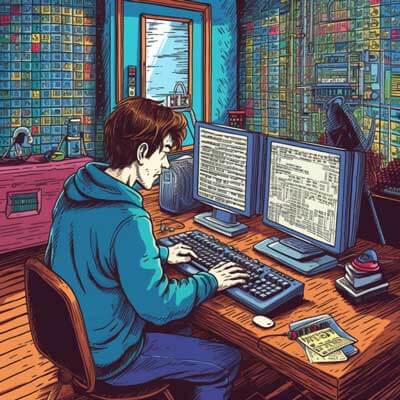
Table of Contents
Overview of String Interpolation in Python
String interpolation is a common technique used in programming languages to embed values or variables into a string. It allows developers to dynamically create strings by including placeholders that will be replaced with actual values at runtime. Python provides multiple ways to perform string interpolation, each with its own syntax and benefits. In this article, we will explore the two main methods of string interpolation in Python: f-strings and the format method.
Related Article: How To Check If List Is Empty In Python
The Use of f-strings for String Interpolation
Introduced in Python 3.6, f-strings provide a concise and readable way to perform string interpolation. They are prefixed with the letter 'f' and allow you to embed expressions or variables directly within curly braces {}. Let's take a look at a simple example:
name = "Alice"age = 25print(f"My name is {name} and I am {age} years old.")
In this example, the variables name
and age
are directly substituted into the string using f-strings. The output will be: "My name is Alice and I am 25 years old."
F-strings also support simple expressions and function calls. This makes them a useful tool for creating dynamic strings. We will explore this in more detail later in the article.
Using the Format Method for String Interpolation
Before the introduction of f-strings, the format method was the most common way to perform string interpolation in Python. It is available in all versions of Python and provides a flexible and useful mechanism for creating formatted strings. The format method works by using placeholder curly braces {} and passing the values to be substituted as arguments to the format method. Let's see an example:
name = "Bob"age = 30print("My name is {} and I am {} years old.".format(name, age))
In this example, the values of name
and age
are passed as arguments to the format method, which replaces the placeholders {} in the string with the corresponding values. The output will be: "My name is Bob and I am 30 years old."
The format method also supports a more advanced formatting syntax, allowing you to specify the width, alignment, precision, and other formatting options for the substituted values. This provides greater control over the appearance of the final string.
Variable Substitution in String Interpolation
Both f-strings and the format method support variable substitution, allowing you to directly substitute variables into strings. This can be useful when you want to include the value of a single variable in a string without any additional formatting. Let's see an example using f-strings:
name = "Charlie"print(f"Hello, {name}!")
In this example, the value of the variable name
is directly substituted into the string using an f-string. The output will be: "Hello, Charlie!"
Similarly, we can achieve the same result using the format method:
name = "Charlie"print("Hello, {}!".format(name))
The output will be the same: "Hello, Charlie!"
Related Article: Fixing "ValueError: Setting Array with a Sequenc" In Python
Comparing f-strings with the Format Method
Both f-strings and the format method provide ways to perform string interpolation in Python, but there are some differences between the two approaches.
One key difference is the syntax. F-strings use a more concise and readable syntax, with variables or expressions directly embedded within curly braces {}. This makes the code easier to write and understand. On the other hand, the format method uses placeholder curly braces {} and requires passing the values as arguments to the format method.
Another difference is the performance. F-strings are generally faster than the format method, as they are evaluated at compile-time rather than runtime. This can be significant when performing string interpolation in loops or in performance-critical sections of code.
In terms of functionality, the format method provides more advanced formatting options, allowing you to control the appearance of the substituted values in more detail. F-strings, on the other hand, are simpler and more straightforward for basic string interpolation.
Syntax for Variable Substitution in f-strings
In addition to simple variable substitution, f-strings support a variety of syntax options for more advanced string interpolation. Let's explore some of these options:
1. Formatting options: You can specify the formatting options for the substituted values within the curly braces {}. For example, you can specify the width, precision, alignment, and other formatting options. Here's an example:
name = "David"age = 35print(f"Name: {name:>10} | Age: {age:03}")
In this example, the width and alignment options are specified for the variable name
and the width and padding options are specified for the variable age
. The output will be: "Name: David | Age: 035".
2. Expressions: You can also include expressions within the curly braces {}. This allows you to perform calculations or manipulate the values before they are substituted into the string. Here's an example:
a = 10b = 20print(f"The sum of {a} and {b} is {a + b}.")
In this example, the sum of the variables a
and b
is calculated and substituted into the string. The output will be: "The sum of 10 and 20 is 30."
3. Method calls: You can even call methods or functions within the curly braces {}. This allows you to perform more complex operations or retrieve dynamic values before they are substituted into the string. Here's an example:
import datetimetoday = datetime.date.today()print(f"Today's date is {today.strftime('%Y-%m-%d')}.")
In this example, the strftime
method is called on the today
object to format the date string before it is substituted into the string. The output will be: "Today's date is 2022-01-01."
Incorporating Expressions or Function Calls in f-strings
As mentioned earlier, f-strings support the inclusion of expressions or function calls within the curly braces {}. This allows you to perform calculations, manipulate values, or retrieve dynamic data at the time of string interpolation. Let's explore some practical examples:
1. Calculations:
radius = 5area = 3.14 * radius ** 2print(f"The area of a circle with radius {radius} is {area:.2f}.")
In this example, the area of a circle is calculated using the formula π * r^2, where π is approximated to 3.14. The output will be: "The area of a circle with radius 5 is 78.50."
2. Manipulating values:
name = "Grace Hopper"print(f"Hello, {name.upper()}!")
In this example, the upper
method is called on the name
string to convert it to uppercase before it is substituted into the string. The output will be: "Hello, GRACE HOPPER!"
3. Retrieving dynamic data:
import randomnumber = random.randint(1, 10)print(f"The random number is {number}.")
In this example, the randint
function from the random
module is called to generate a random number between 1 and 10. The output will be a randomly generated number, such as "The random number is 7."
Additional Resources
- Real Python - String Interpolation: The New Way to Format Strings in Python