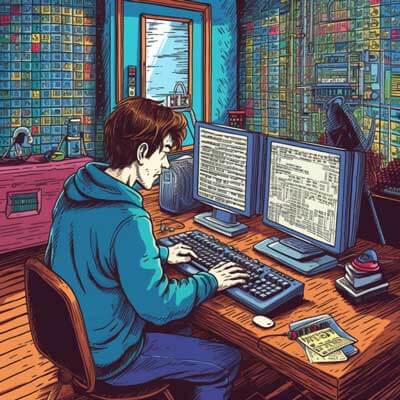
Table of Contents
What is a Gitignore file?
A Gitignore file is a text file that specifies which files or directories should be ignored by Git. When you initialize a Git repository in a directory, Git tracks all the files and directories within that directory. However, there are certain files or directories that you may not want Git to track. These could be generated files, build artifacts, sensitive information, or temporary files. By creating a Gitignore file and specifying the files or directories to ignore, you can prevent them from being committed to the repository.
The Gitignore file uses simple pattern matching rules to determine which files or directories should be ignored. It supports wildcards, negation, and comments to provide flexibility in specifying what to ignore. The Gitignore file can be placed in the root directory of the Git repository or in any subdirectory to specify the files or directories to ignore within that directory and its subdirectories.
Related Article: Tutorial: Working with Dynamic Object Keys in TypeScript
Example of a Gitignore file:
# Ignore build artifactsdist/build/# Ignore log files*.log# Ignore sensitive informationsecrets.txt# Ignore temporary files*.tmp
In this example, the Gitignore file specifies that the dist/
and build/
directories should be ignored, as well as any files with the .log
or .tmp
extension. Additionally, the secrets.txt
file is also ignored.
It's important to note that the Gitignore file only affects the local repository and does not remove ignored files that have already been committed. If you want to completely remove ignored files from the repository history, you'll need to use Git's history rewriting tools, such as git filter-branch
or git rebase
.
For more information on Gitignore patterns and rules, you can refer to the official Git documentation on Gitignore: https://git-scm.com/docs/gitignore
Why is Gitignore important in TypeScript projects?
Gitignore is particularly important in TypeScript projects due to the nature of the TypeScript language and the build process involved. TypeScript is a statically typed superset of JavaScript that compiles down to plain JavaScript. When working on a TypeScript project, you typically have a source directory where you write your TypeScript code, and a build directory where the compiled JavaScript code is generated.
Ignoring the build directory is essential because it contains generated files that can be easily reconstructed from the source code. Including the build directory in the Git repository would lead to unnecessary duplication and bloat. It can also cause conflicts when multiple developers are working on the same project, as the build artifacts may differ between different environments.
In addition to the build directory, there are other files and directories in a TypeScript project that should be ignored. For example, the node_modules
directory, which contains the dependencies installed by npm (Node Package Manager), should be ignored. This directory can be large and contains files that can be easily regenerated using the package.json file and the npm install command.
Creating a Gitignore file for TypeScript projects
To create a Gitignore file for a TypeScript project, you can follow these steps:
1. Open a text editor or your integrated development environment (IDE) and create a new file in the root directory of your project.
2. Save the file with the name .gitignore
. The leading dot (.) indicates that it is a hidden file, which is a convention used by Git for Gitignore files.
3. Specify the files or directories that should be ignored using Gitignore patterns. These patterns can include wildcards, negation, and comments.
Related Article: How to Set Default Values in TypeScript
Example of a basic Gitignore file for a TypeScript project:
# Ignore build artifactsdist/build/# Ignore log files*.log# Ignore sensitive informationsecrets.txt# Ignore temporary files*.tmp
In this example, the Gitignore file specifies that the dist/
and build/
directories should be ignored, as well as any files with the .log
or .tmp
extension. Additionally, the secrets.txt
file is also ignored.
It's important to note that the Gitignore file should be committed to the repository so that all developers working on the project have the same set of ignored files and directories.
Common patterns used in a Gitignore file
Gitignore patterns are used to specify which files or directories should be ignored. These patterns support wildcards, negation, and comments to provide flexibility in specifying what to ignore. Here are some common patterns used in a Gitignore file:
- Wildcards: Wildcards can be used to match multiple files or directories with similar names. The *
character is used to represent any number of characters, while the ?
character is used to represent a single character. For example, *.log
matches any file with the .log
extension, and temp?.txt
matches temp1.txt
, temp2.txt
, and so on.
- Directory patterns: To ignore an entire directory and its contents, you can simply specify the directory name followed by a slash (/). For example, dist/
ignores the dist
directory and all its contents.
- Negation: Negation can be used to exclude specific files or directories from being ignored. The !
character is used to negate a pattern. For example, !important.log
negates the pattern *.log
and includes the important.log
file in the repository.
- Comments: Comments in a Gitignore file start with the #
character. They are used to provide explanations or document the purpose of specific patterns. Comments are ignored by Git and have no effect on the ignored files.
Example of Gitignore patterns:
# Ignore all files with the .tmp extension*.tmp# Ignore the dist directory and its contentsdist/# Include important.log file even though it matches the *.log pattern!important.log
In this example, all files with the .tmp
extension are ignored, the dist
directory and its contents are ignored, and the important.log
file is included in the repository.
For more information on Gitignore patterns and rules, you can refer to the official Git documentation on Gitignore: https://git-scm.com/docs/gitignore
Best practices for using Gitignore in TypeScript
When using Gitignore in TypeScript projects, there are some best practices that you can follow to ensure that your repository remains clean and focused on the actual source code. Here are some best practices for using Gitignore in TypeScript:
1. Keep the Gitignore file in the root directory of your project: Placing the Gitignore file in the root directory ensures that it applies to the entire project and all its subdirectories. This helps to keep the repository clean and consistent across different environments.
2. Use specific patterns: Instead of ignoring individual files, it's often better to use patterns to ignore entire directories or types of files. For example, dist/
ignores the dist
directory and all its contents, and *.log
ignores all files with the .log
extension.
3. Use wildcards sparingly: While wildcards can be useful for ignoring multiple files or directories with similar names, they should be used sparingly to avoid accidentally ignoring important files. Make sure to review the ignored files and test the build process to ensure that no essential files are being ignored.
4. Consider using global Gitignore: If you find yourself specifying the same patterns in multiple Gitignore files across different projects, you can consider using a global Gitignore file. This file applies to all Git repositories on your machine and can save you from duplicating patterns.
5. Regularly review and update the Gitignore file: As the project evolves and new files or directories are added, it's important to review and update the Gitignore file accordingly. Regularly checking the repository status and reviewing the ignored files can help prevent accidentally committing important files.
Related Article: How to Implement and Use Generics in Typescript
Examples of Gitignore files for TypeScript projects
Here are two examples of Gitignore files for TypeScript projects:
Example 1: Basic Gitignore file for a TypeScript project:
# Ignore build artifactsdist/build/# Ignore log files*.log# Ignore sensitive informationsecrets.txt# Ignore temporary files*.tmp
In this example, the Gitignore file ignores the dist/
and build/
directories, as well as any files with the .log
or .tmp
extension. The secrets.txt
file is also ignored.
Example 2: Advanced Gitignore file for a TypeScript project with additional patterns:
# Ignore build artifactsdist/build/# Ignore log files*.log# Ignore sensitive informationsecrets.txt# Ignore temporary files*.tmp# Ignore editor-specific files and directories.vscode/.idea/# Ignore package-lock.json generated by npmpackage-lock.json# Ignore TypeScript declaration files*.d.ts# Ignore test coverage reportscoverage/
In this example, the Gitignore file includes additional patterns to ignore editor-specific files and directories (vscode/
and .idea/
), the package lock file generated by npm (package-lock.json
), TypeScript declaration files (*.d.ts
), and test coverage reports (coverage/
).
Files and folders that should always be ignored in a TypeScript project
When working on a TypeScript project, there are certain files and folders that should always be ignored to keep the repository clean and focused on the source code. Here are some files and folders that should always be ignored in a TypeScript project:
- Build artifacts: The dist/
or build/
directories that contain the compiled JavaScript code should be ignored. These directories can be easily regenerated from the TypeScript source code.
- Editor-specific files and folders: Files and folders specific to your code editor or IDE, such as .vscode/
or .idea/
, should be ignored. These files typically include project settings or temporary files generated by the editor.
- Package lock file: The package-lock.json
file generated by npm should be ignored. This file is automatically generated and contains detailed information about the dependencies installed in the node_modules
directory.
- TypeScript declaration files: Declaration files with the .d.ts
extension should be ignored. These files are generated by the TypeScript compiler and contain type information for external libraries or modules.
- Test coverage reports: If your project includes automated tests and generates test coverage reports, the coverage/
directory should be ignored. These reports can be easily regenerated and can take up significant space in the repository.
It's important to regularly review the ignored files and folders to ensure that no essential files are being ignored.