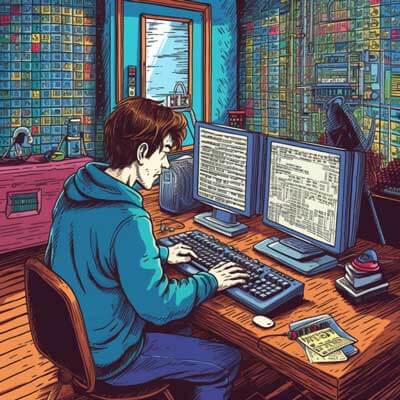
Table of Contents
Implementing a Navbar Component in ReactJS
A navbar is a common component used in web applications to provide navigation links and options to the user. In ReactJS, implementing a navbar component involves creating a reusable component that can be used across different pages of your application.
Related Article: How to Update a State Property in ReactJS
Example: Simple Navbar Component
Let's create a simple navbar component that displays a list of navigation links.
import React from 'react'; class Navbar extends React.Component { render() { return ( <nav> <ul> <li><a href="/">Home</a></li> <li><a href="/about">About</a></li> <li><a href="/contact">Contact</a></li> </ul> </nav> ); } } export default Navbar;
In the above code, we define a class-based component called Navbar
. The render
method returns a JSX element that represents the navbar. It consists of an HTML <nav>
element containing an unordered list <ul>
with three list items <li>
, each containing an anchor tag <a>
representing a navigation link. The href
attribute specifies the URL of the corresponding page.
To use this navbar component in your application, you can import it and include it in the JSX code of your main component, just like we did in the previous example.
Options for Displaying Different Navbar Components
In a ReactJS application, there are various options for displaying different navbar components based on the current page. Some common approaches include:
1. Conditional rendering: You can conditionally render different navbar components based on the current page or route.
2. React Router: React Router is a popular library for handling routing in React applications. It allows you to define routes and associate components with those routes. You can use React Router to display different navbar components based on the current route.
3. Higher-order components (HOCs): Higher-order components are functions that take a component and return a new component with additional functionality. You can use HOCs to wrap your navbar component and modify its behavior based on the current page.
Example: Conditional Rendering
Here's an example of using conditional rendering to display different navbar components based on the current page:
import React from 'react'; import HomePageNavbar from './HomePageNavbar'; import AboutPageNavbar from './AboutPageNavbar'; import ContactPageNavbar from './ContactPageNavbar'; class App extends React.Component { render() { const currentPath = window.location.pathname; let navbarComponent; if (currentPath === '/') { navbarComponent = <HomePageNavbar />; } else if (currentPath === '/about') { navbarComponent = <AboutPageNavbar />; } else if (currentPath === '/contact') { navbarComponent = <ContactPageNavbar />; } else { navbarComponent = <DefaultNavbar />; } return ( <div> {navbarComponent} {/* Rest of the page content */} </div> ); } } export default App;
In the above code, we use the window.location.pathname
property to get the current path of the URL. Based on the current path, we conditionally render different navbar components. If the current path matches a specific route, the corresponding navbar component is rendered. Otherwise, a default navbar component is rendered.
This approach allows you to have different navbar components for different pages and customize the navigation options based on the current page.
Related Article: Enhancing React Applications with Third-Party Integrations
Best Practices for Managing Different Navbar Components
When managing different navbar components in a ReactJS application, it's important to follow some best practices to ensure maintainability and scalability:
1. Use a consistent naming convention: Use clear and descriptive names for your navbar components to make it easier to understand their purpose.
2. Separate concerns: Split your navbar components into smaller, reusable components to separate concerns and improve reusability.
3. Use a centralized routing solution: Consider using a centralized routing solution like React Router to handle navigation and route-based rendering. This allows you to manage different navbar components in a more structured and scalable way.
4. Keep your code modular: Avoid having long and complex navbar components. Break them down into smaller, manageable components that can be easily understood and maintained.
5. Use props to pass data: If your navbar components require data from the parent component or need to react to user interactions, use props to pass the necessary data and callbacks.
Example: Separate Concerns
Let's say we have a complex navbar component that includes dropdown menus, search functionality, and user authentication. Instead of having all this functionality in a single component, we can separate them into smaller, reusable components:
import React from 'react'; import NavbarMenu from './NavbarMenu'; import NavbarSearch from './NavbarSearch'; import NavbarAuth from './NavbarAuth'; class Navbar extends React.Component { render() { return ( <nav> <NavbarMenu /> <NavbarSearch /> <NavbarAuth /> </nav> ); } } export default Navbar;
In the above code, we split the navbar component into three separate components: NavbarMenu
, NavbarSearch
, and NavbarAuth
. Each component is responsible for a specific part of the navbar's functionality. This not only makes the code more modular but also allows us to reuse these components in different parts of our application if needed.
Libraries and Frameworks for Displaying Different Navbar Components
While ReactJS provides the core functionality for displaying different navbar components, there are several libraries and frameworks that can enhance and simplify the process. Here are a few popular options:
1. React Router: React Router is a routing library for React applications. It provides a declarative way to define routes and associate components with those routes. React Router can be used to display different navbar components based on the current route.
2. Next.js: Next.js is a framework for building server-rendered React applications. It includes built-in routing capabilities and provides an easy way to create different navbar components for different pages. Next.js also offers features like code splitting and server-side rendering, which can improve performance.
3. Gatsby.js: Gatsby.js is a static site generator that uses React for building websites. It provides a useful routing system and allows you to create different navbar components for different pages. Gatsby.js also offers features like image optimization and data sourcing from various CMS providers.
4. React Navigation: React Navigation is a popular library for handling navigation in mobile applications built with React Native. It provides a flexible and customizable navigation solution for different platforms. React Navigation can be used to create different navbar components for different screens in a mobile app.
These are just a few examples of libraries and frameworks that can assist in displaying different navbar components in ReactJS applications. The choice depends on the specific requirements of your project and the level of complexity you need.
Styling Different Navbar Components with CSS
Styling is an important aspect of any user interface, including navbar components in ReactJS applications. You have several options for styling your navbar components, including inline styles, CSS modules, and CSS-in-JS libraries like styled-components.
Related Article: How to Use the Render Method in ReactJS
Example: Inline Styles
Inline styles allow you to define styles directly in your JSX code using JavaScript objects. Here's an example of using inline styles to style a navbar component:
import React from 'react'; class Navbar extends React.Component { render() { const navbarStyle = { backgroundColor: 'blue', color: 'white', padding: '10px', }; const linkStyle = { color: 'white', margin: '0 10px', textDecoration: 'none', }; return ( <nav style={navbarStyle}> <a href="/" style={linkStyle}>Home</a> <a href="/about" style={linkStyle}>About</a> <a href="/contact" style={linkStyle}>Contact</a> </nav> ); } } export default Navbar;
In the above code, we define two JavaScript objects (navbarStyle
and linkStyle
) that contain the styles for the navbar and links, respectively. We then use the style
attribute to apply these styles to the corresponding JSX elements.
Inline styles can be useful for simple styling needs, but they can become cumbersome for complex styles or when you need to reuse styles across multiple components.
Passing Data between Different Navbar Components
In a ReactJS application, you may need to pass data between different navbar components. This can be done using props or a shared state management solution like React Context or Redux.
Example: Passing Data with Props
Here's an example of passing data between a parent component and its child navbar component using props:
import React from 'react'; class ParentComponent extends React.Component { state = { isLoggedIn: false, username: '', }; handleLogin = (username) => { this.setState({ isLoggedIn: true, username }); }; render() { return ( <div> <Navbar isLoggedIn={this.state.isLoggedIn} username={this.state.username} /> {/* Rest of the page content */} </div> ); } } class Navbar extends React.Component { render() { const { isLoggedIn, username } = this.props; return ( <nav> {isLoggedIn ? ( <div> Welcome, {username}! <a href="/logout">Logout</a> </div> ) : ( <a href="/login">Login</a> )} </nav> ); } } export default ParentComponent;
In the above code, the parent component (ParentComponent
) manages the state of whether the user is logged in and the username. It passes this data to the child navbar component (Navbar
) as props. Based on the value of the isLoggedIn
prop, the navbar component renders different content.
This approach allows you to pass data between different components and update the UI based on that data.
Dynamically Loading Different Navbar Components based on User Interactions
In some cases, you may want to dynamically load different navbar components based on user interactions, such as clicking on a button or selecting an option from a dropdown menu. This can be achieved by handling events and conditionally rendering the desired component.
Related Article: How to Build Forms in React
Example: Dynamically Loading Navbar Components
Here's an example of dynamically loading different navbar components based on user interactions:
import React from 'react'; import HomePageNavbar from './HomePageNavbar'; import AboutPageNavbar from './AboutPageNavbar'; import ContactPageNavbar from './ContactPageNavbar'; class App extends React.Component { state = { currentPage: 'home', }; handlePageChange = (page) => { this.setState({ currentPage: page }); }; render() { let navbarComponent; switch (this.state.currentPage) { case 'home': navbarComponent = <HomePageNavbar />; break; case 'about': navbarComponent = <AboutPageNavbar />; break; case 'contact': navbarComponent = <ContactPageNavbar />; break; default: navbarComponent = <DefaultNavbar />; } return ( <div> <button onClick={() => this.handlePageChange('home')}>Home</button> <button onClick={() => this.handlePageChange('about')}>About</button> <button onClick={() => this.handlePageChange('contact')}>Contact</button> {navbarComponent} {/* Rest of the page content */} </div> ); } } export default App;
In the above code, we have a state variable currentPage
that represents the current page. When a button is clicked, the handlePageChange
function is called with the corresponding page as an argument, updating the state. Based on the value of currentPage
, the appropriate navbar component is rendered.
This approach allows you to dynamically load different navbar components based on user interactions, providing a more interactive and personalized user experience.
Performance Considerations when Displaying Different Navbar Components
When displaying different navbar components in a ReactJS application, it's important to consider performance to ensure a smooth user experience. Here are some performance considerations to keep in mind:
1. Minimize unnecessary re-renders: Avoid re-rendering the navbar component unnecessarily. Use lifecycle methods like shouldComponentUpdate
or React's memoization techniques to optimize rendering.
2. Use code splitting: If your application has a large number of navbar components or other UI elements, consider using code splitting to load only the necessary components when needed. This can improve initial load times and reduce the overall bundle size.
3. Optimize image loading: If your navbar components include images, optimize their loading by using lazy loading techniques or optimizing image sizes and formats.
4. Avoid unnecessary data fetching: If your navbar components require data from an API, make sure to fetch only the necessary data and avoid unnecessary API calls.
5. Use efficient CSS: Optimize your CSS to reduce unnecessary styles and improve rendering performance. Avoid using complex selectors or excessive use of CSS animations that can impact performance.