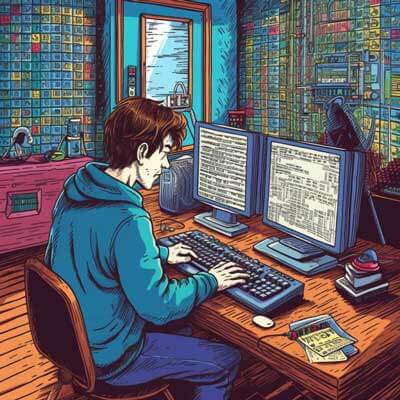
- 1. Inline Styles
- 2. CSS Modules
- What are CSS modules in React?
- Example
- How to add inline styles in ReactJS?
- What is CSS-in-JS and how to use it in React?
- Using styled-components
- What is styled-components and how to use it in React?
- What is react-jss and how to use it in React?
- What are the advantages of using react-css-modules?
- How to use custom CSS with React Bootstrap?
- How to apply global CSS styles in ReactJS?
- Additional Resources
Styling components in ReactJS can be done in various ways. Let’s explore some of the common methods.
1. Inline Styles
One way to style components in ReactJS is by using inline styles. Inline styles allow you to define styles directly within the component’s JSX code. This approach offers a high level of flexibility and allows you to dynamically change styles based on component state or props.
Here’s an example of how to apply inline styles in ReactJS:
import React from 'react'; const MyComponent = () => { const styles = { backgroundColor: 'blue', color: 'white', padding: '10px', }; return <div style={styles}>Hello, world!</div>; }; export default MyComponent;
In the above example, we define a styles
object containing the desired CSS properties. We then pass this object as the style
attribute of the div
element. This will apply the specified styles to the component.
Related Article: Handling Routing in React Apps with React Router
2. CSS Modules
CSS Modules is another approach to styling components in ReactJS. It allows you to write CSS in separate files and then import and use those styles in your components. CSS Modules provide local scoping of styles, preventing clashes with other components.
To use CSS Modules, follow these steps:
1. Create a CSS file with the desired styles. For example, MyComponent.module.css
:
.myComponent { background-color: blue; color: white; padding: 10px; }
2. Import the CSS file in your component:
import React from 'react'; import styles from './MyComponent.module.css'; const MyComponent = () => { return <div className={styles.myComponent}>Hello, world!</div>; }; export default MyComponent;
In the above example, we import the styles from the CSS file using the styles
object. We then apply the styles by setting the className
attribute of the div
element to the corresponding class name defined in the CSS file.
What are CSS modules in React?
CSS Modules is a feature in React that allows you to write modular CSS code for your components. It helps in preventing naming conflicts, as each CSS class is scoped locally to the component it belongs to. This means that the styles defined in one component won’t affect the styles of other components.
CSS Modules work by transforming class names in the CSS file into unique names that are specific to the component. These unique class names are then used in the component’s JSX code.
Using CSS Modules gives you the advantage of encapsulating styles within the component, making it easier to manage and maintain your codebase. It also provides better reusability, as the styles are tightly coupled with the component they belong to.
To use CSS Modules in React, you need to configure your build tool (e.g., webpack) to support CSS Modules. Once configured, you can import the CSS styles from a file and apply them to your components using the className
attribute.
Example:
Let’s say we have a component called Button
that needs to be styled using CSS Modules.
1. Create a CSS file named Button.module.css
with the following styles:
.button { background-color: blue; color: white; padding: 10px; }
2. Import the CSS file in your Button
component:
import React from 'react'; import styles from './Button.module.css'; const Button = () => { return <button className={styles.button}>Click me</button>; }; export default Button;
In the above example, we import the styles from the CSS file using the styles
object. We then apply the styles by setting the className
attribute of the button
element to the corresponding class name defined in the CSS file.
This way, the styles defined in the Button
component won’t interfere with the styles of other components, ensuring a modular and maintainable codebase.
Related Article: How to Build Forms in React
How to add inline styles in ReactJS?
Inline styles in ReactJS allow you to define styles directly within the component’s JSX code. This approach offers a high level of flexibility and allows you to dynamically change styles based on component state or props.
To add inline styles in ReactJS, you need to create a JavaScript object that represents the CSS properties you want to apply. You can then pass this object as the value of the style
attribute of the JSX element.
Here’s an example of how to add inline styles in ReactJS:
import React from 'react'; const MyComponent = () => { const styles = { backgroundColor: 'blue', color: 'white', padding: '10px', }; return <div style={styles}>Hello, world!</div>; }; export default MyComponent;
In the above example, we define a styles
object that contains the desired CSS properties. We then pass this object as the value of the style
attribute of the div
element. This will apply the specified styles to the component.
You can also dynamically change the inline styles based on component state or props. For example:
import React, { useState } from 'react'; const MyComponent = () => { const [isHovered, setIsHovered] = useState(false); const styles = { backgroundColor: isHovered ? 'blue' : 'red', color: 'white', padding: '10px', }; return ( <div style={styles} onMouseEnter={() => setIsHovered(true)} onMouseLeave={() => setIsHovered(false)} > Hello, world! </div> ); }; export default MyComponent;
In this example, we use the useState
hook to manage the isHovered
state. If the component is hovered, we change the background color to blue; otherwise, we set it to red. We update the state using the onMouseEnter
and onMouseLeave
event handlers to provide the hover functionality.
Inline styles in ReactJS give you the flexibility to create dynamic and responsive components without the need for external CSS files.
What is CSS-in-JS and how to use it in React?
CSS-in-JS is an approach to styling in React that allows you to write CSS code directly within your JavaScript code. It aims to solve the problem of CSS class name clashes and provides a more component-centric way of managing styles.
There are several CSS-in-JS libraries available for React, such as styled-components, emotion, and glamorous. These libraries provide a set of APIs and tools to create and manage styles in a more intuitive and efficient manner.
Let’s explore how to use one of the popular CSS-in-JS libraries, styled-components, in React.
Using styled-components
styled-components is a CSS-in-JS library for React that allows you to write CSS as JavaScript code. It provides a way to define styled components using a combination of tagged template literals and CSS syntax.
To use styled-components in your React project, you need to install it first:
$ npm install styled-components
Once installed, you can create styled components by importing the styled
function from the styled-components
package.
Here’s an example of how to use styled-components in React:
import React from 'react'; import styled from 'styled-components'; const Button = styled.button` background-color: blue; color: white; padding: 10px; `; const MyComponent = () => { return <Button>Hello, world!</Button>; }; export default MyComponent;
In the above example, we define a styled component called Button
using the styled
function. We pass a template literal containing the CSS styles as the argument to the styled
function. The resulting component can then be used just like any other React component.
Styled components can also accept props, allowing you to create dynamic styles based on component props. For example:
import React from 'react'; import styled from 'styled-components'; const Button = styled.button` background-color: ${props => (props.primary ? 'blue' : 'red')}; color: white; padding: 10px; `; const MyComponent = () => { return ( <> <Button primary>Hello, world!</Button> <Button>Hello, styled-components!</Button> </> ); }; export default MyComponent;
In this example, we use the props
object to conditionally set the background color of the Button
component. If the primary
prop is true, the background color will be blue; otherwise, it will be red.
Styled-components provide a useful and intuitive way to style components in React using CSS-in-JS. It promotes component reusability, modularity, and eliminates the need for external CSS files.
Related Article: How to Integrate UseHistory from the React Router DOM
What is styled-components and how to use it in React?
styled-components is a popular CSS-in-JS library for React that allows you to write CSS code directly within your JavaScript code. It provides a way to define styled components using a combination of tagged template literals and CSS syntax.
Using styled-components, you can create reusable and composable components with encapsulated styles. It eliminates the need for external CSS files and prevents CSS class name clashes. styled-components also provides advanced features like theming, dynamic styles, and server-side rendering.
To use styled-components in your React project, you need to install it first:
npm install styled-components
Once installed, you can import the styled
function from the styled-components
package to create styled components.
Here’s an example of how to use styled-components in React:
import React from 'react'; import styled from 'styled-components'; const Button = styled.button` background-color: blue; color: white; padding: 10px; `; const MyComponent = () => { return <Button>Hello, world!</Button>; }; export default MyComponent;
In the above example, we define a styled component called Button
using the styled
function. We pass a template literal containing the CSS styles as the argument to the styled
function. The resulting component can then be used just like any other React component.
Styled components can also accept props, allowing you to create dynamic styles based on component props. For example:
import React from 'react'; import styled from 'styled-components'; const Button = styled.button` background-color: ${props => (props.primary ? 'blue' : 'red')}; color: white; padding: 10px; `; const MyComponent = () => { return ( <> <Button primary>Hello, world!</Button> <Button>Hello, styled-components!</Button> </> ); }; export default MyComponent;
In this example, we use the props
object to conditionally set the background color of the Button
component. If the primary
prop is true, the background color will be blue; otherwise, it will be red.
Styled-components provide a useful and intuitive way to style components in React using CSS-in-JS. It promotes component reusability, modularity, and eliminates the need for external CSS files.
What is react-jss and how to use it in React?
react-jss is a library for React that provides a way to use JavaScript to define styles for your components. It combines the power of JavaScript with the flexibility of CSS to create dynamic and reusable styles.
Using react-jss, you can define styles as JavaScript objects and apply them to your components using the Higher Order Component (HOC) pattern. This allows you to easily create and manage styles within your React components.
To use react-jss in your React project, you need to install it first:
npm install react-jss
Once installed, you can import the injectSheet
function from the react-jss
package to create styled components.
Here’s an example of how to use react-jss in React:
import React from 'react'; import injectSheet from 'react-jss'; const styles = { button: { backgroundColor: 'blue', color: 'white', padding: '10px', }, }; const Button = ({ classes }) => { return <button className={classes.button}>Hello, world!</button>; }; export default injectSheet(styles)(Button);
In the above example, we define a JavaScript object called styles
that contains the desired CSS properties. We then pass this object to the injectSheet
function, which returns a Higher Order Component (HOC). The resulting HOC can be used to wrap our Button
component, injecting the styles as a classes
prop.
The classes
prop contains the generated class names for our styles. We can then apply these class names to the corresponding elements in our component.
React-jss also allows you to use dynamic styles based on component props. For example:
import React from 'react'; import injectSheet from 'react-jss'; const styles = { button: { backgroundColor: props => (props.primary ? 'blue' : 'red'), color: 'white', padding: '10px', }, }; const Button = ({ classes, primary }) => { return <button className={classes.button}>Hello, world!</button>; }; export default injectSheet(styles)(Button);
In this example, we use a function to define the backgroundColor
property of the button
style. The function receives the component props as an argument, allowing us to conditionally set the background color based on the primary
prop.
React-jss provides a useful way to create reusable and dynamic styles for your React components. It offers the flexibility of JavaScript and the modularity of CSS, making it a popular choice for styling in React.
What are the advantages of using react-css-modules?
react-css-modules is a library for React that provides a way to use CSS Modules in your components. It allows you to write CSS styles in separate files and then import and use those styles in your components.
There are several advantages of using react-css-modules:
1. Local Scoping: react-css-modules automatically generates unique class names for your CSS styles, preventing clashes with other components. This ensures that your styles are scoped locally to the component they belong to, avoiding global CSS pollution.
2. Improved Maintainability: By separating your styles into individual files, react-css-modules promotes modularity and code organization. It becomes easier to locate and update styles for specific components, leading to a more maintainable codebase.
3. Reusable Styles: react-css-modules allows you to define reusable styles that can be easily applied to multiple components. This promotes code reuse and reduces duplication, making your codebase more efficient and scalable.
4. Dynamic Styling: react-css-modules supports dynamic styling by allowing you to pass props to your CSS styles. This enables you to create dynamic and responsive components based on different states or conditions.
5. Easy Migration: If you already have an existing codebase that uses CSS Modules, react-css-modules provides a seamless way to integrate those styles into your React components. You can simply import the CSS styles and apply them using the styleName
attribute.
To use react-css-modules in your React project, you need to install it first:
npm install react-css-modules
Once installed, you can import the cssModules
higher-order component (HOC) from the react-css-modules
package to enhance your components with CSS Modules functionality.
Here’s an example of how to use react-css-modules in React:
import React from 'react'; import cssModules from 'react-css-modules'; import styles from './Button.module.css'; const Button = ({ styleName }) => { return <button styleName={styleName}>Hello, world!</button>; }; export default cssModules(Button, styles);
In the above example, we import the Button.module.css
file, which contains the CSS styles for our Button
component. We then apply the styles using the styleName
attribute.
React-css-modules enhances the Button
component with the CSS Modules functionality, automatically generating unique class names for the styles defined in the imported CSS file.
Overall, react-css-modules provides a convenient way to use CSS Modules in your React components, offering benefits like local scoping, improved maintainability, code reuse, dynamic styling, and easy migration from existing CSS Modules codebases.
Related Article: Enhancing React Applications with Third-Party Integrations
How to use custom CSS with React Bootstrap?
React Bootstrap is a popular library that provides pre-styled components and a grid system for building responsive web applications. It is based on the Bootstrap CSS framework and is designed to work seamlessly with React.
While React Bootstrap comes with its own set of predefined styles, you may need to customize the styles to match your specific design requirements. Here’s how you can use custom CSS with React Bootstrap:
1. Override Styles: React Bootstrap provides a way to override its default styles by adding custom CSS classes to the components. You can define your custom CSS classes in a separate CSS file and apply them to the React Bootstrap components using the className
attribute.
For example, let’s say you want to change the background color of a Button
component to red. You can define a CSS class in a separate file (custom.css
), import it in your component, and apply it to the Button
component:
import React from 'react'; import 'bootstrap/dist/css/bootstrap.min.css'; // Import the Bootstrap CSS import './custom.css'; // Import your custom CSS const MyComponent = () => { return <Button className="custom-button">Hello, world!</Button>; }; export default MyComponent;
In the custom.css
file, you can define your custom styles:
.custom-button { background-color: red; }
2. Use CSS Modules: If you prefer to use CSS Modules for styling, you can follow the same approach as mentioned earlier in the “How to style components in ReactJS?” section. CSS Modules provide local scoping of styles and prevent class name clashes.
For example, let’s say you want to customize the styles of a Button
component using CSS Modules. You can define your styles in a separate CSS file (MyComponent.module.css
), import it in your component, and apply the styles using the className
attribute:
import React from 'react'; import 'bootstrap/dist/css/bootstrap.min.css'; // Import the Bootstrap CSS import styles from './MyComponent.module.css'; // Import your CSS module const MyComponent = () => { return <Button className={styles.customButton}>Hello, world!</Button>; }; export default MyComponent;
In the MyComponent.module.css
file, you can define your custom styles:
.customButton { background-color: red; }
Using custom CSS with React Bootstrap allows you to customize the appearance of the pre-styled components according to your design needs. It gives you the flexibility to create unique and visually appealing interfaces while leveraging the power of React Bootstrap’s component library.
How to apply global CSS styles in ReactJS?
In ReactJS, applying global CSS styles can be done by importing the CSS file in the root component or using a CSS-in-JS library that supports global styles. Let’s explore both approaches:
1. Importing CSS file: One way to apply global CSS styles in ReactJS is by importing the CSS file in the root component of your application. This will make the styles available to all the components in your application.
First, create a CSS file with your global styles, for example, global.css
:
body { background-color: #f5f5f5; }
Next, import the CSS file in your root component, typically the App.js
file:
import React from 'react'; import './global.css'; // Import the global CSS file const App = () => { return ( <div> {/* Your application components */} </div> ); }; export default App;
2. CSS-in-JS with global styles: If you are using a CSS-in-JS library, such as styled-components, you can define global styles using their APIs. This approach allows you to write CSS styles directly within your JavaScript code.
Using styled-components as an example, you can define global styles by creating a component that sets the styles on the body
element:
import React from 'react'; import { createGlobalStyle } from 'styled-components'; const GlobalStyle = createGlobalStyle` body { background-color: #f5f5f5; } `; const App = () => { return ( <div> <GlobalStyle /> {/* Apply the global styles */} {/* Your application components */} </div> ); }; export default App;
In the above example, we define a GlobalStyle
component using the createGlobalStyle
function from styled-components. Inside the component, we write CSS styles using a tagged template literal. The resulting component is then rendered within the root component to apply the global styles.
Both approaches allow you to apply global CSS styles in your ReactJS application. Choose the one that best fits your project requirements and coding style.