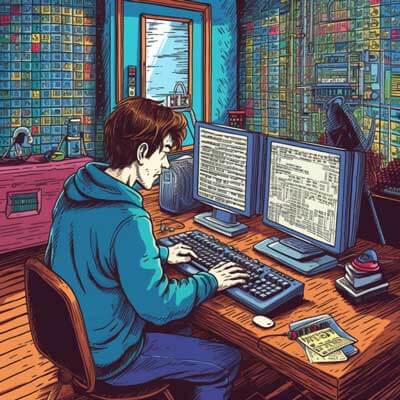
Table of Contents
The Benefits of ReactJS's Virtual DOM
ReactJS's Virtual DOM is a key factor in its high-speed performance. The Virtual DOM is a lightweight representation of the actual DOM (Document Object Model) and serves as an intermediary between React components and the browser's rendering engine. It allows React to efficiently update only the necessary parts of the DOM, minimizing the number of expensive and time-consuming operations required to update the UI.
One of the major benefits of the Virtual DOM is its ability to batch multiple updates into a single operation. Instead of directly manipulating the real DOM for every change in the application state, React builds a virtual representation of the DOM and performs a diffing algorithm to identify the minimal number of changes needed to update the actual DOM. This process significantly reduces the number of operations required and improves overall performance.
Let's consider an example to better understand the benefits of React's Virtual DOM. Imagine a scenario where a user interacts with a form by typing in an input field. With traditional DOM manipulation, every keystroke would trigger a direct update to the DOM, causing unnecessary re-rendering and potentially impacting the performance of the application. However, with React's Virtual DOM, the changes are first applied to the virtual representation, and React intelligently determines the minimal changes required to update the real DOM. This efficient diffing process results in a smoother and faster user experience.
Here's an example code snippet that demonstrates the use of React's Virtual DOM:
import React from 'react';class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } incrementCount() { this.setState(prevState => ({ count: prevState.count + 1 })); } render() { return ( <div> <h1>Count: {this.state.count}</h1> <button onClick={() => this.incrementCount()}>Increment</button> </div> ); }}export default Counter;
In this example, we have a simple counter component that increments the count value when the button is clicked. The changes to the count value are first applied to the Virtual DOM, and React updates the actual DOM with the minimal necessary changes, resulting in a fast and efficient rendering process.
The use of React's Virtual DOM not only improves performance but also simplifies the development process. Developers can focus on writing declarative code and let React handle the efficient updates to the DOM. This abstraction allows for faster development cycles and easier maintenance of complex UIs.
Related Article: Exploring Buffer Usage in ReactJS
Exploring the Performance Optimization Techniques in ReactJS
ReactJS provides several performance optimization techniques that can further enhance the speed of React applications. These techniques involve optimizing rendering, reducing unnecessary re-renders, and optimizing component lifecycles.
One of the key techniques is the use of React's PureComponent and memo higher-order component. These components perform shallow comparisons of the component's props and state to determine if a re-render is necessary. By skipping unnecessary re-renders, React reduces the amount of work required to update the UI and improves performance.
Here's an example code snippet that demonstrates the use of PureComponent:
import React from 'react';class Counter extends React.PureComponent { constructor(props) { super(props); this.state = { count: 0 }; } incrementCount() { this.setState(prevState => ({ count: prevState.count + 1 })); } render() { return ( <div> <h1>Count: {this.state.count}</h1> <button onClick={() => this.incrementCount()}>Increment</button> </div> ); }}export default Counter;
In this example, the Counter component extends React.PureComponent instead of React.Component. This ensures that the component only re-renders when the props or state change, preventing unnecessary updates and improving performance.
Another performance optimization technique in React is the use of key prop when rendering lists of components. The key prop helps React identify the uniqueness of each component in the list, enabling more efficient updates when items are added, removed, or reordered. Providing a stable and unique key for each item in a list can significantly improve rendering performance, especially when dealing with large datasets.
Here's an example code snippet that demonstrates the use of key prop in a list component:
import React from 'react';class List extends React.Component { render() { const { items } = this.props; return ( <ul> {items.map(item => ( <li key={item.id}>{item.name}</li> ))} </ul> ); }}export default List;
In this example, each item in the list has a unique identifier represented by the item.id
property. By providing the key prop with this identifier, React can efficiently update the list when items are added, removed, or reordered, resulting in improved performance.
These are just a few examples of the performance optimization techniques available in ReactJS. By leveraging these techniques and understanding the underlying mechanisms, developers can create highly performant React applications that deliver a seamless user experience.
Understanding the Component-Based Architecture in ReactJS
ReactJS follows a component-based architecture, which is one of the key reasons behind its high-speed performance. This architecture allows developers to break down the user interface into reusable components, each responsible for a specific piece of functionality.
The component-based architecture promotes modularity, reusability, and separation of concerns. By encapsulating functionality within individual components, developers can easily manage and maintain complex UIs. Each component can be developed, tested, and optimized independently, resulting in a more efficient development process.
In React, components are at the core of the architecture. Components can be classified into two types: functional components and class components.
Functional components are purely based on JavaScript functions and have no internal state. They receive data through props and return a JSX representation of the UI. Functional components are lightweight and performant, making them ideal for simple UI elements.
Here's an example of a functional component:
import React from 'react';const Button = ({ onClick, label }) => { return <button onClick={onClick}>{label}</button>;};export default Button;
In this example, the Button component is a functional component that receives an onClick event handler and a label as props. It simply renders a button element with the provided label.
Class components, on the other hand, are JavaScript classes that extend the React.Component class. They have an internal state and can implement lifecycle methods. Class components are suitable for more complex UI elements that require state management and lifecycle hooks.
Here's an example of a class component:
import React from 'react';class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } incrementCount() { this.setState(prevState => ({ count: prevState.count + 1 })); } render() { return ( <div> <h1>Count: {this.state.count}</h1> <button onClick={() => this.incrementCount()}>Increment</button> </div> ); }}export default Counter;
In this example, the Counter component is a class component that manages an internal count state. It renders the count value and a button to increment it.
The component-based architecture in React allows for efficient rendering and updates. When a component's state or props change, React intelligently updates only the affected components, minimizing the number of DOM operations and improving performance. The reusability and modularity offered by the component-based architecture also contribute to faster development cycles and easier maintenance.
The Role of Server-Side Rendering in ReactJS's Speed
Server-side rendering (SSR) plays a crucial role in ReactJS's high-speed performance. SSR allows React to render components on the server and send the pre-rendered HTML to the client, reducing the time required for initial page load and improving perceived performance.
The traditional approach to web development involves rendering the entire UI on the client-side using JavaScript. This approach requires downloading and executing JavaScript code before rendering the UI, resulting in a slower initial page load. However, with SSR, React can generate the initial HTML on the server and send it to the client, enabling faster rendering and improved performance.
When a user requests a page from a React application with SSR enabled, the server generates the HTML markup for the requested page, including the pre-rendered React components. This HTML is then sent to the client, which can display it immediately without waiting for JavaScript to be downloaded and executed. Once the JavaScript bundle is loaded and executed, React takes over control of the UI and hydrates the pre-rendered components, allowing for interactivity and dynamic updates.
To enable SSR in React, developers can use frameworks like Next.js, which provides built-in server-side rendering capabilities. Next.js simplifies the setup and configuration required for SSR, allowing developers to focus on building high-performance React applications.
Here's an example code snippet that demonstrates server-side rendering with Next.js:
// pages/index.jsimport React from 'react';const HomePage = () => { return ( <div> <h1>Hello, world!</h1> <p>Welcome to my React application.</p> </div> );};export default HomePage;
In this example, we have a simple Next.js page component that renders a heading and a paragraph. When a user requests the homepage, Next.js pre-renders the HTML on the server and sends it to the client, improving the initial page load speed.
Related Article: Accessing Array Length in this.state in ReactJS
The Reconciliation Algorithm and its Impact on ReactJS's Performance
ReactJS's reconciliation algorithm is a critical aspect of its high-speed performance. The reconciliation algorithm is responsible for determining the differences between the previous and current versions of the Virtual DOM and efficiently updating the actual DOM with the minimum required changes.
When React components update their state or receive new props, React performs a process called reconciliation. During reconciliation, React compares the previous and current versions of the Virtual DOM and identifies the differences between them. This process is known as the diffing algorithm.
The diffing algorithm analyzes the component tree and identifies the minimal number of changes required to update the DOM. React achieves this by using a heuristic approach that optimizes for common use cases. The algorithm tries to minimize the number of operations required, such as adding, updating, or removing DOM elements.
For example, if a component's props change, React will compare the new props with the previous props and determine which parts of the component's UI need to be updated. It will then update only those specific parts, instead of re-rendering the entire component.
The reconciliation algorithm's efficiency is achieved through several optimization strategies. React uses a top-down, depth-first traversal strategy to efficiently compare components and their children. It also uses a concept called "keys" to uniquely identify components in lists, allowing for more efficient updates in list rendering.
Here's an example code snippet that demonstrates the use of keys in list rendering:
import React from 'react';const List = ({ items }) => { return ( <ul> {items.map(item => ( <li key={item.id}>{item.name}</li> ))} </ul> );};export default List;
In this example, each item in the list has a unique identifier represented by the item.id
property. The key
prop is assigned this unique identifier, allowing React to efficiently update the list when items are added, removed, or reordered.
The reconciliation algorithm plays a crucial role in ReactJS's performance. By efficiently determining the minimal required changes to the DOM, React minimizes the number of expensive DOM operations, resulting in faster rendering and improved overall performance.
Examining the Diffing Algorithm and its Contribution to ReactJS's Speed
The diffing algorithm is a fundamental part of ReactJS's high-speed performance. The diffing algorithm is responsible for efficiently comparing the previous and current versions of the Virtual DOM and determining the minimal changes required to update the actual DOM.
React's diffing algorithm performs a top-down, depth-first traversal of the component tree. It compares components and their children to identify differences and determine the necessary updates to the DOM. The algorithm uses a heuristic approach to optimize for common use cases and minimize the number of operations required.
One of the key strategies used by the diffing algorithm is the concept of "keys". Keys are unique identifiers assigned to components in lists. React uses keys to efficiently update components in lists when items are added, removed, or reordered.
Let's consider an example to better understand the diffing algorithm and the use of keys. Imagine a scenario where we have a list of items and the user can add or remove items dynamically. Without keys, React would have to re-render the entire list every time an item is added or removed, resulting in a slow and inefficient process. However, by using keys, React can efficiently determine the minimal changes required and update only the affected components.
Here's an example code snippet that demonstrates the use of keys in list rendering:
import React from 'react';const List = ({ items }) => { return ( <ul> {items.map(item => ( <li key={item.id}>{item.name}</li> ))} </ul> );};export default List;
In this example, each item in the list has a unique identifier represented by the item.id
property. The key
prop is assigned this unique identifier, allowing React to efficiently update the list when items are added, removed, or reordered.
The diffing algorithm's efficiency is achieved through various optimizations. React compares components by their type and only updates the necessary parts of the DOM. It also uses a concept called "fiber" to break the diffing process into smaller chunks, allowing for incremental updates and better overall performance.
The Enhancements of Async Rendering in ReactJS
Async rendering is a useful feature in ReactJS that enhances its performance by prioritizing high-priority updates and ensuring a responsive user interface. Async rendering allows React to break the rendering process into smaller, incremental updates, avoiding long-running tasks that could block the main thread and cause UI freezes.
Traditionally, React used a synchronous rendering approach, where updates were processed immediately and could potentially block the main thread. With async rendering, React introduces a concept called "fiber" that enables incremental rendering and better control over the rendering process.
The fiber architecture allows React to prioritize and interrupt rendering work based on the priority of the updates. It breaks the rendering process into small units of work called fibers, which can be interrupted and resumed as needed. This enables React to prioritize high-priority updates, such as user interactions, and ensure a responsive UI.
Here's an example code snippet that demonstrates the use of suspense in ReactJS:
import React, { Suspense } from 'react';const LazyComponent = React.lazy(() => import('./LazyComponent'));const App = () => { return ( <div> <h1>My React App</h1> <Suspense fallback={<div>Loading...</div>}> <LazyComponent /> </Suspense> </div> );};export default App;
In this example, the LazyComponent is loaded lazily using React.lazy and Suspense. React.lazy allows for dynamic importing of components, and Suspense provides a fallback UI while the component is being loaded. This ensures a smooth user experience even when dealing with slow-loading components.
Async rendering and its associated features enhance ReactJS's performance by prioritizing high-priority updates, avoiding UI freezes, and providing a responsive user interface. By leveraging async rendering, React enables developers to build fast and interactive applications that deliver a seamless user experience.
The Significance of Immutable Data in ReactJS's Performance
Immutable data plays a significant role in ReactJS's high-speed performance. React encourages the use of immutable data structures to optimize rendering performance and avoid unnecessary updates.
In JavaScript, mutable data can be modified directly, leading to potential side effects and unintended changes. With mutable data, React has to perform deep comparisons to determine if a component needs to be re-rendered. This can be time-consuming, especially when dealing with complex data structures.
Immutable data, on the other hand, cannot be modified once created. Instead of directly modifying the data, immutable data structures create new copies with the desired changes. This ensures that the original data remains unchanged, making it easier to reason about and preventing unintended updates.
Immutable data also enables efficient memoization and caching of intermediate results. React can memoize the results of expensive computations using the reference equality of the input data. This allows React to reuse the memoized results instead of recomputing them, resulting in faster rendering performance.
Here's an example code snippet that demonstrates the use of immutable data with the Immutable.js library:
import { Map } from 'immutable';const data = Map({ count: 0 });const incrementCount = () => { const updatedData = data.set('count', data.get('count') + 1); console.log(updatedData.get('count')); // 1};incrementCount();console.log(data.get('count')); // 0
In this example, we use the Immutable.js library to create an immutable Map data structure. The count value is initially set to 0. When the incrementCount function is called, a new Map is created with the updated count value. The original data remains unchanged, ensuring the immutability of the data.
Related Article: Displaying Arrays with Onclick Events in ReactJS
Understanding the Component Lifecycle and its Effect on ReactJS's Speed
The component lifecycle is a fundamental concept in ReactJS that influences its performance. Understanding the component lifecycle allows developers to optimize rendering and avoid unnecessary updates, resulting in improved overall performance.
React components go through several lifecycle phases, starting from initialization, updating, and eventually unmounting. Each phase provides hooks or lifecycle methods that developers can use to control component behavior and perform necessary operations.
One of the key lifecycle methods is shouldComponentUpdate, which allows developers to optimize rendering by determining if a component needs to update. By implementing shouldComponentUpdate and performing shallow comparisons of props and state, developers can prevent unnecessary re-renders and improve performance.
Here's an example code snippet that demonstrates the use of shouldComponentUpdate:
import React from 'react';class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } shouldComponentUpdate(nextProps, nextState) { return this.state.count !== nextState.count; } incrementCount() { this.setState(prevState => ({ count: prevState.count + 1 })); } render() { return ( <div> <h1>Count: {this.state.count}</h1> <button onClick={() => this.incrementCount()}>Increment</button> </div> ); }}export default Counter;
In this example, the shouldComponentUpdate method is implemented to compare the current count state with the next count state. If the count does not change, the component will not update, preventing unnecessary re-renders.
Why ReactJS Outperforms Other JavaScript Frameworks
ReactJS has gained popularity in the web development community due to its high-speed performance and efficient rendering capabilities. There are several reasons why ReactJS outperforms other JavaScript frameworks:
1. Virtual DOM: React's Virtual DOM is a lightweight representation of the actual DOM. It allows React to efficiently update only the necessary parts of the DOM, minimizing the number of expensive operations required. By using a diffing algorithm, React identifies the minimal changes required to update the actual DOM, resulting in faster rendering and improved performance.
2. Component-Based Architecture: React follows a component-based architecture, which promotes modularity, reusability, and separation of concerns. Components encapsulate functionality and can be developed, tested, and optimized independently. The component-based architecture simplifies development and maintenance, resulting in faster development cycles and better performance.
3. Server-Side Rendering: React supports server-side rendering (SSR), where components are rendered on the server and sent as pre-rendered HTML to the client. SSR improves initial page load times, enhances search engine optimization (SEO), and provides a better user experience. React's ability to pre-render the HTML on the server reduces the time required for the initial page load, resulting in faster rendering and improved performance.
4. Efficient Reconciliation Algorithm: React's reconciliation algorithm efficiently determines the differences between the previous and current versions of the Virtual DOM. By using a diffing algorithm and optimizing for common use cases, React minimizes the number of operations required to update the DOM, resulting in faster rendering and improved performance.
5. Async Rendering: React's async rendering feature allows for incremental rendering and better control over the rendering process. Async rendering breaks the rendering process into smaller, incremental updates, avoiding long-running tasks that could block the main thread and cause UI freezes. This feature ensures a responsive user interface and enhances overall performance.
6. Immutable Data: React encourages the use of immutable data structures, which optimize rendering performance and prevent unintended updates. Immutable data allows React to perform reference equality checks instead of deep comparisons, minimizing the number of expensive operations and improving rendering performance. Immutable data also enables efficient memoization and caching of intermediate results, further enhancing performance.
Additional Resources
- Memoization in React