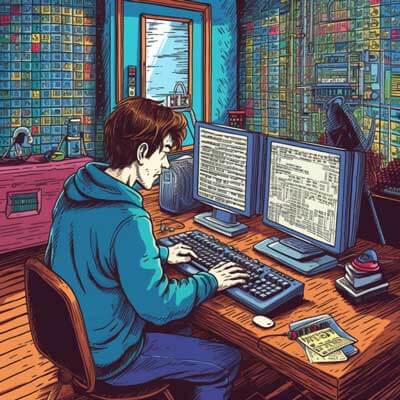
Table of Contents
Overview of String Trimming in Python
String trimming is the process of removing unwanted characters or whitespace from the beginning or end of a string. There are several methods and techniques available to trim strings and manipulate their contents. In this article, we will explore some of the most commonly used methods for trimming strings in Python, including the strip(), split(), substring(), and slice() methods.
Related Article: Fixing 'Dataframe Constructor Not Properly Called' in Python
The strip() Method in Python
The strip() method in Python is a versatile and convenient way to trim strings by removing leading and trailing whitespace. This method can be used to remove spaces, tabs, newlines, or any other whitespace characters from the beginning and end of a string.
To use the strip() method, simply call it on the string object and it will return a new string with the whitespace removed. Here's an example:
text = " Hello, World! " trimmed_text = text.strip() print(trimmed_text)
Output:
Hello, World!
In this example, the strip() method removes the leading and trailing spaces from the string " Hello, World! ", resulting in the trimmed string "Hello, World!".
It's important to note that the strip() method does not modify the original string, but instead returns a new trimmed string. If you want to modify the original string, you can assign the result back to the same variable:
text = " Hello, World! " text = text.strip() print(text)
Output:
Hello, World!
The split() Method
The split() method in Python can be used to split a string into a list of substrings based on a specified delimiter. By using this method, you can effectively separate a string into multiple parts and extract the desired portion.
To split a string using the split() method, you need to provide the delimiter as an argument. The delimiter can be any character or string that acts as a separator. Here's an example:
text = "Hello, World!" parts = text.split(",") print(parts)
Output:
['Hello', ' World!']
In this example, the split() method splits the string "Hello, World!" into two parts based on the comma delimiter. The resulting list contains the substrings "Hello" and " World!".
If you don't specify a delimiter, the split() method will split the string based on whitespace by default. Here's an example:
text = "Hello World!" parts = text.split() print(parts)
Output:
['Hello', 'World!']
In this example, the split() method splits the string "Hello World!" into two parts based on the whitespace delimiter.
The substring() Method
Python does not have a built-in substring() method like some other programming languages. However, you can achieve the same functionality by using string slicing.
String slicing allows you to extract a portion of a string by specifying the start and end indices. The syntax for string slicing is string[start:end]
, where start
is the index of the first character to include and end
is the index of the first character to exclude.
Here's an example of using string slicing to extract a substring:
text = "Hello, World!" substring = text[7:12] print(substring)
Output:
World
In this example, the substring() method extracts the portion of the string "Hello, World!" starting from index 7 (inclusive) and ending at index 12 (exclusive), resulting in the substring "World".
It's important to note that string indices in Python start from 0. So, the first character of a string has an index of 0, the second character has an index of 1, and so on.
Related Article: Comparing Substrings in Python
Removing Whitespace in Python
In addition to the strip() method, there are other ways to remove whitespace from strings in Python. Here are a few techniques you can use:
1. Using the replace() method: The replace() method allows you to replace a specific substring with another substring in a string. To remove whitespace, you can replace it with an empty string. Here's an example:
text = " Hello, World! " trimmed_text = text.replace(" ", "") print(trimmed_text)
Output:
Hello,World!
In this example, the replace() method is used to replace all occurrences of spaces with an empty string, effectively removing the whitespace.
2. Using regular expressions: Python's re module provides useful functions for working with regular expressions. You can use the re.sub()
function to remove whitespace using a regular expression pattern. Here's an example:
import re text = " Hello, World! " trimmed_text = re.sub(r'\s', '', text) print(trimmed_text)
Output:
Hello,World!
In this example, the regular expression pattern '\s'
matches any whitespace character, and the re.sub()
function replaces all matches with an empty string.
The slice() Method
The slice() method in Python allows you to extract a portion of a string by specifying the start, end, and step indices. The syntax for using the slice() method is string[start:end:step]
, where start
is the index of the first character to include, end
is the index of the first character to exclude, and step
is the interval between characters to include.
Here's an example of using the slice() method to extract a portion of a string:
text = "Hello, World!" substring = text[7:12:2] print(substring)
Output:
Wrd
In this example, the slice() method extracts the portion of the string "Hello, World!" starting from index 7 (inclusive) and ending at index 12 (exclusive), with a step size of 2. As a result, every second character within the specified range is included in the substring, resulting in the substring "Wrd".
It's important to note that if the step size is negative, the slice() method will extract the substring in reverse order. For example:
text = "Hello, World!" substring = text[::-1] print(substring)
Output:
!dlroW ,olleH
In this example, the slice() method extracts the entire string "Hello, World!" in reverse order, resulting in the substring "!dlroW ,olleH".
Code Snippet: Removing Leading and Trailing Whitespace
To remove leading and trailing whitespace from a string in Python, you can use the strip() method. Here's an example:
text = " Hello, World! " trimmed_text = text.strip() print(trimmed_text)
Output:
Hello, World!
In this example, the strip() method removes the leading and trailing spaces from the string " Hello, World! ", resulting in the trimmed string "Hello, World!".
Code Snippet: Using strip() vs. replace() in Python
Both the strip() and replace() methods can be used to remove whitespace from strings in Python, but they have subtle differences. Here's an example that demonstrates the difference between using strip() and replace():
text = " Hello, World! " trimmed_text_strip = text.strip() trimmed_text_replace = text.replace(" ", "") print(trimmed_text_strip) print(trimmed_text_replace)
Output:
Hello, World! Hello,World!
In this example, the strip() method removes the leading and trailing spaces from the string " Hello, World! ", resulting in the trimmed string "Hello, World!". On the other hand, the replace() method replaces all occurrences of spaces with an empty string, resulting in the trimmed string "Hello,World!".
The choice between using strip() and replace() depends on your specific requirements. If you only want to remove leading and trailing whitespace, strip() is more suitable. However, if you want to remove all whitespace, including spaces within the string, replace() is a better option.
Related Article: Advanced Django Admin Interface: Custom Views, Actions & Security
Code Snippet: Extracting Specific Portion of a String
To extract a specific portion of a string in Python, you can use string slicing. Here's an example:
text = "Hello, World!" substring = text[7:12] print(substring)
Output:
World
In this example, the substring() method extracts the portion of the string "Hello, World!" starting from index 7 (inclusive) and ending at index 12 (exclusive), resulting in the substring "World".
String slicing allows you to specify the range of indices to include in the extracted substring, giving you flexibility in extracting specific portions of a string.
Additional Resources
- Python String strip() Method