveraging Babel for ReactJS Deployment
Babel is a popular JavaScript compiler that allows developers to write modern JavaScript code and transpile it into backward-compatible versions of JavaScript that can run in older browsers or environments. It is a critical tool for deploying ReactJS apps, as it enables developers to take advantage of the latest JavaScript features and syntax while ensuring compatibility across different platforms.
Babel works by transforming JavaScript code using plugins and presets. Plugins are individual transformations that can be applied to specific syntax or features, while presets are collections of plugins that target specific JavaScript environments or versions.
To leverage Babel for ReactJS deployment, you can configure it manually or use tools like create-react-app, which handle Babel configuration out of the box.
Example 1: Manual Babel configuration
To illustrate the process of manually configuring Babel for a ReactJS app, let's walk through an example. We'll configure Babel to transpile our ReactJS app using the necessary presets and plugins.
Step 1: Create a new file called ".babelrc" in the root directory of your ReactJS app.
Step 2: Open the ".babelrc" file in a text editor and add the following code:
{ "presets": ["@babel/preset-env", "@babel/preset-react"] }
This configuration specifies the presets to use for transpiling the JavaScript code. "@babel/preset-env" targets the latest JavaScript features and automatically determines the plugins needed based on the specified browser or environment targets. "@babel/preset-react" is specifically for transpiling ReactJS code.
Step 3: Install the necessary dependencies by running the following command in your terminal or command prompt:
npm install @babel/core @babel/preset-env @babel/preset-react babel-loader --save-dev
Step 4: Update the Webpack configuration to use Babel for transpiling JavaScript files. Open the "webpack.config.js" file and modify the "module.rules" section as follows:
module: { rules: [ { test: /\.(js|jsx)$/, exclude: /node_modules/, use: { loader: 'babel-loader', }, }, // ... ], },
This configuration specifies that Babel should be used for transpiling JavaScript files with the ".js" or ".jsx" extension.
Step 5: Build your ReactJS app using Webpack:
npx webpack
This command will run Webpack and use Babel to transpile the JavaScript code according to the configuration specified in ".babelrc".
You can now deploy the generated bundle and assets, including the transpiled JavaScript code, to a hosting platform or server of your choice to make your ReactJS app accessible to users.
Related Article: How to Use a For Loop Inside Render in ReactJS
Example 2: Using create-react-app with Babel
create-react-app abstracts away the complexity of configuring Babel and provides a pre-configured setup for transpiling ReactJS apps. However, if you need to customize the Babel configuration, create-react-app provides an eject command that exposes the underlying configuration files.
To illustrate the process of using create-react-app with Babel, let's continue with the previous example.
Step 1: Open your terminal or command prompt.
Step 2: Navigate to the directory of your ReactJS app. For example, if your app is located in a folder called "my-app", run the following command:
cd my-app
Step 3: Eject create-react-app to expose the underlying Babel configuration:
npm run eject
This command will generate the necessary configuration files (such as ".babelrc") in your project directory.
Step 4: Customize the Babel configuration as needed by modifying the generated ".babelrc" file.
Step 5: Build your ReactJS app using the modified Babel configuration:
npm run build
This command will generate a production-ready build of your ReactJS app, incorporating the customizations made to the Babel configuration.
You can now deploy the generated build, including the transpiled JavaScript code, to a hosting platform or server of your choice to make your ReactJS app accessible to users.
Deploying a ReactJS App Using npm
npm (Node Package Manager) is a package manager for JavaScript that allows developers to install, manage, and share reusable code packages. It is commonly used in the deployment process of ReactJS apps to manage dependencies, build scripts, and other configuration files.
To deploy a ReactJS app using npm, you can follow these steps:
Step 1: Ensure that you have Node.js and npm installed on your machine. You can download and install Node.js from the official website (https://nodejs.org).
Step 2: Navigate to the root directory of your ReactJS app in your terminal or command prompt.
Step 3: Install the necessary dependencies by running the following command:
npm install
This command will fetch all the dependencies listed in the "package.json" file and install them in a "node_modules" directory.
Step 4: Build your ReactJS app using the build script defined in the "package.json" file. Typically, the build script is defined as:
"scripts": { "build": "react-scripts build" }
To build your app, run the following command:
npm run build
This command will generate a production-ready build of your ReactJS app in a "build" directory.
Step 5: Deploy the contents of the "build" directory to a hosting platform or server of your choice. This can be done using various methods, such as FTP, Git deployment, or cloud deployment platforms.
You can now access your deployed ReactJS app through the provided URL or domain.
Example 1: Deploying a ReactJS app using npm and FTP
To illustrate the process of deploying a ReactJS app using npm and FTP, let's walk through an example. We'll assume that you have a hosting server with FTP access.
Step 1: Ensure that you have Node.js and npm installed on your machine. You can download and install Node.js from the official website (https://nodejs.org).
Step 2: Create a new ReactJS app using create-react-app by running the following command in your terminal or command prompt:
npx create-react-app my-app
Step 3: Navigate to the root directory of your ReactJS app:
cd my-app
Step 4: Install the necessary dependencies:
npm install
Step 5: Build your ReactJS app:
npm run build
This command will generate a production-ready build of your ReactJS app in a "build" directory.
Step 6: Connect to your hosting server using an FTP client.
Step 7: Upload the contents of the "build" directory to the desired location on your hosting server.
Step 8: Once the upload is complete, access your deployed ReactJS app through the provided URL or domain.
Example 2: Deploying a ReactJS app using npm and cloud deployment platforms
Cloud deployment platforms provide a streamlined and automated way to deploy ReactJS apps. They offer various features, such as continuous integration and delivery, scalability, and easy management of deployments.
To illustrate the process of deploying a ReactJS app using npm and a cloud deployment platform, let's walk through an example using Heroku.
Step 1: Ensure that you have Node.js and npm installed on your machine. You can download and install Node.js from the official website (https://nodejs.org).
Step 2: Create a new ReactJS app using create-react-app by running the following command in your terminal or command prompt:
npx create-react-app my-app
Step 3: Navigate to the root directory of your ReactJS app:
cd my-app
Step 4: Install the necessary dependencies:
npm install
Step 5: Build your ReactJS app:
npm run build
This command will generate a production-ready build of your ReactJS app in a "build" directory.
Step 6: Create a new Heroku app by running the following command:
heroku create
This command will create a new Heroku app and provide you with a unique URL.
Step 7: Deploy your ReactJS app to Heroku by running the following command:
git push heroku master
This command will push the contents of your ReactJS app to Heroku and trigger the deployment process.
Step 8: Once the deployment is complete, access your deployed ReactJS app through the provided Heroku URL.
Related Article: Sharing Variables Between Components in ReactJS
Deploying a ReactJS App Using yarn
Yarn is another package manager for JavaScript that provides faster and more reliable dependency management compared to npm. It is commonly used in the deployment process of ReactJS apps as an alternative to npm.
To deploy a ReactJS app using yarn, you can follow these steps:
Step 1: Ensure that you have Node.js and yarn installed on your machine. You can download and install Node.js from the official website (https://nodejs.org) and install yarn by following the instructions on the yarn website (https://yarnpkg.com).
Step 2: Navigate to the root directory of your ReactJS app in your terminal or command prompt.
Step 3: Install the necessary dependencies by running the following command:
yarn install
This command will fetch all the dependencies listed in the "package.json" file and install them in a "node_modules" directory.
Step 4: Build your ReactJS app using the build script defined in the "package.json" file. Typically, the build script is defined as:
"scripts": { "build": "react-scripts build" }
To build your app, run the following command:
yarn run build
This command will generate a production-ready build of your ReactJS app in a "build" directory.
Step 5: Deploy the contents of the "build" directory to a hosting platform or server of your choice. This can be done using various methods, such as FTP, Git deployment, or cloud deployment platforms.
You can now access your deployed ReactJS app through the provided URL or domain.
Example 1: Deploying a ReactJS app using yarn and FTP
To illustrate the process of deploying a ReactJS app using yarn and FTP, let's walk through an example. We'll assume that you have a hosting server with FTP access.
Step 1: Ensure that you have Node.js and yarn installed on your machine. You can download and install Node.js from the official website (https://nodejs.org) and install yarn by following the instructions on the yarn website (https://yarnpkg.com).
Step 2: Create a new ReactJS app using create-react-app by running the following command in your terminal or command prompt:
npx create-react-app my-app
Step 3: Navigate to the root directory of your ReactJS app:
cd my-app
Step 4: Install the necessary dependencies:
yarn install
Step 5: Build your ReactJS app:
yarn run build
This command will generate a production-ready build of your ReactJS app in a "build" directory.
Step 6: Connect to your hosting server using an FTP client.
Step 7: Upload the contents of the "build" directory to the desired location on your hosting server.
Step 8: Once the upload is complete, access your deployed ReactJS app through the provided URL or domain.
Example 2: Deploying a ReactJS app using yarn and cloud deployment platforms
Cloud deployment platforms provide a streamlined and automated way to deploy ReactJS apps. They offer various features, such as continuous integration and delivery, scalability, and easy management of deployments.
To illustrate the process of deploying a ReactJS app using yarn and a cloud deployment platform, let's walk through an example using Netlify.
Step 1: Ensure that you have Node.js and yarn installed on your machine. You can download and install Node.js from the official website (https://nodejs.org) and install yarn by following the instructions on the yarn website (https://yarnpkg.com).
Step 2: Create a new ReactJS app using create-react-app by running the following command in your terminal or command prompt:
npx create-react-app my-app
Step 3: Navigate to the root directory of your ReactJS app:
cd my-app
Step 4: Install the necessary dependencies:
yarn install
Step 5: Build your ReactJS app:
yarn run build
This command will generate a production-ready build of your ReactJS app in a "build" directory.
Step 6: Create a new Netlify app by running the following command:
npx netlify init
This command will guide you through the process of setting up a new Netlify app and linking it to your Git repository.
Step 7: Deploy your ReactJS app to Netlify by running the following command:
npx netlify deploy
This command will deploy your ReactJS app to Netlify and provide you with a unique URL.
Step 8: Once the deployment is complete, access your deployed ReactJS app through the provided Netlify URL.
Exploring Popular Platforms for ReactJS Deployment
When it comes to deploying ReactJS apps, there are several popular platforms and hosting providers available that offer various features and functionalities. These platforms simplify the deployment process and provide tools for continuous integration and delivery, scalability, and easy management of deployments.
In this section, we will explore some of the popular platforms for ReactJS deployment and discuss their key features and benefits.
Related Article: How to Use the Render Method in ReactJS
Heroku
Heroku is a cloud platform that offers seamless deployment and hosting for web applications, including ReactJS apps. It provides an easy-to-use interface, a scalable infrastructure, and support for various programming languages and frameworks.
Key features of Heroku for ReactJS deployment include:
- Automatic scaling: Heroku automatically scales your app based on the traffic it receives, ensuring high availability and performance.
- Continuous integration and delivery: Heroku integrates with popular version control systems like Git and provides seamless continuous integration and delivery workflows.
- Add-ons: Heroku offers a wide range of add-ons that extend the functionality of your app, such as databases, logging services, and monitoring tools.
Netlify
Netlify is a modern platform for deploying and hosting web applications, including ReactJS apps. It offers a seamless workflow for frontend developers, with features like automatic builds, continuous deployment, and Git integration.
Key features of Netlify for ReactJS deployment include:
- Git-based deployments: Netlify integrates directly with Git repositories, making it easy to deploy and manage your ReactJS app using familiar Git workflows.
- Continuous deployment: Netlify automatically builds and deploys your ReactJS app whenever you push changes to your Git repository, ensuring that your app is always up to date.
- Custom domains and SSL: Netlify allows you to easily configure custom domains for your ReactJS app and provides free SSL certificates for secure HTTPS connections.
Vercel
Vercel is a cloud platform for static sites and serverless functions, making it a great choice for deploying ReactJS apps. It offers a seamless development and deployment experience, with features like zero configuration, automatic scaling, and serverless functions.
Key features of Vercel for ReactJS deployment include:
- Zero configuration: Vercel abstracts away the complexity of configuration and provides a seamless development and deployment experience for ReactJS apps.
- Automatic scaling: Vercel automatically scales your app based on the traffic it receives, ensuring high availability and performance.
- Serverless functions: Vercel allows you to easily deploy serverless functions alongside your ReactJS app, enabling you to build full-stack applications.
Using Vercel for ReactJS App Deployment
Vercel is a cloud platform for static sites and serverless functions, making it a great choice for deploying ReactJS apps. It offers a seamless development and deployment experience, with features like zero configuration, automatic scaling, and serverless functions.
To utilize Vercel for ReactJS app deployment, you can follow these steps:
Step 1: Ensure that you have Node.js and npm installed on your machine. You can download and install Node.js from the official website (https://nodejs.org).
Step 2: Navigate to the root directory of your ReactJS app in your terminal or command prompt.
Step 3: Create a new Git repository for your ReactJS app, if you haven't already done so.
Step 4: Initialize a new Git repository in your ReactJS app by running the following command:
git init
Step 5: Add all the files in your ReactJS app to the Git repository by running the following command:
git add .
Step 6: Commit the changes to the Git repository by running the following command:
git commit -m "Initial commit"
Step 7: Sign up for a Vercel account at https://vercel.com/signup.
Step 8: Log in to your Vercel account.
Step 9: Install the Vercel CLI by running the following command:
npm install -g vercel
Step 10: Deploy your ReactJS app to Vercel by running the following command:
vercel
This command will guide you through the process of deploying your ReactJS app to Vercel. You will be prompted to provide project settings and deployment options.
Step 11: Once the deployment is complete, access your deployed ReactJS app through the provided Vercel URL.
Related Article: How to Integrate UseHistory from the React Router DOM
Options for Deploying a ReactJS App on AWS
Amazon Web Services (AWS) provides a wide range of services and tools for deploying and hosting web applications, including ReactJS apps. It offers flexible and scalable infrastructure, security features, and integration with other AWS services.
When it comes to deploying a ReactJS app on AWS, there are several options available, depending on your specific requirements and preferences. Here are some of the options:
1. AWS Amplify: AWS Amplify is a development platform that provides a set of tools and services for building and deploying full-stack serverless applications. It offers an intuitive CLI, easy integration with Git repositories, and automatic scaling.
2. AWS Elastic Beanstalk: AWS Elastic Beanstalk is a fully managed service that makes it easy to deploy and run applications in multiple languages, including ReactJS. It provides a platform for deploying and managing your app, with built-in scalability, monitoring, and deployment options.
3. AWS S3: AWS S3 (Simple Storage Service) is a highly scalable object storage service that can be used to host your ReactJS app. You can upload the static assets of your app to an S3 bucket and configure the bucket as a static website, making your app accessible to users.
4. AWS CloudFront: AWS CloudFront is a content delivery network (CDN) that can be used to improve the performance and scalability of your ReactJS app. You can configure CloudFront to serve your app's static assets from edge locations around the world, reducing latency and improving user experience.
5. AWS Lambda and API Gateway: If your ReactJS app requires serverless backend functionality, you can use AWS Lambda and API Gateway to deploy your app's API endpoints and serverless functions. This allows you to build full-stack applications with ReactJS as the frontend and serverless functions as the backend.
These are just a few options for deploying a ReactJS app on AWS. Depending on your specific requirements, you can choose the option that best suits your needs and leverage other AWS services to enhance the functionality and scalability of your app.
Please note that deploying a ReactJS app on AWS may require familiarity with AWS services, configuration, and security best practices. It is recommended to refer to the official AWS documentation and seek appropriate guidance when deploying your app on AWS.
Additional Resources
- What is create-react-app and how does it help in deploying ReactJS?
- What is webpack and how is it used in deploying ReactJS?
- Differences between npm and yarn in the context of deploying ReactJS