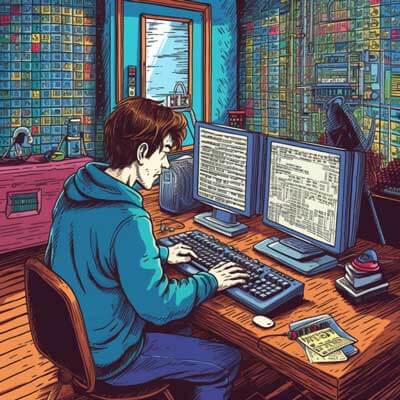
Table of Contents
Defining the Problem
When working with TypeScript, you may often come across situations where you need to convert a string value to a boolean value. This can be required when processing user input, working with API responses, or manipulating data within your application. Converting a string to a boolean is not as straightforward as it may seem, as there are various considerations and edge cases to take into account.
In this tutorial, we will explore different approaches and techniques for converting a string to a boolean in TypeScript. We will discuss the underlying boolean data type, common use cases for string to bool conversion, built-in functions, type assertion, regular expressions, handling truthy and falsy values, case sensitivity, external libraries, performance considerations, caveats and limitations, and best practices.
Related Article: Tutorial on TypeScript Dynamic Object Manipulation
Understanding Boolean Data Type
Before diving into string to bool conversion, it's important to understand the boolean data type in TypeScript. A boolean value can be either true or false, representing the logical values of "true" or "false" respectively. Booleans are commonly used in conditional statements, comparisons, and boolean algebra.
In TypeScript, the boolean data type is denoted by the keyword "boolean". You can declare a boolean variable and assign it a value using the syntax:
let isTrue: boolean = true; let isFalse: boolean = false;
Common Use Cases for Converting String to Bool
There are several common use cases where you may need to convert a string to a boolean in TypeScript. Some of these include:
1. Processing user input: When accepting user input, such as checkboxes or radio buttons, the value is often returned as a string. Converting this string to a boolean allows for easier manipulation and comparison.
2. API responses: When working with APIs, boolean values are often represented as strings. Converting these string representations to actual booleans is necessary for proper data handling and logic.
3. Data manipulation: String values within your application's data may need to be converted to boolean for processing or filtering purposes.
Built-in Functions for Converting String to Bool
TypeScript provides built-in functions that can be used to convert a string to a boolean. Two commonly used functions are:
1. Boolean()
: The Boolean()
function is a built-in JavaScript function that can be used to convert a value to a boolean. It can be used with a string parameter to convert the string to a boolean value.
let str: string = "true"; let boolValue: boolean = Boolean(str); console.log(boolValue); // Output: true
2. JSON.parse()
: The JSON.parse()
function is another built-in JavaScript function that can be used to convert a JSON string to a JavaScript object. When used with a string that represents a boolean value, it will return the corresponding boolean value.
let str: string = "false"; let boolValue: boolean = JSON.parse(str); console.log(boolValue); // Output: false
Related Article: How to Convert a String to a Number in TypeScript
Using Type Assertion to Convert String to Bool
Type assertion is a way to tell the TypeScript compiler that you know more about the type of a value than it does. It is denoted by the syntax value
or value as Type
. Type assertion can be used to convert a string to a boolean by explicitly specifying the boolean type.
let str: string = "true"; let boolValue: boolean = str; console.log(boolValue); // Output: true
Alternatively, you can use the as
keyword for type assertion:
let str: string = "false"; let boolValue: boolean = str as boolean; console.log(boolValue); // Output: false
Using Regular Expressions to Convert String to Bool
Regular expressions can be used to match and extract specific patterns from strings. They can also be used to convert string representations of boolean values to actual boolean values.
function convertStringToBool(str: string): boolean { const trueRegex = /^true$/i; // Matches "true" (case-insensitive) const falseRegex = /^false$/i; // Matches "false" (case-insensitive) if (trueRegex.test(str)) { return true; } else if (falseRegex.test(str)) { return false; } else { throw new Error("Invalid boolean string"); } }
Usage:
let str1: string = "true"; let boolValue1: boolean = convertStringToBool(str1); console.log(boolValue1); // Output: true let str2: string = "false"; let boolValue2: boolean = convertStringToBool(str2); console.log(boolValue2); // Output: false let str3: string = "invalid"; let boolValue3: boolean = convertStringToBool(str3); // Throws an error
Handling Truthy and Falsy Values in String to Bool Conversion
When converting a string to a boolean, it's important to consider how truthy and falsy values are handled. In JavaScript and TypeScript, certain values are considered "truthy" or "falsy" based on their inherent truth value.
The following values are considered falsy:
- false
- 0
- -0
- ""
- null
- undefined
- NaN
All other values are considered truthy. This can affect the conversion of string values to boolean.
let str1: string = "true"; let boolValue1: boolean = Boolean(str1); console.log(boolValue1); // Output: true let str2: string = "0"; let boolValue2: boolean = Boolean(str2); console.log(boolValue2); // Output: false
Converting String 'true' and 'false' to Bool
When converting the string values "true" and "false" to a boolean, you can use the built-in functions or type assertion methods discussed earlier.
Using the Boolean()
function:
let str1: string = "true"; let boolValue1: boolean = Boolean(str1); console.log(boolValue1); // Output: true let str2: string = "false"; let boolValue2: boolean = Boolean(str2); console.log(boolValue2); // Output: false
Using type assertion:
let str1: string = "true"; let boolValue1: boolean = str1; console.log(boolValue1); // Output: true let str2: string = "false"; let boolValue2: boolean = str2 as boolean; console.log(boolValue2); // Output: false
Related Article: Tutorial: Navigating the TypeScript Exit Process
Converting Other Strings to Bool
When converting strings other than "true" and "false" to a boolean, the result will depend on the truthiness or falsiness of the string value. Strings that are not empty will be considered truthy, while empty strings will be considered falsy.
let str1: string = "hello"; let boolValue1: boolean = Boolean(str1); console.log(boolValue1); // Output: true let str2: string = ""; let boolValue2: boolean = Boolean(str2); console.log(boolValue2); // Output: false
Handling Case Sensitivity in String to Bool Conversion
To handle case insensitivity in string to bool conversion, you can use regular expressions with the "i" flag to perform a case-insensitive match.
function convertStringToBool(str: string): boolean { const trueRegex = /^true$/i; const falseRegex = /^false$/i; if (trueRegex.test(str)) { return true; } else if (falseRegex.test(str)) { return false; } else { throw new Error("Invalid boolean string"); } }
Usage:
let str1: string = "True"; let boolValue1: boolean = convertStringToBool(str1); console.log(boolValue1); // Output: true let str2: string = "False"; let boolValue2: boolean = convertStringToBool(str2); console.log(boolValue2); // Output: false
Using External Libraries for String to Bool Conversion
If you require more advanced string to bool conversion functionality or need to handle specific edge cases, you can consider using external libraries. There are several TypeScript libraries available that provide utility functions for string to bool conversion.
One popular library is "lodash", which provides a wide range of utility functions, including a function for converting strings to booleans.
npm install lodash
Usage:
import { parseBoolean } from "lodash"; let str1: string = "true"; let boolValue1: boolean = parseBoolean(str1); console.log(boolValue1); // Output: true let str2: string = "false"; let boolValue2: boolean = parseBoolean(str2); console.log(boolValue2); // Output: false
Performance Considerations for String to Bool Conversion
When performing string to bool conversion, it's important to consider the performance implications, especially when working with large datasets or in performance-critical scenarios.
In general, the built-in functions Boolean()
and JSON.parse()
are efficient and performant for string to bool conversion. Type assertion and regular expressions can also be performant, but the complexity of the regular expression pattern can impact performance.
External libraries may have their own performance characteristics, so it's important to evaluate their performance impact before integrating them into your project.
Related Article: How to Implement and Use Generics in Typescript
Caveats and Limitations of String to Bool Conversion
There are a few caveats and limitations to keep in mind when converting a string to a boolean in TypeScript:
1. String values other than "true" and "false" will be converted based on their truthiness or falsiness. This may not always match the desired behavior.
2. Case sensitivity can affect string to bool conversion. By default, JavaScript and TypeScript are case-sensitive languages, so "True" or "False" will not be recognized as boolean values. Regular expressions can be used to handle case insensitivity.
3. The built-in functions and techniques discussed in this tutorial may not handle all edge cases or specific requirements. In such cases, you may need to implement custom logic or use external libraries.
Best Practices for Converting String to Bool
When converting a string to a boolean in TypeScript, it's important to follow best practices to ensure accurate and reliable results:
1. Use built-in functions like Boolean()
or JSON.parse()
for simple string to bool conversion.
2. Consider using type assertion or regular expressions for more specific or complex conversion requirements.
3. Handle truthy and falsy values appropriately to avoid unexpected results.
4. Pay attention to case sensitivity and consider using regular expressions with the "i" flag for case-insensitive matching.
5. Evaluate the performance implications of your chosen conversion technique, especially when working with large datasets or in performance-critical scenarios.
External Sources
- TypeScript Handbook - Type Assertions