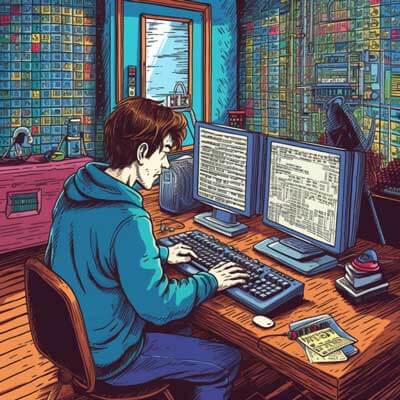
Table of Contents
The Process of Rendering in React
Rendering is the process in React where components are transformed into a representation that can be displayed on the screen. It involves creating a virtual representation of the user interface, comparing it with the previous representation, and updating the necessary parts of the actual DOM.
When a component is rendered in React, it follows a series of steps to generate the final output:
1. The component's render method is called, which returns a JSX representation of the component's output.
2. React converts the JSX into a React element, which is a plain JavaScript object.
3. React then creates a virtual representation of the component's output called the Virtual DOM.
4. React compares the new Virtual DOM with the previous Virtual DOM to determine the minimum number of changes required to update the actual DOM.
5. React updates the necessary parts of the actual DOM based on the changes identified in the previous step.
The rendering process in React is efficient because it minimizes the number of actual DOM manipulations. By using the Virtual DOM, React can batch updates and perform them in an optimized way.
Related Article: How to Update a State Property in ReactJS
Understanding Re-rendering
Re-rendering is the process in React where a component's output is updated due to changes in its state or props. It involves calling the component's render method again to generate a new output, comparing it with the previous output, and updating the necessary parts of the actual DOM.
When a component is re-rendered in React, it follows a similar process as the initial rendering:
1. The component's render method is called again, which returns a new JSX representation of the component's output.
2. React converts the new JSX into a new React element.
3. React then creates a new virtual representation of the component's output.
4. React compares the new virtual representation with the previous virtual representation to determine the minimum number of changes required to update the actual DOM.
5. React updates the necessary parts of the actual DOM based on the changes identified in the previous step.
Re-rendering in React is triggered automatically when a component's state or props change. React intelligently determines which parts of the output need to be updated and performs the necessary updates in an efficient way.
It's important to note that not all changes in state or props will trigger a re-render. React uses a reconciliation algorithm to determine if a re-render is necessary. This algorithm is optimized to minimize the number of updates and improve rendering performance.
How the Render Method Works
The render
method is a special method in React components that is responsible for generating the output of the component. It is called whenever a component needs to be rendered or re-rendered.
The render
method must return a JSX element or null
. JSX is a syntax extension for JavaScript that allows you to write HTML-like code directly in your JavaScript files.
Inside the render
method, you can use JavaScript expressions and logic to dynamically generate the content of the JSX element. You can access the component's props and state, and use them to determine what should be rendered.
Let's take a look at an example of how the render
method works in a class component:
import React from 'react'; class Greeting extends React.Component { render() { const name = this.props.name; return <h1>Hello, {name}!</h1>; } }
In this example, the render
method of the Greeting
class component returns a JSX element that represents a heading with the text "Hello, {name}!". The value of {name}
is determined by the value of the name
prop passed to the component.
The render
method is called automatically by React whenever a component needs to be rendered or re-rendered. It is responsible for generating the output of the component based on its props and state.
It's important to note that the render
method should be a pure function, meaning that it should not have any side effects or modify the component's state or props. It should only return a new JSX element based on the current state and props of the component.
The Purpose of the Render Method
The purpose of the render
method in React is to generate the output of a component. It is a required method in class components and is responsible for returning a JSX element or null
.
The render
method is called whenever a component needs to be rendered or re-rendered. It is called automatically by React and should not be called manually.
The render
method is a pure function, meaning that it should not have any side effects or modify the component's state or props. It should only return a new JSX element based on the current state and props of the component.
The output of the render
method is used by React to generate the actual DOM elements that are displayed on the screen. React creates a virtual representation of the component's output called the Virtual DOM, compares it with the previous Virtual DOM, and updates the necessary parts of the actual DOM.
The render
method is a key part of the rendering process in React and is responsible for generating the visual representation of a component based on its current state and props.
Related Article: Crafting a Function within Render in ReactJS
Parameters of the Render Method
The render
method in React does not take any parameters. It is a special method in class components that is automatically called by React whenever a component needs to be rendered or re-rendered.
The render
method is responsible for returning a JSX element or null
that represents the output of the component. It should be a pure function, meaning that it should only return a new JSX element based on the current state and props of the component.
Here's an example of a class component with a render
method:
import React from 'react'; class Greeting extends React.Component { render() { const name = this.props.name; return <h1>Hello, {name}!</h1>; } }
In this example, the render
method of the Greeting
class component returns a JSX element that represents a heading with the text "Hello, {name}!". The value of {name}
is determined by the value of the name
prop passed to the component.
The render
method does not take any parameters because it has access to the component's props and state through the this.props
and this.state
objects.
It's important to note that the render
method should not have any side effects or modify the component's state or props. It should only return a new JSX element based on the current state and props of the component.
Optimizing the Render Method
The render
method in React is a critical part of the rendering process and can have a significant impact on the performance of your application. By optimizing the render
method, you can improve the rendering speed and efficiency of your components.
Here are some tips for optimizing the render
method in React:
1. Avoid unnecessary re-renders: React re-renders a component whenever its state or props change. To avoid unnecessary re-renders, use the shouldComponentUpdate
lifecycle method to compare the current props and state with the next props and state, and return false
if they are the same.
2. Use memoization: Memoization is a technique that allows you to cache the result of an expensive computation and reuse it when the same inputs occur again. You can use the React.memo
higher-order component or the useMemo
hook to memoize the result of the render
method and prevent unnecessary re-renders.
3. Split complex components into smaller ones: If a component has a large and complex render
method, consider splitting it into smaller, more manageable components. This can improve the readability, maintainability, and performance of your code.
4. Use key props in lists: When rendering lists of items, make sure to provide a unique key
prop to each item. This allows React to efficiently update and reorder the list when items are added, removed, or rearranged.
5. Use pure components: Pure components are a performance optimization in React that automatically implement a shallow comparison of props and state in the shouldComponentUpdate
method. Using pure components can reduce unnecessary re-renders and improve performance.
Optimizing the render
method in React is an important step in improving the performance of your application. By following these tips, you can ensure that your components render efficiently and provide a smooth user experience.
Multiple Render Methods in a React Component
In React, a component can have multiple methods that are responsible for generating different parts of its output. These methods are often referred to as "render methods" and are used to encapsulate the rendering logic of different parts of the component.
Here's an example of a class component with multiple render methods:
import React from 'react'; class Profile extends React.Component { renderName() { const { firstName, lastName } = this.props; return <h1>{firstName} {lastName}</h1>; } renderBio() { const { bio } = this.props; return <p>{bio}</p>; } render() { return ( <div> {this.renderName()} {this.renderBio()} </div> ); } }
In this example, the Profile
class component has two additional render methods: renderName
and renderBio
. These methods encapsulate the rendering logic for the name and bio sections of the profile.
The render
method of the Profile
component then calls these render methods to generate the final output of the component.
Using multiple render methods allows you to separate the rendering logic of different parts of a component and make your code more modular and reusable.
React's Update Handling for State and Props
React provides a mechanism for handling updates to a component's state and props. When a component's state or props change, React automatically triggers a re-render of the component and updates the necessary parts of the actual DOM.
In React, updates to state or props are handled in the following way:
1. State updates: When the setState
method is called in a component, React schedules a re-render of the component. The new state is merged with the previous state, and the render
method is called again to generate the updated output.
2. Prop updates: When a component receives new props from its parent component, React schedules a re-render of the component. The new props are merged with the previous props, and the render
method is called again to generate the updated output.
React uses a reconciliation algorithm to determine the minimum number of changes required to update the actual DOM. It compares the new virtual representation of the component's output with the previous virtual representation and identifies the necessary changes.
React then updates the necessary parts of the actual DOM based on the changes identified in the previous step. This process is efficient because React uses the Virtual DOM to batch updates and perform them in an optimized way.
React's update handling for state and props is a key feature that enables components to be dynamic and interactive. It allows components to respond to user input, network requests, and other asynchronous events, resulting in a more engaging user experience.
Related Article: Accessing Array Length in this.state in ReactJS
Virtual DOM vs. Actual DOM
In React, there are two representations of the user interface: the Virtual DOM and the actual DOM. The Virtual DOM is an abstraction layer that allows React to perform updates and optimizations efficiently.
The Virtual DOM is a lightweight copy of the actual DOM. It is a tree-like structure that represents the current state of the user interface. When a component's state or props change, React updates the Virtual DOM instead of directly manipulating the actual DOM.
React then compares the updated Virtual DOM with the previous Virtual DOM and determines the minimum number of changes required to update the actual DOM. This process is known as reconciliation.
Once the necessary changes have been identified, React updates the necessary parts of the actual DOM. This process is more efficient than directly manipulating the actual DOM because updating the actual DOM can be a costly operation.
Here's a simplified example to illustrate the difference between the Virtual DOM and the actual DOM:
import React from 'react'; import ReactDOM from 'react-dom'; class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } handleClick() { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.handleClick()}>Increment</button> </div> ); } } ReactDOM.render(<Counter />, document.getElementById('root'));
In this example, we have a Counter
class component that manages a count
state variable. When the button is clicked, the count
state is updated using the setState
method.
React updates the Virtual DOM based on the new state, compares it with the previous Virtual DOM, and determines the minimum number of changes required to update the actual DOM. In this case, only the Count
component needs to be updated with the new value of count
.
CSS-in-JS
CSS-in-JS is a technique in React that allows you to write CSS code directly in your JavaScript files. It provides a way to encapsulate styles and make them more modular and reusable.
CSS-in-JS libraries, such as styled-components or Emotion, enable you to define styles as JavaScript objects or template literals. These styles are then applied to the corresponding React components.
Let's take a look at an example of using styled-components to apply CSS styles in React:
import React from 'react'; import styled from 'styled-components'; const Button = styled.button` background-color: blue; color: white; padding: 8px 16px; border-radius: 4px; `; function App() { return ( <div> <h1>Welcome to React</h1> <Button>Click me</Button> </div> ); }
In this example, we use the styled-components
library to define a Button
component with CSS styles. The CSS styles are defined using a template literal syntax, and they are applied to the button
element.
The Button
component can then be used in other components, and the CSS styles will be automatically applied. This allows you to create reusable and self-contained components with their own styles.
CSS-in-JS provides several benefits over traditional CSS stylesheets. It eliminates class name collisions, allows for dynamic styles based on props or state, and enables better code organization and modularity.
CSS-in-JS is a useful technique that is widely used in React applications to style components in a more efficient and maintainable way.
Common Use Cases for CSS-in-JS
CSS-in-JS is a popular technique in React that allows you to write CSS code directly in your JavaScript files. It provides a way to encapsulate styles and make them more modular and reusable.
CSS-in-JS libraries, such as styled-components or Emotion, enable you to define styles as JavaScript objects or template literals. These styles are then applied to the corresponding React components.
There are several common use cases for CSS-in-JS in React:
1. Component-specific styles: CSS-in-JS allows you to define styles that are specific to a particular component. This makes it easier to manage and organize styles, especially in large codebases with many components.
2. Dynamic styles: CSS-in-JS enables you to create dynamic styles based on props or state. You can use JavaScript expressions and logic to generate styles that change dynamically based on different conditions.
3. Scoped styles: CSS-in-JS provides a way to encapsulate styles within a component. This prevents style leakage and class name collisions, making it easier to maintain and reason about your styles.
4. Theming: CSS-in-JS libraries often provide built-in support for theming. This allows you to define a set of theme variables or styles that can be easily applied to different components throughout your application.
5. Server-side rendering: CSS-in-JS libraries are designed to work well with server-side rendering. They ensure that the styles are correctly rendered on the server and applied to the components when they are hydrated on the client.
CSS-in-JS is a useful technique in React that provides a more efficient and maintainable way to style components. It allows you to leverage the full power of JavaScript to create dynamic and reusable styles for your application.
The Impact of Lifecycle Methods on Rendering
Lifecycle methods in React can have a significant impact on the rendering process and performance of your components. They allow you to control the behavior and appearance of your components at different stages of their existence.
Lifecycle methods can affect rendering in the following ways:
1. Mounting methods: Mounting methods are called when a component is being created and inserted into the DOM. They can be used to perform initialization tasks, such as fetching data from a server or setting up event listeners. Mounting methods can affect the initial rendering of a component.
2. Updating methods: Updating methods are called when a component is being re-rendered due to changes in props or state. They can be used to determine whether a component should update or not, or to perform side effects before or after an update. Updating methods can affect the efficiency of the rendering process.
3. Unmounting methods: Unmounting methods are called when a component is being removed from the DOM. They can be used to clean up resources, such as canceling network requests or removing event listeners. Unmounting methods can affect the cleanup of a component after it has been rendered.
Related Article: How to Fetch and Post Data to a Server Using ReactJS
Understanding State
State is an important concept in React that allows components to manage and store data that can change over time. It represents the current state of a component and determines how it should render and behave.
In React, state is managed by the useState
hook or by using a class component's state
property. The useState
hook is a newer feature introduced in React 16.8 that allows functional components to have state.
Let's take a look at an example of using state in a functional component with the useState
hook:
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }
In this example, we define a functional component called Counter
that uses the useState
hook to manage a count
state variable. The useState
hook returns an array with two elements: the current state value (count
) and a function to update the state (setCount
). We can use the setCount
function to update the count
state variable.
The count
state variable is then rendered in the component's output, and the setCount
function is used as the onClick
handler for a button. When the button is clicked, the count
state is incremented by 1.
State is an important concept in React as it allows components to be dynamic and interactive. It enables components to respond to user input, network requests, and other asynchronous events.
Working with Props
Props (short for properties) are a mechanism in React for passing data from a parent component to a child component. They allow components to be reusable and modular by providing a way to customize their behavior and appearance.
In React, props are passed as attributes to the child component when it is included in the parent component's JSX. The child component can then access the props through its function or class parameters.
Let's take a look at an example of using props in a functional component:
import React from 'react'; function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } function App() { return <Greeting name="React" />; }
In this example, we define a functional component called Greeting
that accepts a name
prop. The value of the name
prop is then rendered in the component's output.
The Greeting
component is then included in another functional component called App
, and the name
prop is set to "React". When the App
component is rendered, it will display the Greeting
component with the text "Hello, React!".
Props can also be used in class components. Here's an example of using props in a class component:
import React from 'react'; class Greeting extends React.Component { render() { return <h1>Hello, {this.props.name}!</h1>; } } class App extends React.Component { render() { return <Greeting name="React" />; } }
In this example, the name
prop is accessed through the this.props
object in the Greeting
class component.
Props are a useful mechanism in React that enable components to be flexible and reusable. They allow components to be configured and customized based on the specific needs of each use case.
Exploring the Virtual DOM
The Virtual DOM (VDOM) is a concept in React that represents a virtual representation of the actual DOM. It is an abstraction layer that allows React to perform efficient updates and optimizations.
In React, when a component's state or props change, React updates the VDOM instead of directly manipulating the actual DOM. React then compares the updated VDOM with the previous VDOM and determines the minimum number of changes required to update the actual DOM.
This approach is more efficient than directly manipulating the actual DOM because updating the actual DOM can be a costly operation. By using the VDOM, React can batch updates and perform them in a more optimized way.
Let's take a look at an example to understand how the VDOM works:
import React from 'react'; import ReactDOM from 'react-dom'; class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } handleClick() { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.handleClick()}>Increment</button> </div> ); } } ReactDOM.render(<Counter />, document.getElementById('root'));
In this example, we have a Counter
class component that manages a count
state variable. When the button is clicked, the count
state is updated using the setState
method.
React then updates the VDOM, compares it with the previous VDOM, and determines the minimum number of changes required to update the actual DOM. In this case, only the Count
component needs to be updated with the new value of count
.
Utilizing JSX
JSX is a syntax extension for JavaScript that allows you to write HTML-like code directly in your JavaScript files. It is a key feature of React and provides a concise and expressive way to define the structure and content of components.
JSX looks similar to HTML, but it is actually transpiled to JavaScript by the Babel compiler. JSX elements are represented as plain JavaScript objects called React elements.
Let's take a look at an example of using JSX in a React component:
import React from 'react'; function Greeting() { return ( <div> <h1>Hello, React!</h1> <p>Welcome to the world of JSX.</p> </div> ); }
In this example, we define a functional component called Greeting
that returns JSX. The JSX code represents a <div>
element with an <h1>
heading and a <p>
paragraph.
JSX allows you to include JavaScript expressions inside curly braces {}
. This allows you to dynamically generate the content of JSX elements based on variables, props, or state.
Here's an example of using JavaScript expressions in JSX:
import React from 'react'; function Greeting(props) { const name = props.name; return ( <div> <h1>Hello, {name}!</h1> <p>Welcome to the world of JSX.</p> </div> ); }
In this example, we define a functional component called Greeting
that accepts a name
prop. The value of the name
prop is then used in the JSX expression to dynamically generate the content of the <h1>
element.
JSX is a useful feature of React that makes it easy to create and manipulate the structure and content of components. It combines the power of JavaScript with the simplicity and expressiveness of HTML-like syntax.
Related Article: How to Implement Hover State in ReactJS with Inline Styles
Understanding Reconciliation
Reconciliation is the process in React where it compares the previous state of the VDOM with the updated state and determines the minimum number of changes required to update the actual DOM.
When a component's state or props change, React creates a new VDOM tree and performs a diffing algorithm to find the differences between the new VDOM and the previous VDOM.
React then updates the actual DOM with only the necessary changes, resulting in a more efficient rendering process.
Let's take a look at an example to understand how reconciliation works:
import React from 'react'; import ReactDOM from 'react-dom'; class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } handleClick() { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.handleClick()}>Increment</button> </div> ); } } ReactDOM.render(<Counter />, document.getElementById('root'));
In this example, we have a Counter
class component that manages a count
state variable. When the button is clicked, the count
state is updated using the setState
method.
React then creates a new VDOM tree, compares it with the previous VDOM tree, and determines the minimum number of changes required to update the actual DOM. In this case, only the Count
component needs to be updated with the new value of count
.
React then updates the actual DOM with only the necessary changes, resulting in an efficient rendering process.
Reconciliation is an important process in React as it allows components to be updated efficiently and minimizes the impact on performance. It is one of the key reasons why React is able to provide a smooth and responsive user experience.
Exploring Lifecycle Methods
Lifecycle methods are special methods in React components that are invoked at specific points in the lifecycle of a component. They allow you to perform actions or handle events at different stages of a component's existence.
In React, there are three main categories of lifecycle methods: Mounting, Updating, and Unmounting.
Mounting methods are called when an instance of a component is being created and inserted into the DOM. They are called in the following order:
- constructor()
- static getDerivedStateFromProps()
- render()
- componentDidMount()
Updating methods are called when a component is being re-rendered due to changes in props or state. They are called in the following order:
- static getDerivedStateFromProps()
- shouldComponentUpdate()
- render()
- getSnapshotBeforeUpdate()
- componentDidUpdate()
Unmounting methods are called when a component is being removed from the DOM. They are called in the following order:
- componentWillUnmount()
Let's take a look at an example to understand how lifecycle methods work:
import React from 'react'; class Timer extends React.Component { constructor(props) { super(props); this.state = { seconds: 0 }; } componentDidMount() { this.interval = setInterval(() => { this.setState({ seconds: this.state.seconds + 1 }); }, 1000); } componentWillUnmount() { clearInterval(this.interval); } render() { return <p>Seconds: {this.state.seconds}</p>; } }
In this example, we have a Timer
class component that displays the number of seconds that have elapsed since the component was mounted. The componentDidMount
method is called after the component is inserted into the DOM, and it starts an interval that updates the seconds
state every second.
The componentWillUnmount
method is called before the component is removed from the DOM, and it clears the interval to prevent memory leaks.
Lifecycle methods allow you to handle different stages of a component's existence and perform actions or handle events at the appropriate times. They are a useful tool in React that enables you to control the behavior and appearance of your components.
Additional Resources
- Understanding JSX in React