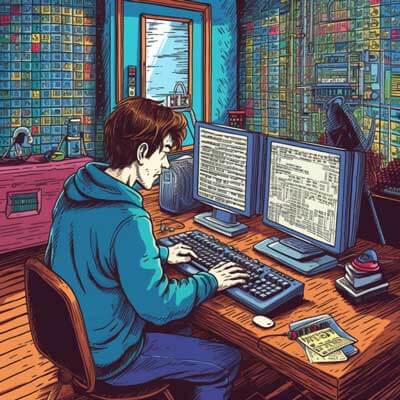
Table of Contents
Introduction
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It provides a command-line interface called redis-cli that allows you to interact with the Redis server using various commands. In this tutorial, we will explore how to install and use redis-cli to perform common operations on Redis.
Related Article: How to use Redis with Laravel and PHP
Installation of redis-cli
To install redis-cli, you first need to install Redis on your system. The installation process may vary depending on your operating system. Here are the steps to install Redis and redis-cli on different platforms:
Installing Redis on Ubuntu
1. Update the package list:
sudo apt update
2. Install Redis:
sudo apt install redis-server
3. Verify the installation:
redis-cli ping
Installing Redis on macOS
1. Install Redis using Homebrew:
brew install redis
2. Start the Redis server:
brew services start redis
3. Verify the installation:
redis-cli ping
Related Article: Tutorial: Redis vs RabbitMQ Comparison
Installing Redis on Windows
1. Download the Redis for Windows package from the official website.
2. Extract the downloaded package to a directory, e.g., C:\Redis
.
3. Open a command prompt and navigate to the Redis installation directory.
4. Start the Redis server:
redis-server.exe redis.windows.conf
5. Open another command prompt and navigate to the Redis installation directory.
6. Start the redis-cli:
redis-cli.exe
Basic Usage of redis-cli
Once you have installed redis-cli, you can start using it to interact with Redis. Here are some basic commands and their usage:
Connecting to Redis Server
To connect to a Redis server, you can use the following command:
redis-cli -h <host> -p <port> -a <password>
- <host>
: The hostname or IP address of the Redis server.
- <port>
: The port on which the Redis server is running (default is 6379).
- <password>
: The password required to authenticate with the Redis server (if applicable).
Example:
redis-cli -h localhost -p 6379
This command connects to the Redis server running on the localhost at the default port.
Getting and Setting Values
To get the value associated with a key, you can use the GET
command:
GET <key>
Example:
GET mykey
This command retrieves the value stored in the mykey
key.
To set the value of a key, you can use the SET
command:
SET <key> <value>
Example:
SET mykey "Hello, Redis!"
This command sets the value of the mykey
key to "Hello, Redis!".
Related Article: Leveraging Redis for Caching Frequently Used Queries
Data Types and Commands
Redis supports various data types, including strings, lists, sets, hashes, and sorted sets. Each data type has specific commands associated with it. Let's explore some of the data types and their commands:
String Commands and Use Cases
Strings are the simplest data type in Redis. They can store any binary data, such as a JSON string, serialized object, or plain text. Here are some commonly used commands for working with strings:
- SET
: Set the value of a key.
- GET
: Get the value of a key.
- INCR
: Increment the integer value of a key.
- APPEND
: Append a value to an existing string.
Example:
SET user:name "John Doe"GET user:name
These commands store and retrieve the name of a user.
List Commands and Use Cases
Lists in Redis are ordered collections of strings. They can be used to implement queues, stacks, and more. Here are some commonly used commands for working with lists:
- LPUSH
: Insert one or more values at the beginning of a list.
- RPUSH
: Insert one or more values at the end of a list.
- LPOP
: Remove and get the first element in a list.
- LRANGE
: Get a range of elements from a list.
Example:
LPUSH queue "task1"RPUSH queue "task2"LPOP queue
These commands simulate a task queue, where tasks are added to the queue and then processed in the order they were added.
Set Commands and Use Cases
Sets in Redis are unordered collections of unique strings. They can be used to represent tags, followers, and more. Here are some commonly used commands for working with sets:
- SADD
: Add one or more members to a set.
- SREM
: Remove one or more members from a set.
- SMEMBERS
: Get all the members of a set.
- SISMEMBER
: Check if a member exists in a set.
Example:
SADD tags "redis"SADD tags "caching"SMEMBERS tags
These commands demonstrate the use of sets to store and retrieve tags associated with a post.
Related Article: Tutorial: Integrating Redis with Spring Boot
Hash Commands and Use Cases
Hashes in Redis are field-value pairs stored in a single key. They can be used to represent objects, user profiles, and more. Here are some commonly used commands for working with hashes:
- HSET
: Set the value of a field in a hash.
- HGET
: Get the value of a field in a hash.
- HGETALL
: Get all the fields and values of a hash.
- HDEL
: Delete one or more fields from a hash.
Example:
HSET user:id1 name "John Doe"HGET user:id1 name
These commands store and retrieve the name of a user using a hash.
Sorted Set Commands and Use Cases
Sorted sets in Redis are similar to sets, but each member is associated with a score. They can be used to represent leaderboards, rankings, and more. Here are some commonly used commands for working with sorted sets:
- ZADD
: Add one or more members to a sorted set with their scores.
- ZREM
: Remove one or more members from a sorted set.
- ZRANK
: Get the rank of a member in a sorted set.
- ZREVRANGE
: Get a range of members from a sorted set in reverse order.
Example:
ZADD leaderboard 100 "PlayerA"ZADD leaderboard 200 "PlayerB"ZRANK leaderboard "PlayerA"
These commands demonstrate the use of sorted sets to create a leaderboard and retrieve the rank of a player.
Key Commands and Use Cases
Redis provides various commands to manage keys, such as deleting, renaming, and checking existence. Here are some commonly used key commands:
- DEL
: Delete one or more keys.
- RENAME
: Rename a key.
- EXISTS
: Check if a key exists.
- KEYS
: Get all the keys matching a pattern.
Example:
DEL mykeyRENAME oldkey newkeyEXISTS mykey
These commands show how to delete a key, rename a key, and check if a key exists.
Advanced Ways to Use redis-cli Commands
In addition to the basic usage, redis-cli provides advanced features and options to enhance your experience when working with Redis. Here are some advanced ways to use redis-cli commands:
Related Article: Tutorial on Redis Sharding Implementation
Loading Commands from a File
You can load a file containing Redis commands and execute them using redis-cli. This is useful when you have a large number of commands or want to automate tasks.
To load commands from a file, use the following command:
redis-cli < commands.txt
This command reads the commands from the commands.txt
file and executes them.
Using Redis Pipelining
Redis pipelining allows you to send multiple commands to the Redis server without waiting for the response of each command. This can significantly improve the performance when executing multiple commands.
To use pipelining with redis-cli, you can use the -pipe
option:
redis-cli -pipe < commands.txt
This command reads the commands from the commands.txt
file and sends them to the Redis server in a pipelined manner.
Use Cases for redis-cli Commands
Redis and redis-cli can be used in various use cases, including:
- Caching: Redis can be used as a high-performance cache to improve the response time of web applications.
- Session Management: Redis can store and manage user sessions, allowing for easy scalability and fault tolerance.
- Real-time Analytics: Redis can store and analyze real-time data, such as user activity or system metrics.
- Pub/Sub Messaging: Redis provides pub/sub capabilities, allowing for real-time messaging between different components of an application.
Best Practices for Using redis-cli
When using redis-cli, it's important to follow best practices to ensure efficient and secure operations:
1. Use authentication: If your Redis server requires authentication, always provide the password using the -a
option or the AUTH
command.
2. Limit access: Restrict access to the Redis server by allowing connections only from trusted sources and using firewall rules.
3. Monitor performance: Monitor the performance of your Redis server using tools like Redis Monitoring or by enabling Redis slow log.
4. Secure connections: Use SSL/TLS encryption when connecting to Redis over the network to protect sensitive data.
5. Handle errors gracefully: Handle errors returned by redis-cli commands properly to ensure robustness and reliability.
Related Article: Exploring Alternatives to Redis
Real World Examples of Using redis-cli
Here are some real-world examples of how redis-cli can be used:
- Storing and retrieving session data for a web application.
- Implementing a rate limiter to control API usage.
- Caching database queries to improve the performance of a website.
- Implementing a distributed lock using Redis to ensure exclusive access to a critical section of code.
- Implementing a real-time leaderboard for a multiplayer game.
Performance Considerations for redis-cli
When using redis-cli, consider the following performance considerations:
- Network latency: Redis commands executed through redis-cli incur network overhead. Minimize the number of round trips by using pipelining or scripting when possible.
- Command optimization: Redis provides various command optimizations, such as using batch operations and pipelining, to improve performance.
- Connection pooling: Reusing connections using connection pooling can reduce the overhead of establishing new connections for each command.
- Efficient data structures: Choose the appropriate Redis data structures and commands to optimize memory usage and execution time.
Error Handling with redis-cli
Redis-cli provides error handling mechanisms to handle errors returned by Redis commands. When a command fails, Redis returns an error message that can be captured and processed by the client.
To handle errors with redis-cli, you can use the following approaches:
- Check the response: After executing a command, check the response to see if it indicates an error. Redis responses that start with the prefix -ERR
indicate an error.
- Use a scripting language: You can use scripting languages like Python or JavaScript to execute Redis commands and handle errors programmatically.
- Wrap commands in a try-catch block: If you are using a language with exception handling support, you can wrap Redis commands in a try-catch block to catch and handle exceptions raised by the Redis client.
Advanced Techniques with redis-cli
Redis-cli offers advanced techniques that can be useful in certain scenarios. Here are some advanced techniques you can use with redis-cli:
- Lua scripting: Redis supports Lua scripting, which allows you to execute complex operations on the server side. You can use redis-cli to execute Lua scripts using the --eval
option.
- Working with Redis modules: Redis modules provide additional functionality to Redis. You can use redis-cli to interact with Redis modules by executing module-specific commands.
- Customizing output format: By default, redis-cli displays responses in a human-readable format. You can customize the output format using the --raw
, --csv
, or --json
options.
Related Article: Tutorial on Implementing Redis Sharding
Code Snippet Ideas: Connecting to Redis Server
Here are some code snippets that demonstrate how to connect to a Redis server using different programming languages:
Python
import redis# Connect to Redisr = redis.Redis(host='localhost', port=6379, password='your_password')# Perform operations on Redisr.set('mykey', 'Hello, Redis!')value = r.get('mykey')# Print the valueprint(value)
Java
import redis.clients.jedis.Jedis;public class RedisExample { public static void main(String[] args) { // Connect to Redis Jedis jedis = new Jedis("localhost", 6379); jedis.auth("your_password"); // Perform operations on Redis jedis.set("mykey", "Hello, Redis!"); String value = jedis.get("mykey"); // Print the value System.out.println(value); // Disconnect from Redis jedis.close(); }}
Code Snippet Ideas: Getting and Setting Values
Here are some code snippets that demonstrate how to get and set values in Redis using different programming languages:
Related Article: Tutorial on Installing and Using redis-cli with Redis
Node.js
const redis = require('redis');// Connect to Redisconst client = redis.createClient({ host: 'localhost', port: 6379, password: 'your_password'});// Perform operations on Redisclient.set('mykey', 'Hello, Redis!', (err, reply) => { if (err) throw err; client.get('mykey', (err, reply) => { if (err) throw err; // Print the value console.log(reply); // Disconnect from Redis client.quit(); });});
C#
using StackExchange.Redis;public class RedisExample{ public static void Main(string[] args) { // Connect to Redis ConnectionMultiplexer redis = ConnectionMultiplexer.Connect("localhost:6379,password=your_password"); IDatabase db = redis.GetDatabase(); // Perform operations on Redis db.StringSet("mykey", "Hello, Redis!"); string value = db.StringGet("mykey"); // Print the value Console.WriteLine(value); // Disconnect from Redis redis.Close(); }}
Code Snippet Ideas: String Commands
Here are some code snippets that demonstrate how to use string commands in Redis using different programming languages:
Ruby
require 'redis'# Connect to Redisredis = Redis.new(host: 'localhost', port: 6379, password: 'your_password')# Perform operations on Redisredis.set('mykey', 'Hello, Redis!')value = redis.get('mykey')# Print the valueputs value# Disconnect from Redisredis.quit
Related Article: Tutorial on Redis Docker Compose
PHP
<?phprequire 'vendor/autoload.php';use Predis\Client;// Connect to Redis$client = new Client([ 'scheme' => 'tcp', 'host' => '127.0.0.1', 'port' => 6379, 'password' => 'your_password',]);// Perform operations on Redis$client->set('mykey', 'Hello, Redis!');$value = $client->get('mykey');// Print the valueecho $value;// Disconnect from Redis$client->disconnect();
Code Snippet Ideas: List Commands
Here are some code snippets that demonstrate how to use list commands in Redis using different programming languages:
Python
import redis# Connect to Redisr = redis.Redis(host='localhost', port=6379, password='your_password')# Perform operations on Redisr.lpush('mylist', 'item1')r.rpush('mylist', 'item2')value = r.lpop('mylist')# Print the valueprint(value)
Java
import redis.clients.jedis.Jedis;public class RedisExample { public static void main(String[] args) { // Connect to Redis Jedis jedis = new Jedis("localhost", 6379); jedis.auth("your_password"); // Perform operations on Redis jedis.lpush("mylist", "item1"); jedis.rpush("mylist", "item2"); String value = jedis.lpop("mylist"); // Print the value System.out.println(value); // Disconnect from Redis jedis.close(); }}
Related Article: How to Use Redis Queue in Redis
Code Snippet Ideas: Set Commands
Here are some code snippets that demonstrate how to use set commands in Redis using different programming languages:
Node.js
const redis = require('redis');// Connect to Redisconst client = redis.createClient({ host: 'localhost', port: 6379, password: 'your_password'});// Perform operations on Redisclient.sadd('myset', 'member1');client.sadd('myset', 'member2');client.srem('myset', 'member1');client.smembers('myset', (err, members) => { if (err) throw err; // Print the members console.log(members); // Disconnect from Redis client.quit();});
C#
using StackExchange.Redis;public class RedisExample{ public static void Main(string[] args) { // Connect to Redis ConnectionMultiplexer redis = ConnectionMultiplexer.Connect("localhost:6379,password=your_password"); IDatabase db = redis.GetDatabase(); // Perform operations on Redis db.SetAdd("myset", "member1"); db.SetAdd("myset", "member2"); db.SetRemove("myset", "member1"); RedisValue[] members = db.SetMembers("myset"); // Print the members foreach (RedisValue member in members) { Console.WriteLine(member); } // Disconnect from Redis redis.Close(); }}
Code Snippet Ideas: Hash Commands
Here are some code snippets that demonstrate how to use hash commands in Redis using different programming languages:
Related Article: Analyzing Redis Query Rate per Second with Sidekiq
Ruby
require 'redis'# Connect to Redisredis = Redis.new(host: 'localhost', port: 6379, password: 'your_password')# Perform operations on Redisredis.hset('myhash', 'field1', 'value1')redis.hset('myhash', 'field2', 'value2')value = redis.hget('myhash', 'field1')# Print the valueputs value# Disconnect from Redisredis.quit
PHP
<?phprequire 'vendor/autoload.php';use Predis\Client;// Connect to Redis$client = new Client([ 'scheme' => 'tcp', 'host' => '127.0.0.1', 'port' => 6379, 'password' => 'your_password',]);// Perform operations on Redis$client->hset('myhash', 'field1', 'value1');$client->hset('myhash', 'field2', 'value2');$value = $client->hget('myhash', 'field1');// Print the valueecho $value;// Disconnect from Redis$client->disconnect();
Code Snippet Ideas: Sorted Set Commands
Here are some code snippets that demonstrate how to use sorted set commands in Redis using different programming languages:
Python
import redis# Connect to Redisr = redis.Redis(host='localhost', port=6379, password='your_password')# Perform operations on Redisr.zadd('leaderboard', {'player1': 100, 'player2': 200})rank = r.zrank('leaderboard', 'player1')# Print the rankprint(rank)
Related Article: Tutorial on Rust Redis: Tools and Techniques
Java
import redis.clients.jedis.Jedis;public class RedisExample { public static void main(String[] args) { // Connect to Redis Jedis jedis = new Jedis("localhost", 6379); jedis.auth("your_password"); // Perform operations on Redis jedis.zadd("leaderboard", 100, "player1"); jedis.zadd("leaderboard", 200, "player2"); Long rank = jedis.zrank("leaderboard", "player1"); // Print the rank System.out.println(rank); // Disconnect from Redis jedis.close(); }}
Code Snippet Ideas: Key Commands
Here are some code snippets that demonstrate how to use key commands in Redis using different programming languages:
Node.js
const redis = require('redis');// Connect to Redisconst client = redis.createClient({ host: 'localhost', port: 6379, password: 'your_password'});// Perform operations on Redisclient.del('mykey');client.rename('oldkey', 'newkey');client.exists('mykey', (err, exists) => { if (err) throw err; // Print if the key exists console.log(exists); // Disconnect from Redis client.quit();});
C#
using StackExchange.Redis;public class RedisExample{ public static void Main(string[] args) { // Connect to Redis ConnectionMultiplexer redis = ConnectionMultiplexer.Connect("localhost:6379,password=your_password"); IDatabase db = redis.GetDatabase(); // Perform operations on Redis db.KeyDelete("mykey"); db.KeyRename("oldkey", "newkey"); bool exists = db.KeyExists("mykey"); // Print if the key exists Console.WriteLine(exists); // Disconnect from Redis redis.Close(); }}