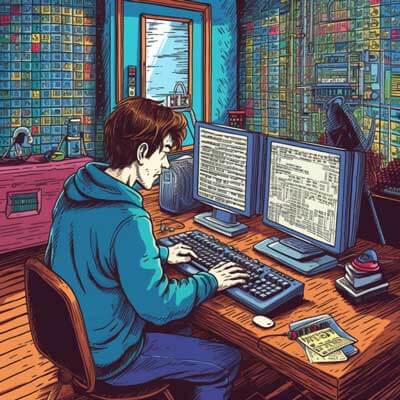
Table of Contents
The Benefits of Virtual DOM in Web Development
ReactJS, a JavaScript library developed by Facebook, has gained immense popularity in the web development community due to its numerous advantages. One of the key benefits of ReactJS is its use of a Virtual DOM (Document Object Model) which greatly enhances the performance of web applications.
Traditionally, when a user interacts with a web application, the entire HTML document must be updated and rendered in the browser. This can be a time-consuming process, especially for complex and dynamic applications. However, ReactJS introduces the concept of a Virtual DOM, which is a lightweight representation of the actual DOM.
When a component's state changes, ReactJS updates the Virtual DOM instead of the real DOM. It then calculates the difference between the previous and current state of the Virtual DOM and applies only the necessary updates to the actual DOM. This approach significantly reduces the number of updates performed on the DOM, resulting in improved performance and a smoother user experience.
Here's an example that demonstrates the use of Virtual DOM in ReactJS:
import React from 'react'; class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } incrementCount() { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <h1>{this.state.count}</h1> <button onClick={() => this.incrementCount()}>Increment</button> </div> ); } } export default Counter;
In the above code, we have a simple Counter
component that displays a count value and a button. When the button is clicked, the incrementCount
function is called, which updates the state of the component. ReactJS then efficiently updates only the necessary parts of the DOM to reflect the new state.
Related Article: Enhancing React Applications with Third-Party Integrations
Exploring Component-based Architecture in Web Development
One of the fundamental concepts in ReactJS is its component-based architecture, which allows developers to build complex user interfaces by breaking them down into smaller, reusable components.
In traditional web development, the user interface is typically built using a combination of HTML, CSS, and JavaScript. However, as the complexity of web applications increases, maintaining and managing the codebase becomes challenging. With ReactJS, developers can create modular components that encapsulate specific functionality and can be reused across different parts of the application.
Let's take a look at an example to illustrate the concept of component-based architecture in ReactJS:
import React from 'react'; class Button extends React.Component { render() { return <button>{this.props.label}</button>; } } class App extends React.Component { render() { return ( <div> <h1>Hello, ReactJS!</h1> <Button label="Click me" /> </div> ); } } export default App;
In the above code, we have two components: Button
and App
. The Button
component represents a simple button element, while the App
component serves as the main entry point of our application. The Button
component can be reused in different parts of the application by simply passing different labels as props.
This component-based approach offers several benefits. Firstly, it promotes reusability, as components can be easily reused in different parts of the application, reducing code duplication. Secondly, it improves maintainability by allowing developers to focus on individual components and their specific functionality. Lastly, it enhances the overall organization and structure of the codebase, making it easier to understand and navigate.
Achieving Reusability in ReactJS
One of the key advantages of ReactJS is its emphasis on reusability. ReactJS provides developers with the ability to create reusable components, which can be easily integrated into different parts of the application.
Reusability is achieved in ReactJS through the use of props and component composition. Props allow developers to pass data from a parent component to its child components, enabling the sharing of information between different parts of the application.
Let's consider an example to illustrate how reusability is achieved in ReactJS:
import React from 'react'; class UserCard extends React.Component { render() { return ( <div> <h1>{this.props.name}</h1> <p>{this.props.email}</p> </div> ); } } class App extends React.Component { render() { return ( <div> <UserCard name="John Doe" email="john@example.com" /> <UserCard name="Jane Smith" email="jane@example.com" /> </div> ); } } export default App;
In the above code, we have a UserCard
component that displays the name and email of a user. The App
component renders two instances of the UserCard
component, each with different data passed as props.
Understanding the Efficiency of ReactJS
Efficiency is a crucial aspect of web development, and ReactJS excels in this area. ReactJS is designed to be highly efficient, ensuring optimal performance for web applications.
One of the key factors contributing to ReactJS's efficiency is its use of a Virtual DOM, as mentioned earlier. By updating the Virtual DOM instead of the actual DOM, ReactJS minimizes the number of updates required and reduces the overall computational cost.
Additionally, ReactJS employs a diffing algorithm to efficiently determine the minimal set of changes needed to update the DOM. When a component's state changes, ReactJS compares the previous and current state of the Virtual DOM and identifies the differences. It then applies only the necessary updates to the actual DOM, resulting in improved performance.
ReactJS also utilizes a technique called "reconciliation" to efficiently update and re-render components. When a component's props or state change, ReactJS determines which components need to be updated and selectively re-renders them, rather than re-rendering the entire component tree. This approach further enhances the efficiency of ReactJS.
Related Article: Extracting URL Parameters in Your ReactJS Component
Enhancing Developer Productivity with ReactJS
ReactJS provides developers with a useful set of tools and features that greatly enhance productivity during the web development process. These features enable developers to write clean, maintainable code and streamline the development workflow.
One of the key productivity-enhancing features of ReactJS is its use of JSX. JSX is a syntax extension for JavaScript that allows developers to write HTML-like code within their JavaScript files. This simplifies the process of creating and manipulating the user interface, making it more intuitive and readable.
Here's an example that demonstrates the use of JSX in ReactJS:
import React from 'react'; class App extends React.Component { render() { return ( <div> <h1>Hello, ReactJS!</h1> <p>This is a JSX example.</p> </div> ); } } export default App;
In the above code, we can see how JSX allows us to write HTML-like code directly within the JavaScript file. This makes it easier to understand and visualize the structure of the user interface.
In addition to JSX, ReactJS also provides a rich set of developer tools, such as React Developer Tools, which enable developers to inspect and debug React components. These tools offer valuable insights into the component hierarchy, state changes, and performance optimization, further enhancing developer productivity.
The Significance of Unidirectional Data Flow in ReactJS
One of the core principles of ReactJS is its emphasis on unidirectional data flow. Unidirectional data flow ensures that data flows in a single direction, from parent components to child components, making it easier to understand and reason about the state of the application.
In ReactJS, data is passed from parent components to child components through props. Child components cannot directly modify the props received from their parent components. Instead, if a child component needs to update its state, it must communicate this to its parent component through callbacks, which in turn updates the state and propagates the changes down the component hierarchy.
This unidirectional data flow simplifies the debugging and testing process, as it eliminates the possibility of unexpected side effects due to direct manipulation of shared state. It also promotes a more predictable and maintainable codebase, as the data flow is explicit and can be easily traced.
Let's consider an example to illustrate the significance of unidirectional data flow:
import React from 'react'; class ParentComponent extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } incrementCount() { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <h1>Count: {this.state.count}</h1> <ChildComponent increment={() => this.incrementCount()} /> </div> ); } } class ChildComponent extends React.Component { render() { return <button onClick={this.props.increment}>Increment</button>; } } export default ParentComponent;
In the above code, we have a ParentComponent
and a ChildComponent
. The ParentComponent
maintains the state of a count value and defines a callback function incrementCount
to update the state. The ChildComponent
renders a button that triggers the increment
callback when clicked.
Through the unidirectional data flow, the ChildComponent
communicates the need to update the state to its parent component, which then updates the state and propagates the changes down the component hierarchy. This ensures that the state is always updated in a controlled and predictable manner.
Exploring the ReactJS Ecosystem and Its Benefits
ReactJS has a vibrant and extensive ecosystem that offers a wide range of libraries, tools, and frameworks to enhance the development experience and extend the capabilities of ReactJS.
One of the most prominent libraries in the ReactJS ecosystem is Redux. Redux is a predictable state container that helps manage the state of an application in a centralized manner. It provides a clear and structured way of managing complex application state, making it easier to reason about and maintain.
Another popular library in the ReactJS ecosystem is React Router. React Router is a routing library that allows developers to handle navigation and routing within a React application. It provides a declarative way to define routes and handle navigation between different components, enabling the creation of single-page applications with ease.
Apart from libraries, there are also numerous tools and frameworks built on top of ReactJS that further enhance its capabilities. Next.js, for example, is a framework that enables server-side rendering and static site generation with ReactJS. It provides an intuitive API and simplifies the process of building highly performant and SEO-friendly web applications.
The ReactJS ecosystem offers immense benefits to developers by providing a wide range of options to solve common development challenges. It allows developers to leverage the power of the community and benefit from the collective knowledge and expertise of other ReactJS developers.
Understanding JSX and Its Advantages in ReactJS
JSX is a syntax extension for JavaScript that allows developers to write HTML-like code within their JavaScript files. It is a fundamental part of ReactJS and offers several advantages in terms of readability, maintainability, and ease of use.
One of the key advantages of JSX is its readability. By allowing developers to write HTML-like code directly within their JavaScript files, JSX makes it easier to visualize and understand the structure of the user interface. This improves code readability and reduces the cognitive load when working with complex UI components.
Another advantage of JSX is its maintainability. Since JSX code closely resembles HTML, it is easier for developers to update and modify the user interface. Changes can be made directly within the JSX code, without the need for additional template files or complex syntax. This streamlines the development process and reduces the chances of introducing errors.
Additionally, JSX provides a seamless integration of JavaScript expressions within the HTML-like code. This allows developers to dynamically generate content, apply conditional rendering, and perform other logic directly within the JSX code. This eliminates the need for separate template syntax or complex string concatenation, making the code more concise and easier to understand.
Here's an example that demonstrates the advantages of JSX in ReactJS:
import React from 'react'; class App extends React.Component { render() { const message = 'Hello, ReactJS!'; const showButton = true; return ( <div> <h1>{message}</h1> {showButton && <button>Click me</button>} </div> ); } } export default App;
In the above code, we can see how JSX allows us to seamlessly integrate JavaScript expressions within the HTML-like code. The message
variable is used to dynamically render the content of the h1
element, and the showButton
variable is used to conditionally render the button. This concise and intuitive syntax makes the code more maintainable and easier to work with.
JSX is a useful feature of ReactJS that greatly simplifies the process of building user interfaces. Its advantages in terms of readability, maintainability, and ease of use make it an indispensable tool for ReactJS developers.
Related Article: Implementing Server Rendering with Ruby on Rails & ReactJS
Performance Optimization in ReactJS
Performance optimization is a critical aspect of web development, and ReactJS provides several techniques and best practices to ensure optimal performance for web applications.
One of the key performance optimization techniques in ReactJS is the use of shouldComponentUpdate lifecycle method. This method allows developers to control whether a component should re-render or not based on the changes in its props or state. By implementing shouldComponentUpdate and performing a shallow comparison of the props and state, unnecessary re-renders can be avoided, resulting in improved performance.
Here's an example that demonstrates the use of shouldComponentUpdate in ReactJS:
import React from 'react'; class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } shouldComponentUpdate(nextProps, nextState) { if (this.props.count !== nextProps.count) { return true; } if (this.state.count !== nextState.count) { return true; } return false; } incrementCount() { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <h1>{this.state.count}</h1> <button onClick={() => this.incrementCount()}>Increment</button> </div> ); } } export default Counter;
In the above code, the Counter
component implements the shouldComponentUpdate method to compare the current props and state with the next props and state. It only re-renders if there are changes in the count prop or the count state. This prevents unnecessary re-renders and improves the performance of the component.
Another performance optimization technique in ReactJS is the use of memoization. Memoization is the process of caching the results of expensive function calls and returning the cached result when the same inputs occur again. ReactJS provides the useMemo and useCallback hooks, which can be used to memoize expensive computations and prevent unnecessary re-computations.
Here's an example that demonstrates the use of memoization in ReactJS with the useMemo hook:
import React, { useMemo } from 'react'; function ExpensiveComponent({ data }) { const result = useMemo(() => { // Perform expensive computation based on data // ... return result; }, [data]); return <div>{result}</div>; } export default ExpensiveComponent;
In the above code, the ExpensiveComponent
uses the useMemo hook to memoize the result of an expensive computation based on the data prop. The result is only re-computed when the data prop changes, preventing unnecessary computations and improving the performance of the component.
The Contributory Role of the ReactJS Community
The ReactJS community plays a significant role in the growth and success of ReactJS as a useful web development library. The community is comprised of developers, contributors, and enthusiasts who actively contribute to the development of ReactJS, share knowledge, and provide support to fellow developers.
One of the key benefits of the ReactJS community is the availability of a vast amount of learning resources. The community has produced a wealth of tutorials, articles, videos, and documentation that cover various aspects of ReactJS and its ecosystem. These resources provide valuable insights, best practices, and real-world examples, enabling developers to quickly learn and master ReactJS.
The ReactJS community also actively maintains and contributes to a wide range of open-source libraries and tools that extend the capabilities of ReactJS. These libraries cover areas such as state management, routing, form handling, and UI components, among others. By leveraging these community-driven libraries, developers can save time and effort, as they don't have to reinvent the wheel and can benefit from the collective expertise of the community.
Additionally, the ReactJS community fosters a collaborative and supportive environment. Developers can seek help, ask questions, and share their knowledge through various online platforms and communities, such as Stack Overflow, Reddit, and Discord. This collaborative nature of the community encourages learning, knowledge sharing, and continuous improvement.
Furthermore, the ReactJS community actively engages with the ReactJS development team, providing feedback, reporting issues, and suggesting enhancements. This close collaboration ensures that ReactJS remains a robust and reliable web development library, addressing the needs and challenges faced by developers in real-world scenarios.